The Ultimate Guide To Getting Started Web Scraping With Java
While data may have been overlooked in the past, today, it forms the foundation of every decision an organization makes, regardless of its industry and size.
Data collection through web scraping has become increasingly common in the last decade because it offers a fast, inexpensive, and flexible way to extract data from the internet.
Web scraping is an efficient way to gather large amounts of information that would otherwise be impossible or very time-consuming to get, either by hand or using traditional crawling techniques like the ones search engines use.
While there are many other reasons for using web scrapers, such as saving money, one major benefit is speed.
This guide will particularly focus on web scraping with Java and how it can help extract data from websites that you can later save in a CSV format for further analysis.
What Is Web Scraping?
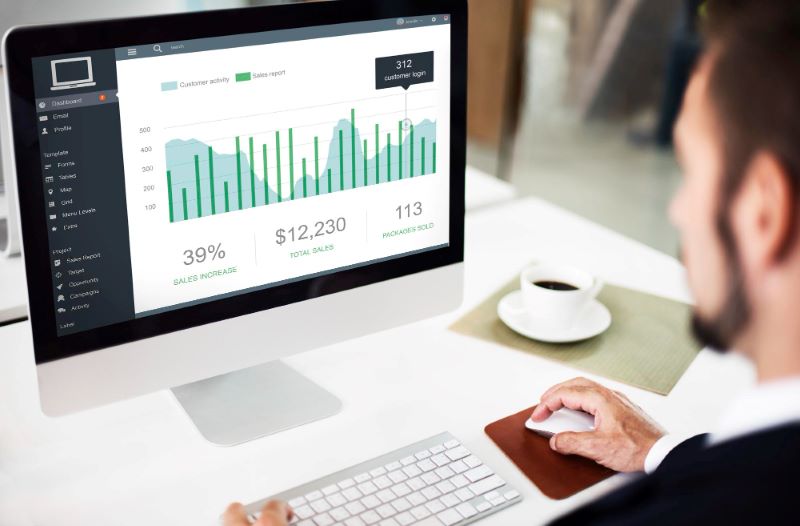
Web scraping refers to the process of automatically extracting data from websites. It’s a technique used to extract large amounts of data for analysis, including data that is not easily available or accessible.
The act of web scraping generally involves three main steps:
- Proxy and Web Parsing: First, the scraper needs to proxy all the requests made to the target website so it can hide the user’s IP address. This is important because webmasters can easily block requests from IP addresses they do not recognize. In addition, the scraper also has to parse (or break down) the HTML code of the target website into its constituent parts so it can be easily accessed and extracted.
- Data Extraction: Once the HTML code has been parsed, the scraper can then extract any data it wants from the target website. This could include text, images, or entire tables of data.
- Export: The final step is to export the data that has been extracted from the target website into a format that can be easily read and analyzed, such as a CSV file.
Importance of web scraping
Web scraping is not merely a hyped practice or a buzzword. It actually has many benefits for organizations, such as lead generation and price intelligence.
- Lead Generation: Many companies use web scraping to gather data on potential leads. This could include contact information, job titles, or other relevant data.
- Price Intelligence: Web scraping can also be used to monitor the prices of goods and services online. This is important for businesses that want to stay competitive and ensure they’re getting the best deals.
- Other Benefits: There are many other benefits of web scraping, such as market research and competitor analysis.
Why Use Java Web Scraping?
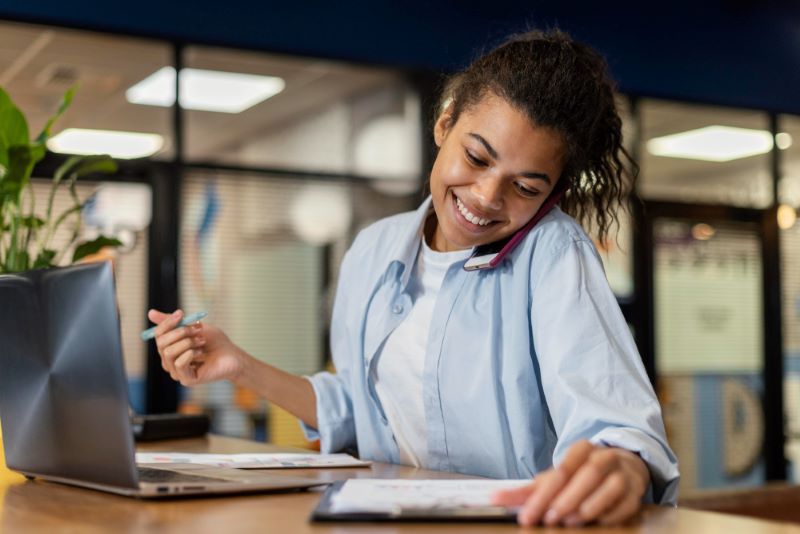
Java is a popular programming language that is used for all sorts of applications, including web scraping. While there are many languages that can be used for web scraping, Java has several advantages that make it a good choice for this task.
