What is Parsing in Python and What are Parsing Errors?
Table of Contents
As an increasingly popular way to extract data from websites, web scraping is used by businesses, researchers, and developers to quickly gain insights or automate mundane tasks. With web scraping and parsing, you can easily collect information such as product prices, customer reviews, and competitor intelligence. The programming language Python offers powerful tools for both web scraping and parsing, making it a go-to language for many in this field.
In this guide, we’ll go briefly through what is web scraping in Python, what is parsing in Python, what is parse error to start with, and how to deal with it. Generally, when you encounter parsing errors when web scraping with Python, first you need to try and identify the source of the error. The most common sources of parsing errors in Python when web scraping are as follows:
Once you have identified where the issue is stemming from, it’s important that you fix it accordingly by taking steps such as updating modules/dependencies and changing the syntax in your code — whatever is the best course of action depending on the issue. This guide will explore what is parsing in Python, parsing error definitions, the most common errors you’ll encounter, and some steps to remediate those issues.
Python scraping 101
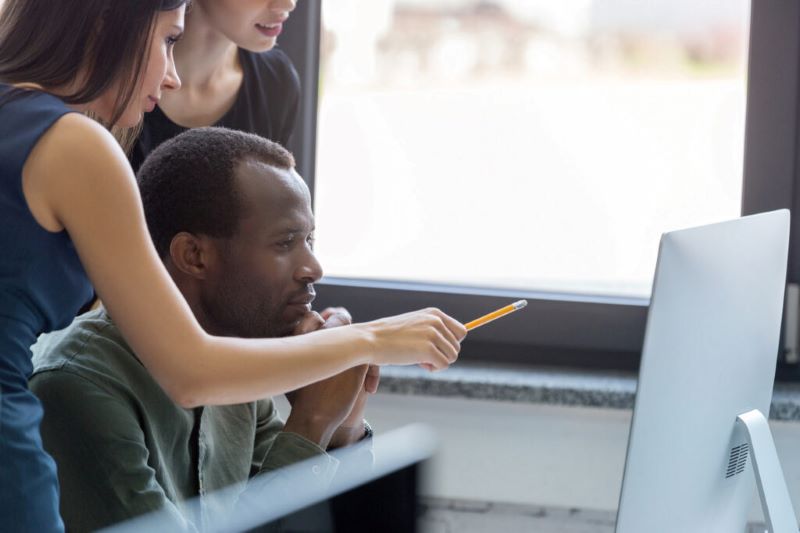
Before we delve into parsing error definitions, we need to gain a decent grasp of contextual information. First off: Python for web scraping.
Python is an incredibly powerful tool for web scraping. With Python, you can write a script to quickly and easily scrape website content such as HTML files, text documents, images, videos, and more. Once the data has been extracted from the website, it can then be used for further analysis or manipulation in other programming languages or applications. Python’s vast library of modules makes web scraping extremely versatile.
For a more in-depth guide on Python web scraping, read our guide.
Before any extracted information can be used, however, it needs to be parsed. This is where a Python file parser or something similar comes in handy. A Python file parser is a program that can read and process data from text files. It’s often used in web scraping, where it helps to extract specific pieces of information from web pages.
What is parsing in Python?
