The Complete Guide To Fixing Syntax Errors In Python
Python is a versatile and widely-used programming language for web scraping. It’s beloved by beginners and seasoned coders alike for its readability and simplicity. Its extensive collection of web scraping resources, such as Beautiful Soup and Scrapy make it even easier to use for data collection. But even in Python, learning to code is fraught with pitfalls. Read more about python syntax errors.
The most common issues are Python syntax errors. These mistakes in your code’s structure can stop your web scraping scripts and interrupt your progress. Syntax errors are an inevitable part of learning to navigate the intricacies of any programming language. But you’ll find it much easier if you learn the common Python syntax errors, how to solve them, and how to avoid them in the future.
What Are Syntax Errors in Python?
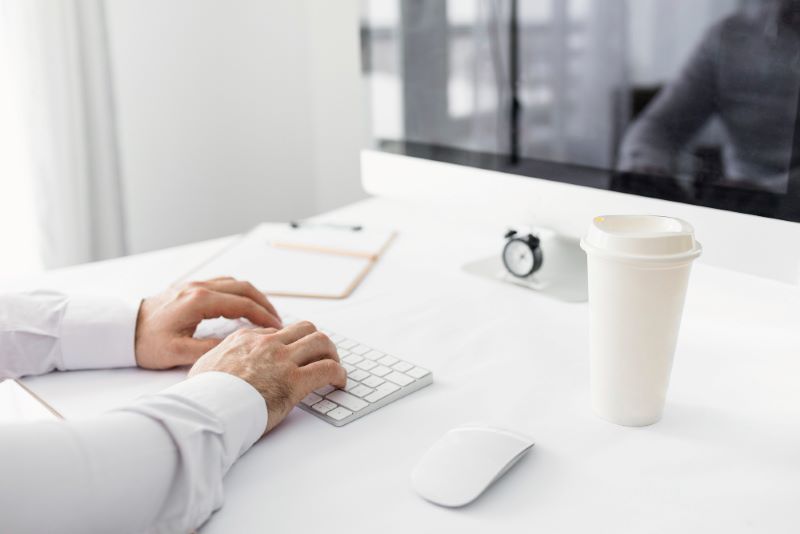
Syntax, in programming, is a set of rules that defines how programs written in a language must be structured. These rules dictate how statements and expressions are formed and how they should be written to create valid programs. When a programmer writes code that does not adhere to these rules, the Python interpreter cannot understand it and raises a syntax error.
Python syntax errors are also known as parsing errors, as they occur when parsing the code. They are the most common type of errors that you’ll encounter, especially when you’re new to programming.
Think of syntax in programming as similar to grammar in natural languages. If a sentence doesn’t follow the rules of grammar, it might be hard for the listener or reader to understand. If a program does not follow the syntax rules of its language, it cannot be understood — or “parsed” — by the computer.
Python’s syntax is designed to be readable and simple, which means the rules are straightforward and generally intuitive. For example, it denotes blocks of code by indentation rather than the brackets used in many other languages. However, even with Python’s simplicity, it’s easy to make mistakes like forgetting a colon at the end of a function definition or not properly aligning your code blocks.
When a Python syntax error occurs, the interpreter stops executing the program. It will also provide an error message (also known as a traceback) that points to the line of code where the error was detected and describes the nature of the error. Reading and understanding these error messages is crucial in debugging Python syntax errors.
There are different types of Python syntax errors, so you’ll need to know to read error messages and some tips for troubleshooting and preventing these errors.
Common Examples of Syntax Errors in Python
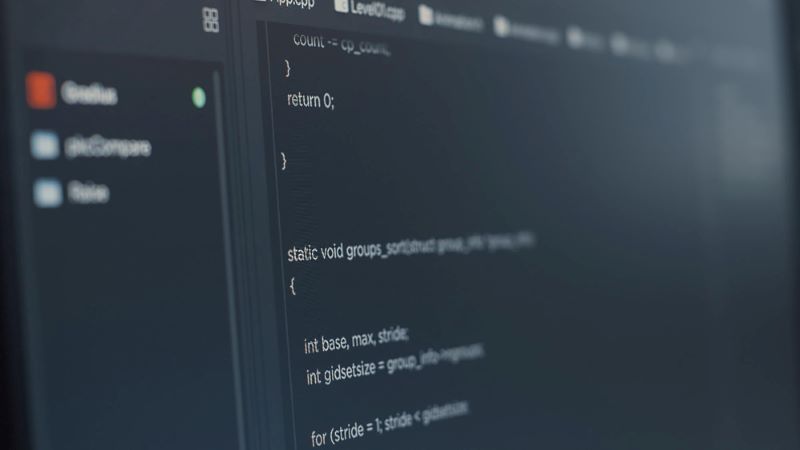
Here are some of the most common Python syntax errors that lead to the dreaded SyntaxError: invalid syntax message.
