The Ultimate Guide to Building a Price Tracker With Python
In today’s digital economy, researching the market and competitors is essential for businesses to remain competitive. With an ever-evolving landscape and new competition entering daily, it’s important to stay on top of pricing trends to make informed decisions. Towards this end, a price tracking solution can help businesses monitor pricing fluctuations across their own products as well as competitor offerings. This allows them to better forecast changes in demand, set prices that are competitive yet profitable, and identify areas where they have an advantage over their competitors. One of the best ways how to build a tracker is to automate the web scraping of prices via Python.
A successful price tracker website or software should provide accurate data from multiple sources. This should include e-commerce platforms like Amazon or eBay. Preferably, data is as close to real-time as possible. So, businesses can quickly adjust their strategies, if necessary. It should also be tailored specifically for each business’ particular industry needs, with the capability for optional customization. Finally, it should output data into an easily actionable format. So, if you’re used to crunching numbers on spreadsheets, for example, outputting scraped pricing information into a CSV might be the fastest way to not only gain insights from the data you scraped but also manipulate all of it so that you can implement some visualization and other analyses.
If it feels too complicated to build your own market and competitor price tracking tool, don’t worry. This guide will show you the basics and how to get started. We’re using Python; so, if you don’t have experience with web scraping, you can learn quickly and easily by following the tutorials on sites like Stack Overflow. We’ve even got our own Python web scraping guides. We’ll go through the basics like what you need to do and how so that you’ve got an overview to follow. We’ll also walk through deployment tips for your very own price tracker software or app.
Why a Shopping Price Tracker?
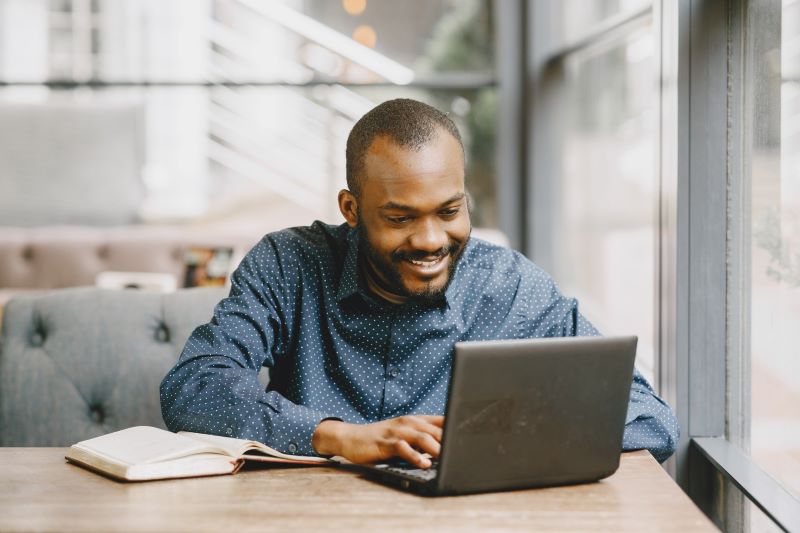
As mentioned earlier, a price tracking solution can provide businesses with accurate market and competitor data so that they can adjust their prices and strategies accordingly. Depending on how much customization a business needs, the right approach may be to DIY their own custom Python monitor software or app rather than rely on existing solutions in the marketplace. Doing this allows them to tailor it exactly to what they need and when they need it — no more waiting for access or updates from someone else. Plus, since web scraping is becoming increasingly popular for research purposes, Python is an optimal language choice as its syntax makes writing code straightforward even if you don’t have any prior coding experience.
Furthermore, depending on your stage of growth, it’s not always the best idea to buy a pre-made solution. There are factors to consider, such as your budget and how effective the tool will really be for your purposes. One of the best reasons to try and build one yourself is to start small. See for yourself whether the steps on how to build a tracker are feasible or not. Try to check if what you can build actually gets you the insight you need. Then scale from there. If you buy versus build, all the features you need are going to be bundled into one tool. You might end up paying for more than you need to — stretching your budget from the onset. It might also be the case that off-the-shelf solutions are too powerful for your needs at this point in time. You might invest in a push-button solution only to find that you’re overpaying for something that performs only a fraction of its functions because you really only need straightforward pricing information from a shopping price tracker.
