Understanding cUrl GET Requests In PHP: A Comprehensive Guide
Whether you’re a seasoned developer or just starting your journey in web development, understanding the power of cUrl is crucial. cUrl, short for “Client for URLs,” is a versatile command-line tool and library for making HTTP requests. In PHP, cUrl is an invaluable tool for interacting with APIs, fetching data from remote servers, and more. It serves as a communication bridge between your PHP code and other servers to retrieve data and send requests. cUrl GET requests in PHP are an essential part of this communication process — they allow you to retrieve information from web servers effortlessly.
So, if you’re aiming to fetch data from external APIs, streamline server-to-server communication, or scrape data, this article will equip you with the knowledge and skills needed to harness the full potential of the cUrl GET request in PHP.
cUrl Functions in PHP
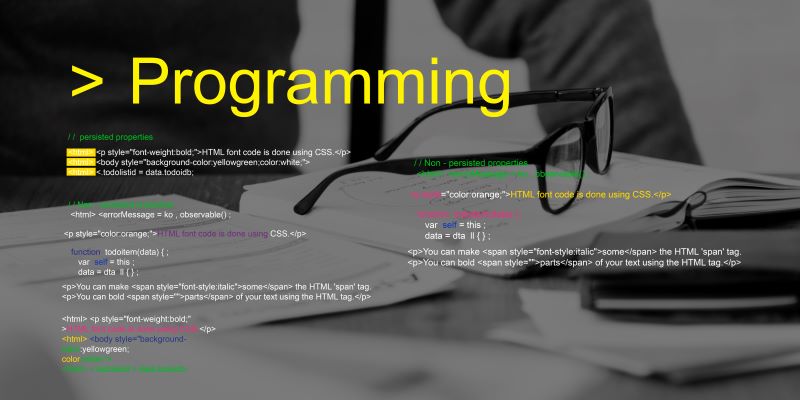
Before getting into the specifics of the cUrl GET request in PHP, it’s essential that you first familiarize yourself with some fundamental cUrl functions in PHP.
- curl_init(): This function initializes a cUrl session and returns a cUrl handle, which is used in subsequent cUrl functions. It’s the first step before making any HTTP requests.
- curl_setopt(): Once you have a cUrl handle, you can set various options using curl_setopt(). This function allows you to customize your cUrl GET request in PHP, specifying details such as the URL, request method, headers, and more.
- curl_exec(): This function executes the cUrl session, sending the request and returning the response. You can capture the response in a variable for further processing.
- curl_close(): After executing the request and handling the response, it’s essential to close the cUrl session using curl_close() to free up resources.
- curl_setopt_array(): This function allows you to set multiple cUrl options simultaneously by passing an associative array. It’s a convenient way to configure various aspects of your cUrl GET request in PHP with a single call.
- PHP curl_getinfo(): To gather information about the cUrl transfer, such as response code, total time, and more, you can usePHP curl_getinfo(). Examples of PHP curl_getinfo() options include CURLINFO_HTTP_CODE, CURLINFO_REDIRECT_COUNT, and CURLINFO_SPEED_DOWNLOAD.
- curl_errno() and curl_error(): When dealing with errors during the cUrl request, these PHP cUrl get error functions become handy. curl_errno() returns the error number, and curl_error() provides a human-readable error message.
cUrl GET Request in PHP
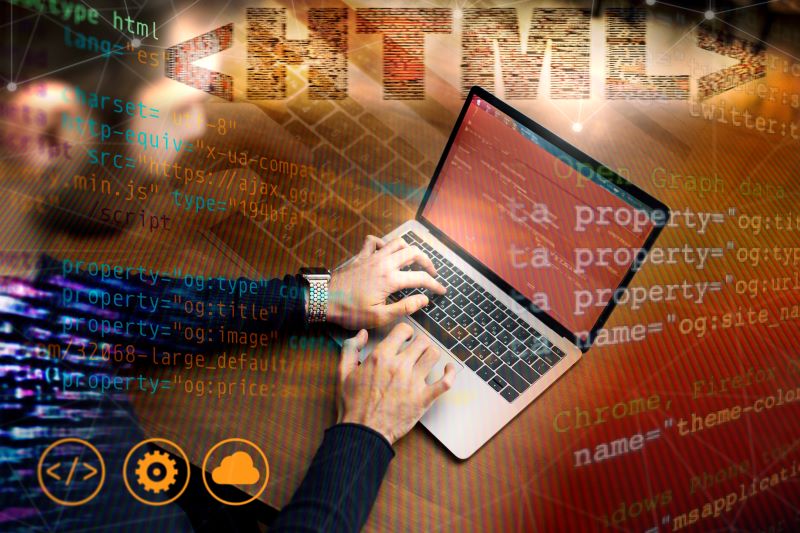
As mentioned earlier, the cUrl GET request is a fundamental method used to retrieve data from a specified resource in web development. It’s employed when you want to request data from a server, typically appending parameters to the URL. In PHP, a cUrl GET request involves constructing a URL with any necessary query parameters. These parameters, appended to the URL, serve as instructions to the server on the specific data you want to retrieve.