Missing parentheses, brackets, or curly braces
Python uses the following in various parts of its syntax:
- Parentheses (): Used in function calls and definitions
- Brackets []: Used for list and dictionary indexing
- Curly braces {}: Used for defining sets and dictionaries
Forgetting or mismatching these symbols is a common mistake. For instance, failing to close a parenthesis when defining a function or calling a method can lead to a Python syntax error.
Incorrect indentation
Indentation indicates a block of code, and Python uses it to determine the grouping of statements. Python syntax errors can occur when code is not indented correctly. Forgetting to indent the code inside a function, loop, or conditional statement can result in IndentationError.
String errors with single and double quotes
In Python, strings can be defined using either ‘ (single quotes) or “ (double quotes). A common Python syntax error is starting a string with one type of quote and trying to end it with the other or when trying to include the same kind of quote character within the string itself. This will cause a syntax error unless escaped properly using \ (backslash).
Invalid syntax errors
Invalid syntax errors are a bit of a catchall category when it comes to Python syntax errors, occurring when the parser encounters a line of code it doesn’t understand. This could be caused by various issues, like a missing colon at the end of a function definition or IF statement, using a Python keyword for a variable name, or trying to use a symbol that’s invalid in the current context.
How Python Interprets Code and How It Affects Python Syntax Errors
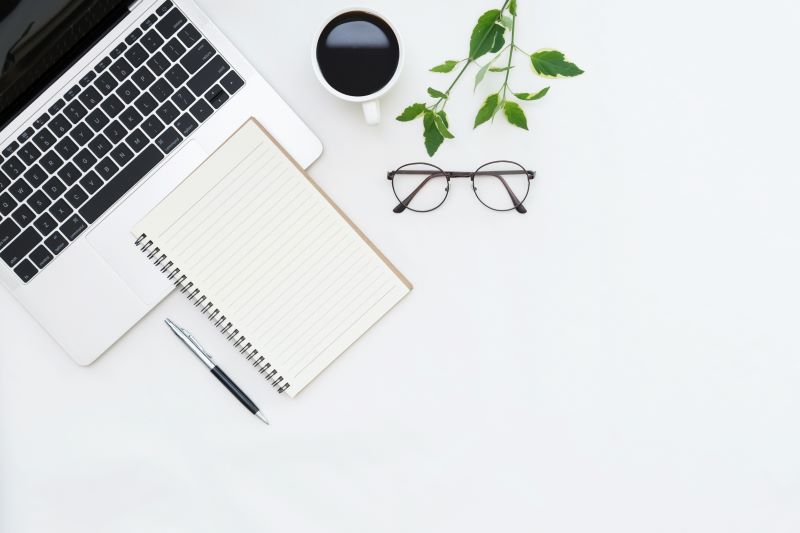
Understanding how Python interprets code is crucial for diagnosing and fixing Python syntax errors. Here’s a high-level view of the process.
Lexing
Lexing, short for lexical analysis, is the first phase of the compilation or interpretation process. The Python interpreter reads your source code as a sequence of characters and translates it into a sequence of lexical units or tokens. Each token is a meaningful character or sequence that has semantic meaning in the Python language, such as keywords, identifiers, literals, operators, etc.
Parsing
Parsing is the next step in interpreting Python code. Parsing applies the rules of a language to the sequence of tokens produced by the lexing phase and organizes them into a more complex structure that represents the syntactic structure of the program.
This structure is typically a treelike data structure called a parse tree or an Abstract Syntax Tree (AST). Python uses a parsing technique called LL(1) parsing, a type of top-down parsing. It starts from the root of the tree and works its way down, left to right.
Python syntax errors occur in this stage if the sequence of tokens doesn’t match any valid application of the grammar rules. For example, if you wrote a = 5 + and then forgot to include the second operand for the + operator, you would get a Python syntax error because + followed by nothing isn’t valid syntax according to Python’s grammar rules.
