Python Requests and the Use of a Proxy in 2024
Table of Contents
Python requests, proxy usage, data scraping — it’s easy to read terms like these and think you’ve stumbled across a foreign language. Read more about python requests proxy.
The good news is that we’ve put together this in-depth guide that can help you understand proxies, Python requests and the use of a proxy, and everything in between. Find all the essentials you need to know below.
Proxy Basics
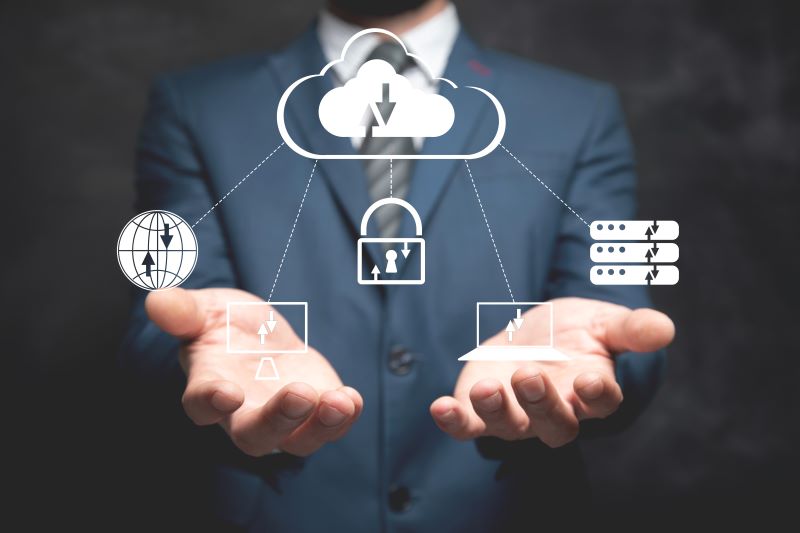
Let’s begin with some basic information regarding proxies before bringing the Python requests into the equation.
A proxy is an intermediary server or software that acts as a gateway between a user’s device (a computer, smartphone, etc.) and the internet. The primary purposes of using a proxy include:
- Anonymity: Proxies can mask a user’s IP address, providing a degree of anonymity. When you connect to the internet through a proxy, websites and online services see the proxy server’s IP address instead of your own — this can be especially handy when carrying out tasks like website scraping.
- Access Control: You can use proxies to restrict or control access to certain websites or online content. This is often implemented in organizations to manage and monitor internet usage.
- Content Filtering: You can configure proxies to filter content, blocking access to specific websites or types of content deemed inappropriate or against company policies.
- Caching: Proxies can cache frequently accessed web pages and resources, reducing the load on the origin server and speeding up access to those resources for subsequent requests.
- Security: Proxies can act as an additional layer of protection by filtering out malicious content, blocking suspicious websites, and protecting against certain types of cyber attacks.
There are several different types of proxies you can utilize to experience the benefits shared above, but the following are some of the most well-known options:
HTTP proxies
Hypertext Transfer Protocol (HTTP) proxies handle HTTP traffic. They are commonly used for web browsing.
HTTPS proxies
Hypertext Transfer Protocol over Secure Socket Layer (HTTPS) proxies are similar to HTTP proxies. However, they are designed to handle secure HTTPS traffic.
SOCKS proxies
Socket Secure, or SOCKS, proxies are more versatile than HTTP and HTTPS proxies, as they can handle various types of traffic, including TCP and UDP. They are commonly used for activities like online gaming.
What Are Python Requests?