First, Java is a versatile language that can be used on both Windows and Apple platforms. Java is also an open-source language, which means that there are many libraries and tools available for free. This is important because it can save time and money when building a web scraper.
Second, Java has a large user base. This means that there is a large community of developers who can help with troubleshooting or give you advice on how to best do a specific task. There are hundreds of Java User Group discussions and resources available for Java developers.
Finally, Java is a powerful language that offers many features that make it ideal for web scraping. These include the ability to create custom classes and objects, as well as the ability to access and extract data from websites.
Java web scraping frameworks
When web scraping with Java, you can use two libraries, namely JSoup and HtmlUnit. Although both of these frameworks work well, HtmlUnit is often the recommended choice because it emulates a browser’s key aspects, such as getting a page’s specific elements and clicking them.
Web scraping in java with JSoup
JSoup is a Java library designed specifically for working with real-world HTML. It can parse and extract data from websites and save it into a structured Java object that can then be further analyzed in your Java program.
HtmlUnit
HtmlUnit is an open-source web scraping framework that uses the latest versions of the browser engine (typically either Chrome or Firefox) to run each website.
Using this approach, there is no need to install or configure specific drivers, because Firefox and Chrome install these automatically. The good thing about this framework is that you can turn off CSS and JavaScript with only one line.
In turn, this is helpful when web scraping with Java, since you don’t need CSS and JavaScript for the process.
Terms to Know
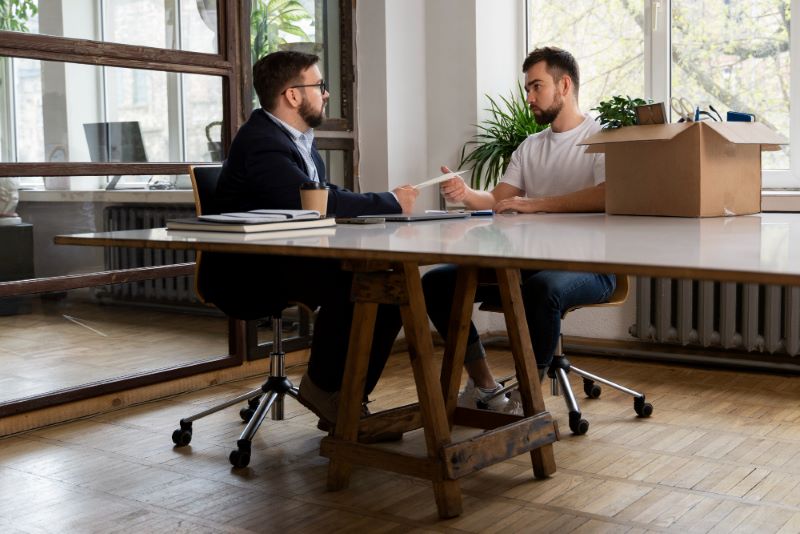
Before we give you an introduction to web scraping with Java, there are a few important terms you need to be familiar with.
Parsing
Parsing refers to the process of taking a string and creating a structure out of it. For example, parsing the following sentence would result in an abstract syntax tree such as this:
{Sentence: “Before we give you an introduction to web scraping with Java, there are a few important terms you need to be familiar with.”;NP:[;
https://app.compose.ly/editor/projects/21240/guidelinesD: “Before”
PP:[;D: “we”]
NP:[;PREP: “to”,
V:”give”,
NP:[;N:’you’],
NP:[;D:”an”,O:’introduction’,
PP:[;P:’to’,
NP:[; “web scraping with Java, there are a few important terms you need to be familiar with.”]
]);}
In computer science, parsing is the process of analyzing a string of symbols to determine its structure. The parser tries to find the syntactic units in the text and build a data structure out of them.
In the Java web scraping tutorial we discuss below, you will parse Java HTML code before it can be further processed. JSoup can parse data from websites and save it as a structured Java object.
HTTP request
An HTTP request is a request sent by a web browser to a web server to retrieve some data.
To scrape a website, you need to send an HTTP request to that website and extract the data you need from its response. You can use a library such as JSoup to extract the text and raw links from the HTML code returned by an HTTP request.
CSV
CSV is a file format that is used to store and share tabular data (numbers and text). You can save a parsed HTML object as one of these CSV files and open it up in Excel, for example.
