The Ultimate Guide to Use cUrl to Execute a Post Request
Maybe you’ve heard of this command line tool but have always wondered: What is a cURLing? Born out of a project in 1996 by Daniel Stenberg, cURL (Client for URLs) is a versatile command-line tool for transferring data across a wide array of protocols. It allows developers to test, debug, and interact with APIs. Whether you’re pulling down a webpage, sending an email, or interacting with a REST API, a cURL POST request can help you get the job done.
Despite its complex exterior, at its core, cURL works by sending HTTP requests to servers and receiving responses. These interactions, coded in the common language of the web — HTTP — are an essential part of cURL’s functionality. The tool supports many HTTP methods, including GET, POST, PUT, and DELETE, among others.
In web development, a cURL POST request is particularly important. Unlike a GET request, which retrieves data, a cURL POST request sends data to a server, a fundamental mechanism that powers interactivity on the web. From submitting form data and uploading files to creating new resources, cURL POST requests are at the heart of client-server communication.
CURL POST requests open the gateway to complex applications, allowing for user personalization, dynamic content, and secure data transmission. These capabilities form the bedrock of the modern web, enabling applications from simple blogs to complex single-page applications and intricate web services.
Using a cURL POST request in APIs is an integral part of web development, allowing services to communicate with each other, exchange data, and create powerful, interconnected systems. In an age where APIs form the backbone of many services, understanding POST requests is a necessary skill for developers.
Using cURL POST requests adds a versatile tool to a developer’s toolkit, powering the seamless interactions and data exchanges that define the modern web experience. By understanding these technologies, you can not only create rich, interactive web applications but also harness the true power of APIs, opening up a world of possibilities for data exchange and service integration. This guide will tell you everything you need to know about how to make a cURL POST request.
Getting Started with cURL Post Requests
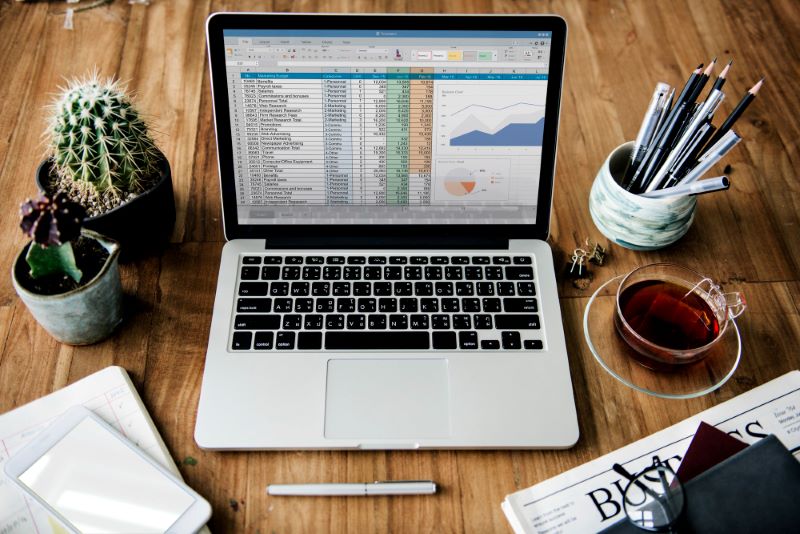
Before you can execute a cURL POST request, you need to install cURL. The process of installing cURL differs slightly depending on the operating system you’re using. For most Linux and Unix-based systems, cURL is often pre-installed. To confirm its presence, open a terminal window and type curl –version. If cURL is installed, this command will display the version number and other relevant information.
For macOS users, cURL is also usually pre-installed. However, if you need to install it, you can use the Homebrew package manager. If you don’t have Homebrew installed, you can install it by running the following command in your terminal:
/bin/bash -c “$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)”
Once you have Homebrew, you can install cURL by running brew install curl.
For Windows users, cURL comes pre-installed with Windows 10 version 1803 and later. For earlier versions, you can download cURL from the official website and follow the instructions provided. Another alternative is to install it via a package manager like Chocolatey, with the command choco install curl.
