The Ultimate Guide to Make HTTP Requests in Node.js With Fetch API
Nowadays, there is an alternative to JavaScript allowing client-server communication without reloading web pages — Fetch API. It’s still experimental but nevertheless available in Node.js. That means it’s now possible to use JavaScript for server-side code, too. So, what exactly is Fetch API? And why should you consider using it instead of Axios or XHR?
Fetch API provides a more streamlined approach when compared with existing technologies such as XHR and Axios. With these, developers can make requests back and forth between the client side and server side quickly, without experiencing page reloads. Fetch API is a big deal because it’s incredibly efficient, allowing developers to make requests with minimal overhead. In other words, websites and applications can be developed significantly faster than before, since you don’t have to wait for pages to reload anymore.
Additionally, Fetch API offers support for both CORS (Cross-Origin Resource Sharing) and HTTP/2 (Hypertext Transfer Protocol). These protocols allow data transfer between web servers in an optimized way which further speeds up development time by reducing the amount of code needed. Finally, Fetch API also supports streaming. So, developers can deliver content in real-time without having to manually manage connections or resources. Thus, your users never miss out on the latest updates. Additionally, you can make an HTTP request (NodeJS) via Fetch API today.
How to Make HTTP Requests (Node.js)?
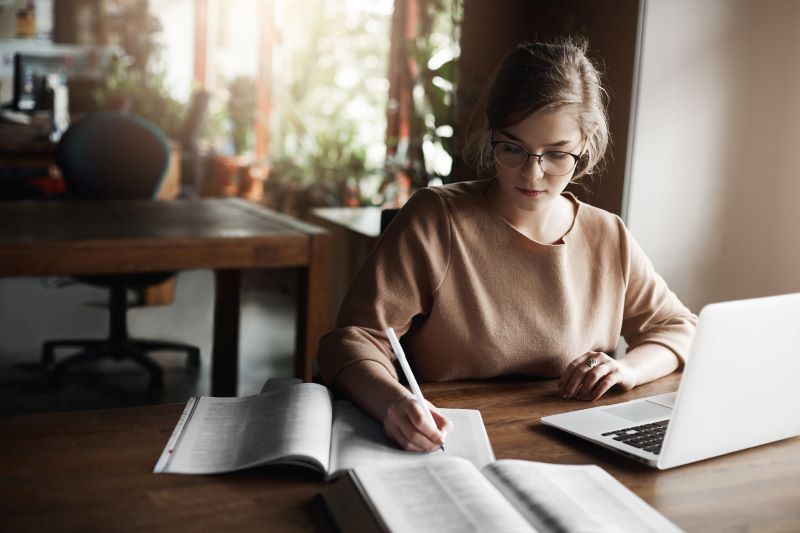
Node.js is a JavaScript runtime environment that enables developers to build server-side applications with JavaScript. It’s based on Google Chrome’s V8 engine and allows for the use of asynchronous programming techniques such as callbacks, promises, and async/await functions. Node also provides access to APIs like HTTP requests so that you can make requests directly from your codebase without needing any libraries or frameworks.
With the addition of Fetch API, there are three ways for Node.js to make HTTP requests:
- Using the Node built-in HTTP module for making request calls to web servers and APIs
- Utilizing a third-party library such as Axios or Request for more comprehensive control over your requests in a simpler syntax than native methods provide
- Using the Fetch API to make HTTP requests from Node.js code directly, without additional libraries or frameworks
The Node built-in HTTP module provides an interface for making requests to web servers and APIs. It is a very low-level API that requires you to write code to make HTTP requests, as opposed to using libraries or frameworks which provide simpler syntax. The HTTP module allows for control over the request methods (GET, POST etc.), headers, and body of the request sent as well as enables you to handle response codes from server responses.
This method provides the following advantages:
- Low-level API that gives you control over HTTP requests, making it more suitable for complex tasks and applications,
- Ability to handle response codes from server responses easily, and
- Ability to add custom headers and bodies to your requests.