Introduction to Your Java Web Scraping Tutorial
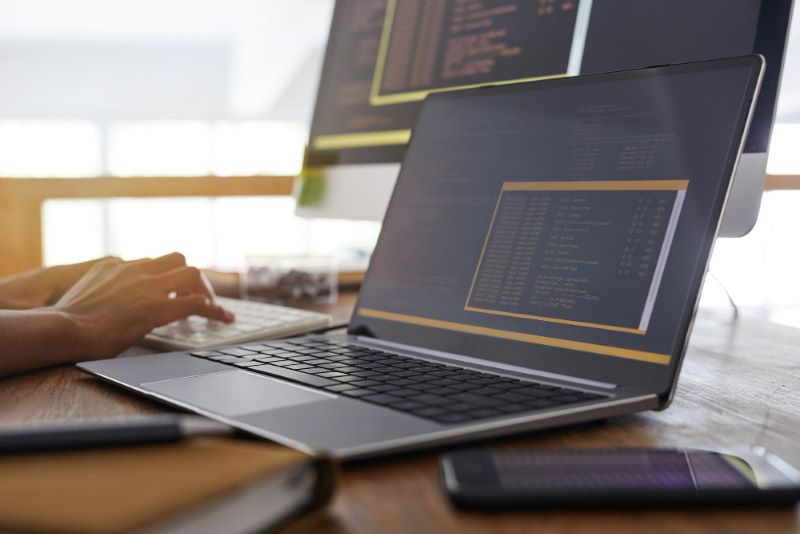
Now that you know the basics of web scraping with Java, let’s take a closer look at Java web scraping and how to build a Java website scraper.
Step 1: get the prerequisites
Before you can start web scraping with Java proxies, you need to ensure you have the prerequisites. These include:
- Java 8: It is important to have a version of Java 8 installed, since this is the only version that currently supports HtmlUnit. In addition, you can use any other IDE such as Eclipse or IntelliJ IDEA.
- HtmlUnit: This is a Java library that allows you to run web pages in your application, just like real browsers do. It provides functions for both rendering and extracting content from HTML documents, which means it is very helpful when scraping websites with Java.
- Gradle: It is a build system that helps you automate the process of compiling, testing, and packaging your Java application.
- Java IDE: You also need a Java IDE for web scraping in Java with JSoup Java. In this guide, we’re using IntelliJ IDEA. It integrates with Gradle quite easily.
After you’ve installed Java, verify if you’ve followed the official guides right. Run the following commands in a terminal:
> Java -version
> Gradle -v
These commands will show you which version of Gradle and Java is installed on your system. If you don’t see any errors at this step, you’re good to go.
Before you start writing code, you need to create a project. Here’s a detailed guide on using Gradle and IntelliJ in case you get confused at any point during the process.
Start by creating a project. Let IDE finish its first build, since you’ll be working with an automatically generated file tree. After the build is finished, open the “build.gradle” file and type the following in the block titles “dependencies.”
implementation(‘net.sourceforge.htmlunit:htmlunit:2.51.0’)
Doing so will lead to the installation of HtmlUnit in the project. To remove the ”Not found” warnings, click the ”Reload” button in the Gradle toolbox.
Step 2: inspect the page to be scraped
Now, go to the page you want to scrape. Right-click anywhere on the web page and click on the “Inspect element.” This will open the developer console. You can find the HTML of the website here.
Step 3: send an HTTP request
Send an HTTP request through HtmlUnit. This will get the HTML on your system. To do this, you need to go to IDE and type the following code to use HtmlUnit:
import com.gargoylesoftware.htmlunit.*;
import com.gargoylesoftware.htmlunit.html.*;
import Java.io.IOException;
import Java.util.List;
Then, you have to send an HTTP request to the website you want to scrape by initializing a WebClient. Once you’ve received a response, you need to close the connection. Otherwise, it will continue to run.
WebClient webClient = new WebClient(BrowserVersion.CHROME);
try {
HtmlPage page = webClient.getPage(“https:website.com/webpage you want to scrape/”);
webClient.getCurrentWindow().getJobManager().removeAllJobs();
webClient.close();
recipesFile.close();
} catch (IOException e) {
System.out.println(“An error occurred: ” + e);
Keep in mind that, when you do this, you’ll see many error messages in your console. Don’t panic when you see these messages, because most of them can be ignored. You can limit some of the useless errors you see by configuring your WebClient:
webClient.getOptions().setCssEnabled(false);
webClient.getOptions().setThrowExceptionOnFailingStatusCode(false);
webClient.getOptions().setThrowExceptionOnScriptError(false);
webClient.getOptions().setPrintContentOnFailingStatusCode(false);
Step 4: extract sections
By now, you should have the HTML document. But you need data that you can work with. The response you received in the previous step must be parsed into information that humans can read.
To start, extract the website’s title. Our project has a built-in method to do this. It’s called getTitleText:
String title = page.getTitleText();
System.out.println(“Page Title: ” + title);
Next, you can extract the website’s links. There are built-in methods for this, too, called getAnchors and getHrefAttribute.These methods will extract the <a> tags from the HTML response.