Compiling
Compiling is the next step in interpreting Python code once the source code has been lexed into tokens and parsed into an Abstract Syntax Tree (AST). During this phase, the AST is transformed into a lower-level form called bytecode.
Bytecode is a simplified, platform-independent form of machine code that is easy for the Python interpreter to execute. Each instruction in bytecode corresponds to a simple operation the interpreter can perform, like pushing a value onto a stack, performing an arithmetic operation, or calling a function.
The Python compiler translates each node in the AST into one or more bytecode instructions. For example, an addition operation in the AST might be compiled into bytecode instructions to load the operands onto a stack, perform the addition, and then store the result back into a variable.
While interesting, as a Python programmer, you typically don’t need to worry about bytecode. The Python interpreter handles all the details of compiling your code into bytecode and then executing that bytecode. This is part of what makes Python a high-level language: you can focus on the structure and logic of your program, and the interpreter takes care of the low-level details of how your code gets executed.
Interpreting
Interpreting is the final stage of the process through which Python runs your code. After the source code is lexed into tokens, parsed into an Abstract Syntax Tree (AST), and compiled into bytecode, the bytecode is fed into the Python interpreter, specifically the Python Virtual Machine (PVM), for execution.
The PVM is essentially a big loop that iterates through the bytecode instructions one by one and performs whatever operation each instruction specifies. These operations can be mathematical computations, memory assignments, control flow changes, etc.
One of the key benefits of this bytecode interpretation process is that it’s platform-independent. The same Python bytecode can be interpreted and run on any platform with a Python interpreter, whether it’s Windows, MacOS, Linux, or anything else.
Python’s interpreting process is what makes it an interpreted language. Compiled languages like C or C++ compile source code directly into machine code that can be run directly by a computer’s hardware.
Using an interpreter makes it easier to write and debug code, as you can catch and handle Python syntax errors more gracefully, but it can also make Python code slower to run than equivalent code in a compiled language. This trade-off between developer productivity and code execution speed is fundamental to Python’s design philosophy.
How the Interpreter Checks Python Code for Syntax Errors
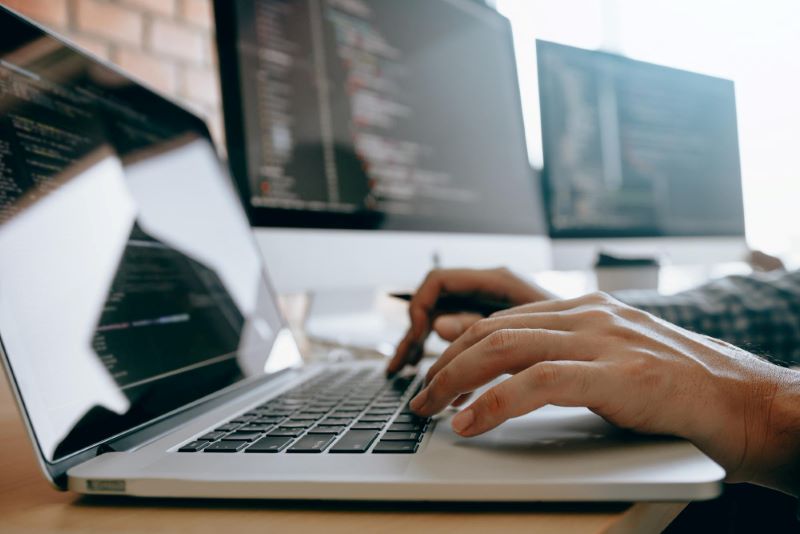
The interpreter plays a crucial role in identifying Python syntax errors. While reading, parsing, and executing your code, the interpreter checks it against Python’s syntax rules.
As mentioned earlier, Python parses tokens into a parse tree representing your program. While building this parse tree, the interpreter follows Python’s syntax rules. It cannot build a valid parse tree if it encounters a Python syntax error, such as a token or series of tokens that don’t fit these rules. In such cases, it raises a SyntaxError and provides an error message. This error message typically includes the file name and line number where the error was detected, along with a brief description.
For instance, if you forgot to add a closing parenthesis on a line of code, the interpreter would raise a Python syntax error with a message like this:
File “<stdin>”, line 1
print(“Hello, World!”