Naturally, it comes with its own drawbacks:
- It requires coding to make the request as opposed to libraries or frameworks which provide simpler syntax.
- It can be time-consuming when writing code for multiple requests.
As this built-in method is pretty standard, its common use cases are pretty simple:
- Making simple HTTP requests, such as sending a GET request for static files,
- Creating and managing custom web servers in Node-based applications,
- Sending streaming data over the internet, such as audio or video streams, and
- Providing access to server-side events like client connections or disconnections.
Meanwhile, third-party libraries like Axios and Request provide a more comprehensive yet simpler way to make HTTP requests in Node.js. These libraries offer an easier syntax than native methods, allowing you to control the details of your request such as headers, query strings, and body data with fewer lines of code. Additionally, they can also help manage response codes and errors that may be encountered while making requests so that you can handle them gracefully without having to manually check for each one.
Through these libraries, users reap the following benefits:
- Easier syntax than native methods, allowing for more efficient request handling and manipulation,
- Ability to manage response codes and errors gracefully without having to manually check for each one,
- Ability to customize request/response behavior before and after making the actual request with interceptors, and
- Automatically transforming response data into JavaScript objects for easier readability and manipulation.
But, they may also encounter these disadvantages:
- Additional setup time, if not already installed in your project’s dependencies list.
- Potentially longer execution times due to additional overhead from third-party libraries.
These third-party libraries are popularly used for the following cases:
- Making API calls, such as retrieving data from an external source or submitting data to a backend server,
- Handling requests with complex parameters and headers, such as those used in authentication flows,
- Creating interceptors for customizing request/response behavior before and after making the actual request, and
- Automatically transforming response data into JavaScript objects for easier manipulation.
And then finally, there’s Fetch API.
Fetch API for Node.js
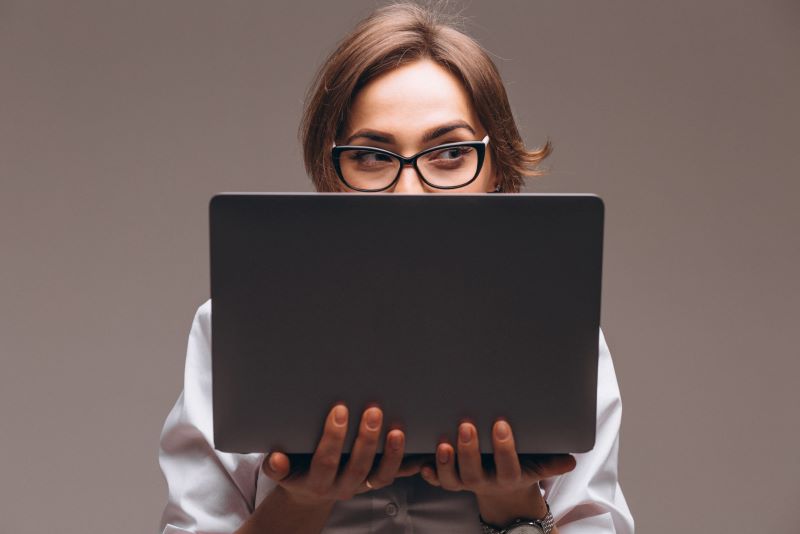
The Fetch API is a programming interface that enables developers to make HTTP requests, such as GET and POST. It takes advantage of the latest standards like Promise, which makes coding easier by eliminating callbacks. All major web browsers support the native Fetch API. However, those working with NodeJS on server-side code will need to install an NPM (Node Package Manager) node fetch package for their code to use this feature — extremely popular with millions of downloads each week. NPM is the default package manager for Node.js and the JavaScript programming language. Recently (version 17.5), Node JS added experimental support for using the Fetch API without requiring additional libraries.
Ultimately, Fetch API allows Node.js developers to make HTTP requests in a much simpler way as compared to earlier versions. It eliminates the need for additional libraries and packages, making it easier and faster to write code. Furthermore, since Fetch is supported by all major web browsers, this also simplifies development across various platforms.