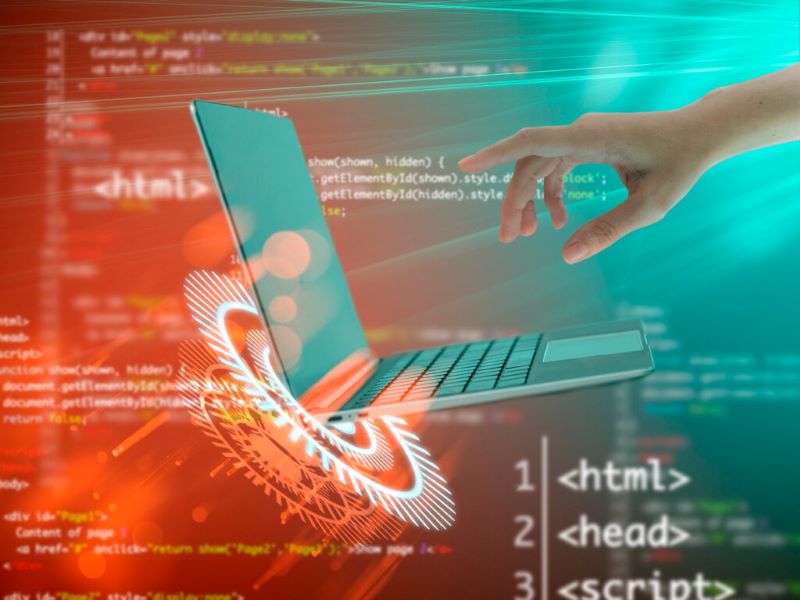
Parsing is the process of analyzing a text and breaking it into smaller chunks to gain a better understanding.
Python has several libraries and modules that make web scraping and parsing easy: BeautifulSoup, Scrapy, Requests, and lxml. Each library has its own advantages. For example, BeautifulSoup is great for working with HTML documents while Requests lets you easily access HTTP requests. With any of these tools, you can quickly parse through websites or other sources of unstructured data — like PDFs or spreadsheets.
Now, what does parse error mean?
When you get a parse error, it means that some sort of error relating to Python parsing has been encountered in the code. This usually occurs when the structure of the code does not meet Python’s expectations, such as improper indentation or incorrect data types being used. When this happens, it can cause your program to crash or produce unexpected results. So, what is a parse error? It’s a message telling you to troubleshoot your Python web scraping program, usually with phrases or code errors to identify the specific issue.
Now that we’ve covered what is parsing in Python and also parsing error definitions for our use, it’s time to familiarize yourself with the most common ones you’ll encounter.
The most common parsing errors in Python web scraping
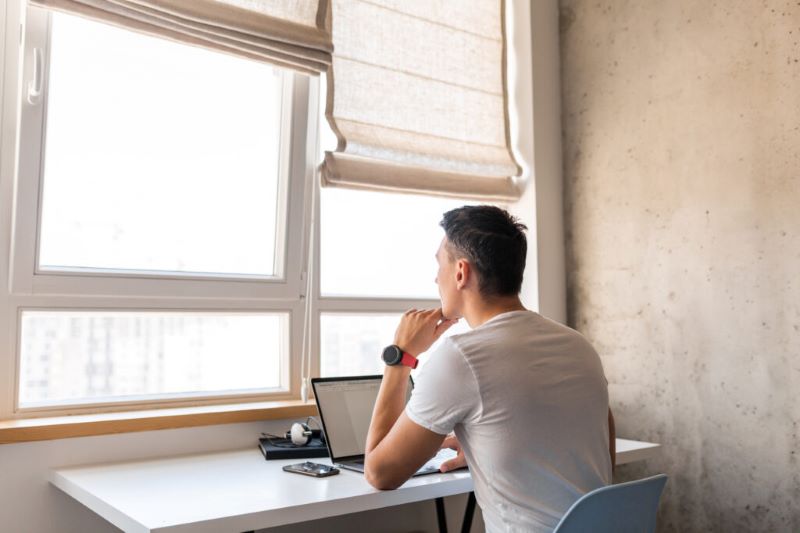
When you get a parse error, Python usually gives you useful hints. Below are the most common parsing errors you will encounter when web scraping with Python, what you’ll see when they come up, and the usual ways to remedy them.
Incorrect data structure or formatting
Data structure and formatting are important aspects of web scraping as they determine how the data is stored and organized. If the wrong data structure or format is used, parsing errors may occur during web scraping, which can lead to unexpected results when attempting a scrape.