List<HtmlAnchor> links = page.getAnchors();
for (HtmlAnchor link : links) {
String href = link.getHrefAttribute();
System.out.println(“Link: ” + href);
}
As evident, HtmlUnit has many built-in methods that you can use to extract information of your choice from the documentation. You can then store the data too.
Store scraped data in GridDB
GridDB is a distributed, high performance NoSQL database that is based on a scalable and distributed multi-dimensional sorted map. After scraping data using Java, you can store it in GridDB.
To do this, you firstly need to create a container schema as a static class:
public static class Post{
@RowKey String post_title;
String when;
}
Then, you need to create a Properties instance through the GridDB installation particulars. It should have the name of the cluster you want to connect to, the user’s name who wants to connect, and the user’s password. This code demonstrates these things:
Properties props = new Properties();
props.setProperty(“notificationAddress”, “239.0.0.1”);
props.setProperty(“notificationPort”, “31999”);
props.setProperty(“clusterName”, “defaultCluster”);
props.setProperty(“user”, “admin”);
props.setProperty(“password”, “admin”);
GridStore store = GridStoreFactory.getInstance().getGridStore(props);
You need to get the container to run queries. You already created the ”Post” container earlier. To get it, type the following code:
Collection<String, Post> coll = store.putCollection(“col01”, Post.class);
Now, you’ve created a container instance with the name ”coll”. This is what you’ll now use to refer to the container. Next, you can create indexes for both columns of the container.
coll.createIndex(“post_title”);
coll.createIndex(“when”);
coll.setAutoCommit(false);
By typing this command, you’ve set the auto-commit to “false.” Thus, you’ll have to commit the changes manually. Now, you have to create an instance of the container “Post” and use it to insert data into the container.
Post post = new Post();
post.post_title = title.text();
post.when = time.text();
coll.put(post);
coll.commit();
Step 5: Export the Data
The final step of this web scraping tutorial is exporting the data to CSV. Doing so will make it easier for you to share this data with analysts in your organization. Type this command:
import Java.io.FileWriter;
Then, initialize the FileWriter. Suppose you’re scraping recipes from a website and want to name your CSV file accordingly.
FileWriter recipesFile = new FileWriter(“recipes.csv”, true);
recipesFile.write(“id,name,link\n”);
After doing this, you can specify that the first line of the file will be the head of the table.
recipesFile.write(i + “,” + recipeTitle + “,” + recipeLink + “\n”);
With that, you’re done writing the CSV file. You can now close it.
recipesFile.close();
Now, you can see your data in a format that you can easily process further and even open in Excel.
Should You Build a Web Scraper?
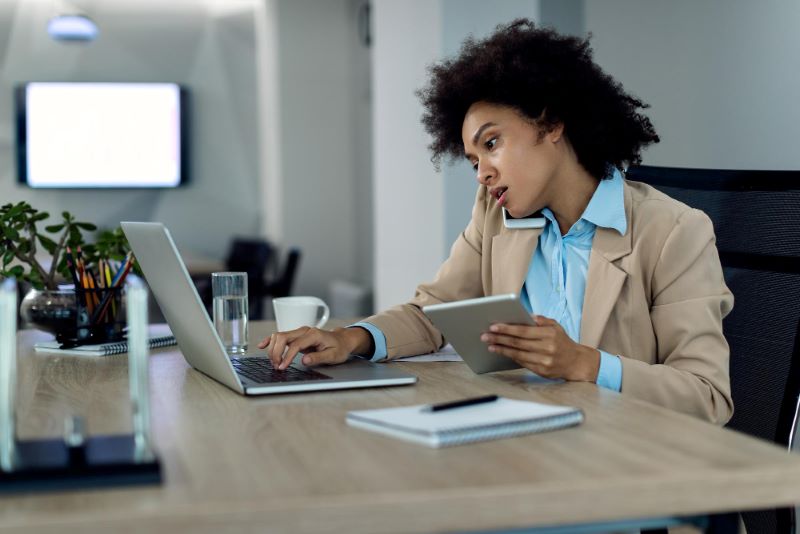
Enterprises that want to scrape the web have two options. They can either build their own web scraper or use a pre-built one.
You should choose to build your own web scraper if:
- You’re scraping a website that is not publicly available
- The website you’re scraping has authentication or session cookies that need to be handled
- You want more control over the data extraction process
- You want to scrape a website that is constantly changing
If you don’t fall into any of the categories above, you should consider using a pre-built web scraper. Pre-built web scrapers are easier to use and require less technical expertise. They also come with built-in features that allow you to extract data quickly and easily.
Rayobyte’s Web Scraping API is a web-scraping tool built for developers that you can easily plug into your API. While Rayobyte’s Web Scraping API takes care of all your scraping needs, you can focus on other aspects of your business. Rayobyte’s Web Scraping API provides tools that let you scrape websites into JSON and other formats quickly and easily.
