Perl Web Scraping Tutorial: Useful Libraries And Modules
Few programming languages are as suitable for web scraping as Perl. But first, what is Perl, exactly?
The powerful and versatile Perl programming language is designed for text manipulation and system administration. It’s used to create programs that can process data quickly and efficiently, allowing users to automate tedious tasks. Perl stands out because of its flexibility: it’s easy to learn the basics but also has advanced capabilities like web scraping.
Both Perl and the hugely popular Python are excellent languages for web scraping. When it comes to Python vs. Perl for web scraping, the former is a great choice for beginners because of its simple syntax, while experienced coders enjoy the flexibility the latter offers. But when it comes to speed, Perl is usually faster — making it the ideal language when you need to process large amounts of data quickly.
So this Perl web scraping tutorial will focus on the libraries and modules that this specific programming language can use for the task. If you’re more interested in Python, however, you can start with our Python requests and proxy use guides.
Popular Tools for Perl-Based Web Scraping
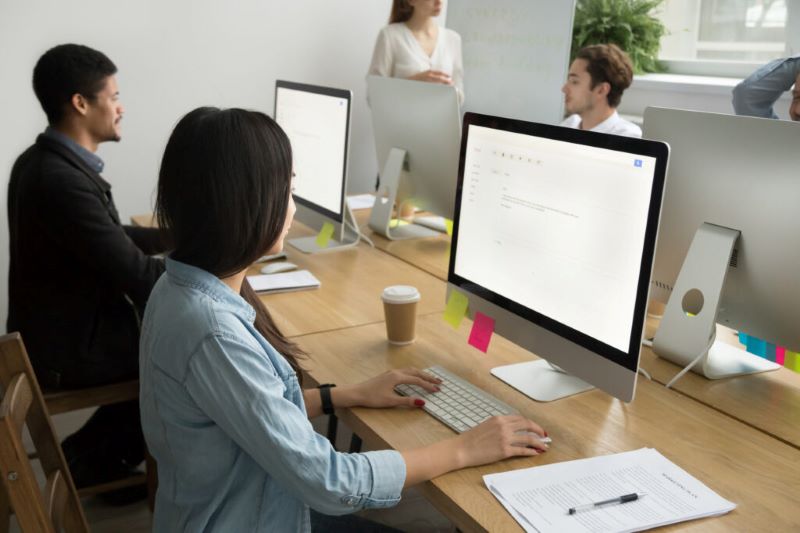
The three most prominent Perl web scraping libraries are WWW::Mechanize, HTML::TreeBuilder, and Selenium. These provide an easy-to-use interface for automating user actions on websites, such as filling out forms, clicking buttons, and navigating through pages. They also offer convenient methods like GET, POST, PUT, and DELETE, making it much easier to construct HTTP requests automatically. Properly automating tasks with these tools can also reduce human error in the data collection process.
But web scraping with Perl isn’t without its challenges. Some websites may have security measures that prevent automated access or even detect it the moment it starts, so careful consideration must be taken when using the approach for website access or manipulation. Furthermore, since no two websites are exactly alike in structure and content delivery methods, customizing a script to scrape each site can become complicated very quickly if not done correctly from the start.
An experienced user is recommended for undertaking such tasks with Perl scripts designed explicitly for this purpose. However, you can find a lot of support from online communities and forums that offer tutorials, knowledge bases, troubleshooting tips, and more.
Using Perl and WWW::Mechanize for Web Scraping
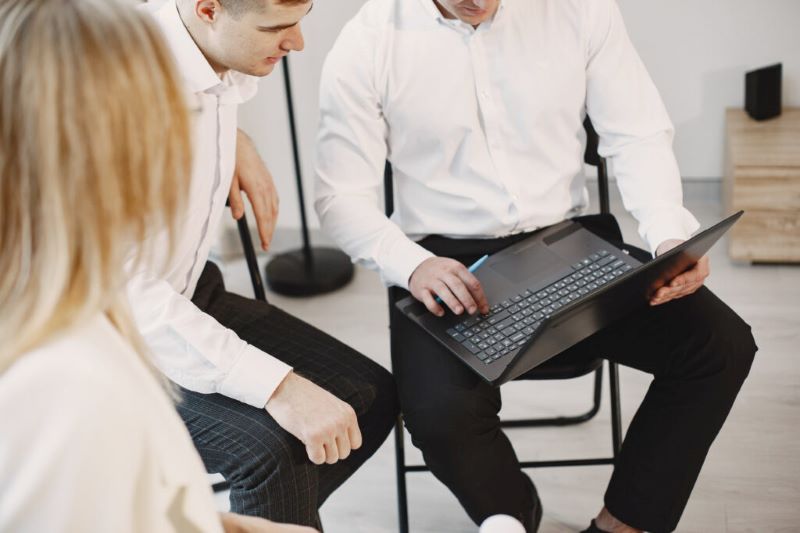
WWW::Mechanize is a Perl module that enables users to create and control web browsers. It can be used for a variety of tasks, including creating a web scraping crawler in Perl, submitting forms, following links, and downloading content. WWW::Mechanize provides methods for navigating the DOM (Document Object Model) and retrieving data from HTML tables or AJAX requests.
