What Is Parsing? (A Complete Guide)
If you’ve been working with web scrapers, chances are you’ve encountered the term parsing. Parsing is an important component not just of web scrapers but of data reading applications as a whole.
But have you ever wondered, “what is parsing?” and how is it used in data applications?
This guide will explore data parsing in more detail:
- What is parsing?
- parsing databases
- parsing use cases
So stick with us to learn what parsing is and how parsers parse data!
What Is Parsing?
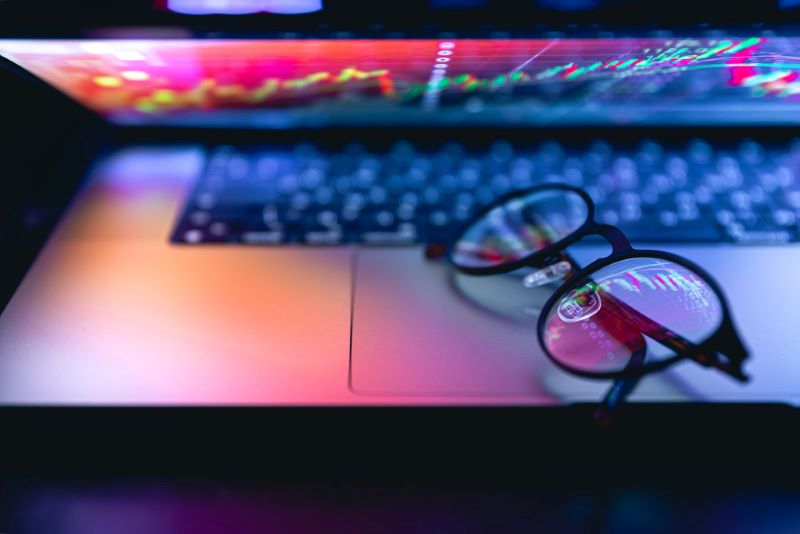
Simply put, parsing is the process of transforming data from one format to another, making it a more readable format.
Parsing is also known as syntax analysis, as it involves analyzing a list of symbols with some rules. Parsing can occur with linguistics, natural language processing (NLP), or computer science.
For our purposes, we’ll be dealing with parsing, which is a computer science application.
What Languages Can Parsing Methods Be Used With?
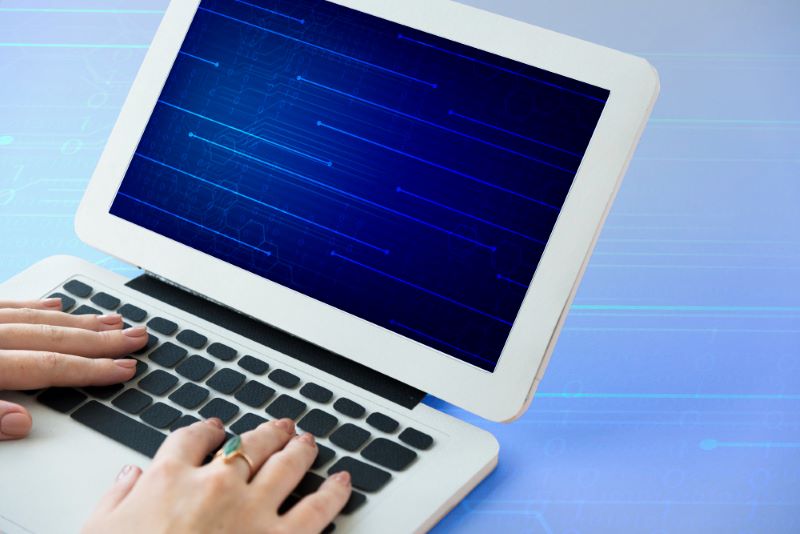
Nearly all programming languages across the board support parsing in one form or another. Below, we list some popular programming languages for parsing and text parsing. We’ve also included some prominent features of these programming languages to help you better decide what option to go with.
Perl
The OG parsing programming language, Perl, has many features that make it an ideal programming language for parsing. In addition, the Perl syntax is well-suited for writing regular expressions and has become one of the standard syntaxes for this task.
The Perl syntax is so well-liked by programmers for parsing that they wrote the Perl Compatible Regular Expressions (PCRE) library in the C programming language. This library provides the implementation of a regular expression engine in other programming languages.
Key features
- High-level
- General-purpose
- Interpreted
- Dynamic
- RegEX-friendly syntax
JavaScript
As one of the core technologies of the modern World Wide Web, JavaScript comes pre-packaged with all modern web browsers. When scraping dynamic web pages, JavaScript can prove to be an indispensable tool that can help parse dynamic data.
Furthermore, the language has a high degree of interoperability with other programming languages. As such, you can use features such as the PCRE library in JavaScript for smoother regEX handling.