Node Fetch Example
The Fetch API provides a JavaScript interface for accessing and manipulating parts of the HTTP pipeline, such as requests and responses. It also provides a global fetch() method that takes in an input URL and returns a Promise that resolves to the response. Here’s an example of how it works:
fetch(“https://example.com/endpoint”) // Make request
.then(response => response.json()) // Transform data into json format
.then((data) => {
console.log(data);
});
This code makes a request to the endpoint at “https://example.com/endpoint” using the Fetch API. Then, it transforms the response into a JSON format and logs it in the console for inspection or further manipulation.
Node JS HTTP.GET Example
Let’s compare a familiar HTTP request in a few formats and how they function. Below, we try a NodeJS HTTPS GET method via the built-in module, Axios, and Fetch.
First up, the most fundamental approach:
“`
const https = require(“https”);
const url = “https://example.com/”;
https.get(url, (res) => {
res.on(“data”, (d) => {
process.stdout.write(d);
});
}).on(“error”, (e) => {
console.error(e); }); “`
The code above uses NodeJS’ built-in HTTPS module to make a GET request to the specified URL. It then prints out any data it receives from that request and logs any errors along the way. This is a basic example of an HTTP request, but if you need more control over your requests, such as adding headers or customizing parameters, then using something like Axios or Fetch might be better suited for the job.
Now, let’s see the same thing in action via Axios:
“`
const axios = require(“axios”);
const url = “https://example.com/”;
axios.get(url) .then((res) => {
console.log(res); }) .catch((err) => {
console.error(err); }); “`
This code uses Axios to make a GET request to the specified URL, then prints out any data it receives from that request, and logs any errors along the way in an asynchronous manner using Promises instead of callbacks like with NodeJS’ built-in HTTPS module example above. The advantage here is that we can chain several requests together if necessary and have more control over our requests by adding custom headers or parameters before sending them off to their destination URL’s server endpoints easily with Axios’ built-in methods for doing so without needing complex logic or extra libraries when making HTTP calls from NodeJS applications.
Finally, in Fetch:
“`
fetch(“https://example.com/”)
.then((res) => res.json())
.then((data) => {
console.log(data);
})
.catch((err) => {
console.error(err);}); “`
This essentially does the same as the above, but in Fetch.
The advantage of using the Fetch API over NodeJS’ built-in module and Axios is that Fetch allows for a more streamlined way to make asynchronous requests. With Fetch, you get the same advantage as Axios as you can chain together multiple requests and specify request headers and parameters before sending the request off. BUT, Fetch is designed to handle responses more efficiently, as it automatically parses the response body into a usable format like JSON.
It also offers automatic error handling for failed requests and has better support for streaming out data from larger files compared to Axios or NodeJS’ built-in module. Additionally, Fetch allows developers to easily access all of the request details with just one call. With Axios or NodeJS’s HTTPS Module, you would need multiple calls in order to get all of this information.
Fetch is ultimately similar to Axios but with an improved syntax for modern JavaScript projects such as React apps. React often utilizes this approach due to its lightweight nature compared to other solutions available in the NPM library ecosystem today.
Node Fetch Headers Best Practices
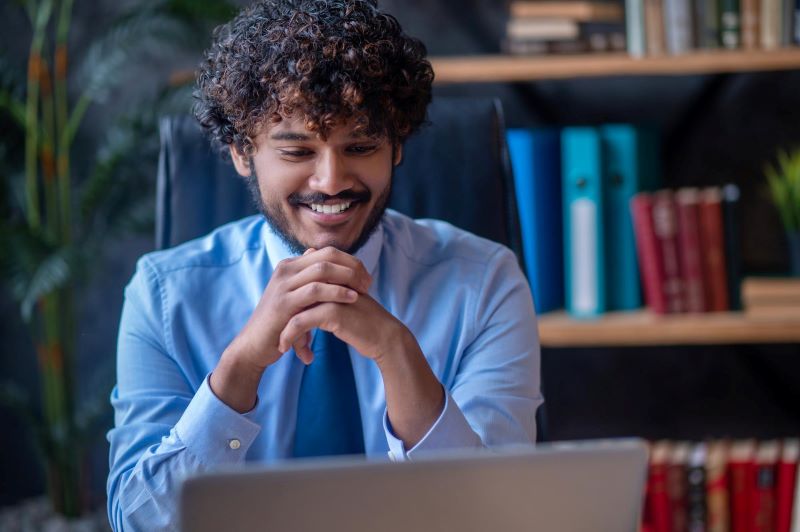
When making HTTP requests with Node Fetch, it’s important to understand how headers work. Headers are key-value pairs sent along in your request that provide additional information about the request itself. You can use headers to specify the content type of a response or make authentication easier. Additionally, you can also set custom header fields and add query parameters for extra specificity when making requests.