Here’s a breakdown of its essential components:
- HTTP method: GET is one of the standard HTTP methods, defined as a safe and idempotent operation. It indicates that the request’s purpose is to retrieve data without altering the server’s state.
- URL (uniform resource locator): The URL specifies the location of the resource you want to access (the endpoint). It includes the protocol (such as “http” or “https”), the domain, and the path to the specific resource.
- Query parameters: GET requests can include parameters appended to the URL, typically following a question mark. These parameters are key-value pairs separated by ampersands and allow you to send additional information to the server.
Here’s an example of a basic GET request URL:
https://api.example.com/data?param1=value1¶m2=value2
In this URL:
“https://api.example.com/data” is the endpoint.
“param1=value1” and “param2=value2” are query parameters.
How cUrl GET requests work
When you initiate a GET request, the client — your PHP application, in this case — communicates with the server to fetch the specified resource. The parameters, if any, are sent as part of the URL. Unlike PHP cUrl POST requests (cUrl -d PHP, which allows you to include data in the request body), a GET request appends parameters to the URL, making them visible in the address bar and easier to debug.
Here’s a step-by-step overview of how a GET request works:
- Client initiates request: Your PHP code uses cUrl to initialize a GET request by specifying the URL and any necessary options.
- cUrl sends request: The cUrl library translates your request into an HTTP GET request and sends it to the specified server.
- Server processes request: The server receives the GET request, interprets the URL and parameters, and fetches the requested resource.
- Server sends response: The server sends back the requested data as part of the HTTP response.
- cUrl receives response: cUrl captures the response, allowing you to further process or use the retrieved data in your PHP application.
Crafting a basic cUrl GET request in PHP
Let’s translate this understanding into a basic cUrl GET request using PHP:
1. Initializing a cUrl session
You achieve this using the curl_init() function. This function returns a cUrl handle, which is essential for subsequent cUrl operations.
Initialize cUrl session:
$ch = curl_init();
The cUrl handle ($ch in this example) is a reference for the ongoing cUrl session, allowing you to configure various options and execute requests.
2. Setting cUrl options
After initializing the cUrl session, you need to set various options to tailor your GET request. You can do this using the curl_setopt() function. Options include the target URL, request type, headers, and more. You’ll typically set the URL using cUrlOPT_URL for a basic GET request.
Set the URL with query parameters:
curl_setopt($ch, cUrlOPT_URL, ‘https://api.example.com/data?param1=value1¶m2=value2’);
Additional options can be set based on your specific requirements. For example, cUrlOPT_RETURNTRANSFER is often used to instruct cUrl to return the response as a string instead of outputting it directly.
3. Using the cUrl exec function
With the cUrl session initialized and options set, the next step is executing the GET request using the curl_exec() function. This function sends the request to the server and returns the response.
Execute the request
$response = curl_exec($ch);
4. Handling response data
After executing the request, handling the response data appropriately is crucial. The response could be in various formats, such as JSON or XML. You can then process, parse, and use this data within your PHP application.
Check for errors using PHP cUrl GET error functions
if (curl_errno($ch)) {echo ‘cUrl Error: ‘ . curl_error($ch);}
Close the cUrl session
curl_close($ch);
Process and display the response
echo $response;
You can use the PHP curl_error() and curl_errno() functions to check for errors while executing the cUrl GET request. If any errors occur, you can capture and handle them accordingly. Finally, you can close the cUrl session using curl_close() to free up resources.
Handling troubleshooting issues and errors
Working with a cUrl GET request in PHP can sometimes lead to challenges, ranging from connectivity issues to misconfigurations. Knowing how to troubleshoot and handle errors is crucial for maintaining the reliability of your PHP applications. Troubleshooting tips you can use include:
- Check connectivity: Ensure that your server has internet connectivity. Network issues, firewalls, and proxy configurations can hinder cUrl’s communication with external servers.