Key features
- High-level
- Multi-paradigm
- Just-in-time compilation
- Dynamic
- Highly interoperable with other programming languages
C#
A popular language for web scraping, C# is another high-level programming language that lends itself well to parsing. The .NET framework provides library support for various functions, such as regEX handling and data structures.
What’s more, since you build most C# web applications in Visual Studio, it’s fairly intuitive to make a data parser using the Visual Studio GUI and form functionality. C# syntax is quite similar to Java’s, so if you have experience in the latter language, you’ll have a much easier time coding parsers in C#.
Key features
- High-level
- Multi-paradigm
- Both interpreted and compiled
- Statically-typed
- .NET framework support
Python
Python is currently one of the most popular programming languages in the world. This popularity is partly due to easy-to-read syntax that’s much more intuitive than languages like C#.
Furthermore, Python also has massive open-source library support for writing parsers. These libraries include pyPEG, PLY, and ANTLR, just to name a few. Even if you don’t wish to write your own parser in Python, you can use other languages such as Extensible Markup Language (XML) to easily parse web documents in Python.
Key features
- High-level
- Line-by-line code execution
- Both interpreted and compiled
- Dynamically typed
- Extensive open-source code libraries
HTML
Finally, although it might not strictly be a “programming language” per se, we have HyperText Markup Language (HTML). HTML is a markup language that is the Web Hypertext Application Technology Working Group’s (WHATWG) standard language for writing web pages.
If you’re intimidated by most programming languages like C#, the good news is that HTML syntax is pretty easy to follow. As long as you’re familiar with HTML tags and elements, you’ll be able to follow most of what’s going on. HTML also has a built-in parsing module for parsing web documents, which we’ll talk about next.
Key features
- Markup language
- Uses tags and elements
- WHATWG Standard for the web (HTML 5.0)
- Can easily handle web documents
- Built-in HTML parser
Types of Parsers
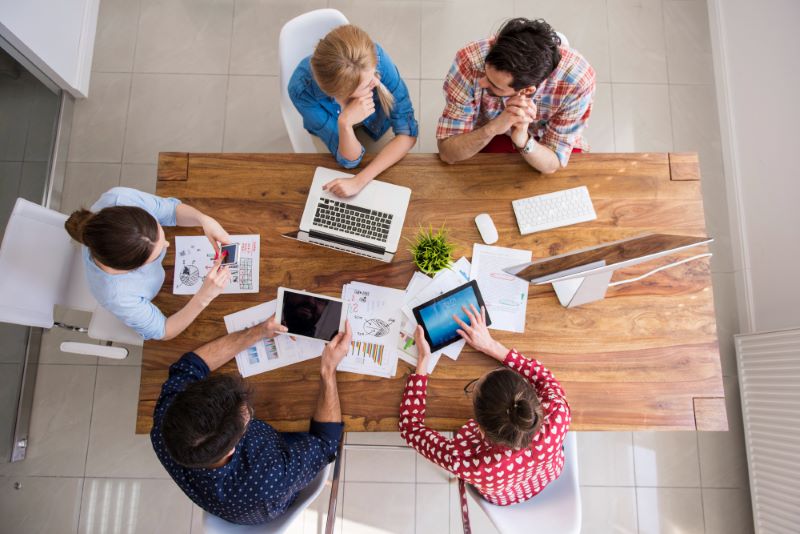
When it comes to parsers, there are no one-size-fits-all solutions. Instead, several web parsers exist, each based on different operating principles and optimized for specific applications.
Let’s have a closer look at some typical data parsers in use these days.
DOM parser
The Document Object Model (DOM) parser is one of the most widely used parsers today. This parser uses an object-based parsing approach. This approach means that the DOM Parser first loads the entire XML file into memory, converts the file into objects based on XML nodes, and only starts parsing after this process is complete.
This type of parser is only useful when you absolutely must validate the entire XML document before parsing it. Otherwise, the parser will parse the whole document from the start ’til the ending node. There is no way to parse just a few selected XML nodes.
DOM parsers are not recommended for very large XML files as the file can become difficult to validate.
SAX parser
The Simple API for XML (SAX) parser is an alternative to the DOM Parser. SAX parsers adopt an event-based approach rather than relying on an object-based approach. They also do not create parse trees, unlike DOM parsers.
Much like DOM parsers, SAX parsers also parse the entire document from start to finish. In other words, it’s not possible to parse just particular nodes with SAX parsers. Since SAX parsers only parse in forward-only mode, we recommend using them when you have consistent access to the XML document you wish to parse.
Data is also available in these parsers as soon as they see it, making them ideal for XML data that arrives over a stream.
XPath parser
XPath is a querying language that queries over XML nodes. It is the officially recommended XML querying language by the World Wide Web Consortium (W3C). As such, XPath parsers primarily use XPath queries to query over the XML document and its nodes. These queries can include node traversal, update, insert, and delete operations.