One of the biggest advantages of having your own price tracker software is learning about product pricing faster than the competitors. This, in turn, allows you to stay ahead of trends before everyone else jumps aboard later. This insight helps inform corporate decisions such as:
- which products should be discounted during certain periods (i.e., the holiday season),
- where higher margins are achieved best (i.e., through subscription services versus one-time payments),
- gaining key insights into pricing models used by competitors, and
- uncovering any weak points in a competitor’s strategy that can then be exploited.
Furthermore, understanding changes in customer demand enables businesses to better forecast supply needs and plan inventory accordingly. This way, there’s never a shortage or overstock situation due to surprises outside your control (such as new regulations). Ultimately, this saves both time and money while keeping customers happy, too.
In summary, having your own price tracker is not only incredibly useful but potentially provides much-needed competitive advantages for businesses looking to stay ahead of the curve and maximize profits from sales opportunities. Ultimately, investing resources into creating an effective custom tool may very well pay off in spades once you start benefiting from all its data-driven insights.
How to Build a Tracker 101?
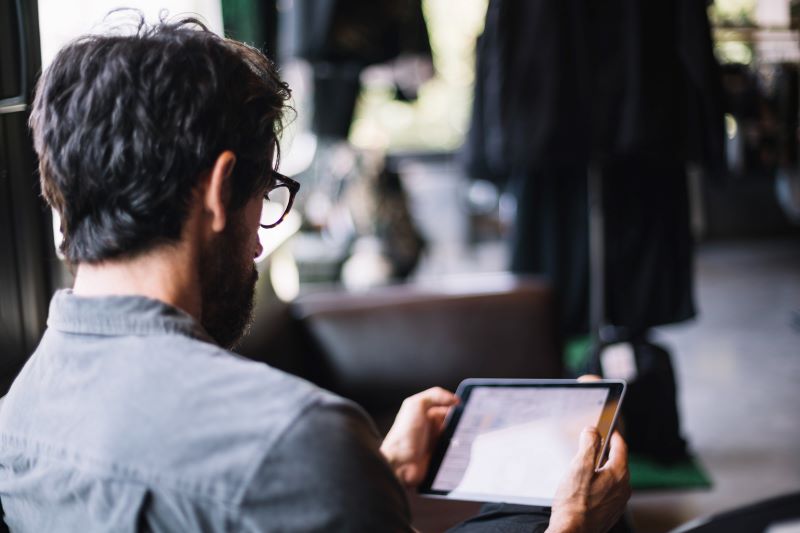
Web scraping offers an easy way to build a Python monitoring tool that tracks prices and meets all of your business’s needs as discussed above. With a few simple steps, you can automate the process so that it works continuously and reliably in collecting data from multiple e-commerce sources. You essentially need to build a target price history tracker that scrapes quality data in near real-time, outputting it into — for the purposes of our example — CSV format. To further simplify the example, we’re not going to be specific. That is, we won’t build an individual competitor price tracking tool for each of your rival businesses. We’ll target a well-known online store (e.g. Amazon) and take all the prices of a certain product category. We’re going to make Python check website for changes in price and alert us.
The fundamental use case is simple: a web scraper that checks the pricing relevant information of a product from Amazon. That’s good enough to start with. You can scale to add more details later. You should have a list of product URLs with prices listed. Our app is going to scrape each URL and see if the prices have changed. So, you’ll also be adding a feature into the tool that instructs a Python script to check the website for changes. More specifically, in our example, you want to check if prices go down. If they do, you fire off a notification email. That completes the simple Python price tracker.
What You’ll Need to Build Your own Python Price Tracker?