Using WWW::Mechanize in conjunction with CSS selectors or XPath expressions makes it easy to extract structured data from any website quickly and accurately. Additionally, its built-in support for cookies allows you to access websites that require authentication without having to manually log in each time — a huge time saver! All these features make WWW::Mechanize an ideal choice when automating web activities with Perl scripts.
Advantages of WWW::Mechanize
WWW::Mechanize offers several advantages that make it especially well-suited to web scraping:
- Automation: WWW::Mechanize automates the web scraping process, allowing you to gather data with minimal effort. This makes it much easier and faster than manually extracting information from websites yourself.
- Easy interaction: WWW::Mechanize simplifies the interaction between software programs and websites, making it easy to retrieve data programmatically without writing complex code or using specialized libraries for each website. With this module, users can control a virtual browser as if they were controlling an actual one.
- Comprehensive support: In addition to its automation capabilities, WWW::Mechanize provides comprehensive support for HTML forms submission (GET/POST), cookies handling, HTTP headers modification, proxy server usage, and more. This support increases accuracy when retrieving large amounts of web content in a single pass, ensuring high-performance results every time.
- Customization: As mentioned earlier, its ability to make adjustments on both the server and client sides allows users greater flexibility when dealing with different types of pages. This enables customized solutions that best suit individual needs depending on the type of information extracted from any given page or site structure encountered.
- Extensibility: Last but not least, thanks to its extendable architecture, developers can add new functionalities in addition to default ones by implementing plugins or adding overriding custom methods. This is especially handy when working with more complicated scraping efforts requiring advanced features.
Installing and using WWW::Mechanize
To install WWW::Mechanize, open a terminal window and type “cpan” to enter the CPAN shell. Then type “install WWW::Mechanize.” This will download and install all necessary files to begin web scraping with Perl using this module.
Once installed, you can start writing code in Perl that uses WWW::Mechanize for web scraping tasks. Include the following line at the top of your script:
use WWW::Mechanize;
Alternatively, you can enter the following full command in the shell:
perl -MCPAN -e install WWW::Mechanize
Once installed, you will be able to access all of Mechanize’s methods, such as get(), post(), submit_form(), and others:
- get(): Retrieves content from a specified URL and stores it in an object, allowing you to access the HTML code or other data associated with that page.
- post(): Sends POST requests to web pages, which can be useful for submitting forms or other data that may not be accessible through the get() method.
- content(): Saves all scraped content in a scalar variable for later use.
- click(): Simulates clicking on a link or button within the page loaded by WWW::Mechanize without having to manually do so each time.
- submit_form(): Fill out and submit forms on websites, which can be helpful for logging into accounts or submitting search queries as part of your scraping process.
- submit_form_ok(): This is similar to submit_form(), but it also checks whether the form submission was successful before further processing.
- follow_link(): Click links within pages that have been loaded by WWW::Mechanize so that they open up in new tabs or windows automatically.
- follow_link_ok(): Similar to follow_link(), but will also check if following the link was successful before proceeding with any further processing in your script, which can help ensure all of your requests are handled correctly and efficiently.
- find_all_links(): Locates all links on a given page. It will return them as an array reference which makes them easy to work with programmatically when writing scripts using Perl and WWW::Mechanize.
Here’s an example of how to grab the content from a dummy knowledge base article:
First, create an instance of WWW::Mechanize and get the page:
my $mech = WWW::Mechanize->new(); # Create instance of Mechanize object
$mech->get(‘http://example.com/knowledge-base’); # Get page contents from URL
Then use the content() method:
my $page_content = $mech->content; # Save all HTML as scalar variable
Finally, use Perl regular expressions (regex) to extract only the desired information from within that scalar string. For example, if we wanted just the title and body text of our KB article, we could do something like this:
If ($page_content =~ m/<h1>(.*?)<\/h1>/) { #, look for opening <h1> tag with regex.
my $title = “$1”; # Capture everything between tags into var
} else {
die “Title not found!”