The main advantage of using an XPath parser is that you can access individual XML nodes. Unlike DOM or SAX parsers, XPath parsers do not parse the entire document from the beginning node to the end. Additionally, you can use the XPath structure definitions and path expressions to query over elements, attributes, text, and even comments.
Push parser
The push parser is a type of parser which uses XML data pushes. The push parser uses XML data that it pushes to the client. This type of parser is also an event-based parser. As the parser parses documents, it generates synchronous events. These events are then processed by the parser using callback handling.
One thing to note about push parsers is that the parser will send the data regardless of whether the client is ready to use it or not. That’s because the parser controls the application thread; the client can only handle the events, not generate them.
Callback functions like startDocument() and endDocument() are beyond a programmer’s control here. Push parsing libraries are often trickier to work with and are more extensive.
Pull parser
The last significant type of parser is the pull parser. In this type of parser, the client “pulls” the data when it explicitly needs it. The client also controls the entire application thread, unlike the push parser, where the parser handles the application thread. The client pulls the data by calling specific methods on the XML parsing library in use.
There are several advantages of using a pull parser over a push parser. A pull parser can read more than one document at a time with just a single thread. Additionally, pull parsing libraries are much more compact and more straightforward to use than push libraries.
Finally, some pull parsers can filter XML documents effectively, remove all unnecessary elements, and view non-XML data as an XML document.
How Do Data Parsers Work?
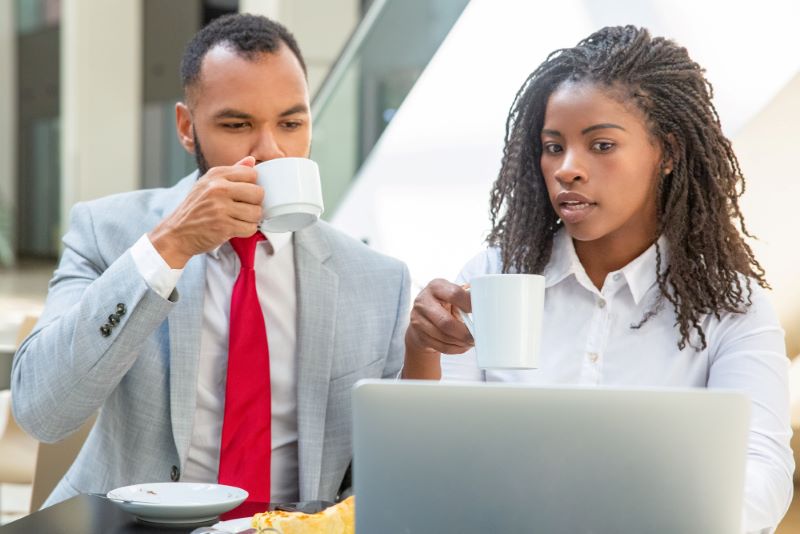
Now that you’re familiar with some different types of parsers, it’s time to get into the nuts and bolts of how data parsers work. We’ve divided this section into two parts: one for HTML parsers and another for XML parsers.
How HTML document parsers work
HTML has been around since the early days of the web. HTML is based on Standard Generalized Markup Language (SGML), which is based on the commands typesetters used in the 1960s to format documents. These days, HTML5 is the standard HTML version in use, which includes functionality for parsing documents.
The goal of any HTML document parser is to generate a DOM tree. The DOM represents data objects in a web document and represents the document as a tree of nodes and objects. The DOM is also a programming interface and makes it simpler for programming languages to interact with web page elements and objects.
There are several layers to an HTML document parser. Let’s briefly go over each layer to see what it does in the overall parser:
Network layer
At the very top of the HTML parser, we have the network layer. This is basically the network the data is transmitted over. In our case, the network is the internet, from where we will download the web page to parse.
Byte stream decoder layer
The next layer is the byte stream decoder. The data we receive from the network is in bytes, which are groups of binary digits (ones and zeros). The byte stream decoder helps convert the data block transmitted over the network from a byte array to strings. The exact conversion depends on the specified encoding format of the web document.
Input stream processor layer
Now, we reach the input stream processing layer. The input stream is an abstract class of the byte stream involved in the file reading. It is also known as the superclass of all I/O classes.
In our case, the type of file we want to read is a web document so that the input stream processor will work with this file type. The input to this layer is often directly from the network byte stream, but sometimes it can take input from the user agent using scripts. In that case, the script input comes through API methods such as the document.write() method.
Tokenizer
Next up, we have the tokenizer layers. Tokenization, or lexical analysis, takes input strings and splits them up into smaller units. These smaller units can be individual words, terms, or elements. The smaller units are called tokens.