- Verify URL and parameters: Double-check the URL and any parameters in your cUrl GET request in PHP. Typos or incorrect configurations can result in unexpected errors. Ensure that special characters in parameters are properly encoded.
- Review server logs: Examine server logs for any error messages related to the cUrl request. You can log server-side issues, such as misconfigurations and resource limitations, which can provide valuable insights.
- Inspect SSL/TLS configuration: If your cUrl request involves HTTPS, ensure that your server has the necessary SSL/TLS certificates configured. cUrl may encounter issues if it cannot verify the server’s certificate. Use cUrlOPT_SSL_VERIFYPEER and cUrlOPT_SSL_VERIFYHOST options cautiously, considering the security implications.
- Test with the cUrl command line: Validate your cUrl request by reproducing it using the command line. This can help identify whether the issue is specific to your PHP environment or if it persists at the system level.
Handling errors
Handling errors is crucial for providing a good user experience and debugging your application effectively. In PHP, you can use curl_errno() and curl_error() to check for errors during the cUrl request.
- Logging errors: Consider logging errors to a file or centralized system. This helps in tracking issues and diagnosing problems in a production environment.
- User-friendly messages: Provide clear and concise messages when presenting errors to users. Avoid exposing sensitive information that malicious users could exploit.
- Retrying requests: Implementing a retry mechanism can be beneficial for transient errors. This can be particularly useful for scenarios in which the issue is temporary, such as network glitches.
- Exception handling: Incorporate exception handling in your code. Depending on your application’s architecture, you may throw exceptions when cUrl errors occur, allowing for centralized error handling.
Here’s an illustration of these techniques:
Check for errors using PHP cUrl GET error functions
if (curl_errno($ch)) {
$error_message = ‘cUrl Error: ‘ . curl_error($ch);
Log the error
error_log($error_message, 0);
Throw an exception or handle the error in a way that suits your application:
echo $error_message; } else {
Process and display the response
echo $response;}
PHP cUrl POST vs. PHP cUrl GET request in PHP
The main difference between PHP cUrl POST and GET requests lies in how they send data to the server and the type of request they make. In a POST request, you send data in the request body. This is often used for submitting form data or sending complex data structures. PHP cUrl POST uses cUrl -d PHP options, specifically CURLOPT_POST and CURLOPT_POSTFIELDS, to indicate that it’s a POST request and to specify the data you want to send.
URL to send the POST request to:
$url = ‘https://example.com/api/resource’;
Data to send in the POST request
$postData = array(
‘key1’ => ‘value1’,
‘key2’ => ‘value2’
);
Initialize cUrl session
$ch = curl_init($url);
Set cUrl -d PHP options for POST request
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true); Indicates a POST request
curl_setopt($ch, CURLOPT_POSTFIELDS, $postData); Specifies the data to send
Execute the cUrl session and get the response
$response = curl_exec($ch);
Close cUrl session
curl_close($ch);
Process the response as needed
echo $response;
On the other hand, for a cUrl GET request in PHP, data is typically sent as part of the URL query parameters. This is suitable for sending smaller amounts of data, and the data is visible in the URL.
URL to send the GET request to with query parameters:
$url = ‘https://example.com/api/resource?key1=value1&key2=value2’;
Initialize cUrl session
$ch = curl_init($url);
Set cUrl options (defaults to GET request)
Execute the cUrl session and get the response
$response = curl_exec($ch);
Close cUrl session
curl_close($ch);
Process the response as needed
echo $response;
In the GET example, the data (key1=value1&key2=value2) is directly appended to the URL. Note that for a GET request, the CURLOPT_HTTPGET option is not explicitly set because it is the default behavior of cUrl.