^
SyntaxError: unexpected EOF while parsing
The ^ character points to the place in the line where the Python interpreter detected a problem. In this case, the error message “unexpected EOF while parsing” tells you that the interpreter reached the end of the file (EOF) without finding a closing parenthesis.
While the interpreter is very good at catching Python syntax errors, it can sometimes get confused. For example, if you forget a closing parenthesis on one line, the interpreter might not raise an error until the next when it encounters a token that doesn’t make sense with the unclosed parenthesis. This can make Python syntax errors tricky to debug, especially in complex programs. That’s why understanding Python’s syntax rules and knowing how to read error messages are so important for Python programmers.
How To Fix a Syntax Error in Python: Strategies and Techniques
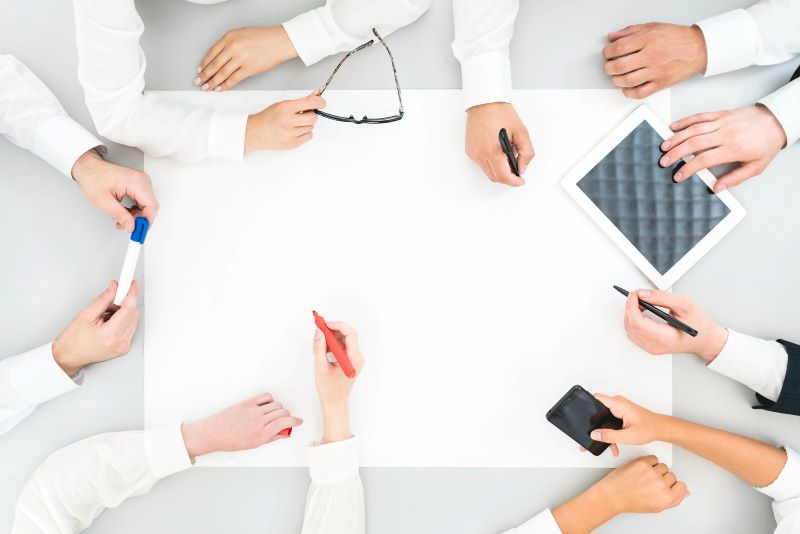
Python syntax errors can be frustrating, but you can resolve them effectively with the right approach and tools. Here are some ways you can check code for Python syntax errors.
Reading and understanding error messages
When your program encounters a Python syntax error, it stops execution and outputs an error message known as a traceback. This traceback contains valuable information that can help you locate and understand the nature of the error. Here’s how to interpret each part of the traceback.
File name and line number
The traceback begins with information about where the error occurred and includes the file name and the line number where the error was detected. For instance:
File “my_script.py”, line 5
The error was detected in the file “my_script.py” on line 5 in this case.
Code snippet
Next, the traceback shows the line of code where the error was detected:
print(“Hello, world!
This is the line of code from “my_script.py” that caused the error.
Indicator
Below the line of code, Python uses a caret (^) to indicate where the Python syntax error was detected on the line. In the following example, Python detected an error at the end of the line:
print(“Hello, world!
^
Error type and message
Finally, the traceback ends with the type of error and a brief message describing the error:
SyntaxError: EOL while scanning string literal
In this case, the error is a SyntaxError, which means there was a problem with the program’s syntax. The message “EOL while scanning string literal” means that Python reached the end of the line (EOL stands for end of line) while still looking for the end of a string.
By reading and understanding these Python syntax error messages, you can often identify and fix syntax errors without stepping through your code or using a debugger. However, error messages can sometimes be cryptic or misleading, especially to beginners. If you’re having trouble understanding a particular Python syntax error message, it can be helpful to search for the error type and message online. Chances are, someone else has encountered the same error and found a solution.
Using debugging tools
Debugging tools can significantly ease the process of finding and fixing errors in your code, including Python syntax errors. Here are some useful debugging tools for Python.
Integrated Development Environments (IDEs)
IDEs like PyCharm, Visual Studio Code, or Jupyter Notebook provide features like syntax highlighting and real-time error checking, which can help you spot and fix syntax errors as you write code. They also often include built-in debuggers, which allow you to step through your code line by line and inspect the state of your program at each step.
Python’s Built-in Debugger (pdb)
Python comes with a built-in debugger called pdb. The pdb module defines an interactive source code debugger for Python programs. It allows you to step through your code, examine data in detail, run code in the context of any stack frame, and alter the execution of your program.