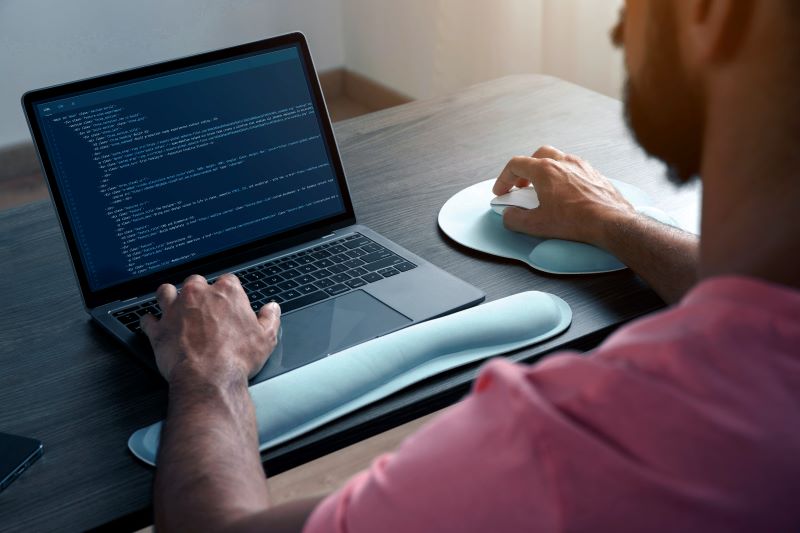
Now that you’re caught up on proxies, let’s discuss Python requests.
Python requests is a library that you can use to make HTTP requests. It offers an easy-to-navigate interface that simplifies the process of sending and receiving data from websites. Python requests are fast, support multiple languages, and can be incorporated into other programs, making processing tasks easier.
Here are some of the most common types of requests you can make using the requests library:
GET request
import requests
response = requests.get(‘https://example.com’)
POST request
import requests
data = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
response = requests.post(‘https://example.com/post_endpoint’, data=data)
PUT request
import requests
data = {‘key1’: ‘new_value1’, ‘key2’: ‘new_value2’}
response = requests.put(‘https://example.com/put_endpoint’, data=data)
DELETE request
import requests
response = requests.delete(‘https://example.com/delete_endpoint’)
Benefits of Using Python Requests with Proxies
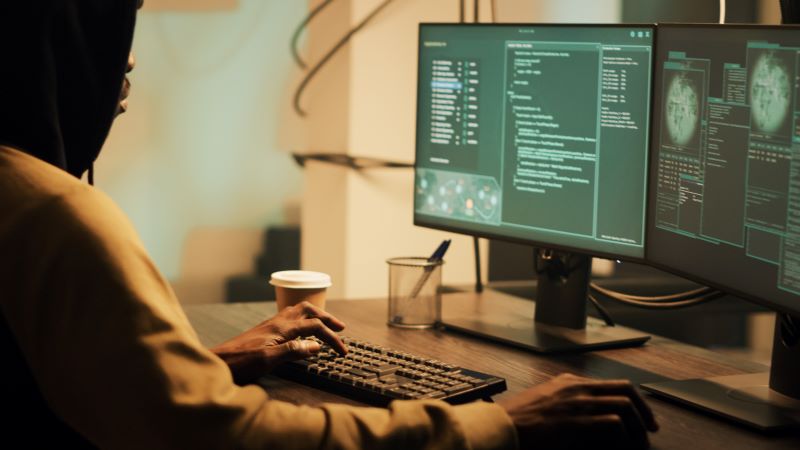
Now, let’s bring everything together. Why would you want to use Python requests with proxy?
Using Python requests with proxies offers several benefits in different scenarios, including those listed below:
Privacy and anonymity
Proxies can help you mask your IP address, providing a superior level of anonymity while making Python requests. This can be useful when you want to protect your identity or avoid being tracked by websites.
Bypassing Geographic Restrictions
Proxies allow you to route your requests through servers in different locations, potentially bypassing the geographical restrictions imposed by certain websites or services.
Web Scraping and Data Collection
For web scraping and data collection tasks, using proxies can help distribute requests across multiple IP addresses. Doing so reduces the likelihood that you will be blocked by websites that impose rate limits or other restrictions.
Load Balancing
Proxies can also be used for load balancing. They distribute requests across multiple servers to optimize performance and prevent overloading a single server.
Avoiding IP Blocking and Rate Limiting
Some websites or APIs may implement IP blocking or rate limiting to prevent abuse. Using proxies allows you to rotate IP addresses, making it more challenging for a website to block your requests or enforce rate limits.