The tokenizer splits up the string from the byte stream decoder into smaller tokens. In doing so, the tokenizer tries to follow rules that make the tokens easy to work with, such as removing whitespace, stemming, and lemmatization. The parser later processes these tokens and uses them to construct the DOM tree.
Tree construction layer
This layer is where the magic happens; the parser constructs a DOM tree from the tokens. Both the tokenizer and tree construction layer only have a single set of states. However, the tree construction layer is reentrant; the tokenizer can resume while the tree construction layer handles one token. This means that the previous layers keep feeding tokens to the tree construction layer, even while processing the first token.
Script execution layer
The script execution layer is not below the tree construction layer but operates at the same level. This layer also works parallel with the other layers and can sometimes feed back into the input stream processing layer. As the name suggests, the script execution layer also executes any scripts in the parser.
To see an example of the tree construction layer and the script execution layer in action, consider the following HTML block:
…
<script>
document.write(‘<p>’);
</script>
…
The <p> tag is a start tag in the code above that triggers the tree in the code above. The script then executes and the document.write() method runs. When the compiler reaches the </script> tag, it treats it as an end tag token and ends the script. The parser specifically has what is known as a script nesting level and a parser pause flag for handling such cases, which are initially set to zero and false, respectively.
DOM layer
The final piece of the puzzle is the DOM layer. Once the network sends the byte stream, which is processed as a string, tokenized, and turned into a tree, the final stage is the DOM. The DOM starts from the first node of the document and ends at the last node. Once the last node is appended to the DOM, the parser has finished parsing the HTML document.
Note that this process provides a very simple view of things; in practice, the HTML parser will most likely encounter some errors at some stage. These errors are often syntax errors that programmers can fix by correcting the syntax. An example is an end-tag-with-attributes error, which occurs if the parser encounters an end tag with attributes.
How XML document parsers work
Understanding how XML document parsers work is another key component of understanding parsing. However, before we jump straight into how XML document parsers work, it helps to know a thing or two about XML first.
Like HTML, XML is another markup language. The difference is that HTML displays data and describes a webpage’s structure, whereas XML merely stores, modifies, and transfers data. XML is also a standard language standardized by W3C. Programmers can also use XML to define other programming or computer languages (hence the name “extensible.”) In contrast, HTML is predefined with its list of rules and implications. Both XML and HTML are widely used for web development.
XML document parsers are pretty similar to HTML parsers in how they operate. The goal here is also to make a parse tree with XML nodes and structures. The only difference is that the XML parser follows XML rules for mapping byte arrays into strings or characters that ultimately combine to form a document object. In other words, it transforms the XML document into readable code that a program can easily work with.
Some commonly used XML parsers include the following:
- Saxon
- Java built-in parser
- Microsoft Core XML Services (MSXML)
- System.Xml.XmlDocument (.NET library)
HTML parser vs. XML parser
XML parsing is still different from HTML parsing despite a similar overall structure with more or less the same layers.
The major difference is that XML parsing rules are much stricter than HTML parsing rules. For example, XML requires every markup element to be well-formed. By well-formed, we mean that every element must have both a start and end tag or have an empty element tag in case it’s empty. In addition, boolean variables in XML markup must also have explicitly defined attribute values, which must be quoted. The XML parser must follow these rules strictly; even a minor violation may lead to a runtime or compilation error.
By contrast, HTML has few strict rules in place for parsing. For example, many HTML elements can have their end tag omitted, yet an HTML parser can still parse them. Syntax rules are also more relaxed in HTML parsers; quotes around attribute values in HTML parsers are optional but mandatory in XML parsing. XML parsing also supports element interfaces and custom elements, which operate on XML data structures.
Other than these rules, the process of XML parsing is quite similar to HTML parsing. For example, the document object still has to be populated with DOM nodes representing the tree structure of the parser input.
Since both are similar in operation, but HTML parsers have less rigid rules, we find HTML parsers to be a superior choice for generalized parsers. However, most HTML parsing libraries also include support for XML parsing by default, so you’ll be able to work with both depending on the parsing application.
