The Ultimate Guide To Node.js Web Scraping For Enterprise
You might already use web scraping to easily collect a large volume of information from the web in mere minutes to improve your business operations and get an edge over your competitors. However, to make the most out of this practice, you might want to build a customized web scraper that adapts to your needs rather than getting a cookie-cutter tool that will end up throwing out more information — or less accurate data — than you actually need.
Writing your own scraping program could save you time in the long run by providing you with the exact data you’re looking for, and you can tweak it as your business needs evolve. Before you get started, however, you must compare and contrast coding languages and runtime environments to learn which ones are most convenient to use while developing your specific program. One of the most popular options is JavaScript.
JavaScript has certain characteristics that make it drastically different from all other dynamic languages available. While it lacks a thread concept, its model is entirely event-based, making it more efficient for some projects. To take full advantage of JavaScript’s many features, you need to use a server like Node.js. The guide below will help you understand the basics of this runtime environment and the pros and cons of building a web scraper in Node.js. Feel free to use the table of contents to skip to the parts that apply most to your specific needs, depending on your level of familiarity with Node.js.
What is Node.js?
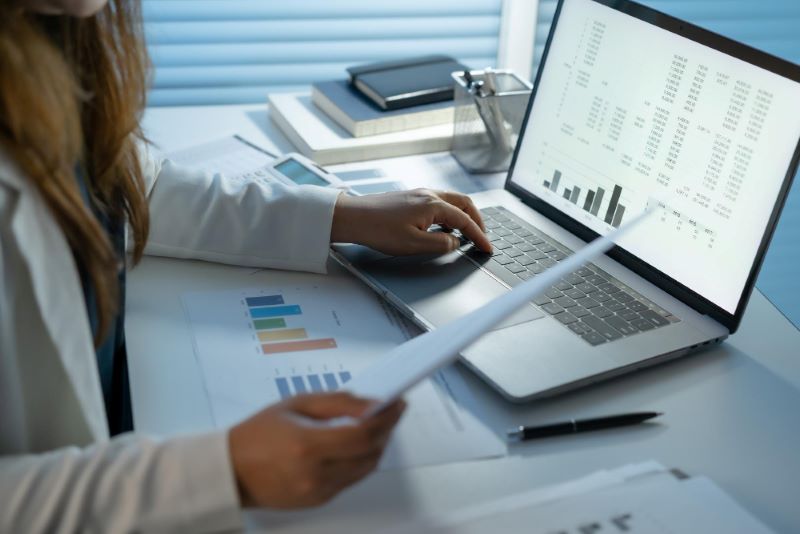
Node.js is a cross-platform, open-source, JavaScript runtime environment, like Apache, IIS, TOM, and many others. However, it doesn’t deal with PHP, .NET, or JAVA. It allows you to execute the programing language on the server side and outside of a web browser. This lightweight tool is popular among beginners, but it’s also used by huge SaaS companies. It’s a common choice to write backend servers and databases because it lets you run your code as a standalone application, rather than one that depends on a browser environment.
Node.js is an excellent tool for data-intensive applications such as streaming and real-time information extraction. It’s important to note, however, that Node.js is not a framework. It simplifies the process of building the code. This server even allows you to use JavaScipt on the front-end and the middleware. That’s what makes it so coveted among web development stacks.
This runtime environment is built on the Chrome V8 JavaScript engine, which takes the programing language and makes it readable. It has a node package manager with over 350,000 packages to easily create numerous projects and applications from scratch. Since JavaScript relies on user interactions to run, Node.js has an event-driven, single-threaded input and output model which allows you to run asynchronous tasks efficiently.
Fundamentals of Node.js
Now that you know what Node.js is, here are the fundamentals of this tool:
Console
This module is similar to the JavaScript console that you find in the browser when you’re inspecting a webpage. The Node.js console has numerous methods you can use for debugging purposes. Some of them are:
- console.log(): This is to log some sort of output.
- console.warn(): This is to explicitly deliver a warning.
- console.error(): This is to produce an error message.
- console.trace(): This is to log a traceback when an error occurs in your code.
Buffer
This temporary storage solution for file systems allows you to allocate memory. It’s low-level in nature, so it’s hardly ever used by more experienced web developers.
File system
This module allows you to interact with files in Node.js. To write or read a file using it, you can use both synchronous and asynchronous methods. However, unlike the buffer class, it requires importing the file system module into each file you’re looking to use.
Event loop
As mentioned before, Node.js is an event-driven server. An event is what happens when you interact with a certain interface and something will happen as a result — like when you click a button or fill up a field in a form. Event loops allow you to attach a function, or a set of them, to a particular event.