Testing and Development
Proxies are useful for testing applications in different network environments. They allow developers to simulate requests from different locations and test how their applications handle various network conditions.
Security
Proxies can add an extra layer of security by hiding the original IP address of the user. This can be beneficial when you want to protect your system from potential security threats.
What Is a Python Proxy Server?
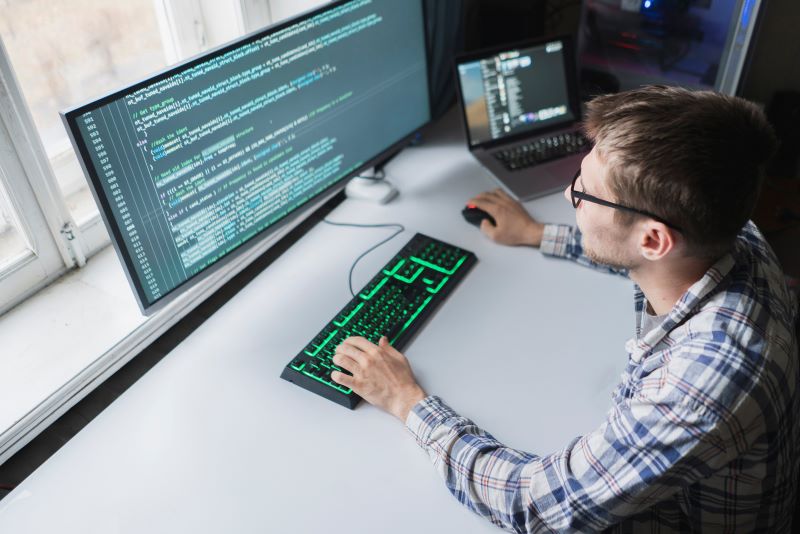
A proxy server is an intermediate server that acts as a gateway between a user’s device (such as a computer or smartphone) and the internet.
When a user requests a resource (like a web page or file), the request is first sent to the proxy server, which then forwards the request to the internet on behalf of the user. Similarly, when the internet responds, the proxy server receives the data and sends it back to the user.
A Python proxy server is a proxy server implemented using the Python programming language. In this context, a proxy server written in Python can serve various purposes, such as handling and forwarding network requests, modifying requests or responses, or providing additional functionalities like caching or security measures.
How to Set Up an HTTP/HTTPS Proxy Python Server
Setting up an HTTPS/HTTP proxy Python server might seem complicated at first. If you follow these steps, though, it’s a relatively straightforward process:
Step 1: Install proxy.py
Install proxy.py (this is a Python library that provides the tools and infrastructure needed to build and configure proxy servers).
You can install proxy.py using pip, Python’s package manager. To do so, open your terminal and run this command:
pip install proxy.py
Step 2: Setup and configuration
After you install proxy.py, configure it for basic usage by running the following command:
proxy
This will start a proxy server. From here, you can specify the IP address and port as command-line arguments. For example, you could type in the following:
proxy –hostname 0.0.0.0 –port 9000
Step 3: Create a simple proxy server
Now that Proxy.py is up and running, it’s time to set up a standard proxy server.
Configure your HTTP or HTTPS requests to route through the proxy server in your web scraping script. For instance, if you’re using Python’s requests library, you could do this as follows:
import requests
proxies = {
‘http’: ‘http://0.0.0.0:9000,
‘https’: ‘http://0.0.0.0:9000,
}
response = requests.get(‘http://example.com’, proxies=proxies)
print(response.text)
Step 4: Test and verify
Run your script after setting up the web scraper to use the proxy server. If you set everything up correctly, the scraper should successfully collect data using the proxy server. Check the proxy.py logs — you should see incoming requests.
Can You Use Python Requests with a SOCKS Proxy?