Basic Syntax and Usage of cURL Commands
The fundamental syntax for cURL is curl [options] [URL]. The URL is the address you want to send a cURL POST request to, and the options are flags that control the behavior of the request. For instance, to send a GET request to a website, you would simply type curl https://example.com.
By default, cURL uses the GET HTTP method, which retrieves information from the server. To use other methods like POST, PUT, or DELETE, you’ll need to specify them with an option flag. For instance, to send a cURL POST request, you would use the -X or –request flag followed by POST, like so:
curl -X POST https://example.com.
Another common option is -d or –data, used to include data in a cURL POST request. This could look like:
curl -d “username=user&password=pass” -X POST https://example.com/login
This example sends a cURL POST request with data (username and password) to the URL.
Understanding HTTP Requests
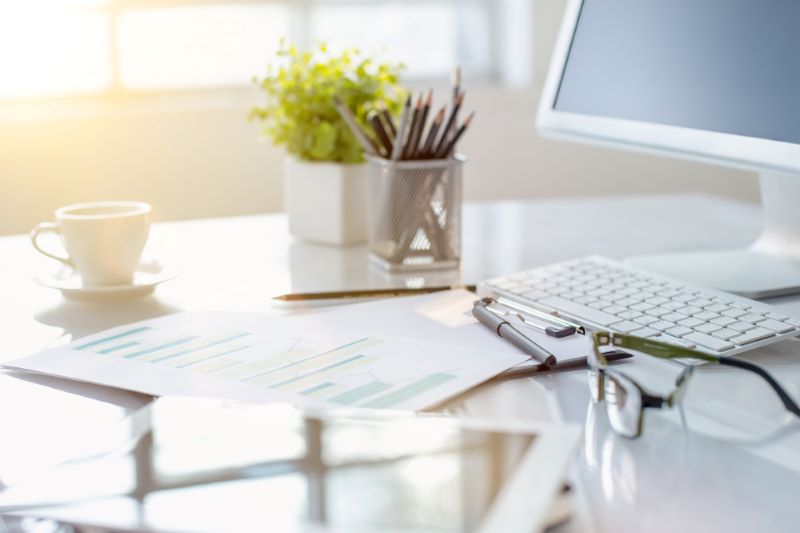
Hypertext Transfer Protocol (HTTP) requests are the primary means of communication between clients and servers. These requests follow a set structure and can be categorized into several types, most commonly being GET, POST, PUT, and DELETE.
GET requests are used to retrieve data from a server. When you type a URL into your browser, you send a GET request to the server to fetch and display the web page. In the context of APIs, GET requests are used to retrieve resources.
CURL POST requests, on the other hand, are used to send data to a server. This could be in the form of form data, JSON objects, or files. These cURL POST requests are essential when you need to create new resources or submit data to a server.
PUT requests are used to update existing resources on a server, while DELETE requests, as the name suggests, are used to remove resources.
Each HTTP request comprises headers and a body. The headers contain metadata about the request, like the content type or authentication tokens. The body contains the actual data being sent in the case of POST and PUT requests.
You’ll need to understand HTTP requests to use cURL, as it provides the basis for constructing requests and understanding server responses. CURL is a tool to make these HTTP requests outside a browser context, making it a valuable asset in testing and developing web applications.
Understanding POST Requests
A cURL POST request is a type of HTTP request method used by clients to send data to a server. The data is included in the body of the request and is typically used to create new resources, submit data to a server, or trigger certain actions. Examples of cURL POST requests in action include submitting a web form, uploading a file, or creating a new user in a database. Unlike GET requests, POST requests can send large amounts of data and do not leave a record in the browser history, making them more secure for sensitive data transmission.
Difference between GET and POST Requests
GET and POST are the two most commonly used HTTP request methods. The primary difference lies in their purpose and how they handle data. A GET request is used to retrieve data from a server, and it appends data to the URL in the form of query parameters. On the other hand, a cURL POST request is used to send data to a server, and it includes the data in the body of the request, making it suitable for sending large and sensitive data.