The Best HTML Parsing Libraries
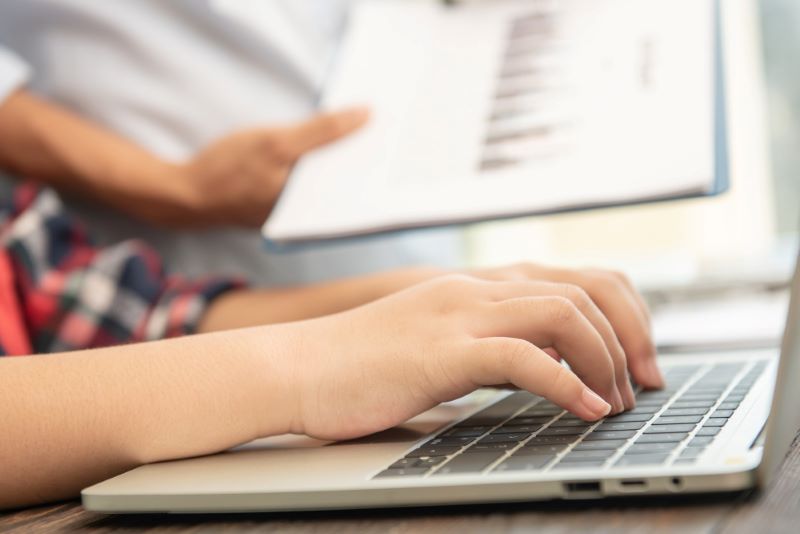
By now, you’re probably convinced that HTML is an ideal choice for parsing web documents. The good news is, most programming languages come built-in with HTML parsing libraries. These libraries help automate the parsing process via API calls according to their built-in functionality. To better understand what parsing is, it’s essential to familiarize yourself with some of these libraries.
Here are a few popular HTML parsing libraries that you can use in your web scraper today:
BeautifulSoup
Language: Python
First on our list is BeautifulSoup, a Python library for HTML and XML data manipulation. The library provides a Rest API, which provides the parsing functionality for navigating through, modifying, or searching the parse trees. This functionality can ultimately end up saving hours of work for the programmer. So whether you’re scraping data from HTML or XML, Beautiful Soup lets you easily parse through tags, attributes, or special strings with a single API.
HtmlAgilityPack
Language: C#
If you’re looking for a reliable web scraping and parsing library in C#, you can’t go wrong with HtmlAgilityPack (HAP). HAP is an HTML parsing library written in C# that can help read, write, or access the DOM. HAP supports XPATH, a query language for selecting nodes in XML, and XSLT, a language for transforming XML documents. If you’re looking to build a C# web scraper, HtmlAgilityPack will provide you with all the functionality for making one straight out of the box.
Cheerio
Language: JavaScript
When it comes to JavaScript, Cheerio is one of the best libraries available for parsing. The library provides a smooth API that can easily parse markup and generate a data structure from the parsed data. Cheerio is based on a subset of core jQuery. Unlike with typical jQuery, Cheerio is free of DOM inconsistencies. Instead, it works with a simpler, more consistent DOM model that is easy to parse and manipulate. As a result, the library can parse any HTML or XML document.
JSoup
Language: Java
There’s no need for Java users to feel left out; there are library options for parsing in Java, such as JSoup. The JSoup library is optimized to work with HTML5, using HTML5 DOM manipulation methods with CSS selectors. This optimization results in an intuitive API that is exceptionally well-suited for modern HTML web pages according to the WHATWG HTML5 specification. JSoup is suitable for both web scraping and parsing from files, data strings, or even URLs. The library also offers additional security features, such as using a safelist to verify user-submitted content. This feature is helpful to prevent Cross-Site Scripting (XSS) cyberattacks.
Nokogiri
Language: Ruby
Finally, we have Nokogiri, an HTML web scraping and parsing library for Ruby. Unlike some other APIs, the Nokogiri API is intuitive to work with and easy to understand. For this reason, it can make parsing in Ruby, which is usually a difficult task, much more manageable. The API also treats all web documents as untrusted unless specified otherwise, adding an extra layer of default security. Nokogiri also supports multiple parser types, such as the DOM parser, the SAX parser, and the push parser.
Parsing: Use Cases
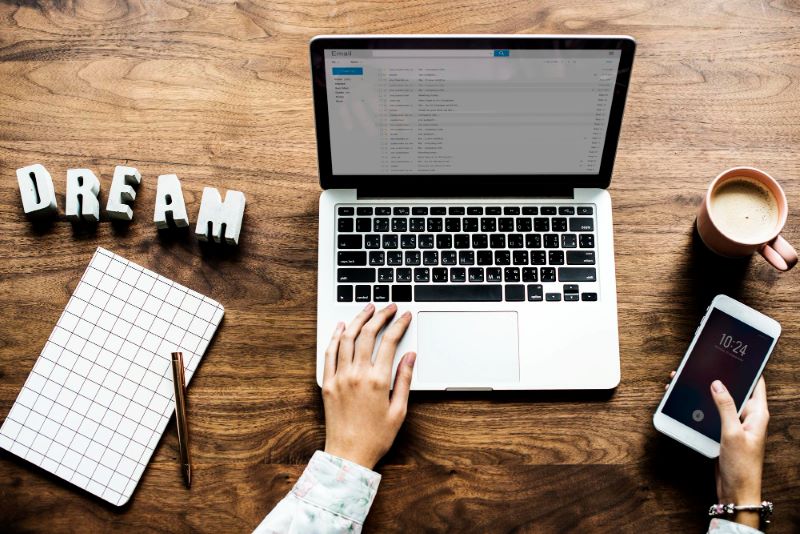
There are several uses for parsers in computer science and software. As long as an application requires converting data from one format to another, chances are you’ll need a parser to deal with it.