When you’re web scraping using Java or any other language, you run into multiple issues, such as proxy rotation and browser scalability. But thanks to Rayobyte’s Web Scraping API’s robust functionality, it takes care of all these things and gets your desired information from target websites.
Moreover, Rayobyte’s Web Scraping API shows you beautiful graphs of the number of scrapes you’ve performed in the past week, month, or even day. In this way, you can keep a record of all your scrapes.
You also don’t have to worry about CAPTCHAs and bans, since Rayobyte’s Web Scraping API can tackle these problems easily.
Challenges in Web Scraping Using Java
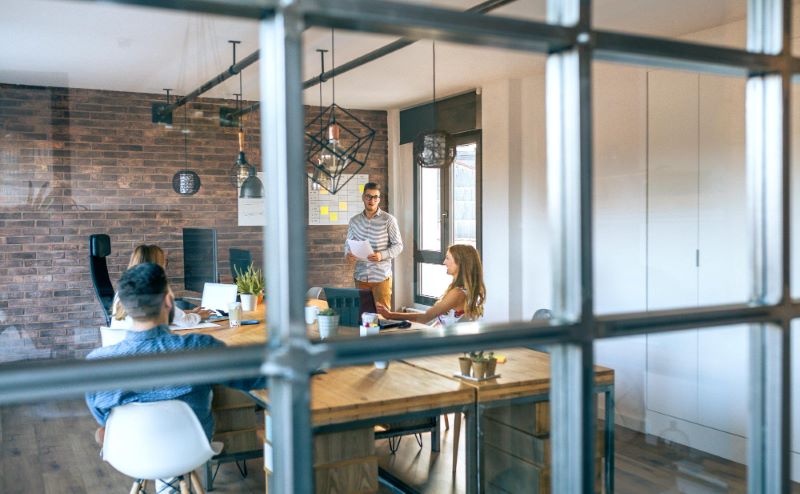
Although web scraping offers a host of benefits, it comes with a few challenges. These challenges can hinder you from scraping data from a website using a Java website scraper.
Bot access blocked
The first challenge you might face is accessing websites blocked by robots.txt. The robots.txt file is a set of instructions for web robots. Most webmasters use the file to guide the behavior of crawlers. This is what makes it a common challenge for many data scrapers.
To resolve this issue, you can either try to gain permission from website owners or simply ignore the file by overriding it.
Unstructured data
Another challenge that might arise during web scraping is related to unstructured data. Many websites do not follow a standard format when presenting their data, making it difficult for a web scraper to identify patterns and extract information.
To resolve this situation, you need to make sure you check out all variations of formats used on a website before extracting any information from it.
Server side request failure
There are times when your scraper will fail due to a Server Side Request Failure.
This is because some servers send an HTTP error even though the page exists. In short, the server can’t find anything. For example, if you request for https://rayobyte.com/games, you will get a 404 Not Found Error, as Rayobyte doesn’t have a “games” page.
CAPTCHA
CAPTCHA refers to those pesky little tests that websites use to figure out if you’re a human or not. They’re often used as a security measure to keep bots from scraping data from websites.
There is currently no known workaround for CAPTCHA, although some people have reported success by using virtual machines with different IP addresses.
Honeypot traps
A honeypot trap is a technique that websites use to detect and deter web-scraping activities. Websites use honeypot traps to lure bots into providing sensitive information, such as usernames and passwords.
One way to avoid falling victim to honeypot traps is by using proxies or changing the User-Agent string of your scraper.
Slow load speed
If a website gets too many requests, its load speed will get slower and slower. When a human is browsing the web, they can simply click the reload button to load the website. Web scrapers don’t have the ability to deal with such emergencies.
Login requirement
Some websites have login requirements to gain access to their data. This can be a challenge for web scrapers, as they need to find a way to log in to the website automatically.
IP blocking
IP blocking is a method that website administrators use to prevent access to their website by certain IP addresses. This can be a challenge for scrapers, as they rely on IP addresses to function.
To work around this issue, you can either use proxy servers or change your IP address regularly.
Although web scraping comes with its own set of challenges, it remains one of the most efficient ways to extract data from websites. By understanding and overcoming these challenges, you can make sure your data-scraping activities are successful.
What Are Proxy Servers?