If this is the case, you may encounter something like an “IndexError,” indicating that your code was trying to access a certain element but it did not exist in the given list or dictionary due to incorrect indexing. Moreover, you may see an error stating that certain elements could not be found due to invalid formatting being used for searching them. There are also straightforward but persistent issues such as unexpected EOR. What is unexpected EOF while parsing? This error message occurs when Python encounters unexpected end-of-file (EOF) characters. This can happen if the code contains a missing or incomplete statement, or if it has mismatched parentheses, brackets, and quotes. Additionally, if the website was recently updated and your scraping code hasn’t been updated accordingly, you may see an error stating that certain elements could not be found.
The best way to address this is by closely investigating your code and looking for any possible causes for errors. Additionally, examine the source code of the website you’re scraping to ensure that elements are structured in a consistent manner. Make sure there are no missing end tags or mismatched parentheses, brackets, and quotes. Update your scraping code to match if the website has recently been updated so that it reflects any changes made on their end.
Missing modules or dependencies
Modules or dependencies in Python are various packages and libraries that your code needs in order to run correctly. Examples of these include Requests, BeautifulSoup4, lxml, Pandas, and many more. These are necessary for web scraping as they provide the tools needed to interact with websites and extract data from them. If any of these modules or dependencies are missing, you may encounter an “ImportError,” indicating that the module could not be imported. Additionally, you may see an error stating that certain packages are missing from your system environment.
The best way to address this is by ensuring all necessary packages and modules are installed on your system before running any scraping code. You should also check for updates of existing packages and install them if needed to prevent any compatibility issues with newer versions of CPython.
Syntax issues in your code
Syntax issues in the way you code in Python can also lead to parsing errors when attempting a scrape. If the code contains incorrect or out-of-date syntax or if there are mismatched parentheses, brackets, and quotes in the code, you may encounter various errors. One example is “IndentationError,” indicating that there is a problem with the indentation of your code. Further, you may see an error stating that certain elements could not be found due to unexpected characters or keywords being used. You may also encounter an unexpected parser state, which generally means there is a syntax or structural issue in your code.
The best way to address this is by closely examining your scraping code for any potential causes for errors and ensuring all syntax is up-to-date and correct according to the version of Python being used. Additionally, check if any new keywords have been introduced since last updating your scraping script. So, they can be incorporated into it.
Timeouts
Timeouts due to high website traffic, slow connection speed, or other network-related issues can lead to parsing errors when attempting a web scrape. Timeouts refer to the amount of time that a connection between two systems is allowed to remain idle before it is terminated. If the server takes too long to respond or if the connection is interrupted for any reason, you may encounter various errors. One example is “ConnectionError,” indicating that there was an issue establishing a connection with the server. You may see an error stating that certain elements could not be found due to timeouts and/or slow response times from websites.
The best way to address this is by ensuring your internet connection has sufficient bandwidth and speed before running any scraping code so as not to overload it with requests, which could cause timeouts on certain websites. Additionally, using caching techniques such as request pools can help reduce the latency of requests by reusing existing connections.
HTML tags that don’t match the expected output format
HTML tags are the building blocks of a website and they define how content is displayed. When web scraping, if any HTML tags don’t match the expected output format or elements aren’t found on the page, you may encounter errors such as “NoSuchElementException,” indicating that an element could not be located by your code. Additionally, you may see an error stating that certain elements were not found due to unexpected characters or keywords being used.
The best way to address this is by closely examining all HTML tags in your scraping code for any potential causes for errors and ensuring all syntax is up-to-date and correct according to the version of Python being used. You need to check if any new keywords or HTML tags have been introduced since last updating your scraping script and then incorporate them into updated versions.
Inadequate memory allocation for running scripts/storing data
Adequate memory allocation for running scripts or storing data is essential to ensure that web scraping processes can be completed successfully. Without enough RAM available, the script may not be able to execute properly and errors will occur. For example, if there is insufficient space in your system’s memory when attempting a scrape, you may encounter an “Out of Memory Error,” indicating that more resources need to be allocated before proceeding. Additionally, if the script is too large and requires more RAM than what is available on your machine, you may see an error stating that certain elements could not be found due to inadequate resources being allocated for running it.