The Anatomy of a cURL POST Request
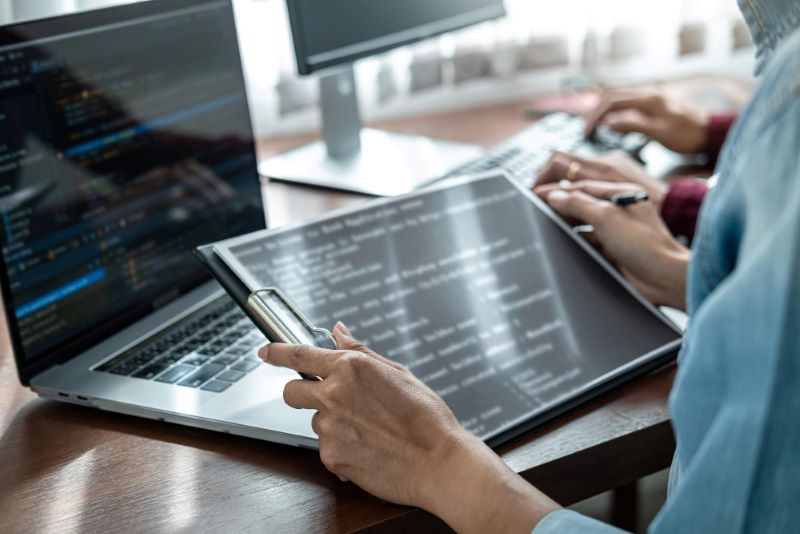
A cURL POST request consists of several key components: the request method, URL, headers, and the body. The request method for a cURL POST request is, of course, “POST”. The URL is the address to which the request is sent. The headers provide metadata about the request, such as the content type or authentication tokens. Lastly, the body contains the data being sent to the server.
The data in a cURL POST request body can be in various formats, including plain text, JSON, XML, or form data. The “Content-Type” header is particularly important in a cURL POST request because it tells the server what format the data is in, allowing it to interpret the data correctly.
Request Headers
Request headers are part of the HTTP request that provide metadata about the request. They define aspects such as the content type, the character set, acceptable response types, authorization information, and more. Headers are crucial in controlling how a cURL POST request or response should be handled. For instance, the “Content-Type” header tells the server the media type of the body, while the “Authorization” header is used to authenticate a user.
Request Body
The cURL request body is part of an HTTP request where data is stored that needs to be sent to the server. In a cURL POST request, this is typically where the data to create a new resource is placed. The data can take many forms, including form data, JSON, or XML, and the server uses the “Content-Type” header to interpret the body correctly.
Request URL
The request URL is the address to which the HTTP request is sent. For a cURL POST request, this URL typically corresponds to the server or API endpoint that will handle the creation of the new resource or process the provided data. It is important to note that, unlike GET requests, cURL POST request parameters are not included in the URL, enhancing the security of data transmission.
Executing a Simple cURL POST Request
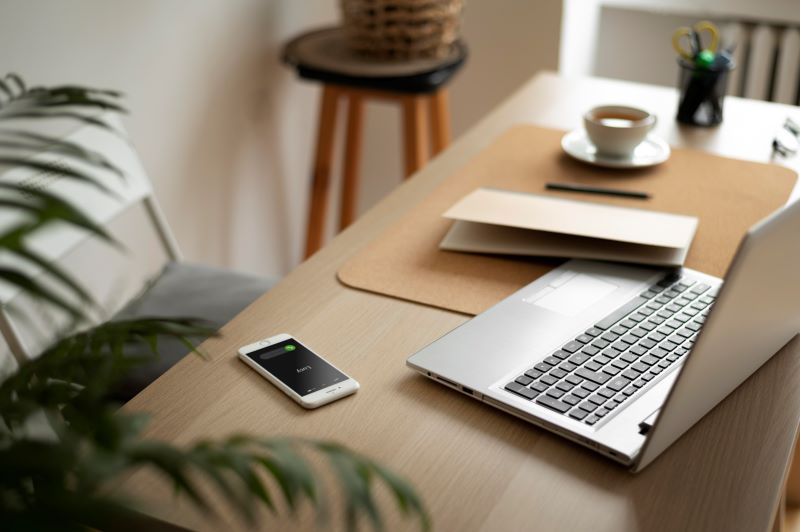
Executing a cURL send POST request involves using the -d or –data option, which allows you to specify the data you want to send to the server. For example, if you want to send a JSON object to a server, you could use the following command:
curl -X POST -H “Content-Type: application/json” -d ‘{“username”:”user”,”password”:”pass”}’ https://example.com/login
In this example, -X POST specifies the HTTP method. -H “Content-Type: application/json” sets the header to tell the server that we’re sending JSON data. The -d flag followed by the data in single quotes is the actual data we’re sending. The URL at the end is the endpoint where we’re sending the data. When sending JSON data, the JSON must be properly formatted, with names and string values in double quotes.