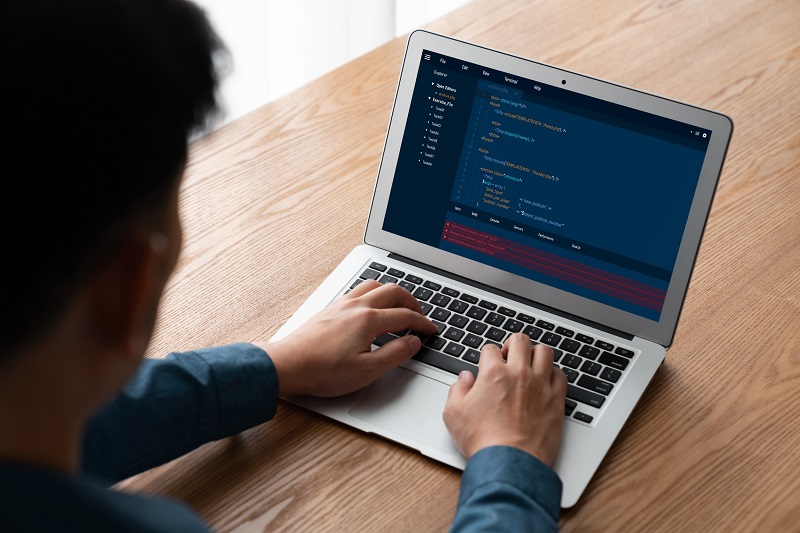
The short answer is yes, you can use the Requests library with a SOCKS proxy in Python. This functionality is not built directly into Requests, though.
To make it work, you’ll have to install another dependency called requests[socks]. This dependency allows you to enable SOCKS support.
You can install the necessary package using pip:
pip install requests[socks]
You can also install the dependencies separately:
pip install requests pysocks
Once you have installed the required package (or packages), you can use a SOCKS proxy with Requests.
Here is an example of how you might use a SOCKS proxy:
import requests
proxies = {
‘http’: ‘socks5://user:password@host:port’,
‘https’: ‘socks5://user:password@host:port’
}
response = requests.get(‘http://example.com’, proxies=proxies)
print(response.text)
Remember that you need to replace the user, password, host, and port with your real credentials and server details.
Advanced Techniques with Python Requests & Proxy Usage
Proxy.py is a highly configurable library that you can customize to suit specific needs and goals. Here are some advanced features you can utilize after you master the basics.
Rotating proxies for scraping
Web scrapers can rotate proxies to avoid detection. A rotating proxy will periodically send each request to a different proxy server, spreading the scraping burden among IP addresses and making it harder for websites to detect and halt it.
Threading
Thanks to its threading capabilities, proxy.py can handle multiple requests at once. You can also enable threading if needed with the –threaded flag when starting the proxy. This is particularly useful when you need to handle many connections simultaneously.
Plugin system
One of the most beneficial features of Proxy.py is its plugin-based architecture.
Plugins help you extend a proxy server’s functionality. For example, you can write custom plugins for various tasks, from modifying request headers and logging to request filtering and building custom caching layers.
To use a plugin, pass it as a command-line argument while starting the proxy server. For example, you could type in the following:
proxy –plugins plugin_module.PluginClassName
Custom code
You can also write plugins for specific tasks, such as rotating IPs, handling CAPTCHAs, managing sessions, or modifying user agents. These customization options allow you to tailor the proxy server to your unique needs.
Handling complex scenarios
Say you need to scrape a website that has rate-limiting. In that case, you could write a plugin to rotate IP addresses or add delays between requests.
Performance optimization
You can optimize the performance of proxy.py by adjusting its configuration parameters. For example, you might change the number of threads, enable or disable specific plugins, or adjust timeout settings.
Security enhancements
If you are dealing with sensitive data, you can enhance the security of your proxy server with encryption plugins or other plugins that anonymize your data.