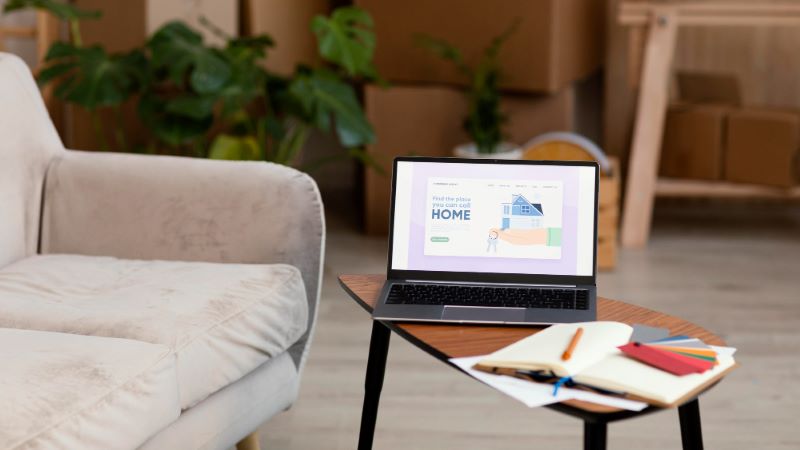
As mentioned earlier, you can avoid IP blocking by changing your IP address or using a proxy server. The former is tedious, since you’re sure to run out of IP addresses after a few scraping requests.
On the other hand, proxy servers can make web scraping using Java a breeze. A proxy server is a server that sits between your computer and the website you’re trying to scrape. When you make a request to a website, the proxy server will relay that request to the target website on your behalf.
This way, the target website only sees the proxy server’s IP address instead of your computer’s IP address. As such, it’s very difficult for websites to block requests from proxy servers.
There are many different types of proxy servers, but the most popular type is the HTTP proxy server. An HTTP proxy is a proxy server that relays requests for web pages (HTTP requests) from clients to servers and vice versa.
Most HTTP proxies are configured to allow clients to connect to any destination server. However, the HTTP proxy will only allow requests to certain destination servers if they are allowed by a configuration file.
These proxies may be residential (from home servers) or data centers (from data centers).
Using residential proxies for web scraping
Residential proxies refer to IP addresses that are assigned to home users. These proxies are often seen as more reliable than data center proxies because they are less likely to be blocked by websites.
Data center proxies, on the other hand, are IP addresses that are assigned to data centers. These proxies are often seen as less reliable because they are more likely to be blocked by websites.
However, you could use Rayobyte’s data center proxies if you want to scrape a large number of web pages. With 99% uptime and effective proxy rotation, these proxies can give you access to data from over 27 Countries.
But if you want ban protection, it’s best to use residential proxies. Due to their reliability, residential proxies are a better choice for web scraping than data center proxies.
You can find residential proxy providers online, and most of them offer a subscription-based pricing model. For example, you can buy a monthly subscription from a provider and get access to a set number of residential proxies.
Rayobyte’s residential proxies are the most efficient for web scraping, allowing you to get your desired information from websites without getting blocked. Rayobyte is undeniably the most reliable proxy seller, following ethical proxy management.
All residential partners of Rayobyte have full liberty to decide how their IP addresses are used. They can also opt out of the program whenever they want. Rayobyte residential proxies are offered with legal thoughtfulness in mind, ensuring efficient scraping for users.
Depending on your business needs, you can use residential proxies for different purposes.
Ad verification
Residential proxies play an important role in ad verification. They are used to verify the accuracy of ads by matching them against a user’s real-world location. This helps to ensure that ads are not being displayed in the wrong location or to the wrong audience.
Residential proxies are unique in that they are sourced from actual residential IP addresses. This makes them ideal for ad verification, as they provide a more accurate location match than other proxy types.
By using residential proxies for ad verification, you can be sure that your ads are being shown to the right people in the right places.
This can help you improve your campaign performance and reduce wasted advertising spending.
SEO monitoring
Residential proxies can also be used for SEO monitoring, since they’re not associated with any particular IP address. This means that you can use them to check the rankings of your website on different search engines without revealing your identity or location.
You can also use residential proxies to track how your competitors are ranking and the strategies they’re using to outperform you.
By checking how well your SEO practices are doing, you can improve your rankings and increase your website’s visibility on search engines.
SEO monitoring can also help you to see how well a specific page is performing, as certain pages might not be achieving the desired results even though they have been optimized for SEO.
In addition to optimizing web content, you can also use residential proxies to run pay-per-click campaigns through platforms like Google Adwords.
This will allow you to learn about the number of clicks that each ad is getting, which you can then use to figure out whether it should be part of the campaign.
Social media monitoring
Today, your target audience is present on a number of social media sites. When you have to reach out to different audiences and promote your business in different locations, you can use residential proxies.
They provide you with an accurate location match without the risk of being blocked by social media sites due to proxy detection.
Social media sites have different geo-restriction settings for each country or region that they cater to. When you’re targeting a specific audience in these regional locations, you can use residential proxies to access your target audience on social media platforms.
To get even better web scraping results, it’s best to rotate the proxies.
What Are Rotating Proxies?