Here are some of the common parsing use cases:
Compiler construction
Parsers are used for compiler construction and are an essential part of the process. A compiler is a computer program that translates code written from one programming language into another. Most programming languages have a built-in compiler, which translates the programming language into a lower language code, such as assembly language, that the computer can read.
SQL queries
Structured Query Language (SQL) queries are another critical use case of compilers. Whether you run queries in MySQL, SQL Lite, or Oracle, the result is the same: The SQL engine sends any query you write to a parser, translating it into another machine-friendly language. Although SQL queries may seem intuitive for humans, they are illegible to machines without parsing. Parsing is also required for parsing databases using queries; any query you issue using SQL will parse the database until it finds the appropriate entry. Exceptions exist when using indexing to improve queries.
Web scraping
parsing is a core part of web scraping, without which the entire process would be voided. Often, parsing happens after the scraper scrapes data from a target web page and downloads it. The parser takes the raw data, cleans it up for smoother operation, and stores it in a more readable format, such as a .csv file (comma-separated value list). parsing in web scraping may involve manipulating HTML strings, tables, or other elements until they display only the relevant information.
Data structures
Data structures are one of the most important constructs in data science. A data structure is a format that helps with organizing, managing, and storing data so that computers can access and modify it efficiently. In addition, data parsers can help build some data structures. Examples of such data structures include parse trees, abstract syntactic trees, or other data structures that have some form of hierarchy.
Regular expressions (RegEX)
Regular expressions are character sequences with well-defined search patterns. Often, programmers deploy string-searching algorithms over regular expressions to search for patterns. The programming language Raku, formerly known as Perl 6, includes dedicated support for parsing expression grammars. Additionally, Raku allows building different types of parsers, such as recursive descent parsers.
Natural language processing (NLP)
Parsing has been a part of NLP since the early days of computer science. Natural language data, such as human language, can be difficult to parse without performing some operations over the text first. The computer scientist must first define the grammar for the text, ideally context-free grammar. Then, they must decide what kind of parsing technique to use. Semantic parsers, for instance, can convert strings of texts into representations of what they mean.
How Parsing Helps Your Business Goals
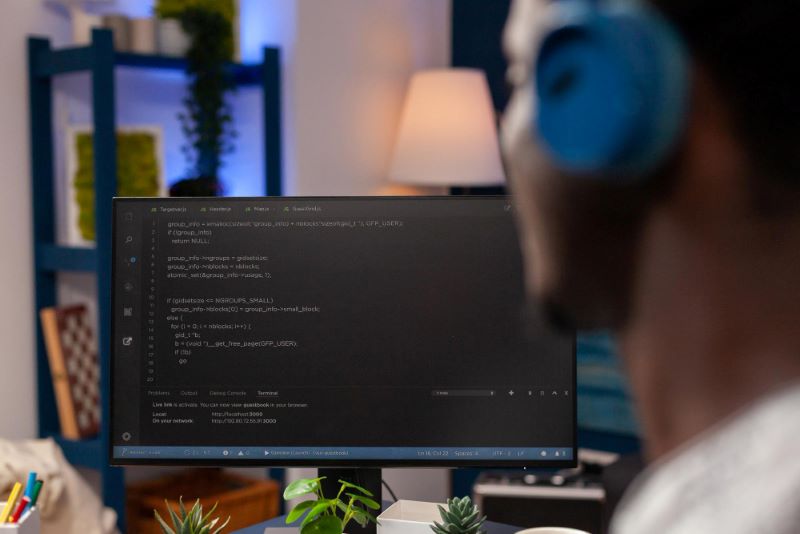
So now, you know what parsing is, parsing databases using queries, the different types of parsers, and which programming languages are the best for writing your parser. However, all this knowledge begs the question: how exactly does parsing help my business and its goals at the end of the day?
The answer is that there are many ways parsing can help both small businesses and corporations alike. In other words, parsing isn’t restricted to theoretical computer science.
Here’s how parsing can help your business meet its goals:
Saves time and money
Before the days of parsing, working with data used to be a slow and painstaking process. After all, who would want to read through document after document, carefully looking for data to extract manually? However, with the right parsing software, data operations can run exponentially faster.
Although there is a cost upfront of buying a parser or building your own, it more than pays off in the long run. This is especially true in the data age, where people generate more and more data every single day. Ultimately, small business owners can better utilize their resources in more productive channels by investing in a data parser.