}; # Exit script if no title is found
If ($page_contents=~m/<p>(.*?)</p>) { #, look for opening <p> tag with regex.
my @bodytext=split /\n/,$2; # Split body into individual lines
foreach (@bodytext){
print $_.”\n”
}; # Print each line on its own line
} else {
die “Body Text not found!”
}; # Exit script if no body text is found
Using Perl and HTML::TreeBuilder for Web Scraping
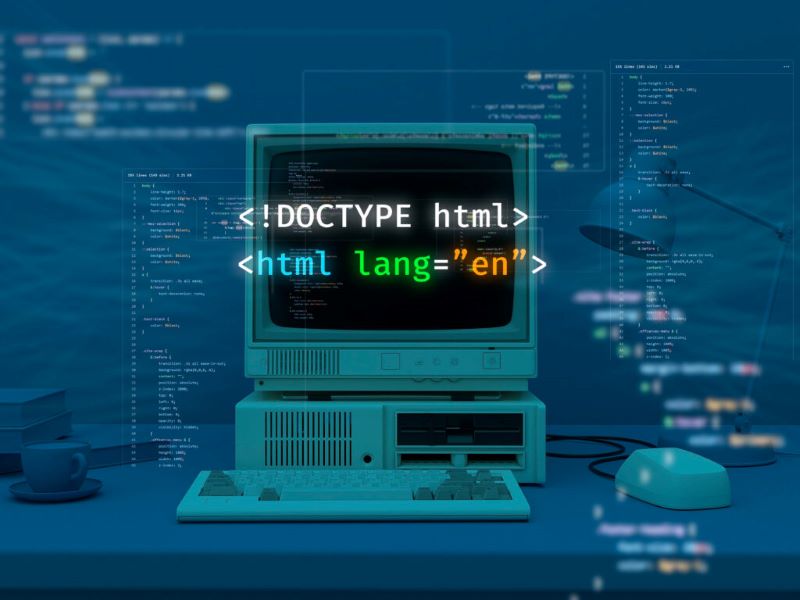
HTML::TreeBuilder is a Perl module that makes it easy to parse HTML documents and extract data from webpages. It provides methods for traversing, searching, and manipulating an HTML document tree with simple syntax.
With this powerful tool, you can quickly scrape websites for content or automate tedious tasks like form completion without coding complicated regexes or writing long-winded scripts. The module offers robust support for tag attributes as well as a range of convenient manipulation functions such as insert(), delete(), cut(), and paste().
Advantages of HTML::TreeBuilder
HTML::TreeBuilder offers the following advantages:
- Easy to use: With its intuitive syntax and thorough documentation, it is easy to learn and start using quickly. Furthermore, users can easily access methods that help them navigate through elements of a page’s structure, like links or images, without having knowledge of HTML or CSS selectors.
- Flexible: Users can customize their approach to get exactly what they need from webpages by parsing out specific data points, such as text content or attributes like class names, without having any prior experience with DOM APIs. This makes it perfect for web scraping projects ranging from simple tasks, such as finding contact information on one webpage, to complicated ones with multiple steps for extracting data from many pages at once.
- Scalable: The module uses an efficient tree-based representation that helps keep memory usage low even when dealing with large datasets consisting of hundreds, if not thousands, of pages, making sure scripts run smoothly no matter how much information needs to be extracted.
With all these combined features, HTML::TreeBuilder provides developers with an effective way to quickly create reliable web scraping solutions while avoiding headaches caused by writing custom code every time you need something extracted from websites.
Installing and using HTML::TreeBuilder
Installing HTML::TreeBuilder is straightforward. First, make sure you have Perl installed on your computer. Then open a terminal window and type:
cpan install HTML::TreeBuilder
Alternatively, you can install manually directly from the command shell with the line:
perl -MCPAN -e ‘install HTML::TreeBuilder’
This will download the module from CPAN and install it for use in your Perl scripts.
HTML::TreeBuilder provides several methods that can be used to extract data from HTML documents. Here are the most important ones:
- parse_file(): Reads an HTML file and builds a tree structure of the document’s elements, allowing you to traverse it and extract information as needed.