cUrl Options and Parameters for GET Requests
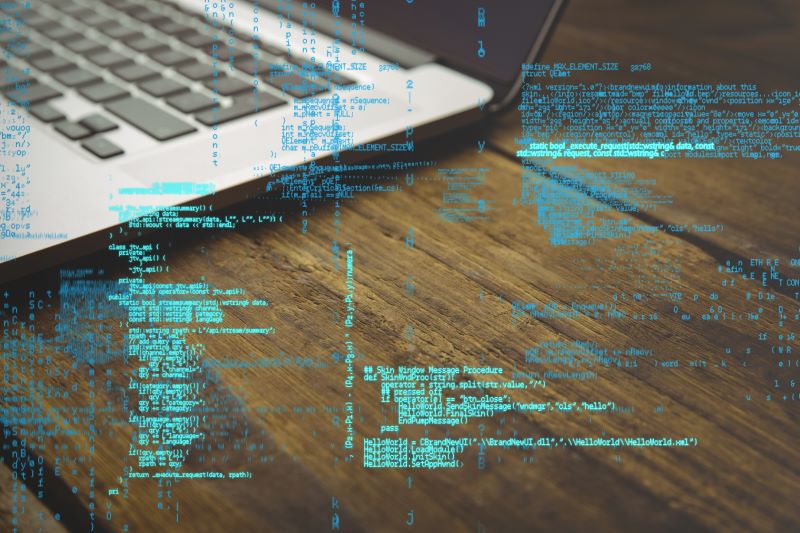
When working with GET requests, you can use cUrl options and parameters to customize the behavior of a cUrl request in PHP when interacting with web servers. These options provide you with a flexible way to control various aspects of the HTTP request and response process.
Here are commonly used options for cUrl GET request in PHP:
- CURLOPT_URL: It specifies the URL to which you send the cUrl request.
- CURLOPT_RETURNTRANSFER: Returns the response as a string instead of outputting it directly.
- CURLOPT_FOLLOWLOCATION: Follows any HTTP 3xx redirects.
- CURLOPT_HTTPHEADER: Sets an array of HTTP headers to include in the request.
- CURLOPT_SSL_VERIFYHOST and CURLOPT_SSL_VERIFYPEER: Enables or disables SSL certificate verification.
- CURLOPT_TIMEOUT: Sets the maximum time, in seconds, that the request should take.
- CURLOPT_POSTFIELDS: For a GET request with parameters, you can pass them using this PHP curl form.
Here are some basic examples of a cUrl GET request in PHP using some of these options:
Appending parameters to the URL
GET requests often involve querying a server for specific data. Parameters appended to the URL allow for a clear and straightforward way to express the nature of the request. This is ideal for static queries.
Example:
$baseURL = ‘https://api.example.com/data’;
$queryParams = [‘param1’ => ‘value1’, ‘param2’ => ‘value2’];
Construct the URL with the parameters:
$urlWithParams = $baseURL . ‘?’ . http_build_query($queryParams);
Initialize cUrl session:
$ch = curl_init($urlWithParams);
Set cUrl options:
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
Execute cUrl session:
$response = curl_exec($ch);
Close cUrl session:
curl_close($ch);
Process the response:
echo $response;
Using cUrl options for parameters
In dynamic scenarios, you may need to construct the URL based on runtime conditions or user inputs. Using cUrl options to set parameters within the cUrl session allows for programmatic and flexible URL construction.
Example:
$baseURL = ‘https://api.example.com/data’;
Initialize cUrl session:
$ch = curl_init();
Set cUrl options:
curl_setopt($ch, CURLOPT_URL, $baseURL);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
Additional parameters set using cUrl options:
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query([‘param1’ => ‘value1’, ‘param2’ => ‘value2’]));
Execute cUrl session:
$response = curl_exec($ch);
Close cUrl session:
curl_close($ch);
Process the response:
echo $response;
Customizing headers in a GET request
HTTP headers play a critical role in conveying additional information about the request and response. While headers are commonly associated with POST requests, customizing headers in a GET request is necessary when interacting with APIs that require you to include specific information.
Custom headers provide a means to include additional information in the request, such as authentication tokens, content types, or other specifics required by the API. This customization enhances the adaptability of the GET request.