You can fix this issue by ensuring that sufficient resources are allocated before starting any scraping process. This includes increasing the amount of RAM available on your machine as well as reducing the size of any scripts used. So, they do not require excessive amounts of memory.
Incorrect use of RegEx
Regular expressions are powerful tools used to search and manipulate strings. They can be used in web scraping to find specific patterns of text on a page and extract the desired data. However, if regex is not written correctly or if it does not match the format of the data you are attempting to scrape, errors may occur during parsing. For example, you may encounter an “Invalid Syntax Error,” indicating that your regex was incorrect or did not match what was expected from it. Additionally, you may see an error stating that certain elements could not be found due to invalid syntax being used for searching them.
This sort of problem is very similar to incorrect data structures or formatting or code syntax issues, except instead of the problem being in your Python code, it’ll be in your use of regex.
Fix this issue by ensuring that all regular expressions are properly written before running any scraping code and testing them against sample datasets first. So, they would work as intended when extracting real-world data.
Best practices for dealing with parsing errors in Python
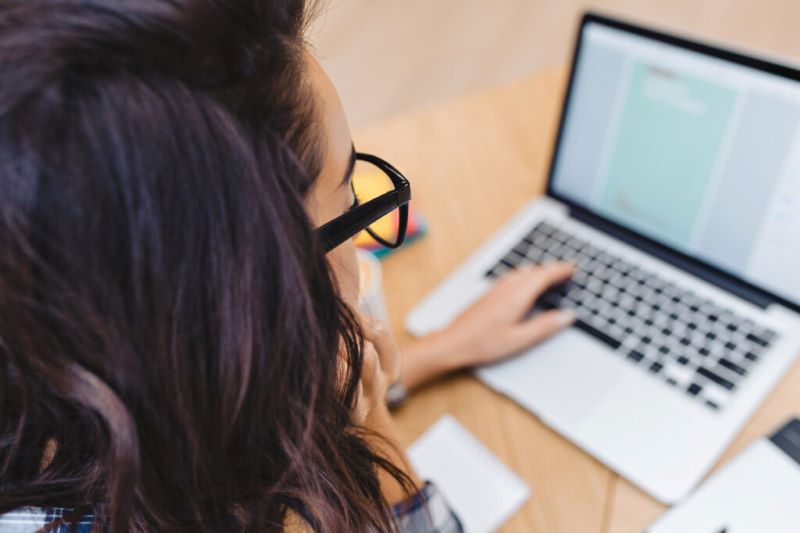
Given the most common issues encountered as outlined above, there are some handy best practices for preparation and troubleshooting you can adopt to improve your web scraping efforts:
- Ensure all necessary packages and modules are installed on your system before running any scraping code (e.g., Python, BeautifulSoup, Selenium).
- Check for updates of existing packages and install them if needed in order to prevent compatibility issues with newer versions of Python.
- Examine the source code of the website you’re scraping to ensure that elements are structured in a consistent manner. Update your scraping code accordingly if changes have been made at their end since the last scrape was conducted or attempted.
- Closely examine your scraping code for any potential causes for errors including syntax issues, incorrect data structures or formatting, missing end tags or mismatched parentheses, brackets, or quotes, among other small but encompassing syntax requirements.
- Test regular expressions against sample datasets first before running them against real-world data sources. This will help identify incorrect syntax or other problems early on, which can save time later down the line.
- Make sure there is sufficient RAM available when attempting a scrape. Increase resources allocated as needed and reduce the size of scripts used accordingly. So, they don’t require excessive amounts of memory allocation.
- Monitor network-related factors, such as connection speed and website traffic levels, that could cause timeouts during web scraping.
- If possible, set up alerts whenever websites being scraped undergo major changes. So, scrapers can be updated appropriately without having to manually check at regular intervals.
Additionally, sometimes proxy servers can cause errors in the process of extracting data. In order to prevent these issues, it’s important to use a reliable anonymizing service that masks your IP address. So, you don’t run into any problems when parsing with Python. This is in addition, of course, to the above.