Globals
Every module in Node.js has global objects. They can be used without importing the whole deal. Some examples of this are:
- The class in buffers
- The console object
- Timers
- The process object
Pros and Cons of Building a Web Scraper in Node.js
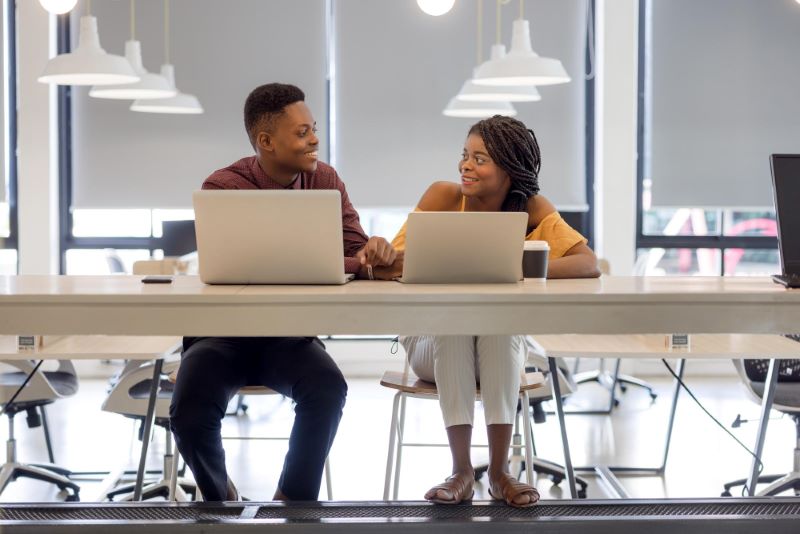
With JavaScript still being so popular, many companies across all industries resort to web scraping using Node.js. Much like all the other options available, this server has numerous advantages as well as some drawbacks. Before moving forward to building a Node.js web scraper, let’s explore the pros and cons of this alternative.
Node.js’s asynchronous and event-driven input and output enables a more efficient request handling than many other options and allows you to stream big files. This server uses JavaScript, which is common and relatively easy to learn. It shares the same piece of code with both the client and the server side. There are numerous node package modules available for simplifying several functions. Node.js has also grown an active community, so it’s easy to find previously written and perfectly functional codes online to use as a reference to programming your own scraping tool.
Why use Node.js?
Node.js has a non-blocking code, which is one of the strongest reasons to choose it as your server. Since it’s fully event-driven, the majority of its codes run based on callbacks. This approach keeps your apps available for other requests instead of pausing the scraping process for inactivity. This server uses the same runtime engine as Google Chrome, which allows for fast processing. This is especially handy when building network applications. Keep in mind that both Node.js and the V8 JavaScript engine are built in C#, making them much faster than other programs, like Python, for example.
Node. js can handle thousands of concurrent connections with close to zero overhead on a single process. Using JavaScript on both your web server and the browser will minimize the incompatibility between programming environments. This will significantly reduce the risk of errors when communicating data structures with JSON, apart from letting you share the validation code between server and client.
Node.js vs. Python
Python is one of the most used programming languages for scraping tools. Let’s see how it compares to Node.js in different aspects.
Architecture
The rules that apply when creating, connecting, and enhancing modules during the developing process are defined by the architecture of computer languages. The event-driven design of Node.js allows for concurrent input and output, and ensures no program stops the thread when a particular process takes place. Node.js uses a single-thread-event-loop architecture instead of generating numerous threads to manage obstructing requests. That’s why it doesn’t require much memory and resources.
Python, on the other hand, needs the help of other tools, like Asyncio, to create asynchronously event-driven apps. However, most popular Python frameworks don’t include such packages. To integrate asynchronous programming into Python’s architecture, you need unique modules to perform unblocking requests and provide input-output capabilities.
Libraries
Programming often requires a set collection of modules with different features that help write new, more effective codes. Libraries with those different modules make coding much easier. Node.js has the Node Package Manager, which is among the largest sources for libraries and packages. The manager is simple to understand and pretty intuitive.
Python uses Pip Installs Python, which is a quick and dependable library and package manager with over 22,000 packages to choose from. The library categories include:
- Data analytics
- Image processing
- Computation
- And more
The Node Package Manager has over 350,000 resources, however, making it the indisputable champion.
Syntax
Python is known for having straightforward syntax. That’s perhaps one of its biggest strengths. It requires developers to use fewer lines when coding, unlike Node.js. This is particularly useful for people who don’t already have a technical skillset.