Example:
$apiURL = ‘https://api.example.com/data’;
Initialize cUrl session:
$ch = curl_init($apiURL);
Set cUrl options:
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
Set custom headers:
$headers = [ ‘Authorization: Bearer your_access_token’,
‘Accept: application/json’,];
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
Execute cUrl session:
$response = curl_exec($ch);
Close cUrl session:
curl_close($ch);
Process the response:
echo $response;
Handling cUrl Output in PHP
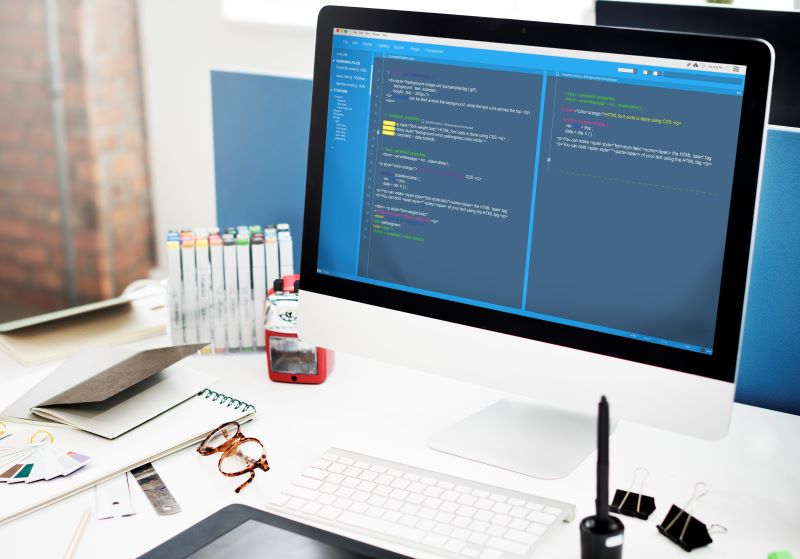
Once you have successfully executed a cUrl GET request in PHP, the next step is to handle the output or response that the server provides. Handling cUrl output effectively allows you to process the information, make decisions based on the response, and integrate the data into your application seamlessly.
Extracting information from the response
After executing a cUrl request, you can process and use the response data. This might involve parsing JSON, XML, or other data formats, depending on the nature of the response. Here’s how to go about it:
Check for a successful response (assuming a JSON response)
$http_code = curl_getinfo($ch, CURLINFO_HTTP_CODE);
if ($http_code === 200)
Parse JSON response or perform other processing
{$decoded_response = json_decode($response, true);
Use the processed data in your application
print_r($decoded_response);
} else {
Handle non-200 HTTP responses
echo ‘Unexpected HTTP status code: ‘ . $http_code;
}
Saving cUrl output to file
Sometimes, you might want to save the cUrl output to file for further analysis or archival purposes. Here’s an example of how you can redirect the output to a file:
$url = ‘https://api.example.com/data’;
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$output = curl_exec($ch);
if (curl_errno($ch)) {
echo ‘Error: ‘ . curl_error($ch);
} else {
Save output to a file
file_put_contents(‘output.txt’, $output);
echo ‘Output saved to file.’;
}
This code saves the raw cUrl output to file named ‘output.txt.’ You can adjust the filename and path according to your requirements.
Specifying file formats
If you’re dealing with different types of content, you may want to save the output with appropriate file extensions. For example, if the response is an image, you can save it as an image file:
$url = ‘https://example.com/image.jpg’;
$ch = curl_init($url);
Specify a filename with the appropriate extension
$filename = ‘downloaded_image.jpg’;
$fp = fopen($filename, ‘w’);
curl_setopt($ch, CURLOPT_FILE, $fp);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true);
curl_exec($ch);
if (curl_errno($ch)) {
echo ‘Error: ‘ . curl_error($ch);
} else {
echo “Output saved to file: $filename”;
}
PHP cUrl Timeout and Request Management
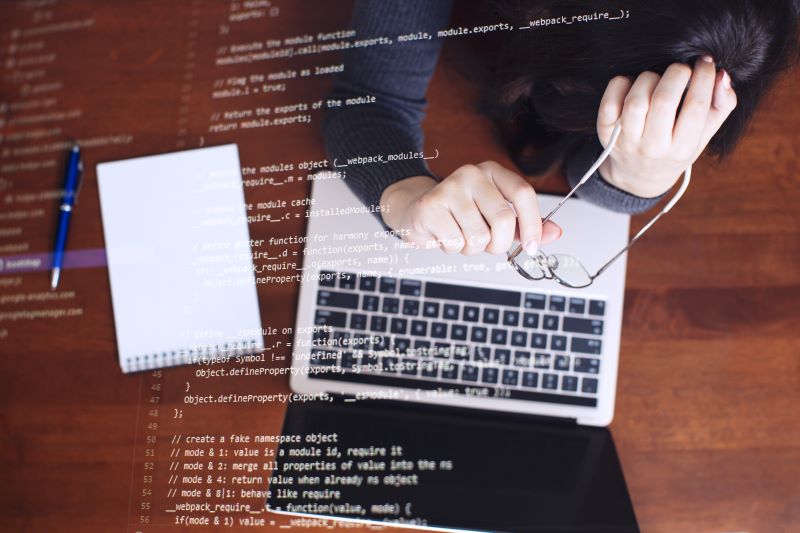
PHP cUrl timeout is crucial to managing HTTP requests effectively. It refers to the maximum time you’ll allow a cUrl request to take before the application considers it as timed out. This is particularly important in scenarios in which a request might take longer than expected, and you want to prevent it from impacting the performance of your application.