The considerations for using headers when making HTTP requests with Node Fetch are generally the same as when using other modules like the built-in NodeJS HTTP module or Axios. However, it’s important to note that some features may be unique to each module.
- Setting the correct content type for your response,
- Including any authentication information needed for successful requests,
- Utilizing query parameters and custom header fields to add extra specificity when making a request, and
- Ensuring all headers have the correct values depending on what kind of data they contain.
Commonly used headers include “Content-Type,” which specifies the type of response you’re expecting, and “Authorization” for authentication purposes. You can also set custom header fields or add query parameters for extra specificity when making requests.
For example, if your API requires a unique ID to make a successful request, you could specify this ID as part of your header or query parameter field. So, it will be included every time you make that particular request. It’s important to ensure all headers have the correct values depending on what kind of data they contain — sending incorrect types may result in errors or unexpected behavior from the server side.
Fetch API Benefits
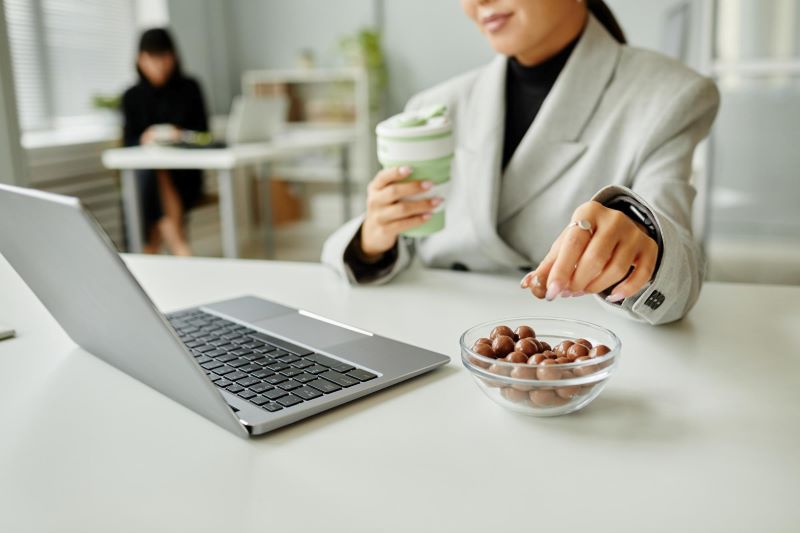
We’ve already briefly covered some of the ways Fetch API is a step up from the usual ways you can make HTTP requests in NodeJS. Let’s look a little deeper at the advantages conferred by this new development:
Fetch is Modern and Efficient
Fetch API is a modern and efficient way to make HTTP requests in Node.js because it uses promises instead of callbacks for asynchronous operations. This makes writing the code cleaner, more modular, and easier to read since the callback hierarchy can become confusing quickly when dealing with complex requests that require multiple layers of nested functions or if you need to chain several calls together in sequence before completing a task.
Promises are also more flexible than other methods such as XMLHttpRequest (XHR) because they provide an easy way for developers to handle errors without having to manually wrap each request into try-catch blocks. This simplifies debugging by making error messages easier to locate within your codebase.