The syntax in Node.js and Python is pretty similar, though. This is good news for developers who are experienced in Python and are trying to learn their way around Node.js back-end development.
Performance
The speed and performance of a computer language are affected by the responsiveness of the client’s requests. Node.js’s speed is outstanding thanks to the V8 JavaScript engine. Nodejs/s processes don’t require the browser, which allows the app to consume fewer resources — thus improving its performance. The browserless coding feature of Node.js also lets you use technologies like TCP sockets and process multiple requests at the same time. This speeds up the code execution and app loading. Python is a single-flow language, which makes request handling a lot slower than Node.js.
Scalability
When creating a web scraper, you want it to accommodate a large number of uses without crashing. That’s why you need the possibility to make space for improvements and updates. Node.js doesn’t require you to build a massive unified core. Instead, you create a collection of modules that run their own processes communicate via a compact system. This flexible model allows you to introduce new microservices quickly when needed.
Python uses the Global Interpreter Lock (GIL), which doesn’t allow multithreading, even when this language contains multithreading libraries. The GIL also prevents numerous threads from running at the same time. This allows for little to no scalability.
Universality
With Node.js you can use JavaScript for both front and back-end development. This allows you to handle web pages and apps with ease across a variety of devices and operating systems. Python is also a full-stack, cross-platform programming language, meaning even a Python program created on Windows will run on Mac or Linux.
While Python works seamlessly on Linux and Mac, you need to set up a Python interpreter if you wish to use it on Windows. This coding language is great for computer development but still has a long way to go in mobile devices. That’s why most mobile apps are not built with Python.
Popular Node.js Applications
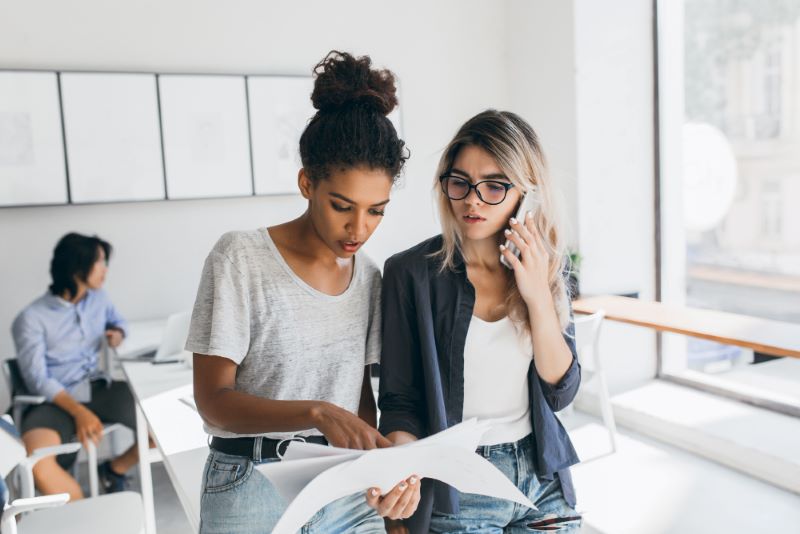
Node.js can be an excellent solution in numerous applications. Here are a few examples in which using it may be the best idea over others.
Data streaming
Node.js can easily be used for streaming data flow as it deals with callback concepts. This last concept refers to a function passed into another one as an argument, which is then invoked in the outer function to perform an action or routine. This process is particularly useful for the travel or events industry, in which businesses fetch results from numerous application programming interfaces (APIs) from various providers.
Web socket servers
Sockets are typical flows of events used for client-server interactions. The non-blocking nature of Node.js makes it the best match for broadcasting apps and socket server programs. This is particularly useful for making chat servers more efficient.
Stock exchange software
The stock market moves quickly, so when trying to extract information about stocks and ETFs, you need to act fast. That’s why a financial web scraper built in Node.js is incredibly useful. It can help you collect information in real-time and keep it up to date.
Ad servers
Advertisers want their content to load as quickly as possible. They only have a few seconds to grab their audience’s attention, so slow loading times can significantly affect their possibilities to impact their target. Node.js is a lightweight solution that allows ads created on it to load a lot faster than other much heavier elements on a site.