Using proper web scraping infrastructure to further minimize parsing errors

So you’re now well acquainted with what is parsing in Python, what parsing errors are, and which are the most common ones. One last important factor to take into account is digital infrastructure.
The infrastructure you use to support your web scraping efforts via Python or any other approach can make or break your operation. Reliable proxy servers and features like proxy rotation and rate limiting can help to address some of the issues related to web scraping in Python.
Scrapers need to be careful when dealing with websites that are protective of their data or use aggressive defense mechanisms. These sites usually want to avoid server overloads and malicious attacks. To guarantee successful results, use proxy rotation, which means switching between different IP addresses to stay undetected and get past access restrictions.
Proxy rotation can be improved by rate limiting. This involves delaying requests so that the server does not receive too many at once, making it think they are from humans rather than bots. By slowing down access to data, you can avoid detection and make sure your scraping activities don’t put an excessive strain on the server. Proper proxy use combined with measures such as rate limiting help websites to stay unaware of any automated activity taking place.
This profiling based on data access behavior is called fingerprinting. It can be used by websites to identify if someone has tried accessing information from multiple IP addresses in a short amount of time. When fingerprinting, an “identity profile” is created depending on various factors like browser type and version, language settings, OS details, and plug-ins installed in their browsers. This profile allows sites to guess whether requests are coming from a real person or not.
Technology such as fingerprinting can classify website scrapers as non-malicious if they adhere to certain standards. For instance, a web browser that is widely used for legitimate purposes like Chrome or Edge should be used instead of something like Tor Browser. Additionally, the user’s language preferences ought to correlate with the location of the site being scraped. All these values may be adjusted in web scraping by modifying request headers and other settings. So, ensure you pay due attention to those as well as the other considerations mentioned above to avoid parsing errors.
Parse error Python cheat sheet
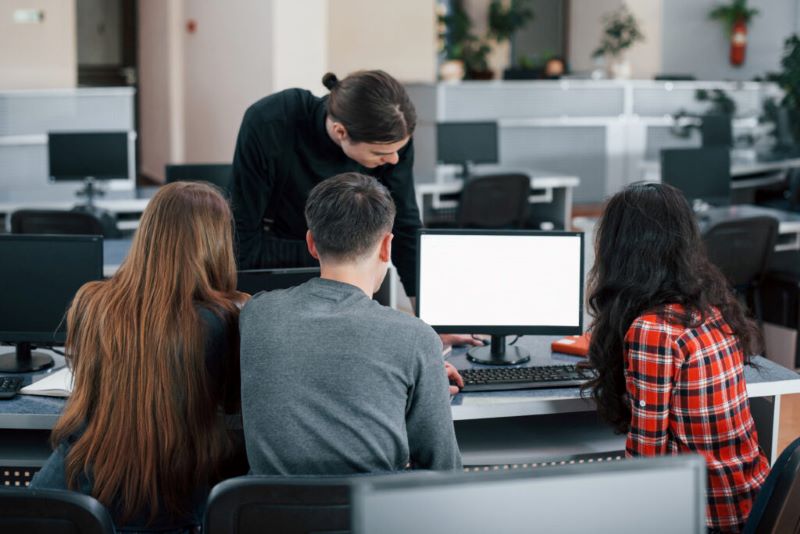
Here’s a cheat sheet containing critical information you can refer to when you encounter parsing errors:
Symptom(s) | Error | To-Do (Solution) |
Index Error, unexpected EOF, elements not found | Incorrect data structure or formatting | Closely investigate code and look for any possible causes for errors. Examine the source code of the website you’re scraping to ensure that elements are structured in a consistent manner. Update your scraping code if needed. |
ImportError, packages missing from the system environment | Missing modules or dependencies | Ensure all necessary packages and modules are installed on your system before running any scraping code. Check for updates of existing packages and install them if needed. |
IndentationError, elements not found (due to unexpected characters or keywords), unexpected parser states | Syntax issues in your code | Closely examine scraping code for any potential causes for errors. Ensure all syntax is up-to-date and correct according to the version of Python being used. Check if any new keywords have been introduced since last updating your scraping script. So, they can be incorporated into it. |