- look_down(): Searches through the tree structure created by parse_file(), looking for specific tags or attributes with given values. Use this to quickly find what you’re looking for in an HTML document.
- elementify(): Takes a list of nodes found using look_down() and turns them into objects with additional methods that make extracting data easier than working with plain text strings or DOM nodes alone.
- as_text(): Once you’ve identified some elements using elementify(), this method will return their contents as plain text, making it easy to manipulate them further if necessary before storing them in your database or outputting them elsewhere on your website/application.
- delete(): Deletes a node from the tree structure created by parse_file(). It’s useful for removing unwanted elements or attributes before extracting data.
- look_up(): This is similar to look_down() but searches up the tree instead of down, allowing you to find parent nodes and their associated information more easily.
- dump(): If you need to debug your code while web scraping, this method will print out a representation of the current state of your HTML document in an easy-to-read format so that you can quickly identify any issues or problems with how it’s being parsed and manipulated.
Here’s an example of how to use HTML::TreeBuilder by scraping a dummy webpage for knowledge base articles:
use strict;
use warnings;
use LWP::Simple;
use HTML::TreeBuilder 5 -weak;
my $url = ‘http://www.examplewebsite/knowledge-base’;
my $content = get($url); die “Unable to get $url” unless defined $content;
my $tree=HTML::Treebuilder->new(); # Parse the content
$tree->parse($content); # Extract all <h2> elements
my @headings=$tree->look_down(‘_tag’,’h2′); foreach (@headings){ print $_,”\n”; }
The strict and warnings pragmas force the declaration of variables and enable warning messages, respectively. These will help make the program less prone to errors by catching any mistakes and typos early.
Then it imports LWP::Simple — a suite of language functions and classes for HTTP requests — and a version of HTML::TreeBuilder. The URL of the page is stored in $url, which is used with get() to retrieve its contents into the variable $content. This is then parsed using Treebuilder’s parse() method before look_down() extracts all <h2> elements into an array called @headings that can be printed out line-by-line using a foreach loop.
Using Perl With Selenium for Web Scraping
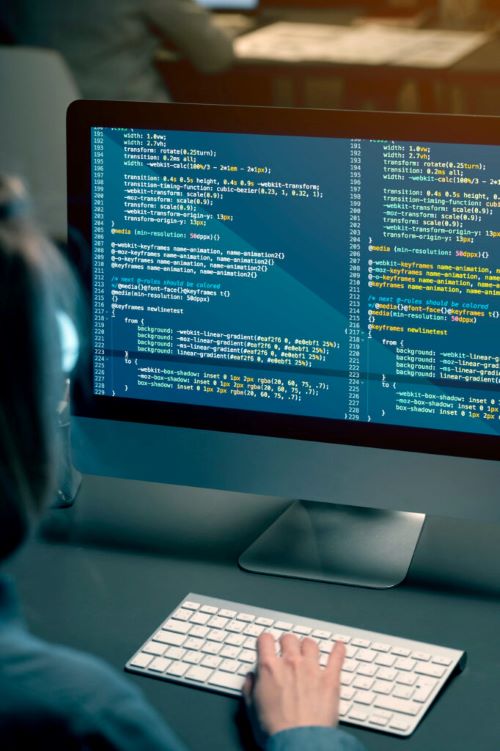
Selenium is a powerful tool for automating web browsers. It can create scripts that run specific tasks in the browser, such as clicking buttons or filling out forms. Scraping with Selenium and Perl is much like using Perl’s own modules, WWW::Mechanize and HTML::TreeBuilder, except it’s a third-party alternative. Selenium works with a variety of programming languages, and it’s a good idea to use it for web scraping if you’re already using it for other web browser automation tasks (or you’re planning to).
Selenium::Remote::Driver is the module you’ll need to use for web scraping with Perl and Selenium. This library provides an API for automating interactions with websites using the Selenium WebDriver.
Advantages of Selenium
Selenium offers numerous advantages when used alongside Perl for web scraping:
- Cross-platform capability: Selenium works on multiple platforms, including Windows, macOS, and Linux. This makes it easy to set up web scraping tasks using Perl no matter what environment you’re working in.
- Easy integration: Integrating Selenium into existing projects written with Perl is easy due to the vast number of libraries available for this purpose. Additionally, Selenium’s open-source nature means there are plenty of resources online to help get you started.