Building a Node.js Web Scraping Tool
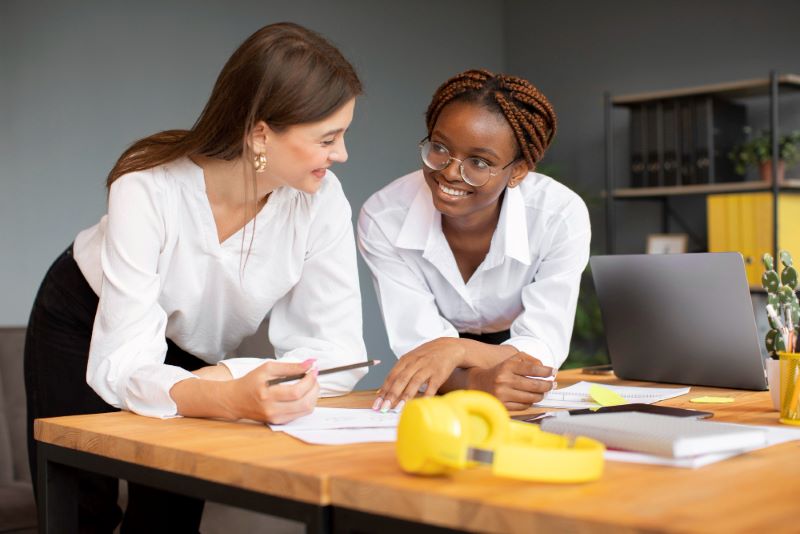
Before you put your programming skills to the test, you need you’ll do some prep work. To build an effective crawler, you must be clear on your information targets. In other words, you need to choose the data you want to collect. Remember, you can scrape anything that’s publicly available — which can include near-endless information. You’ll save a lot of time if you narrow down your possibilities. If this is your first rodeo, target a simple data set to begin and go from there.
Once you know what types of sites you want to scrape, study how they’re structured. No two websites are built exactly the same, and although they may have similar elements, they can use numerous names to differentiate them or even change layouts between pages. Keep an eye out for patterns you can use in your favor while programming your scraping tool.
With a clearer concept of what the Node.js server is all about and what you want to achieve with it, you can start working on your very own spider to crawl the web. Here’s a step-by-step guide on how to make a scraper with Node.js.
1. Prerequisites
Before you get started, you’ll need to download Node.js. You’ll also need a source code editor, like Atom or Virtual Studio Code, to write your program. A little understanding of JavaScript is also extremely helpful. While you can still follow along with this tutorial if you don’t, knowing the basics of this language and Node.js will certainly expedite things.
2. Installation
Once you’ve downloaded the Node.js files from their site, all you’ll need to do is follow the installation prompts. If you’re a bit more experienced, you could also use a package manager to finish the installation process. To test that it’s working, print the version using the following command:
> node -v
You’ll also need to install any dependencies you might be using later. The most common are:
- Axios: This is a popular HTTP client that performs Node.js HTTPS requests.
- Cheerio: This is a parser tool.
- Pretty: This is a node package manager application for making a markup readable.
3. Creating a working directory
This step should allow you to see a folder with the name you choose. If you’ve created it successfully, open your directory in your favorite text editor and initialize your project.
4. Creating a file
When you’ve properly installed Node.js into your machine, you can proceed to create a Node.js file. You can name it however you want, like “spider.js”, for example. To save the file on your computer, you’ll need the following code:
C:\Users\Your Name\spider.js
Navigate to the folder that contains your file. To initiate a command-line interface Node.js file, you’ll need to use your command-line interface program. Start it by writing node spider.js and pressing enter like so:
C:\Users\Your Name>node spider.js
This will allow you to set up your computer as a server. Open a new text file and write a function to access the HTML of the site you’re trying to scrape.
5. Using DevTools
Once you’ve got the raw HTML of the site, you can search through it with Chrome DevTools. Open the browser and right-click on the element you want to scrape. Next, click “inspect” for Chrome to place it on the DevTools pane for an easier inspection. What you see here is the type of tag you should be looking for in the site’s code. This will help you scrape similar elements.
6. Parsing HTML
For effective Node.js page scraping, request the dependencies you installed earlier in your node package. You’ll need them for Node.js to parse the URL you’re extracting data from.
You can now use Cheerio.js — a library in Node.js that helps you interpret and analyze web pages with a jQuery-like syntax — to parse HTML. Once you identify the syntax of the code you want to parse using DevTools, you can extract the information you need with Cheerio. To make the most out of this dependency, you can use it to:
- Load Markups: To do this, you’ll need the cheerio.load method, which takes the markup as an argument. You can execute the code in your app so you’re able to see the markup in the terminal.
- Select an Element: This dependency supports class, ID, element, and other common CSS selectors. You can use code to select an element and log it into the console. This will allow you to see the text you need on the terminal when you execute the command on your directory.