Fetch Syntax is Less Cumbersome
The syntax of Fetch API is less cumbersome than traditional methods like XMLHttpRequest or even jQuery’s AJAX library because it uses promises to handle asynchronous operations instead of callbacks. This makes code easier to read and debug with fewer lines required overall, resulting in more concise and organized applications that are easier for developers to maintain.
Additionally, Fetch allows developers to easily incorporate headers into their requests without having to manually construct the values each time they’re used. This saves time during development cycles.
Fetch Allows Easy Integration of Headers
Fetch API simplifies the process of adding headers to HTTP requests by abstracting away the need for the manual creation of those values. Instead, developers can just pass an object containing their desired header values as a parameter when making their request, and Fetch will take care of constructing them automatically. This is more efficient than having to manually create and add each value one-by-one every time they are needed.
Additionally, it allows developers to easily add custom authorization tokens or other authentication methods that may be required for servers/APIs being interacted with over HTTP requests/response cycles. This makes development faster compared to other NodeJS options like Axios which require manual construction of headers before sending out requests. It ultimately saves precious time during development.
Fetch Supports Streaming Uploads
Fetch API supports streaming uploads. That is, large files can be uploaded to a server without blocking the main thread while they are processed. This allows for better performance and responsiveness in applications compared to other methods of making HTTP requests via NodeJS. Those other methods require all data transfers to take place on the main thread, potentially slowing down your application UI updates while they occur.
Fetch’s streaming uploads also allow developers more control over how long it takes for their requests/response cycles to complete. This means that you can prioritize certain tasks or limit request timeouts if needed.
Using a Node Fetch Proxy
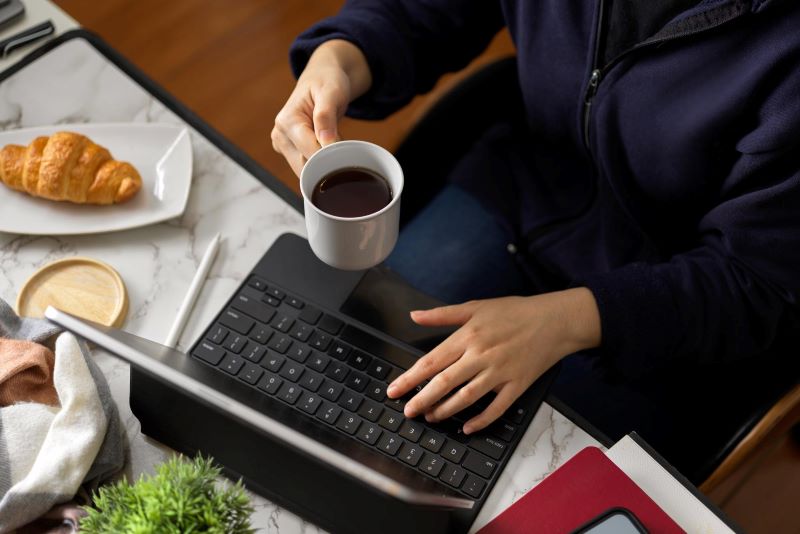
Fetch is a great option for making HTTP requests in Node.js. So, it will probably find a lot of use in web scraping. It’s handy in cases when the data you need is not available via an API but must instead be scraped from multiple sources on the web. By leveraging Fetch API’s headers customization feature, developers can make HTTP requests that appear to come directly from a browser and mimic user behavior more accurately than traditional methods like XMLHttpRequest or jQuery’s AJAX library. Furthermore, with modern browsers increasingly supporting more features of ES6 functionality (like Promises), Fetch API makes it easier to write efficient code that handles complex scraping tasks.
So, expect to need a proxy server if you’re planning to use Fetch for activities like web scraping. A proxy can help mask your IP address and add an extra layer of security when making requests over the internet, which is especially useful if you’re dealing with sensitive data or data from outside sources that may not be secure. In these cases, using a node fetch proxy will provide added peace of mind and make sure your data stays safe and secure no matter where it’s coming from.
Below are some of the use cases where a node fetch proxy would be useful:
- Data mining/scraping – These can be risky endeavors, particularly when you’re collecting data from outside sources that may not be secure. In these cases, using a node fetch proxy offers an extra layer of protection.