Setting a PHP cUrl timeout for a request
There are several ways you can set timeouts:
Using the cUrl -m flag
You can use the cUrl -m flag (or –max-time) directly in the command line to set the timeout for a cUrl request. This can be useful when making requests via the command line:
curl -m 10 https://api.example.com/data
In this example, the maximum time that the cUrl request should take is set to 10 seconds. If the request takes longer than 10 seconds to complete, the cUrl -m flag will terminate the request and display an error or timeout message.
Implementing custom timeout in PHP
You can set the timeout using the CURLOPT_TIMEOUT option when configuring cUrl like this for 10 seconds:
curl_setopt($ch, CURLOPT_TIMEOUT, 10);
Managing asynchronous requests
An asynchronous cUrl GET request in PHP enables you to initiate multiple requests concurrently without waiting for each to complete before starting the next one. PHP provides libraries like curl_multi for managing asynchronous requests. Here’s a simplified example:
$urls = [‘https://api.example.com/data1’, ‘https://api.example.com/data2’, ‘https://api.example.com/data3’];
$multiHandle = curl_multi_init();
$handles = [];
foreach ($urls as $url) {
$handle = curl_init($url);
curl_setopt($handle, CURLOPT_RETURNTRANSFER, true);
Additional cUrl configurations
// …
curl_multi_add_handle($multiHandle, $handle);
$handles[] = $handle;
}
Execute multiple requests simultaneously
do {
curl_multi_exec($multiHandle, $running);
} while ($running > 0);
Process responses
foreach ($handles as $handle) {
$response = curl_multi_getcontent($handle);
Process each response as needed
echo $response;
Remove the handle from the multiHandle
curl_multi_remove_handle($multiHandle, $handle);
curl_close($handle);
}
curl_multi_close($multiHandle);
Managing parallel requests
You can achieve a parallel cUrl GET request in PHP by using libraries like RollingCurl or Requests in PHP. These libraries allow you to send multiple requests in parallel, optimizing the efficiency of your application.
Here’s a simplified example using the Requests library:
require ‘vendor/autoload.php’; // Include the Requests library
$urls = [‘https://api.example.com/data1’, ‘https://api.example.com/data2’];
$options = [
‘timeout’ => 10, // Set a timeout for each request
];
$responses = Requests::request_multiple($urls, $options);
foreach ($responses as $response) {
// Process each $response as needed
}
Conclusion
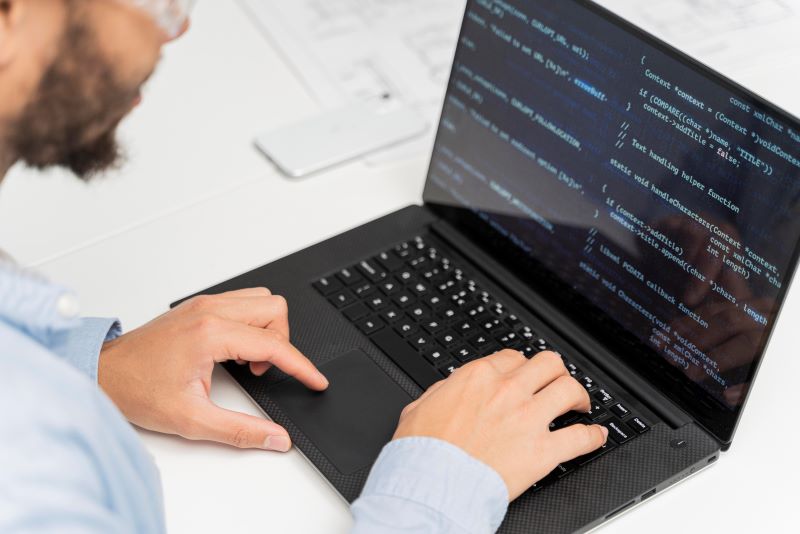
One of the uses of cUrl GET requests in PHP is web scraping. However, you must use reliable proxies to enhance your web scraping efforts. As a dedicated partner for proxy users, Rayobyte offers a range of high-quality proxy solutions, including data center and residential proxies, to ensure that your cUrl GET requests in PHP are seamlessly integrated, enabling not only effective web scraping but also empowering you to leverage proxies for various applications such as social media management, streaming, cybersecurity, geolocation concerns, and privacy enhancement.
Sign up for a free trial today.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.