- Get an Attribute: Cheerio allows you to select specific attributes of an element and its corresponding values.
- Loop Through a List of Elements: There’s a .each method in Cheerio that allows you to loop through numerous items you’ve previously selected.
- Append or Prepend an Element to a Markup: The append method provided by Cheerio will add an element passed as an argument after its last child node, while the prepend will add the pass element before its last child node.
7. Exporting your data
Once you’ve successfully written your code and collected all your data, it’s time to export the results onto a file in your favorite output language. You could use JSON, CVS, XML, or your other preferred kind.
Why Use Cheerio.js in Your Scraping Program
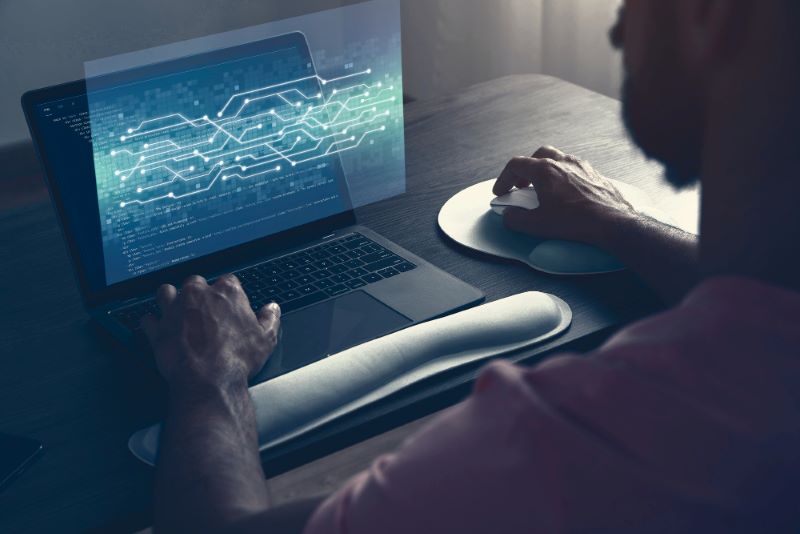
With over 24K stars in GitHub, Cheerio.js is a popular HTML and XML parsing tool in Node.js. This dependency is known for being flexible, fast, and straightforward to use since it incorporates a subset of JQuery. Apart from parsing markup, Cheerio offers an API for manipulating data structure. However, it cannot interpret the results like your average web browser.
Cheerio doesn’t produce visual rendering as web browsers do. It also doesn’t load external resources or execute JavaScript. All it does is parse code incredibly fast. Paired with packages like Node-Fetch and Axios, Cheerio is an excellent tool for scraping a web page. This JavaScript technology perfectly complements your scripted methods of extracting data from the web and allows you to tailor your program based on your needs.
Cheerio.js’s usage
Cheerio lets you identify elements on a webpage and examine the collected information based on your particular use case. Once you have the data you need, you can treat it as you would any object in a programming language, meaning you can count instances of a particular object, loop through them, and extract the information you’re looking for with ease.
Best Proxies To Use for Web Scraping With Node.js
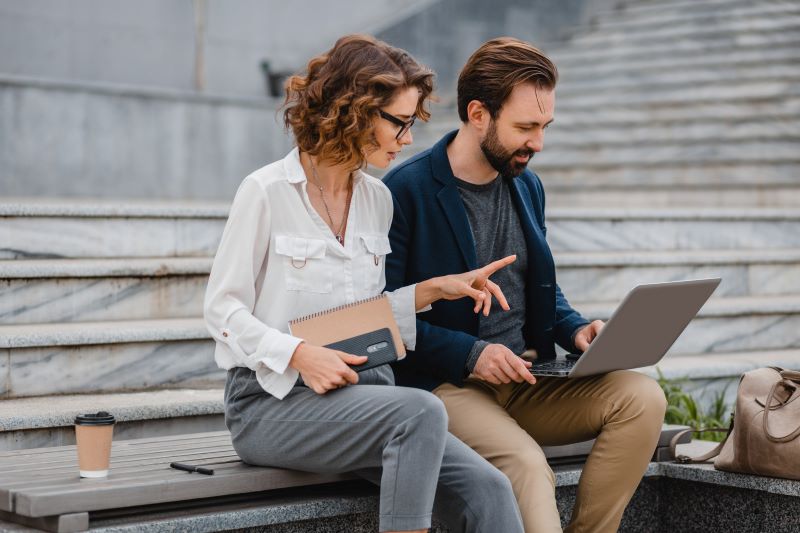
For many reasons you probably already know, web scraping is incredibly useful for any business owner trying to leverage the power of data. However, not all sites are happy having bots extracting their information. At the end of the day, it’s hard for admins to distinguish between genuine researchers and malicious actors, and they’re not going to stop and ask which one you are.