After executing this command, cURL will send the cURL POST request to the specified URL and then output the response from the server. This response will help you understand whether the cURL POST request was successful or if there were any issues.
Common Response Codes and What They Mean
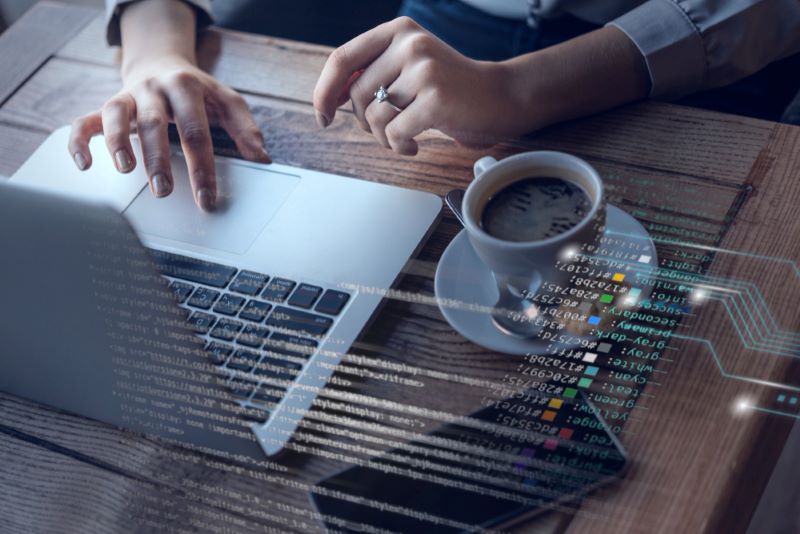
Response codes, also known as HTTP status codes, are three-digit numbers returned by servers to indicate the status of a web element. Here are some common ones you might encounter with a POST request cURL:
- 2xx – Success: These codes indicate that the request was successfully received, understood, and accepted. The most common is 200 (OK), which signifies that the request was successful and the response contains the requested data.
- 3xx – Redirection: These codes mean that the client must take additional action to complete the request. 301 (Moved Permanently) is a common one, indicating that the URL of the requested resource has been changed permanently.
- 4xx – Client Errors: These signify that there was an error in the request made by the client. A common code is 404 (Not Found), meaning the server couldn’t find the requested resource. Another is 400 (Bad Request), indicating that the server couldn’t understand the request due to invalid syntax.
- 5xx – Server Errors: These indicate that the server failed to fulfill valid request. The most common is 500 (Internal Server Error), meaning that an unexpected condition was encountered and no more specific message is suitable.
Advanced cURL Options for POST Requests
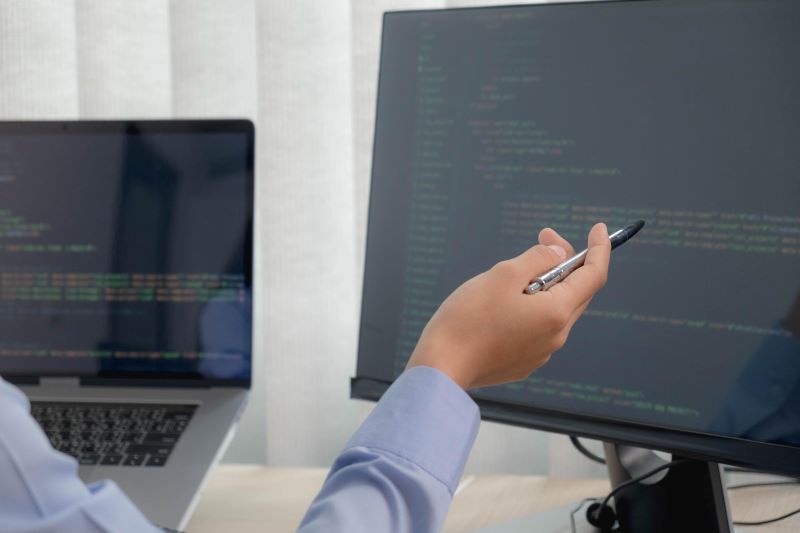
Once you understand the basics of how to cURL a POST request, you can try out some advanced techniques.