Python Proxy Server HTTPS/HTTP Pros and Cons
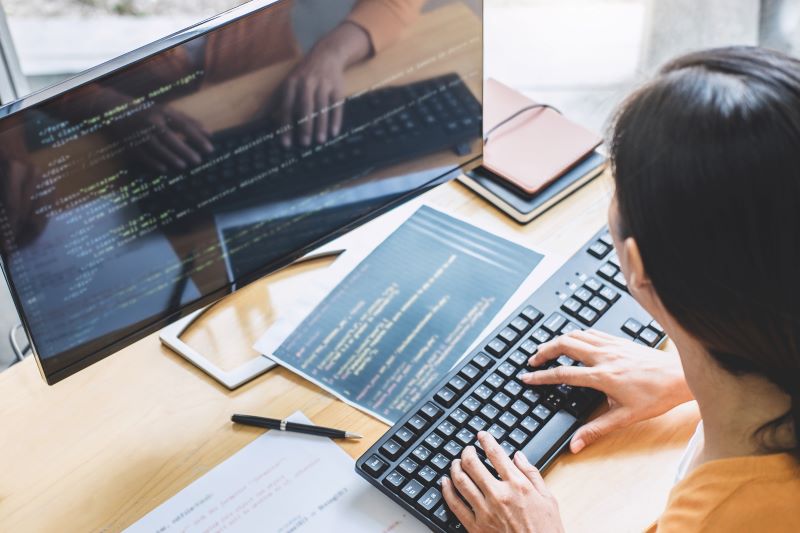
There are pros and cons that come with implementing a Python-based proxy server. The following are some of the most noteworthy ones to keep in mind.
Pro: Customization
Using Python allows for a high level of customization and flexibility in building a proxy server tailored to specific needs. Python’s ease of use and extensive libraries can expedite development.
Pro: Rapid development
Python is known for its concise syntax and quick development cycle. This can be advantageous when prototyping or deploying a proxy server in a short timeframe.
Pro: Rich ecosystem
Python has a vast ecosystem of libraries and frameworks. Leveraging these resources can simplify the development process and enhance the functionality of the proxy server.
Pro: Cross-platform compatibility
Python is cross-platform, making it easy to deploy proxy servers on various operating systems without major modifications.
Pro: Community support
Python has a large and active community, which means that developers can find ample resources, documentation, and support when working on a Python-based proxy server.
Pro: Integration with web frameworks
Python’s compatibility with web frameworks like Flask or Django allows for the easy integration of a proxy server into a larger web application or service.
Con: Performance
Python, compared to lower-level languages, may introduce some performance overhead. For high-performance scenarios, especially in network-intensive applications like proxy servers, this could be a limitation.
Con: Resource consumption
Python may consume more resources (CPU and memory) compared to lower-level languages, which might be a concern in resource-constrained environments or high-traffic scenarios.
Con: Concurrency
While Python has solutions for asynchronous programming (e.g., asyncio), it may not perform as well as languages explicitly designed for concurrency in scenarios with a high volume of simultaneous connections.
Con: Security concerns
The ease of development in Python might also lead to security oversights if not careful. Proper attention to security practices is crucial to avoid vulnerabilities in the proxy server.
Con: Dependency management
Python projects often depend on external libraries. Managing dependencies and ensuring compatibility between different library versions can be challenging and may introduce maintenance issues.
Con: Limited low-level control
Python’s high-level nature means that some low-level networking controls available in languages like C may not be as easily accessible. This could be a limitation in scenarios where fine-grained control is essential.
Con: Scalability
While Python is suitable for many applications, it might not be the best choice for extraordinarily high-traffic or resource-demanding scenarios where other languages may offer better scalability.