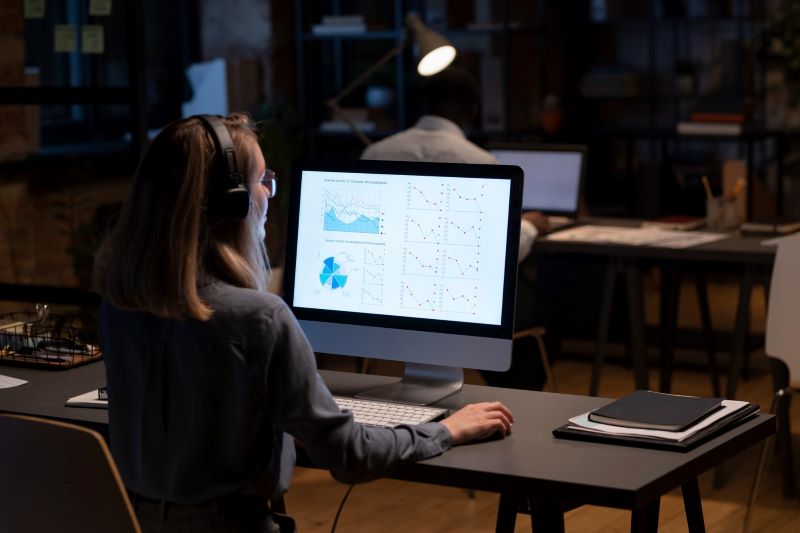
So, you’re now going to build your own Python monitor or tracker. You need to install the required Python libraries for your purposes.
Installing the Necessary Python Libraries
Below is an overview of what needs to be done:
- Install pip: Pip is a package manager for Python that simplifies library installation and helps keep packages up-to-date. To install this, open the command line in Windows (or terminal in Mac) and type “pip install –upgrade pip.”
- Install BeautifulSoup4: This library allows us to read webpages with ease when scraping Amazon product pages later on; it handles HTML parsing well, allowing us access past all those tags! In your command prompt/terminal window type “pip install beautifulsoup4.”
- Install Requests Library: This makes it easier for our program to send HTTP requests so that we can obtain information from websites like Amazon. Simply enter “pip install request” into the terminal/command line window and wait while Requests gets installed.
You’ll also need SMTP (Simple Mail Transfer Protocol) for sending out emails, and CSV to manipulate CSV files, but those are built into Python. So, you don’t need them installed. You can freely import them in the code.
This is a fundamental setup, and you can do much more with additional Python libraries. Some additional libraries that can help out with your endeavor include:
- Selenium: This allows us to automate interactions with webpages by simulating user actions such as clicking buttons and entering text into forms. This is one way to not have to manually scrape product pages for each update (saving time).
- PyQt5: Use this library if you want a GUI interface that displays live pricing data updates from Amazon — instead of just relying on exported files for the data.
For now, we’ll keep it simple.
Understanding the Scraping Process
What you need to be able to do is scrape the specified product URL. Say, for example, your product URL is https://www.amazon.com/dummy-product/dp/B01MXX4GL8, then the code would look like
import requests
from bs4 import BeautifulSoup
# Provide the URL of the dummy product page
url = ‘https://www.amazon.com/dummy-product/dp/B01MXX4GL8’
# Make a request to the page
page = requests.get(url)
# Parse the page into BeautifulSoup
soup = BeautifulSoup(page.content, ‘html.parser’)
# Find the price of the product and save it into the variable “price”
price = soup.find(‘span’, id=’priceblock_ourprice’).text
Notice the attribute “priceblock_ourprice” is the ID of the HTML element that contains the price of the product. This code tells BeautifulSoup to find that specific ID and output it as text (and save it as the value of the variable “price”). To find the price of the product, you need to find the correct HTML element, which contains the price information. This is going to be different from website to website. So, Amazon’s code is going to be different from another online marketplace’s, for instance. Luckily, it’s easy to find. For example, on Google Chrome, just right-click on the product price and hit inspect. This will show the ID or attribute you’re looking for in the code. By using the ID of the element, we can easily find it and return the price.
Fortunately, this ID or attribute should be uniform across an entire website. So, you can use the same ID to scrape the right data from Amazon, and the same ID for another marketplace, and so on.