Linters
A linter is a tool that analyzes your code to detect potential errors, bugs, stylistic errors, and suspicious constructs. In Python, popular linters include pylint and flake8.
- Pylint: Pylint is a highly configurable tool that checks for coding standards and has some nice features like checking line code’s length, checking if variable names are well-formed according to your coding standard, and more.
- Flake8: Flake8 is a wrapper around several tools, including PyFlakes, pycodestyle, and Ned Batchelder’s McCabe script. It is a great tool kit for checking your code base against coding style (PEP8), programming errors (like “library imported but unused” and “Undefined name”) and checking cyclomatic complexity.
Code formatters
Code formatters automatically format your code to conform to a specific style guide. They can save you time by automatically fixing Python syntax errors and other formatting issues and ensuring consistency across your codebase. In Python, popular code formatters include Black and autopep8.
- Black: Black is a code formatter that takes total control of your Python code formatting in an uncompromising way. You just run Black on your codebase, and it will reformat all of your code.
- autopep8: autopep8 is another code formatter that automatically formats Python code to conform to the PEP 8 style guide. It uses the pycodestyle utility to determine what parts of the code need to be formatted.
Online resources
Online resources can be extremely valuable when troubleshooting Python syntax errors or other programming issues. These resources often include detailed explanations, code examples, and community discussions that can help you understand and resolve your problem. Here are some of the most useful online resources for Python developers.
- Stack Overflow: Stack Overflow is one of the most popular online platforms for developers. You can search for your Python syntax error message or other problem, and you’ll likely find that other developers have found a solution. You can also ask your own questions. Remember to provide a minimal, reproducible example of your problem to get a helpful response.
- Python documentation: The official Python documentation is a comprehensive resource that covers all aspects of the Python language, including syntax, built-in functions, modules, and more. If you’re getting a Python syntax error, it can be helpful to check the documentation to ensure you’re using the correct syntax.
- GitHub: GitHub is a platform for code hosting with millions of open-source projects. You can often find solutions to your problems in the issues section of relevant projects. In addition, reading and understanding other people’s code is a great way to improve your own coding skills.
- Python community forums: Forums like the Python Forum or the Google Python Group are places where you can ask questions and get answers from experienced Python developers.
- Online courses and tutorials: Websites like Coursera, Udemy, Codecademy, and Khan Academy offer online courses that can help you learn Python and improve your coding skills. Many Python tutorials are also available on sites like Real Python, Tutorialspoint, and W3Schools.
When using these resources, remember that the goal isn’t just to find a solution to your immediate problem but also to learn from the problem and the solution. Over time, this will help you become a more effective and independent problem-solver.
Examples of Syntax Errors in Python and Their Solutions
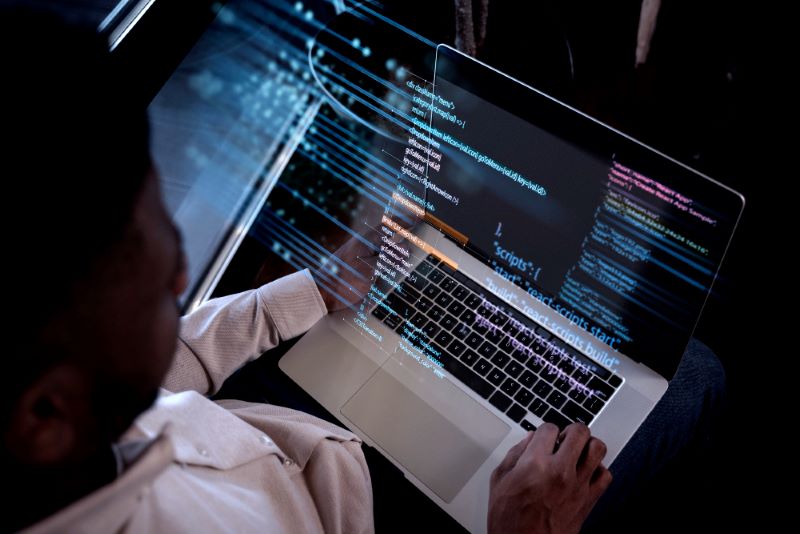
Here are some examples of Python syntax errors and how you might resolve them:
- Missing Parentheses in Call to ‘print’:
- Python 2.x supports print without parentheses, while Python 3.x requires parentheses.