Improves data interfacing
If there’s a challenge when working with data, other than dealing with sheer volume, it’s handling the data effectively. That’s why effective user interfaces for handling, manipulating, and working with data are paramount to any business’s success. Unfortunately, companies can sometimes struggle when they have massive amounts of data but no proper interface for handling it.
A data parser can help with the interface, as it makes data more accessible and easily searchable. Parsing data in a DOM tree is much simpler than manually scanning entire documents for search phrases. parsing tools also make data files much easier to work with and visualize. The result is that using a data parser can make data much more straightforward to read and manipulate for business professionals, often requiring just a few clicks of a mouse to navigate.
Adapts data
The data age hasn’t just revolutionized the sheer volume of data in production but also the formats of said data. Sometimes, businesses can use data and data-handling tools that are severely outdated.
Fortunately, a data parser can help change the data format to one much easier to work with. Even if the data is old, the right parser can make it a breeze to navigate through. In addition, since data parsers can store data in multiple formats, there is no shortage of ways to make the data more adaptable and accessible in a modern format. You can store your parsed data in a spreadsheet, for example, and share it with your co-workers and employees. A data parser helps you optimize new data streams and modernize your old data with otherwise redundant storage formats.
Should I Code My Own Parser?
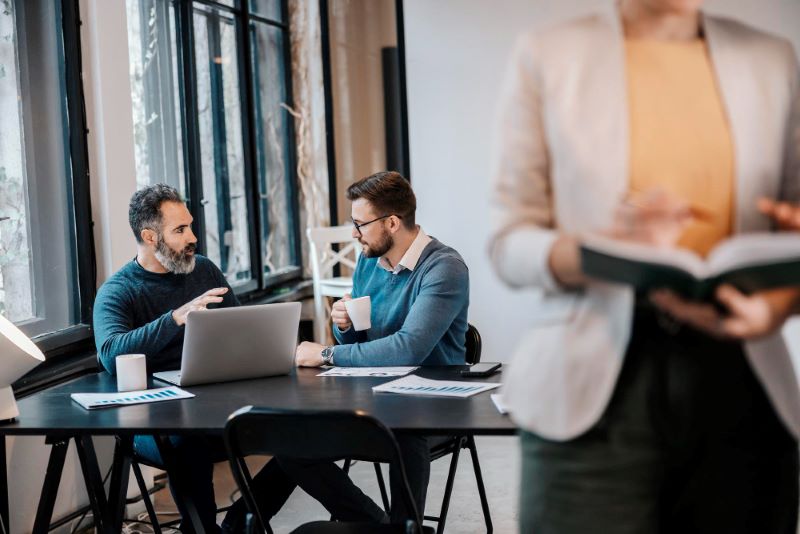
If you’re still with us so far, you’re probably convinced that it’s a good idea to have a data parser for your business. However, when it comes to acquiring a data parser, there are two routes you can take: build your own parser from scratch or buy a ready-made parsing solution. So which one should you choose?
Here are some pros and cons of coding your own parser vs. outsourcing one.
Coding your own parser
Pros:
- Greater autonomy over your program; you can code the parser in any programming language of your choice and optimize it the way you see fit.
- It is usually cheaper to build your own data parser from scratch, especially if you have a dedicated team of software developers.
- Coding your own parser gives you granular control over the parsing. This granularity means that if you wish to target specific tags or content keywords, you can specify them.
- You can update and maintain your parser as you see fit.
Cons:
- A dedicated in-house software development team is required to build your own parser.
- You may need to build, buy, and later maintain a separate server to host your parser. The server also needs to be fast to parse the data quickly.
- Web pages are rarely fixed as their HTML is constantly changing. By choosing to build your own parser, you’ll have to code separate logic to handle such cases.
Buying a pre-built data parser
Pros:
- No extra cost of hiring a dedicated development team.
- Server building and maintenance are taken care of automatically.
- Several people have already used pre-built parsers, so they’re often free of common parsing bugs and errors.
- Tech support and maintenance are much easier when you outsource your parser, as the supplier often provides dedicated tech support.
Cons:
- Slightly more expensive; higher initial costs.
- Little control over the parser and its source code.
So even though coding your parser gives you a greater degree of control and autonomy, outsourcing your data parser has fewer overall cons, even with the higher initial cost.
What Data Parser Should I Buy?