Customizing Headers
Customizing headers in a cURL POST request is essential for controlling the specifics of the request and the subsequent response. Headers allow you to set the content type, handle caching, authorization, and more.
To customize headers with cURL, you use the -H or –header option, followed by the header you want to set, in the format Header-Name: Header-Value. For instance, if you’re sending a POST request with JSON data, you’d need to set the “Content-Type” header to “application/json” to let the server know to expect JSON data:
curl -X POST -H “Content-Type: application/json” -d ‘{“key”:”value”}’ https://example.com
In this command, -H “Content-Type: application/json” sets the header.
Headers are also used for authorization. For example, you might use the “Authorization” header to include a token or credentials with your request:
curl -H “Authorization: Bearer YOUR_TOKEN” https://example.com
Multiple headers can be included by specifying -H before each one:
curl -H “Content-Type: application/json” -H “Authorization: Bearer YOUR_TOKEN” https://example.com
Customizing headers gives you more control over your HTTP requests and responses. It allows you to define the type of data being sent, provide authorization, control caching, and more. Understanding and using headers effectively can improve your interactions with web servers and APIs.
Sending Data in Different Formats
When sending data to a server using cURL POST requests, two of the most commonly used formats are JSON and form data. The choice between these two often depends on the requirements of the API or web server you’re interacting with.
JSON Data
JSON, short for JavaScript Object Notation, is a popular data interchange format due to its simplicity and compatibility with many programming languages. It’s particularly common when working with REST APIs.
To send JSON data using cURL, you need to set the “Content-Type” header to “application/json” and then include your data as a JSON-formatted string. The data must be properly formatted according to JSON syntax rules: property names and string values must be enclosed in double quotes, and each key-value pair must be separated by a comma.
Here’s an example of sending JSON data:
curl -X POST -H “Content-Type: application/json” -d ‘{“username”:”myuser”,”password”:”mypassword”}’ https://example.com/login
Form Data
Form data is the other commonly used format for sending data in POST requests. This format mimics the way a web browser sends data when a user submits a form and is often used when interacting with web pages and older APIs.
To send form data using cURL, you need to set the “Content-Type” header to “application/x-www-form-urlencoded”. Then, your data should be included as key-value pairs separated by an equal sign, with different pairs separated by an ampersand. Unlike JSON, form data does not require keys or values to be enclosed in quotes.
Here’s an example of sending form data:
curl -X POST -H “Content-Type: application/x-www-form-urlencoded” -d “username=myuser&password=mypassword” https://example.com/login
It’s worth mentioning that cURL can also handle multipart form data, often used for file uploads. To send a file, you need to precede the file path with an @ symbol. For instance:
curl -X POST -H “Content-Type: multipart/form-data” -F “file=@/path/to/myfile.txt” https://example.com/upload
In this command, -F is used instead of -d, which ensures the content type is set correctly for multipart form data.
Whether you’re sending JSON or form data, you need to know the expectations of the server or API you’re interacting with. Always check the documentation to determine if you’re using the correct data format, as this can prevent errors and ensure smooth data exchange.
Handling Redirects
HTTP redirects are server responses that inform the client that the requested resource resides at a different URL. This is commonly used when a webpage has moved to a new address and the server wants to guide the client to the new location. The status codes for these types of responses are in the 3xx range, with 301 (Moved Permanently) and 302 (Found, or Moved Temporarily) being the most common.
By default, cURL does not follow redirects. That is, if you make a request to a URL that returns a redirect response, cURL will display the redirect message, but it won’t automatically request the new URL provided in the Location response header.