- Error: print “Hello, world”
- Solution: print(“Hello, world”)
- Missing Colon:
- Python requires a colon at the end of the expression before starting a block of code.
- Error: if True print(“True”)
- Solution: if True: print(“True”)
- Mismatched Brackets:
- Brackets need to be properly opened and closed.
- Error: print(“Hello, world”
- Solution: print(“Hello, world”)
- Using = in Comparisons:
- Python uses == for comparison and = for assignment.
- Error: if a = 10: print(“a is 10”)
- Solution: if a == 10: print(“a is 10”)
- Undefined Variables:
- Python raises a NameError if you try to use a variable before it’s been defined.
- Error: print(x)
- Solution: x = 10 print(x)
- Improper use of the import statement:
- Python needs a module or package name after the import statement.
- Error: import
- Solution: import math
- Incorrect String Delimiters:
- Python strings should be enclosed by either single (”) or double (“”) quotes.
- Error: print(“Hello, world’)
- Solution: print(‘Hello, world’) or print(“Hello, world”)
Remember, Python is case-sensitive and pays attention to whitespace. Both of these factors can lead to syntax errors if not properly managed.
Preventing SyntaxError: Invalid Syntax
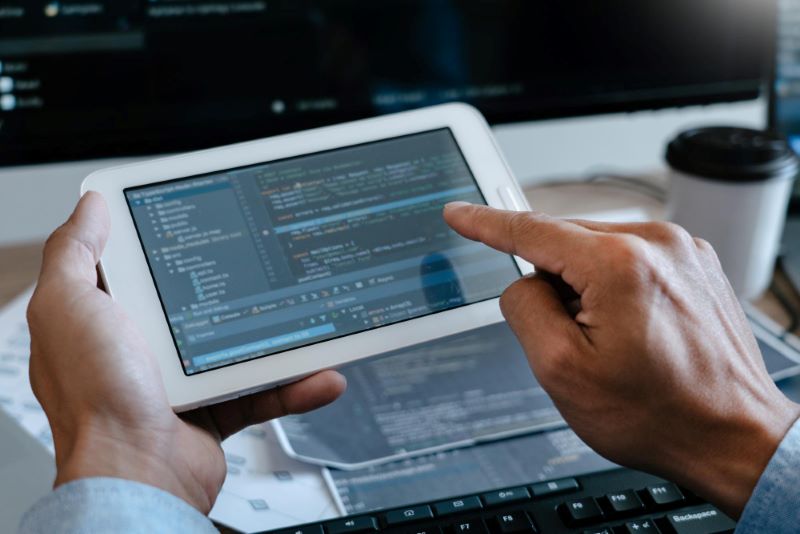
Preventing Python syntax errors is largely about adopting good coding practices. Here are some best practices that can help you write clean, error-free Python code:
Follow the PEP 8 style guide
PEP 8, also known as the Python Enhancement Proposal 8, is the official style guide for writing Python code. It was written to help Python developers write more readable and consistent code. Here are some of the key guidelines from PEP 8 that can help prevent Python syntax errors and improve the readability of your code:
- Indentation: Use four spaces per indentation level. This is preferred over tabs.
- Maximum line length: Limit all lines to a maximum of 79 characters. For flowing long blocks of text with fewer structural restrictions (docstrings or comments), limit the line length to 72 characters.
- Blank lines: Separate top-level function and class definitions with two blank lines. Method definitions inside a class are separated by a single blank line.
- Imports: Imports should usually be on separate lines and placed at the top of the file, after any module comments and docstrings but before module globals and constants.
- Whitespace in expressions and statements: Avoid extraneous whitespace immediately inside parentheses, brackets, or braces and immediately before a comma, semicolon, or colon. Use whitespace in a way that is visually clear.
- Comments: Comments should be complete sentences. If a comment is a phrase or sentence, its first word should be capitalized unless it is an identifier that begins with a lowercase letter.
These are just a few examples of the guidelines in PEP 8. You can refer to the full PEP 8 documentation for more detailed information. Following these guidelines can help you write more readable and maintainable Python code and can also help prevent syntax errors.
Use linters and code formatters
As mentioned earlier, using linters and code formatters in your development process can greatly help prevent a number of Python syntax errors and ensure that the code adheres to Python’s best practices. They can be integrated into most text editors and IDEs, and many teams include them as part of their continuous integration setup so that code is automatically checked and formatted whenever it’s committed to a version control repository.