Many websites have implemented anti-scraping tools in an attempt to reduce the risk of having their data stolen or falling victims to hacker attacks. These measures include CAPTCHAs, IP bans, honeypot traps, and more. To circumvent these challenges while web scraping, you must avoid raising any red flags while collecting your data. That’s where a proxy can come in handy.
Not only do proxies help you look less bot-like to the eyes of website managers, but they also keep your machine a lot safer from the threats of the internet. They can also help you dodge geo-location restrictions and send numerous requests in a shorter amount of time than you could with a single proxy. However, keep in mind that not all proxies are a safe enough alternative.
You might feel tempted to save your hard-earned cash by seeking free, public proxies to perform your web scraping duties. While this might work (initially), you could be jeopardizing your information and your network’s security. One problem with public proxies is that because they’re shared with many other people, you have no idea or control over what sites and methods others are accessing on the same IPs. This puts you at risk of getting blocked or banned — and unable to gather the data you need. Moreover, free proxies are less likely to have a dedicated team to back you up in case something goes wrong.
Choose rotating residential proxies
Your best bet is to purchase your proxies from a trustworthy provider. Make sure to assess your options and look for one that allows you to emulate the real-world operations of flesh-and-blood internet users. After all, you want your scraping tool to look as human as possible when interacting with a site’s interface. In this case, Rayobyte’s rotating residential proxies are the best choice.
Rotating residential proxies offer IP addresses that look like they come from real users in their homes or offices. Since they are constantly changing, sites don’t have time to hone in on one particular IP address. This gives your scraping tools the ability to handle numerous requests at the same time while appearing to do so from different locations. All this keeps your identity and reputation safe and makes it much harder for websites to ban you.
With our rotating residential proxies, you can be sure all your IPs are ethically sourced. What’s more, you’ll scrape the web with peace of mind knowing you have the support of our experts if ever a server’s down. You can also take advantage of our free Proxy Pilot proxy management application, which comes included with all of our proxies — but you also can use it on its own. This helpful tool can be easily built into your Node.js web scraping tool and will help you handle retries, detect bans, handle cooldown logic, and more.
Common Web Scraping Challenges
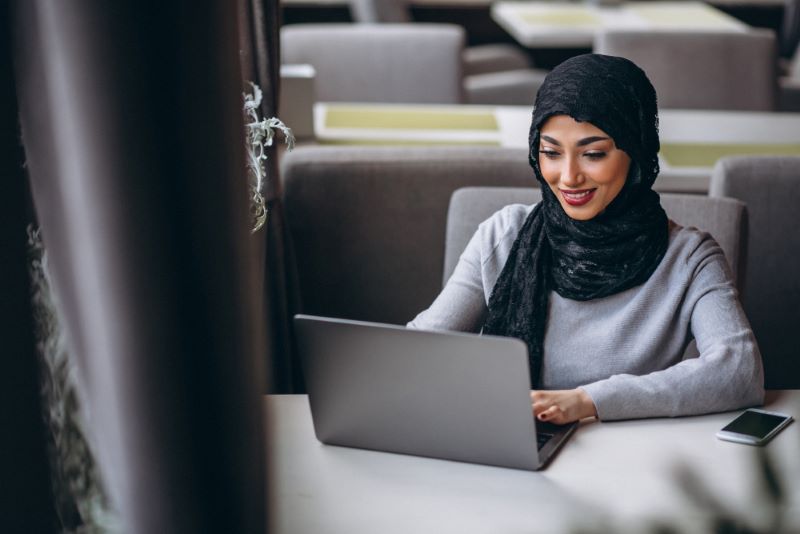
Whether you use a pre-built web scraper or choose to program your own, you can encounter numerous challenges while trying to collect data from the web. The internet evolves constantly, and new errors may appear over time — whether it is from new anti-scraping measures or code-related elements. These are the most common web scraping problems you can encounter while extracting data nowadays.
Honeypots
Some sites set up traps to identify bots and stop them on the spot. Honeypots are the most common and they’re typically hidden behind invisible CSS elements. Humans cannot see them, and therefore are unable to click on them. However, scrapers can find them in a heartbeat and are more vulnerable to their effects. To successfully avoid honeypots, program your spider to ignore certain CSS tags, like “hidden”.