- Flexibility and automation: With Selenium powering your web scraping with Perl, you’ll be able to automate data extraction processes and maintain more flexibility when dealing with dynamic websites or content changes. It supports multiple browsers (e.,g Chrome, Firefox) and can work around many common problems, such as popups or authentication issues, without needing manual intervention every time.
- Open-source nature: Selenium is open-source software, meaning anyone who wishes to can contribute new features or bug fixes that could benefit everyone. This also ensures the tool remains up-to-date even years after initial development ceased (the original dev team only performs maintenance on Selenium 2). Furthermore, many third-party companies offer support services if needed by users facing complex problems regarding their usage.
Installing and using Selenium::Remote::Driver
You can install this module by using these statements:
cpanm Selenium::Remote::Driver
Or
cpan Selenium::Remote::Driver
Some of the most notable methods in this module include:
- get(): Opens a new browser window and navigates to the specified URL.
- find_element(): Locates an element on the page by its ID, class name, tag name, or XPath expression. It returns a Selenium WebElement object that you can interact with using other methods in this module, such as click() and send_keys().
- execute_script(): Executes JavaScript code within the current page context and returns any values returned by that script execution (if applicable). It’s useful for manipulating elements on the page or extracting data from them without having to parse HTML directly yourself.
- screenshot(): Takes a screenshot of what is currently displayed in your browser window. It will return an image encoded as base64 data URI string that you can then save locally or process further as needed.
- refresh(): Refreshes the current page. This is useful for reloading dynamic content or ensuring that your browser window is up-to-date with the latest version of a page.
- quit(): Closes all open browser windows and exits Selenium::Remote::Driver, freeing up any resources it was using.
And below is a sample code snippet:
my $driver = Selenium::Remote->new; # Create driver object
$driver->get(‘https://www.exampleknowledgebasearticleurl’); # Load webpage
my ($title) = $driver->find_elements(‘h1’, ‘css’); # Retrieve title element text with CSS selector h1
my ($content) = map { $_->get_text } @{$driver ->find_elements(‘.content p’)}; # Retrieve content element text with CSS selector .content p
Now that we have both the title and content stored as scalar variables, we can easily insert them into our database table.
Building Infrastructure To Support Web Scraping With Perl
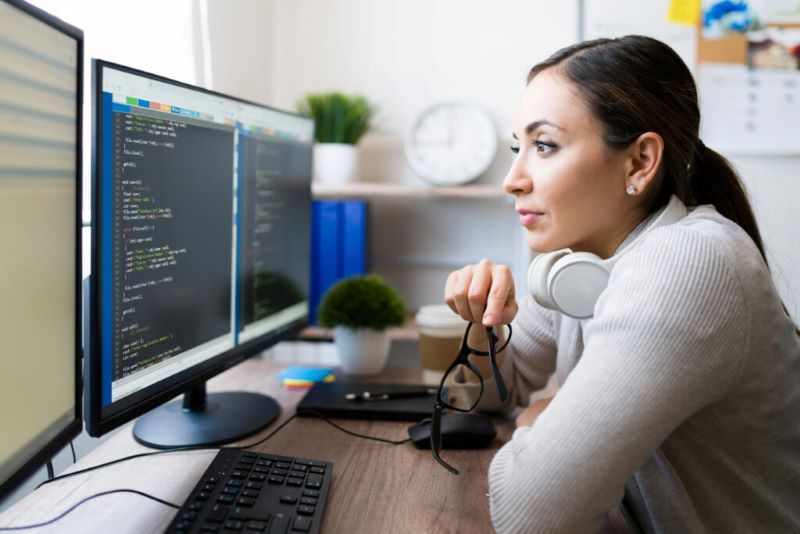
Regardless of your intentions — using Perl for web scraping emails from a list or prices from product pages — you will need the right infrastructure to scale your operations. This is especially true if you want your Perl web scraping encrypted or made more secure and reliable. The last important point in this Perl web scraping tutorial is finding the right proxies.
Proxies act as intermediaries between the user and the website, masking your IP address and allowing you to access blocked or restricted content. With proxies, you can quickly make multiple requests from different IP addresses with less chance of getting blocked by anti-scraping measures. This makes it easier for Perl scripts to scrape data efficiently from large amounts of websites quickly and reliably.
But you’re going to need the right provider with the right services. You’ll need reliable proxy servers to make sure your project runs smoothly, especially if you’re aiming for scalability. Rayobyte offers a range of proxies and an advanced Scraping Robot that can simplify the automation process. Check out our various proxy solutions now!
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.