- Linters analyze your code and warn about potential errors before you run your program. Linters can prevent Python syntax errors and enforce coding standards and best practices. Popular Python linters include the pylint and flake8 linters covered under fixing Python syntax errors. These linters are great for maintaining a consistent coding style.
- Code formatters like Black or autopep8 (also covered earlier) can automatically format your code according to Python’s PEP 8 style guide. That will help prevent Python syntax errors by ensuring your code is properly structured and formatted.
Write simple and clear code
Writing simple and clear code is not only a best practice for Python but for all programming languages. It enhances code readability and maintainability and reduces the chance of introducing errors. Here are some recommendations for writing simple and clear code.
- Use descriptive names: Use clear and descriptive names for variables, functions, and classes. Names should reflect the purpose or meaning of the item. For instance, a variable holding a user’s email address might be called user_email, rather than something generic like x or data. Creating clear descriptions of elements in your code takes advantage of Python’s readability and can make Python syntax errors more noticeable.
- Break down complex functions: If a function becomes overly complex, consider breaking it down into smaller, simpler ones. Each function should ideally have a single responsibility. This reduces the chances of syntax errors due to overly complex code.
- Keep lines short: Long lines of code can be hard to read, especially if you have to scroll horizontally. Try to keep lines of code short, ideally not exceeding 79 characters, as recommended by PEP 8, so Python syntax errors are easy to spot.
- Avoid deep nesting: Deeply nested code — code with many levels of indentation due to loops, conditionals, or function calls — can be hard to read and understand. Try to keep your code as flat as possible to reduce the likelihood of omitting critical syntax elements.
- Use consistent formatting: Consistent formatting makes your code easier to read. This includes consistent indentation, spacing, and use of brackets and parentheses, which are frequently associated with Python syntax errors. Following a style guide like PEP 8 can help with this.
- Prioritize readability: Sometimes, there are clever or “tricky” ways to achieve something in fewer lines of code. However, if this makes your code harder to understand, it’s usually better to stick with a more straightforward solution so you don’t have to navigate unfamiliar notation looking for Python syntax errors.
Use comments and docstrings
Comments and docstrings are an important part of writing clean, maintainable Python code. They document your code, making it easier for others — and your future self — to understand what your code is doing and why. Taking shortcuts with your code can cause you to overlook common Python syntax errors, so make sure you take the time to explain a step when needed.
Comments:
In Python, comments are lines of text in your code that the interpreter ignores. They are used to explain what the code does or provide additional context. Comments in Python start with the # character.
Here’s an example of a comment in Python:
# Calculate the square of the number square = number ** 2
Good comments are concise and to the point, and they explain things that the code doesn’t make obvious. However, it’s generally better not to rely on comments to make your code understandable.
Convoluted code is more prone to Python syntax errors, and comments are no substitute for straightforward code. Avoid using comments to explain what your code is doing, and write your code so it’s self-explanatory. Use comments to explain why your code is doing something if it’s not immediately obvious. You’re less likely to make a Python syntax error with less extraneous information in your code.
Docstrings
Docstrings, short for “documentation strings,” are a type of comment used to explain the purpose of a function, method, class, or module. They are enclosed in triple quotes (“””) and can span multiple lines.
Here’s an example of a docstring for a function:
def add_numbers(a, b): “”” Add two numbers together. Parameters: a (int): The first number to add. b (int): The second number to add. Returns: int: The sum of the two numbers. “”” return a + b
In addition to explaining what the function does, this docstring explains the function’s parameters and return value. This can be very helpful for someone trying to understand or use this function.
Docstrings can be accessed at runtime using the .__doc__ attribute, making them useful for automatically generating documentation. Tools like Sphinx can generate HTML documentation from your docstrings, and interactive environments like Jupyter and IPython display your docstrings as help messages.
The goal of comments and docstrings is to make your code easier to understand. They should provide information that the code itself doesn’t make obvious. If you find yourself writing a comment to explain what a complex line of code is doing, it might be better to rewrite that line in a simpler, more understandable way. Simple code is easier to read, making it more likely that you’ll spot Python syntax errors before you run your program.
Test your code regularly
Testing your code regularly is an integral part of software development that helps ensure your code is functioning as expected. This practice is particularly useful in catching and preventing errors, including Python syntax errors. Here are some aspects to consider.