If you want cURL to follow the redirect and automatically request the new URL, you can include the -L or –location option in your cURL command:
curl -L https://example.com
In this example, if https://example.com returns a 301 or 302 status code with a Location header pointing to a new URL, cURL will automatically send a new cURL POST request to that URL. However, automatically following redirects can sometimes pose security risks, especially when dealing with untrusted sources, so use the -L option judiciously.
Using Cookies and Sessions
Cookies are used to maintain state information between the server and the client across multiple requests. They are often used for tracking user sessions, personalization, and various other tasks. Cookies set by the server are sent back and forth in the headers of HTTP requests and responses.
In cURL, you can use the -b or –cookie option to send cookies with your request. For example:
curl -b “name=value” https://example.com
This command sends a cookie named “name” with the value “value” to https://example.com.
Sessions, which are used to persist user data across multiple requests, are typically managed with cookies. The server will often send a “Set-Cookie” header with a session ID, and the client should send back this session ID with each subsequent request to maintain the session.
To maintain sessions with cURL, you can use the -c or –cookie-jar option to save cookies after a request, and -b to send them with subsequent requests. For instance:
curl -c cookies.txt https://example.com
curl -b cookies.txt https://example.com
The first command saves any “Set-Cookie” headers from https://example.com to cookies.txt. The second command sends the cookies from cookies.txt with the request to https://example.com.
Dealing with Authentication
Authentication is a critical aspect of web development, as it verifies the identity of users and ensures that only authorized individuals can access certain resources. cURL supports several methods of authentication, making it a versatile tool for interacting with different APIs or web servers.
Basic Authentication
Basic Authentication is a simple method where the username and password are concatenated with a colon (username:password), base64-encoded, and sent in the “Authorization” header. To use Basic Authentication with cURL, you can use the -u or –user option, followed by the username and password separated by a colon:
curl -u username:password https://example.com
Bearer Tokens
Bearer tokens, commonly used in OAuth 2.0 and JWT (JSON Web Tokens), are another authentication method. The token is sent in the “Authorization” header with the format “Bearer: token”. To use a bearer token with cURL, you can set it using the -H or –header option:
curl -H “Authorization: Bearer your-token” https://example.com
Cookies
Cookies can also be used for authentication. When you log in to a website, the server usually sets a cookie with a session ID or similar identifier. Subsequent requests to the server include the cookie, which the server uses to identify the client.
curl -b “sessionid=your-session-id” https://example.com
In any authentication scenario, it’s critical to secure sensitive information like passwords and tokens, so always use secure connections (HTTPS) when sending this information.
Troubleshooting and Debugging a cURL Post Request
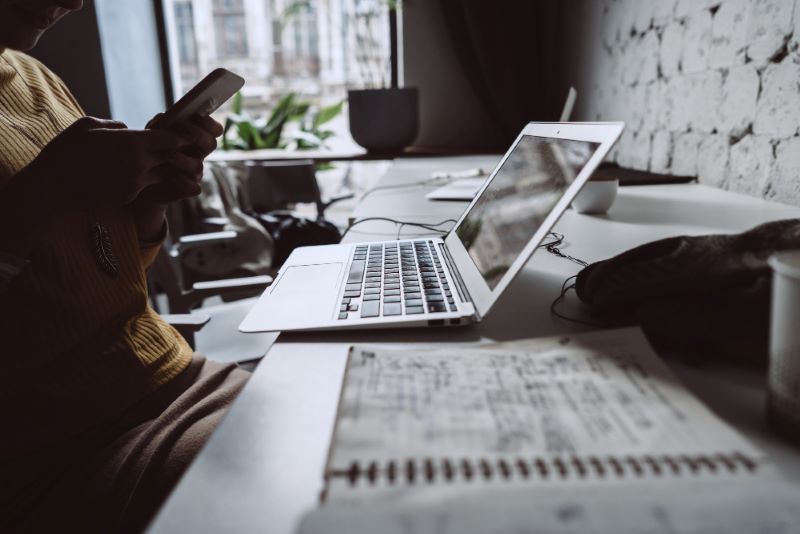
As with all aspects of development, understanding what can go wrong with a cURL POST request can help you figure out how to fix a bad cURL POST bad request.