Understanding the Email Alert Process
Now let’s incorporate the code above and add a feature: if the price value is lower than the original price indicated by the variable “target_value,” the program will send a price alert email.
import requests
from bs4 import BeautifulSoup
import smtplib
# Provide the URL of the dummy product page
url = ‘https://www.amazon.com/dummy-product/dp/B01MXX4GL8’
# Enter target value
target_value = 50
# Make a request to the page
page = requests.get(url)
# Parse the page into BeautifulSoup
soup = BeautifulSoup(page.content, ‘html.parser’)
# Find the price of the product and save it into the variable “price”
price = soup.find(‘span’, id=’priceblock_ourprice’).text
# Compare current price to target value
if int(price[1:]) <= target_value:
# Initialize the server
server = smtplib.SMTP(‘smtp.gmail.com’, 587)
server.ehlo()
server.starttls()
server.ehlo()
# Log into the email account
server.login(‘your_email_address’, ‘your_password’)
# Send the email
subject = ‘Price Alert!’
body = ‘The price of the product has dropped to ‘ + price + ‘. Check the Amazon page here: ‘ + url
msg = f”Subject: {subject}\n\n{body}”
server.sendmail(
‘sender_email_address’,
‘receiver_email_address’,
msg
)
# Terminate the server
server.quit()
Here, we’re using the SMTP library of Python to fire off an email if the “price” is lower than our indicated “target_value.” SMTP opens a server with the appropriate details with which to send the email. In this case, values like the server and port, your_email_address, and your_password need to be changed to the proper values for this code to work. Naturally, you can also customize the email subject and body.
Pulling from a CSV List of URLs
Finally, we incorporate that code again but instead of just setting a “target_value,” we pull it from a CSV file. In this example, we’ll use a single line from a CSV file, but you can easily loop through several lines to run the script on each line.
import requests
from bs4 import BeautifulSoup
import smtplib
import csv
# Provide the URL of the dummy product page
url = ‘https://www.amazon.com/dummy-product/dp/B01MXX4GL8’
# Read the target value from the dummy CSV file
with open(‘target_values.csv’) as csv_file:
csv_reader = csv.reader(csv_file, delimiter=’,’)
for row in csv_reader:
target_value = row[1]
# Make a request to the page
page = requests.get(url)
# Parse the page into BeautifulSoup
soup = BeautifulSoup(page.content, ‘html.parser’)
# Find the price of the product and save it into the variable “price”
price = soup.find(‘span’, id=’priceblock_ourprice’).text
# Compare current price to target value
if int(price[1:]) <= int(target_value):
# Initialize the server
server = smtplib.SMTP(‘smtp.gmail.com’, 587)
server.ehlo()
server.starttls()
server.ehlo()
# Log into the email account
server.login(‘your_email_address’, ‘your_password’)
# Send the email
subject = ‘Price Alert!’
body = ‘The price of the product has dropped to ‘ + price + ‘. Check the Amazon page here: ‘ + url
msg = f”Subject: {subject}\n\n{body}”
server.sendmail(
‘sender_email_address’,
‘receiver_email_address’,
msg
)
# Terminate the server
server.quit()
In this code, the target_value = 50 lines is replaced with a way to open a CSV file called “arget_values.csv” where row[1] is the “target_value” that we will compare with the “price.”
As mentioned earlier, you can visit online forums and even videos to get more detailed guidance on how to work with Python and its libraries. What is presented here is a simple breakdown of what needs to be done. So, you have an overview of how to do it. This should be sufficient to demonstrate how to build a tracker from a high-level perspective.