- Unit testing: Unit testing involves testing individual components of your software (like functions or methods) in isolation. This helps ensure that each component is working correctly. Python’s built-in unit test module is a valuable tool, as are third-party libraries like pytest.
- Test-Driven Development (TDD): In TDD, you write tests for your code before writing the code itself. Start by writing a test that fails, then write the minimum amount of code necessary to pass the test. Finally, refactor your code to the ideal solution. TDD helps ensure that your code is thoroughly tested and encourages you to think about your code’s behavior before implementation.
- Continuous testing: Continuous testing is the practice of running your tests every time you make changes to your code. This can be automated using a continuous integration service, which will automatically run your tests every time you commit changes to your code repository. It can help you catch and fix errors as soon as they’re introduced.
- Regression testing: When you fix a bug or add a new feature, it’s important to test the changed code and other parts of your software that might be affected by the change. This helps catch regression errors, where a change unintentionally breaks something that was working before.
Remember, the goal of testing isn’t just to find bugs but also to provide assurance that your code is working correctly. Well-written tests can also serve as documentation, showing others (and your future self) how your code is supposed to be used.
Use version control
Version control is a system that records changes to a file or set of files over time so that you can recall specific versions later. It is a crucial tool in modern software development practices, and here’s how it can help prevent Python syntax errors:
- Track changes: Version control allows you to keep a history of changes to your code. This can be extremely useful when trying to identify when and where a bug was introduced. You can look back at the changes you made to your code and see what might have caused the problem.
- Collaborative work: If you’re working on a project with others, version control is essential. It allows multiple people to work on the same codebase without overwriting each other’s changes. You can also see who made which changes, which can be helpful when you’re trying to understand a piece of code or identify the cause of a syntax error.
- Backup and restore: With version control, every copy of the codebase is a full backup. This means you can recover your code from any point in its history. If you introduce a syntax error and can’t figure out how to fix it, you can simply revert your code to a previous state.
- Experimentation: Version control makes it easy to create separate “branches” of your code, so you can experiment with different approaches without affecting the main (“master” or “main”) branch. Once you’re satisfied with your changes, you can merge them back into the main branch.
One of the most popular version control systems is Git, and GitHub is a hosting service for Git repositories. They offer a web-based graphical interface and provide access control and several collaboration features such as bug tracking, feature requests, task management, and wikis for every project.
Final Thoughts
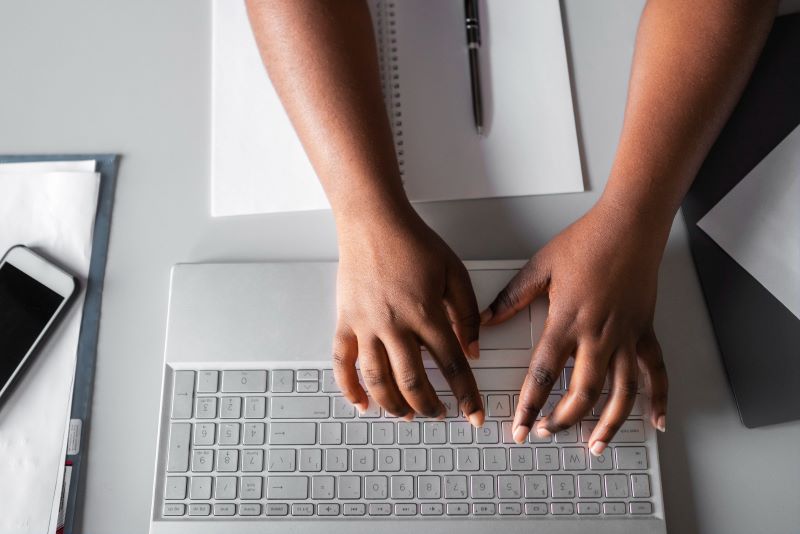
Python syntax errors occur when the Python interpreter encounters code that violates Python’s grammar rules. Syntax errors are quite common, especially for beginners. They can be caused by various mistakes such as incorrect indentation, missing or extra punctuation, incorrect usage of operators and constructs, etc.
The best way to avoid syntax errors is to gain a solid understanding of Python’s syntax rules, write code carefully, and frequently test the code to ensure changes do not introduce new syntax errors that could interfere with web scraping. Working with an easy language like Python and high-quality proxies from Rayobyte can help you make the most of your data strategy. Reach out today to find out how our experts can partner with you for your data needs.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.