Common Errors and Solutions
When working with cURL, you may encounter several common errors. Here are some, along with their solutions:
- Could not resolve host: This error occurs when the specified server cannot be found. This could be due to a typo in the URL, network issues, or DNS resolution problems. Check the URL you’re using and your network connection.
- Failed to connect to host: This error typically indicates a network or firewall issue. It means cURL can’t establish a TCP connection to the server. Ensure the server is running, the port is correct, and any firewalls are configured to allow the connection.
- SSL certificate problem: This error means that cURL can’t verify the SSL certificate of the server. If you’re testing locally or in a trusted environment, you can bypass this by adding -k or –insecure to your cURL command. However, for production usage, you should resolve the certificate issue or use a server with a valid certificate.
- Empty reply from server: This typically means the server accepted the connection but didn’t send a response. Check the server logs for any issues and ensure the server is configured to respond to the type of request you’re sending.
- Malformed: This error usually occurs with -d or –data when cURL cannot understand the data you’re sending. Ensure your data is properly formatted for the type of request you’re making. If you’re sending JSON data, use a linter to validate it.
- Operation timed out: This error occurs when cURL can’t establish a connection to the server within the default connect-timeout period (300 seconds). This could be due to network latency or the server being overloaded. You may try to increase the timeout using -m or –max-time option but ensure there isn’t a server or network issue that needs addressing.
- Upload failed: If you’re trying to upload a file or data, and cURL returns this error, it could mean that the server doesn’t support the type of upload you’re trying to do or the file or data you’re uploading is in the wrong format. Check the server’s documentation to ensure you’re uploading correctly.
- URL using bad/illegal format or missing URL: This error usually means that the URL you’re using in the cURL command is incorrectly formatted. Check that you’ve included the protocol, such as http:// or https://, and that the rest of the URL is correctly formed.
- Access denied: This error typically means that the server rejected your request due to a lack of authentication or insufficient permissions. Double-check that you’re sending the correct authentication information, such as tokens, cookies, or credentials, and that your user or token has permission to access the resource.
- Peer’s certificate issuer is not recognized: This error occurs when cURL doesn’t recognize the Certificate Authority (CA) that issued the server’s SSL certificate. If you trust the server and the CA, you can tell cURL to trust the certificate using the –cacert option with the path to the CA’s certificate. However, be careful when bypassing these warnings, as they can be a sign of a man-in-the-middle attack.
Using Verbose Mode for Debugging
The -v or –verbose option in cURL is a valuable tool for debugging. When used, cURL outputs detailed information about the request and response, including the headers and data sent and received. This can help identify issues with your cURL POST request.
For example:
curl -v https://example.com
This command will display a detailed log of the cURL POST request and response process. If the server doesn’t respond as expected, the verbose log can help identify the issue. You might discover that you’re sending incorrect headers, your request is being redirected, or the server is returning an error. The verbose output can include sensitive information, such as cookies or authorization headers, so be careful when sharing the output.
Best Practices for Troubleshooting
When troubleshooting a cURL POST request:
- Start simple: Begin with a simple request and progressively add complexity. This makes it easier to isolate the issue. If a simple GET request works but a POST request doesn’t, the issue likely lies with your POST data or headers.
- Use verbose mode: As mentioned, -v or –verbose gives detailed information about the request and response. This can be vital for identifying issues.
- Check the cURL man page: The cURL man page (man curl in a Unix-like system’s terminal) is a great resource. It provides detailed explanations of all options and features.
- Refer to API or server documentation: If you’re interacting with a specific API or server, refer to its documentation. It may have specific requirements for requests.
- Isolate the issue: If a request fails, try to isolate the issue. If you suspect the problem lies with the data you’re sending, try sending a request without it. If that works, you’ve likely found the issue.
- Be mindful of sensitive data: cURL commands often include sensitive data like tokens or passwords. Be careful when sharing commands or verbose output, and remember to redact sensitive data.