Using Proxies for Your Python Price Tracker
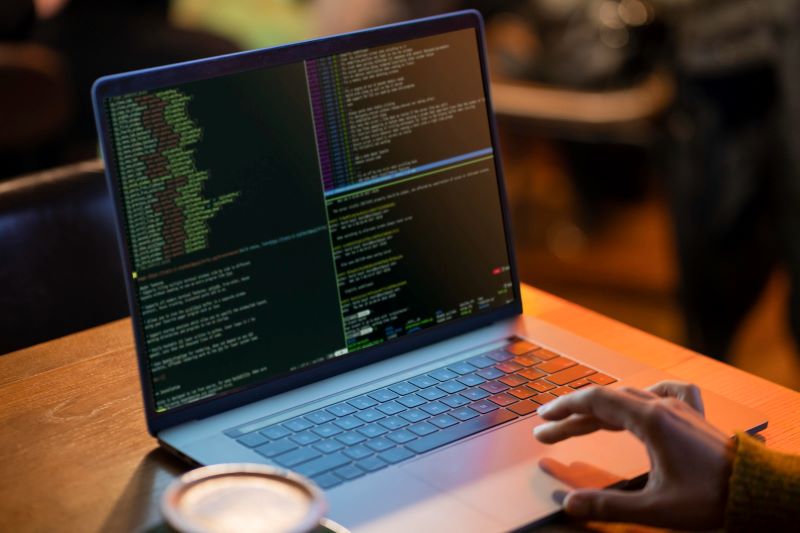
Now that you have a decent idea of how to build a tracker with Python that alerts you of price changes, you need to make sure your web scraper will always be functional. Otherwise, it won’t be reliable enough to track prices.
Web scrapers are essentially programs designed to automatically extract information from websites. They work by using a set of instructions (like the script you just saw) to identify and collect the data you need from a website (in your case, product prices). The script will go through a website’s pages, look for certain pieces of data, and then save that data in a structured format. The data collected can be used for various purposes, such as market research or competitive intelligence — in your case, a price change alert. As you can imagine, web scrapers are an efficient way to gather data from multiple websites quickly and with minimal effort.
The problem is that web scrapers are prevented from doing their job for two main reasons.
Firstly, web scraping can put a strain on a server’s resources by sending large amounts of traffic to it and extracting data too quickly. This affects the performance of other websites hosted on the same server, or even existing visitors to the website in question.
Additionally, automated requests made by web scrapers can be misused to launch distributed denial-of-service (DDoS) attacks. This is when malicious actors try to take down a website or network by overwhelming it with traffic. Since these attempts rely heavily on the automation of thousands of requests hitting the target at once, web scraping scripts can easily be repurposed for such purposes if not properly secured and monitored. That’s why servers also prevent this type of automated request from taking place to avoid any disruption or damage caused as a result.
Secondly, web scraping allows users to access data that they would otherwise not have access to without paying for it or requesting permission (such as product prices). Web servers, therefore, protect their content and prevent people from stealing sensitive information without consent by blocking automated requests coming from web scrapers/bots. They do this mainly through rate limits (limiting how many requests can be made within a certain time period) or identifying commonly-used user agents and IP addresses associated with automated requests.
Using Reliable Proxy Servers
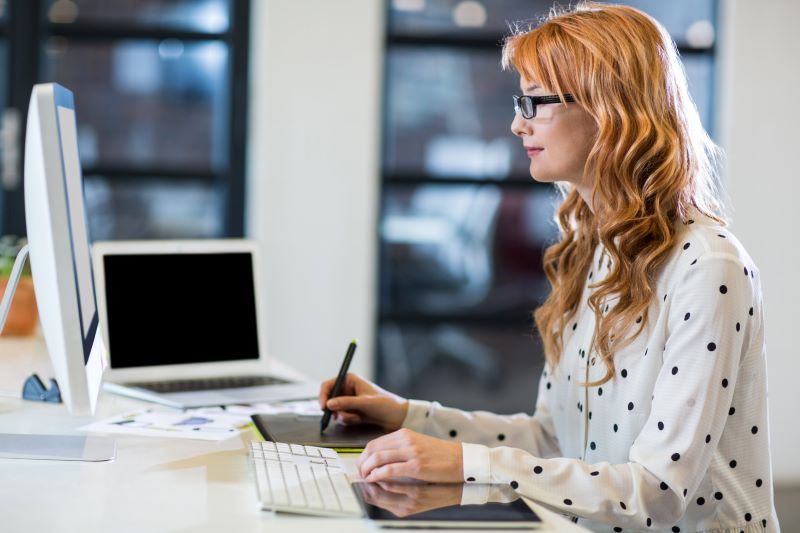
Fortunately, there is a way to get around these restrictions while still extracting the data you need: by using proxy servers. A proxy server acts as an intermediary between your computer and the website you are trying to access, masking your IP address and hiding both sender and receiver information so that it appears as if a different user (in this case, a web scraper) is making the request.