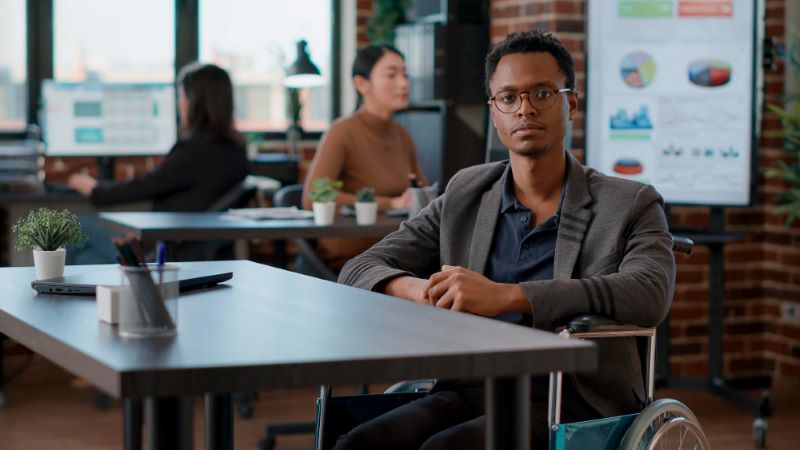
If you’re looking for a pre-built data parser to purchase, look no further than Rayobyte’s Web Scraping API.
Rayobyte’s Web Scraping API is a state-of-the-art scraping bot that comes pre-packaged with all the tools you need for successful web scraping, including parsing. No matter what type of web data you’re parsing, Rayobyte’s Web Scraping API can help you prevent the common pitfalls and errors associated with parsing — additionally, Rayobyte’s Web Scraping API outputs structured JSON data from any website’s parsed metadata, which is simple to work with. New modules are constantly added to Rayobyte’s Web Scraping API for increased functionality.
But what about IP bans and blocklists? Try Rayobyte rotating residential proxies. With Rayobyte Rotating Residential IPs, honeypots, CAPTCHA, and similar web scraping pitfalls will be a thing of the past. Rayobyte Rotating Residential IPs periodically swap your IP address from a list of residential IP addresses. Rather than having to switch your IP addresses occasionally to prevent bans manually, you can rest easy knowing Rayobyte rotating IPs will handle everything for you.
Are you looking for a data center IP instead? Rayobyte datacenter IPs provide you with over 300,000 IP addresses in over 29 different countries worldwide. These include the USA, UK, Canada, France, Germany, and even South Korea, to name a few. What’s more, Rayobyte datacenter IPs can handle a staggering 25 petabytes of data every month while giving users over nine autonomous number systems (ASNs) to choose from. This feature maximizes redundancy; even if a web admin bans an entire ASN of proxies, you’ll still have eight other ASNs to select from with Rayobyte datacenter IPs.
If ISP Proxies are more your type, Rayobyte also offers the best ISP Proxies in the market. Rayobyte is the number one ranked US-based proxy provider, giving users unmatched control and customization over their residential proxies. Additionally, Rayobyte ISP Proxies provides users with over 1 GBPS of speed and offers users over three real IP ASNs. The best part? Rayobyte ISP Proxies are 100% ethically sourced. So not only do you get residential-level authority and ban prevention, but you can also rest easy knowing your ISP proxy is completely ethical!
Final Thoughts
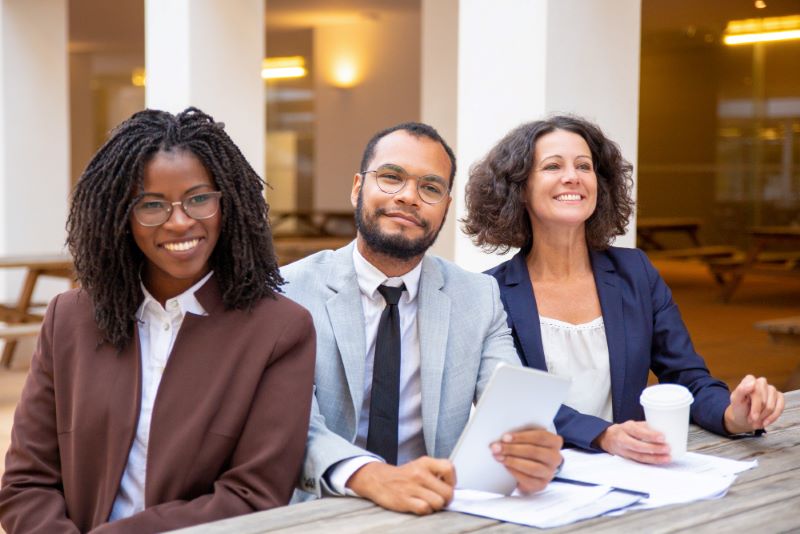
parsing is an integral part of the web that can also help businesses with their data processing needs. To learn more about parsing, it also helps to understand how it works, the different types of parsers, and which programming language to choose for your parser.
Although you can code your own parser, the process can be time-consuming and difficult without the proper expertise. So why not try outsourcing your parser with a well-known application, such as Rayobyte’s Web Scraping API?
Get Rayobyte’s Web Scraping API with other Rayobyte solutions today to take your parsing game to the next level. We guarantee you’ll be on your way to a superior parsing experience.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.