Troubleshooting Python Requests with Proxies
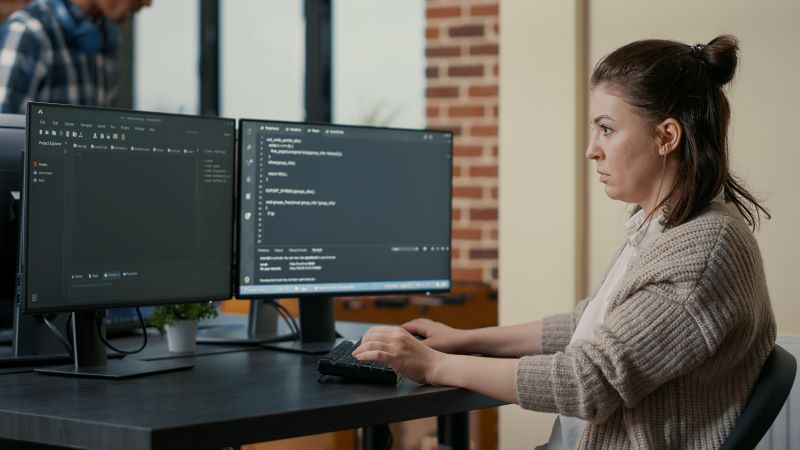
When using proxies with the requests library in Python, you may encounter issues that require troubleshooting. Here are some common problems you might face with potential solutions:
Proxy configuration
A good starting point when you run into trouble is to ensure that you have correctly configured the proxy settings. Remember that you can pass the proxy information as a dictionary to the proxy parameter in the request by doing the following:
import requests
proxy = {
‘http’: ‘http://proxy.example.com:8080’,
‘https’: ‘http://proxy.example.com:8080’,
}
response = requests.get(‘https://example.com’, proxies=proxy)
Proxy authentication
If your proxy requires authentication, be sure to provide the correct username and password. You could do this as follows:
import requests
proxy = {
‘http’: ‘http://username:[email protected]:8080’,
‘https’: ‘http://username:[email protected]:8080’,
}
response = requests.get(‘https://example.com’, proxies=proxy)
Connection issues
Check to see if you can access the proxy server and the target URL manually. Ensure that no network issues or firewalls are blocking the connection.
SSL/TLS issues
If you are making HTTPS requests through a proxy, make sure you handle SSL/TLS properly. You might need to turn off SSL verification for the proxy.
Here’s an example of how you could do this:
import requests
proxy = {
‘http’: ‘http://proxy.example.com:8080’,
‘https’: ‘http://proxy.example.com:8080’,
}
response = requests.get(‘https://example.com’, proxies=proxy, verify=False)
Check proxy logs
Inspect the logs of the proxy server for any error messages or issues. Doing so can help you identify whether or not the problem is on the proxy side.
Update requests library
Ensure that you are using the most up-to-date version of the requests library. Newer versions may include bug fixes and other improvements.
Test with a different proxy
If possible, test with a different proxy to see if the issue persists. This can help determine if the problem is specific to a particular proxy server.
What to Look for in a Proxy Provider
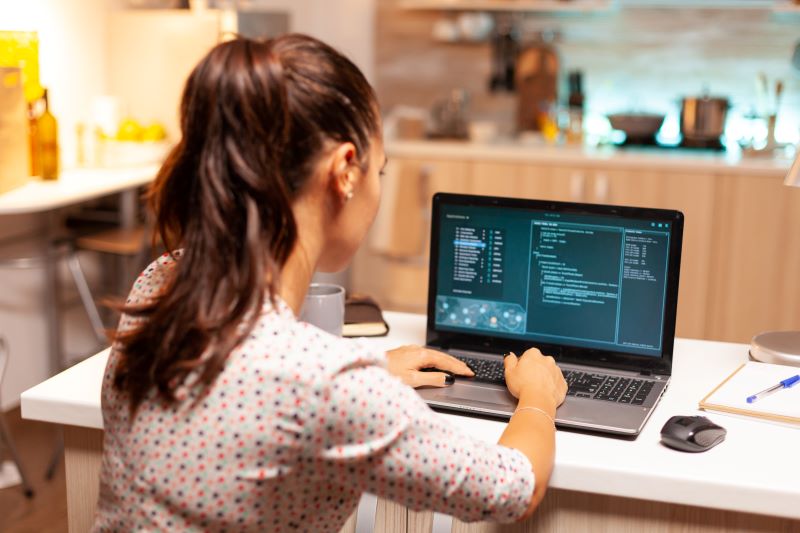
Maybe you aren’t confident in your coding abilities, or perhaps you are looking for a more efficient proxy solution. In either case, there are many trustworthy and reliable proxy providers you can partner with.
Here are some of the most important factors to consider when choosing a proxy provider:
Type of proxies
Start by reviewing the type (or types) of proxies the provider offers, such as the following:
- Residential Proxies: Residential proxies are sourced from real residential IP addresses, making them more legitimate and less likely to be blocked.