- Navigating unfamiliar networks – When running Node applications on unfamiliar networks, such as in the cloud, it’s important to maximize security. In these cases, a node fetch proxy can help secure your requests and responses between your application and the server hosting the data.
- Dealing with sensitive data – Proxy servers can protect sensitive data in the same way as mentioned above. A node fetch proxy can help mask your IP address and anonymize your requests so that no one can trace them back to you. Additionally, a node fetch proxy can also provide additional features like rate limiting, throttling, and caching that further improve the performance of Node applications while ensuring all requests are handled securely.
Throughout all these cases, the proxy adds an additional security layer by masking your IP address and encrypting the request/response traffic between your application and the server hosting the data. Additionally, it also facilitates the faster transfer of large amounts of data without having to worry about slowdowns due to connection issues or other performance-related problems associated with unsecured networks.
Finding Reliable Proxies
Using a proxy server such as those provided by Rayobyte can be advantageous when using Fetch API. The proxy serves as an intermediary between the client and server, allowing for faster routing which reduces latency — on top of the extra security and privacy it provides. Investing in this type of service ensures that requests are sent to the right destination smoothly and reliably, providing a more effective and efficient user experience.
Using low-quality proxies with Fetch API is not ideal since they won’t provide the same benefits or use technologies like split tunneling or caching. Additionally, if servers detect someone is using a public proxy their IP address may get blocked from accessing certain services or content.
At Rayobyte, we provide residential, ISP, and data center proxies for your project. Our ethical and professional team is dedicated to ensuring that you find the perfect proxy solution for your needs.
Getting residential proxies is usually your smartest move. This is because they give you IPs assigned to real people by their ISPs. Not only does this mean the IP addresses are accurate, but also that they rotate frequently, allowing web scrapers to do their job without raising any red flags. Additionally, we source our residential proxies responsibly and strive for minimal downtime.
Proxies in data centers can give you a speed boost. When you use them, your traffic is routed through the data center instead of residential IPs which makes connections faster. This comes with a cost, however. You won’t have as many unique and nonresidential IPs but they’re generally more budget-friendly.
ISP proxies offer a combination of speed and privacy. Located in data centers, these proxies benefit from the quickness of such locations while also providing users with the trustworthiness that comes from using an ISP.
Final Thoughts
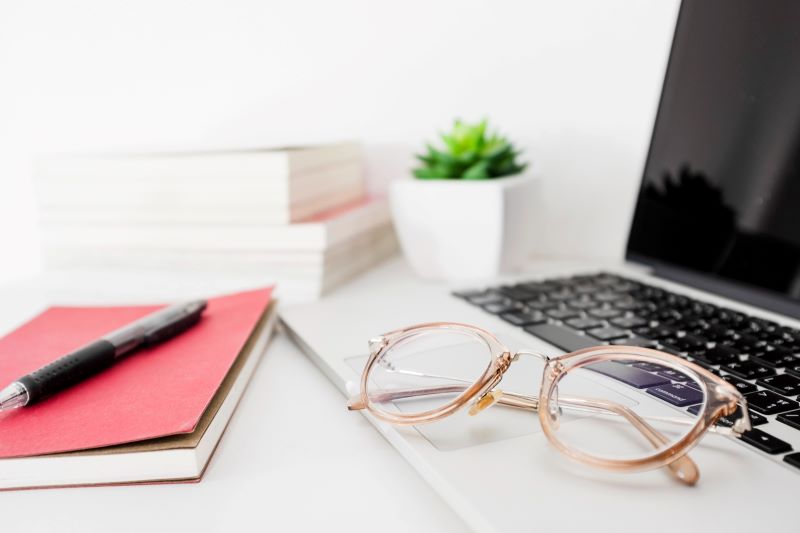
Rayobyte’s proxy servers are ideal for any of your projects requiring Fetch API use on NodeJS. Leverage our automated features to make set-up painless, and use our advanced Scraping Robot for any data mining work. Take a look at our solutions today.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.