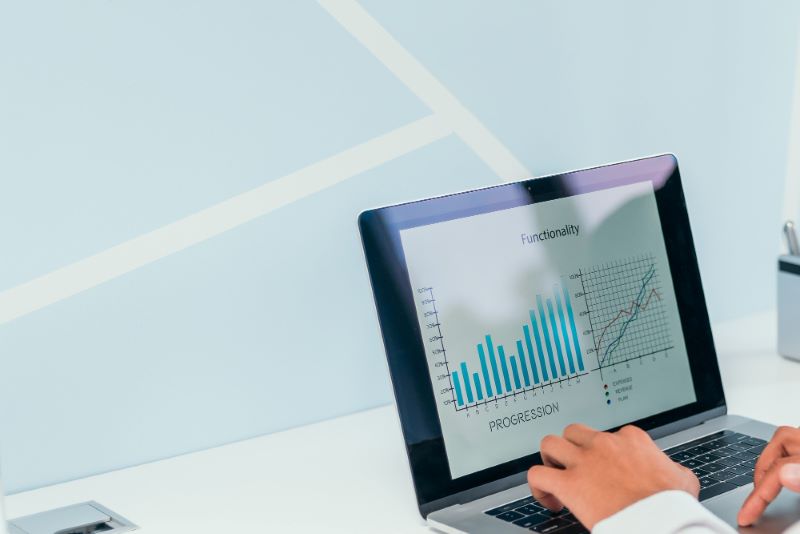
Rotating Proxies are a type of proxy service that provides you with a different proxy IP address each time you access the internet. This helps to keep your identity and online activities private by obscuring your real IP address.
How do rotating proxies work?
When you connect to the internet through a rotating proxy service, you are given a new proxy IP address each time.
This prevents websites and other online services from tracking your movements and activities. It also helps to keep your identity hidden from prying eyes.
Benefits of a rotating proxy
There are many reasons why you might want to use a rotating proxy service. Some of the most common reasons include:
Availability of IP address pool
When you’re scraping multiple websites, you need to have a number of IP addresses to send a multitude of requests without getting blocked. With a rotating proxy, you get a new IP address for each request, so you can keep scraping without getting blocked.
You can also create a proxy pool to ensure there’s no downtime even if one IP address gets blocked.
A proxy pool is a group of proxies that can be used by your web scraping program. A proxy server is identified with an IP address and port number. Proxies in the same proxy pool will have different IP addresses, but all share the same port.
Anonymity and privacy
If you’re concerned about your privacy and want to keep your identity hidden online, a rotating proxy is the best way to go.
By hiding your real IP address, you can surf the internet anonymously and keep your activities private.
Ease of use
Rotating proxies are extremely easy to use. Simply connect to the proxy server and start surfing the internet. You don’t have to worry about changing your IP address or configuring anything — the proxy service takes care of everything for you.
Rayobyte offers rotating residential proxies, saving you the hassle of manually managing proxy rotation. The proxies are rotated through a pool of thousands of residential IP addresses to ensure you always have a fresh proxy.
Web Scraping with Java Proxies: Use Cases
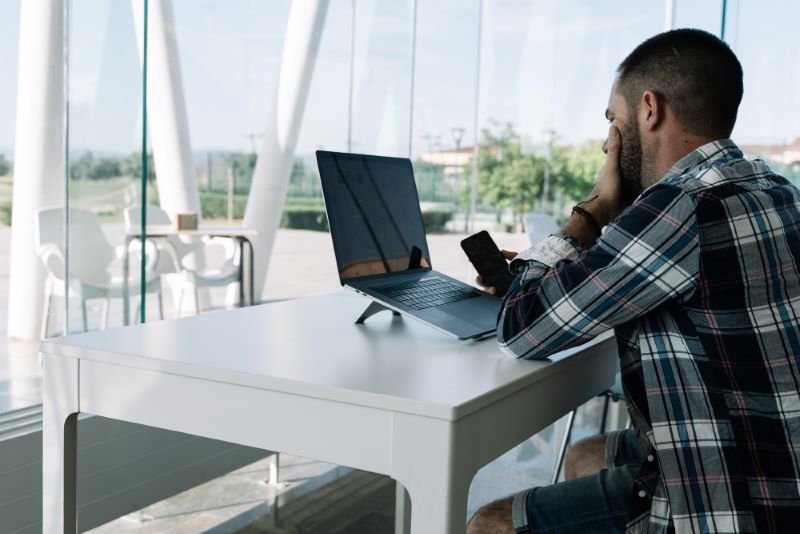
The main reasons for using web proxies are to hide your IP address and get past the rate limit set by the target website. Enterprises use Java web crawling and scraping for multiple reasons, such as price comparisons, identifying market trends, and getting an insight into customer sentiment.
Real estate listing
Real estate agents use web scraping for populating their database with properties available for rent and sale. For instance, real estate agencies can scrape data from Multiple Listing Services to build APIs that further populate the information about real estate listings on their website.