- Datacenter Proxies: Datacenter proxies are from servers and, in some cases, could be more prone to detection.
- Rotating Proxies: Rotating proxies change IP addresses at regular intervals.
- Static Proxies: Static proxies maintain a fixed IP address.
All of these proxy types come with benefits and drawbacks, so it’s essential to consider your specific needs and goals before making a final decision. A good proxy provider should be able to share additional details to help you pick the correct option.
Geographic coverage
Ensure the proxy provider offers a diverse range of IP addresses from various locations worldwide. This is essential for tasks that require geographically distributed requests.
Reliability and uptime
Look for a provider with high reliability and minimal downtime. Check if they provide any uptime guarantees in their service level agreements.
Speed and performance
Test the speed of the proxies to ensure they meet your performance requirements. Faster proxies contribute to better response times for your requests and allow you to work more efficiently.
Protocol support
Verify that the proxy provider supports the protocols you need, such as HTTP, HTTPS, SOCKS, etc. The type of protocols required depends on your specific use case.
Authentication methods
Check the authentication methods offered by the proxy provider. Username/password authentication or IP whitelisting are common methods. Some providers may also offer API access for better automation.
Scalability
Consider the scalability of the proxy solution. Ensure that the provider can accommodate your growing needs regarding the number of proxies and the traffic volume.
Security and anonymity
If anonymity is crucial, ensure the proxy provider prioritizes security and doesn’t log user activity. Look for providers that offer features like IP rotation to enhance anonymity.
Customer support
Take into account the amount of customer support the proxy provider offers. The more responsive and knowledgeable their customer support team is, the easier it will be to resolve issues quickly.
Trial period
Choose a provider that offers a trial period or a money-back guarantee. This allows you to test the proxies and ensure they meet your requirements before making a long-term commitment.
Cost and pricing structure
Consider the pricing structure and ensure it aligns with your budget. Some providers charge based on bandwidth usage, while others may have a subscription-based model.
Reviews and reputation
Research reviews and testimonials from other users to gauge the reputation of the proxy provider. Look for providers with positive feedback regarding reliability and customer support.
Conclusion
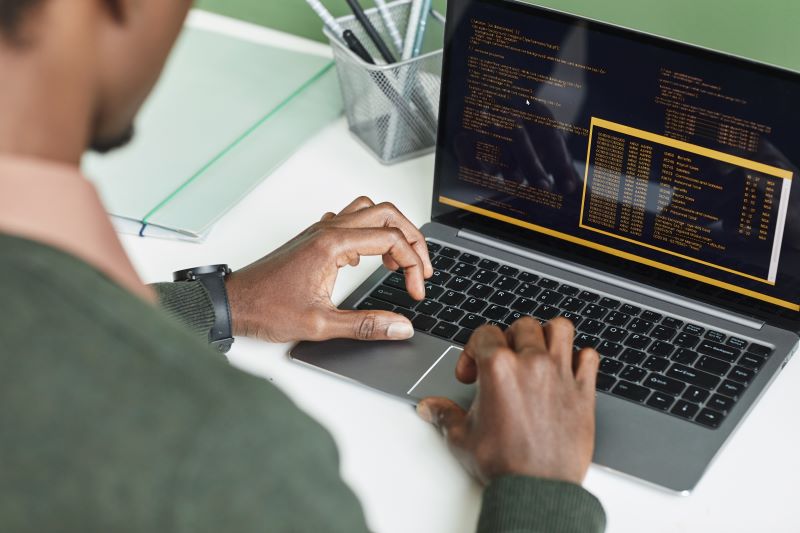
Proxies offer many benefits when you’re trying to maintain anonymity while making Python requests.
Whether you want to set up your own Python proxy server or work with a proxy provider, the information in this guide can help you get started and experience all the benefits proxies can provide.
Are you looking for a reliable and reputable proxy provider? Rayobyte has got you covered.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.
Sign Up for our Mailing List
To get exclusive deals and more information about proxies.
Start a risk-free, money-back guarantee trial today and see the Rayobyte
difference for yourself!