ConnectionError, slow response times from websites | Timeouts | Ensure your internet connection has sufficient bandwidth and speed before running any scraping code. Use caching techniques such as request pools to reduce the latency of requests by reusing existing connections. |
NoSuchElementException, elements not found (due to unexpected characters or keywords) | HTML tags that don’t match the expected output format | Closely examine all HTML tags in your scraping code for any potential causes for errors. Ensure all syntax is up-to-date and correct according to the version of Python being used. Check if any new keywords or HTML tags have been introduced since last updating your scraping script and then incorporate them into updated versions. |
Out of Memory Error, elements not found (due to inadequate resources being allocated for running it) | Inadequate memory allocation | Ensure that sufficient resources are allocated before starting any scraping process. This includes increasing the amount of RAM available on your machine as well as reducing the size of any scripts used so they do not require excessive amounts of memory. |
Invalid Syntax Error, elements not found (due to invalid syntax being used for searching them) | Incorrect use of RegEx | Ensure that all regular expressions are properly written before running any scraping code and test them against sample datasets first. So, they work as intended when extracting real-world data. |
Ethical proxy use
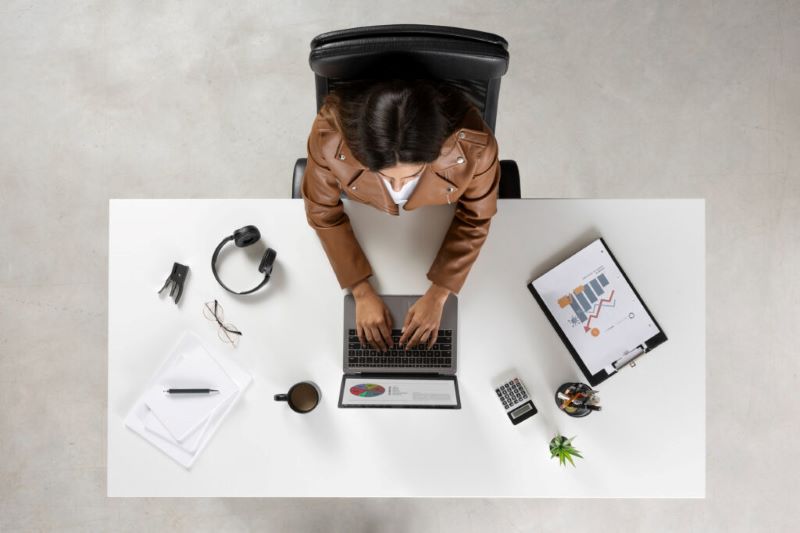
This guide delved into what is parsing in Python and common parsing errors and best practices to fix them. The above cheat sheet is also provided to make the guide more readily accessible and efficient for use.
Remember that properly configuring your web scraping approach is essential when you want to get hold of valuable data from multiple sources. It’s key to determine a method and configure its settings correctly to suit your needs and make sure you use reliable infrastructure (like proxy servers) to support it.
Rayobyte is the go-to proxy provider for web scraping. We offer residential proxies, ISP (Internet Service Provider) proxies, and data center proxies to fit your needs — all with a highly professional and ethical team.
Residential proxies are ideal if you need to scrape the web since we only source top-notch IP addresses provided by ISPs with minimal downtime expected. Data center proxies can help increase speed when retrieving data from the internet. However, using them could reduce unique non-residential IP addresses used in your project. An ISP proxy strikes a balance between both worlds: fast speeds through data centers plus trustworthiness associated with an internet service provider.
If you want to maximize your web scraping and parsing with Python, Rayobyte has the perfect solution. Our proxies are a great way to bypass anti-scraping measures, while our Scraping Robot tool helps automate parts of the process. See how we can help you get started today!
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.
Sign Up for our Mailing List
To get exclusive deals and more information about proxies.
Start a risk-free, money-back guarantee trial today and see the Rayobyte
difference for yourself!