Price comparison websites
You can use web scraping to identify price trends of products over time or across geographies.
For instance, you may use web scraping in Java with JSoup Java to get a price comparison from your competitors. Suppose you’re introducing a smartwatch. You may want to get information about the prices your competitors are charging.
In this way, you’ll be able to come up with a price that’s not too low or too high.
Lead generation
Web scraping can also be used for lead generation, especially if you’re looking for a specific type of lead. Say, for example, you’re a real estate agent who specializes in luxury homes.
You could use web scraping to find people who have recently listed their homes for sale on the internet. This would give you a list of potential leads to contact and market your services to.
Another way that you can use web scraping is lead generation, by scraping job boards for potential candidates. This can be a great way to find people who are already interested in finding a new job.
By scraping job boards, you can get a list of potential candidates that you can then reach out to and see if they are interested in your services.
Market research
Marketing professionals use web crawling and data extraction tools to identify people’s thoughts about their products. This information can help improve the brand image, launch new products, and understand existing competition.
By web scraping with Java proxies, you can conduct market research effectively without the risk of getting banned. Even if you need to conduct market research in a different geographical location than your own, it’s possible to do so with proxies.
Proxies can go past the geographical barriers, making the website think it’s getting a request from a regional server. In this way, you can get information about a foreign market even if the websites have blocked access from your country.
FAQs: How to Web Scrape With Java
Here are some frequently asked questions to help you understand how to web scrape with Java.
Which language is best for web scraping?
The best programming language for web scraping is Python. It’s considered an all-rounder, since it can handle most web crawling and web scraping tasks easily. However, Java can also be used for web scraping, if you’re okay with getting a few libraries.
What do I need to web scrape into a database using java?
To scrape the web using Java, you need a web scraping framework and a database.
HtmlUnit and JSoup are commonly used web scraping frameworks that you can use with Java. In terms of databases, MongoDB and MySQL are popular choices.
What are the limitations of java for web scraping?
Java has some limitations when it comes to web scraping. Firstly, Java is not as powerful as Python for web crawling. Secondly, it can be difficult to extract data from complex HTML pages using Java code.
Despite these limitations, Java can still be used for basic web scraping tasks. If you need a more powerful and versatile solution, though, Python is the better option.
Which data should I scrape?
You can scrape different kinds of data, such as product data, pricing data, or consumer sentiment data.
For instance, if you want to scrape product data, you can collect information about the products on a given website, including the name of the product, the price, the description, and the manufacturer.
Final Words
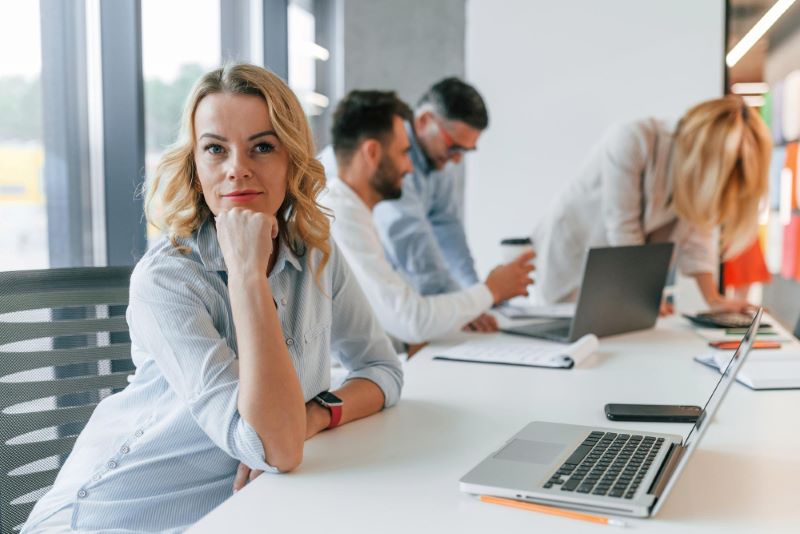
Summing up, web scraping with java can be a very powerful tool for data extraction and analysis. Data plays a huge role in decision-making in today’s time. Good knowledge of web scraping can be extremely useful in creating amazing content on your website to lure customers into buying products and services you promote.
It also helps you stay on top of market trends and competition. You can keep track of your competitors, such as the prices they’re offering and the trends they’re leveraging to make a place for themselves in the market.
To prevent bans and get easy access through geographical barriers, it’s best to use residential proxies, since they offer a wide range of IP addresses. Paid proxies work great for scraping, but make sure the provider is reputable and has a good track record.
By now, you should know how to web scrape in Java with JSoup and other popular libraries. Alternatively, you can use a scraping tool like Rayobyte’s Web Scraping API to collect the data for you while you manage other business operations.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.