Authentication
The site you want to scrape might require you to log in with certain credentials before you can access the information you’re looking for. It might look suspicious if you submit the login form numerous times while sending your requests for each site. Luckily, you can resort to using some commands to avoid logging in on every page.
A pro tip is to use developer tools to see what the server is requesting before scraping a site. This will give you a clearer idea of what type of command you’ll need to program into your bot.
CAPTCHAs
Even if you’re not an avid internet user, you’ve probably stumbled upon one of these puzzles at least once. CAPTCHA stands for Completely Automated Public Turing test to tell Computers and Humans Apart. They can require you to identify a series of images, rewrite a distorted code, or simply check on a box to prove you’re not a bot. To bypass them, you’ll need to write a program that can beat the test or hire captcha solving services.
Redirects
Some sites might try to redirect your bot. In that case, you can use the RSelenium library, which works with the Selenium tool to help you follow any potential redirects web admins can throw at you.
JavaScript elements
Numerous sites use JavaScript to implement enhanced features like infinite scrolling into their sites. While that can be attractive and convenient to real users, it could represent a challenge to your scraping bot. To prevent your spider from not being able to extract much information from the HTML of the site because of these added features, you can use tools like PhantomJS and a headless browser. This way, you can load and convert the information so that your program can read and interpret it.
Robotic behavior
A bot that’s too obvious in its behavior is certain to trigger a site’s anti-scraping mechanisms. After all, these types of programs look for suspicious signs, like clicking too fast or constantly trying to open the same links. You’ll need to slow down your request speed and use proxies and a unique user agent to make your interactions look as natural as possible, This way, you’ll be less likely to get caught.
Poorly structured HTML
Web scrapers might come across messy sites with unstructured HTML. If the site you’re trying to crawl uses CSS classes and attributes on the server side, you might have trouble accessing the data you need. The same goes for poorly designed sites that don’t follow a specific pattern. Although it may be a little time-consuming, you could try scraping one page at a time to avoid errors.
Best Practices and Tactics for Web Scraping with Node.js
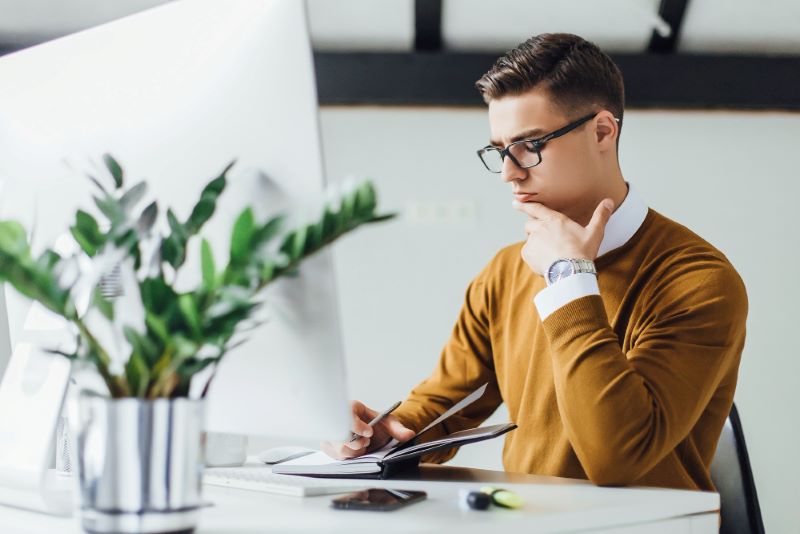
As mentioned above, web scraping is typically frowned upon by many sites, especially those that handle sensitive data. Unfortunately, cybercrime is on the rise, and websites of all types need to protect their information from any suspicious activity. While the need for information from the web to advance in business is understandable, you need to keep your web scraping activity ethical. Here are some suggestions to make your web crawling activity with your Node.js scrapper as successful as possible.
Respect the robots.txt file
Webmasters create very specific instructions for robots to follow while crawling and indexing pages on their sites. This text file is called robots.txt, and contains all you need to know to respectfully extract data from a website. Before you even start programming your Node.js web scrapper, you should always look at this file. It will tell you what’s off-limits, determine the frequency interval of your requests, or even stop you on your tracks and tell you to abort the mission. If a site explicitly states that web scraping is forbidden, always listen. You don’t want to have any legal issues.
Space out your requests
We cannot stress this enough. To successfully scrape the web, your must bots look like real-life users. Sending numerous requests at the same time — even when using a proxy — will raise a red flag. Humans wouldn’t send 1,000 inquiries in a matter of seconds. Besides, sending many requests in a short time might overwhelm the site’s servers and slow down their loading times, or worse, make the website crash. Affecting the user experience might make them lose clients, and ultimately, revenue.