This allows web scrapers to bypass rate limits present on websites or evade detection of malicious automated requests without ever having their activities actively monitored by website administrators. Beyond improved privacy, proxies have an extra perk. They speed up response times and reduce everyone’s data usage by caching sites locally on the server itself when multiple people are accessing them through it.
At Rayobyte, we provide dependable and compliant proxy servers to make sure your web scraping is a success. Our selection includes residential, ISP, and data center proxies — making it easy to find an option that’s just right for you. Plus, as a reputable company with great customer service capabilities, you can count on us for stellar support.
Typically, the optimal option would be to go with residential proxies. Their IP addresses are given out by ISPs to individual computers. Therefore, they remain up-to-date and will likely not raise any suspicions when connecting to online servers. At Rayobyte, we guarantee that our residential proxies have unmatched quality and work towards providing minimal downtime issues.
Data center proxies can be an excellent pick if you need more rapid speeds. As they let traffic move through data centers, this normally means speedier links. Regrettably, a smaller amount of noncommercial and one-of-a-kind IP addresses will likely be accessible, but in exchange, these do cost less. These proxies could make web scraping projects easier especially when dealing with immense volumes of information — which may apply to your case if you’re scraping a lot of pricing information.
A combination of speed and privacy can be attained by way of ISP proxies. These are associated with an internet service provider, but the actual location is in a data center. Consequently, you can benefit from what your ISP offers along with greatly expedited connections. They’re not as secure as residential proxies, however.
Final Thoughts
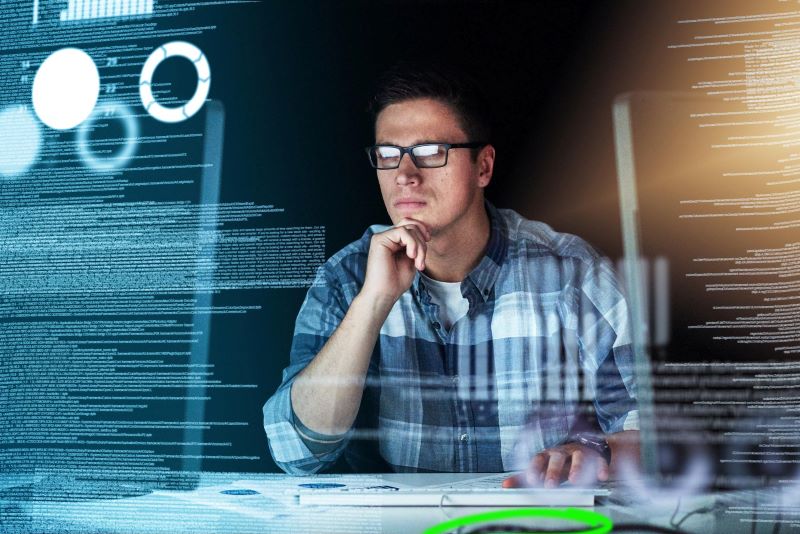
So, now you know the basics of how to build a tracker. Ultimately, with the right expertise and tools, you can develop your own shopping price tracker that is tailored to fit the particular needs of your business. By taking advantage of web scraping and keeping an eye on competitor prices in near-real time through automated emails (as achieved here), businesses can gain key insights into pricing models used by their competitors while acquiring competitive advantages due to faster detection of market changes than would otherwise be possible.
Remember, it’s vital to make use of a reliable proxy server such as those offered by Rayobyte for web scraping. With our advanced options, you can automate even more of the process. To get started on your venture well-equipped, we suggest trying out our Scraping Robot. The right tools are essential — we’re here to help!
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.