If a site is allowing you to extract the information you need, be considerate and respect the interval they’ve established on the robot.txt file. If they don’t have a clear limit, a good rule of thumb is to delay your requests by 10 seconds.
Rotate your user agent
Each request you make while web scraping has a unique user agent string in the header. This allows sites to identify the browser you’re using along with the version and other relevant information. Much like an IP, it essentially lets sites know if the same person is behind a certain pattern. Using the same user agent in every request your scraper makes will immediately give you away. To avoid this, try rotating the user agent between requests every now and then. Look for legitimate user agent strings online and try them in your program. Alternatively, use tools with a user agent rotation property, like Scrapy.
Disguise your requests
As mentioned above, using rotating proxy servers is one of the best alternatives you have to hide your identity and make your requests look more natural. Constantly changing your IP address will make it seem like your requests are coming from various users in different locations and make it harder for sites to catch up with your scraping tasks and block you.
Switch crawling patters
Robots are routinary by nature. If you leave your robot alone, it will do the same thing over and over again, alerting anti-scraping technologies and making it easier for you to get caught. Humans wouldn’t act like this. We’re a lot more unpredictable and tend to showcase a different behavior every time. To avoid making a site’s security team too suspicious, make sure to implement different actions to break your bot’s patterns. This can include things like clicking random links, making different mouse movements, and so on.
Scrape during off-peak hours
One of the biggest fears sites have when it comes to web scraping is having their serves saturated. If a site crashes, it impacts user experience and might affect a merchant’s reputation to the point where they lose sales and money. You can help your favorite sites by having your robots scrape during times when a site’s traffic is significantly lower. This way, your bot can send a decent amount of requests without harming the site.
Use scraped data responsibly
If you’re extracting data from any site, it should be for your eyes and your eyes only (this may also include members of your team). You should always abide by ethical practices when collecting information that doesn’t inherently belong to you. By no means are you allowed to republish the information you obtained, especially when trying to make a profit out of it. You can fall into copyright infringements and get in serious legal trouble. Always check the site’s terms of service to ensure you’re not breaking the rules while scraping.
Avoid duplicate URLs
If you scrape the same page twice, you’ll end up with duplicate data. Some sites use different URLs for sites that hold the same information. For each duplicate URL, however, there must be a canonical URL that displays the original page. With the help of some frameworks, you can identify duplicate URLs by default to avoid extracting data you already have.
Conclusion
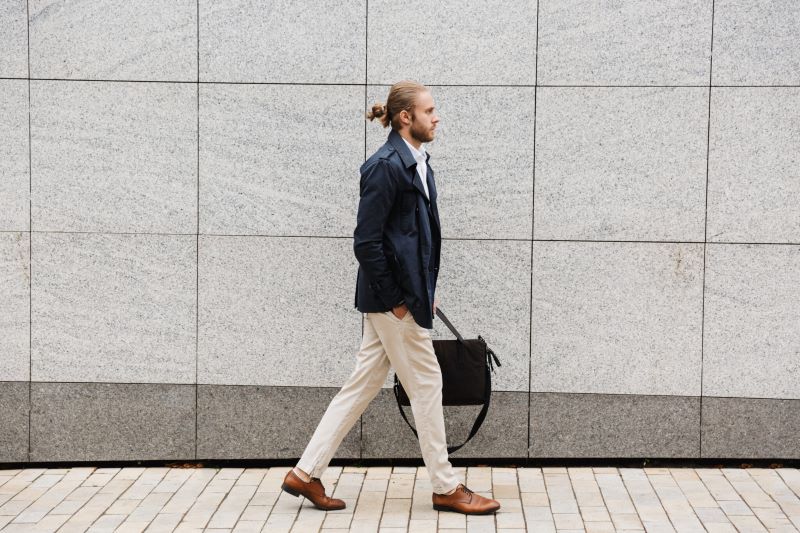
There are numerous programming languages and servers to build effective web scraping tools. Before you pick one, however, you should always make sure it’s convenient and easy to use — especially if you’re a beginner or have no coding experience. Node.js is an excellent alternative to create a lightweight, fast, and straightforward app. The guide above will help you learn the basics of this JavaScript tool and build a spider to extract relevant information for your business in no time.
Remember that to ensure a successful web scraping experience, you should always use proxies to protect your identity and make your interactions with the web look more humanlike. If you’re ready to start your Node.js web scraping journey, visit our site and explore the many products we have to suit your specific needs.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.