- Use online tools: Online validator tools can validate your JSON, XML, or form data, ensuring they are correctly formatted before you send them in a request.
cURL Request Examples
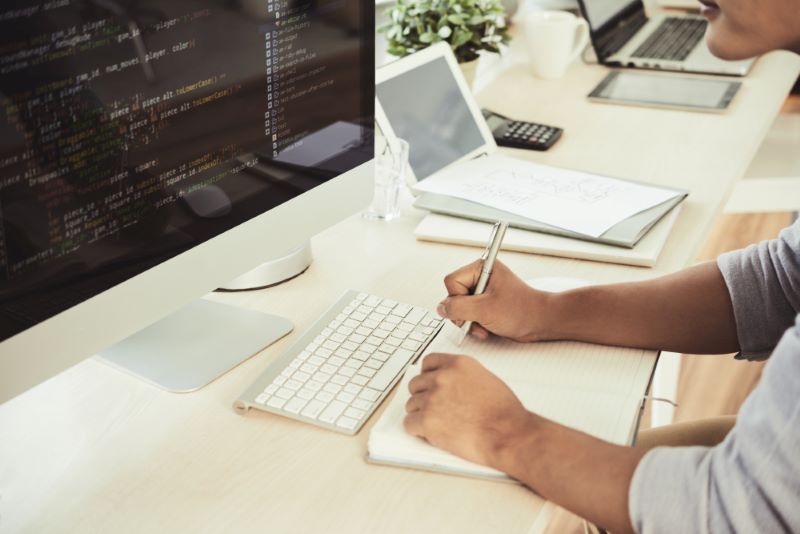
The use cases for cURL requests are extensive and varied. We’ll discuss some of the most popular real-world cURL post request examples to give you ideas for using this powerful tool.
Interacting with RESTful APIs
Interacting with RESTful APIs using cURL is a common practice in modern web development. These APIs, which follow the principles of Representational State Transfer (REST), are used to perform CRUD operations (Create, Read, Update, Delete) on server resources. Here are a few real-world examples and use cases for cURL POST requests and other types of cURL requests.
Fetching Data (GET Request)
Consider a weather application that fetches data from a weather API. Using cURL, developers can send a GET request to the API’s endpoint to retrieve current weather information:
curl https://api.weatherapi.com/v1/current.json?key=YOUR_KEY&q=London
The API responds with weather data in JSON format, which can be parsed and displayed to the user.
Creating a New Resource (POST Request)
Social media platforms often expose APIs to allow third-party developers to interact with their platforms. For example, a tool might automatically create new tweets based on certain triggers. This would involve sending a cURL POST request to the social media’s API:
curl -X POST -H “Content-Type: application/json” -d ‘{“status”:”Hello, Social Media!”}’ https://api.socialmedia.com/1.1/statuses/update.json
This cURL post request creates a new post with the text “Hello, Social Media!”.
Updating an Existing Resource (PUT/PATCH Request)
Suppose you’re using a task management API and you want to update the status of a task. This might involve a PUT or PATCH request, such as:
curl -X PUT -H “Content-Type: application/json” -d ‘{“status”:”completed”}’ https://api.taskapi.com/tasks/123
This request updates the status of the task with ID 123 to “completed”.
Deleting a Resource (DELETE Request)
Perhaps you’re working with a user management system and you need to delete a user. This would involve a DELETE request:
curl -X DELETE https://api.userapi.com/users/123
This request deletes the user with ID 123.
These examples demonstrate the power and versatility of cURL when interacting with RESTful APIs. Whether you’re fetching data, creating, updating, or deleting resources, a cURL post request is an invaluable tool for web development.
Automating Form Submissions With cURL POST Requests
Automating form submissions using cURL POST requests can save considerable time and effort in a variety of situations. Here are a few examples where this could be beneficial:
- Automated testing: If a web application has numerous forms that need to be tested to ensure proper operation, you can make the job easier with cURL. Writing scripts using cURL commands to automate form submission can greatly enhance efficiency. These scripts can emulate form filling and submission, checking for expected response codes and data to ensure the form is behaving correctly. This could also be an integral part of a larger continuous integration (CI) or continuous deployment (CD) process.
- Data entry automation: CURL can be useful for any organization that regularly submits large quantities of structured data into a web-based system through forms. This could be a university submitting student records to a government portal, or a business sending order data to a supplier’s website. A cURL script could automate this process, looping over the data entries, formatting them correctly for each form field, and submitting them.
- Web scraping: In cases where a site requires form submission to access certain data, such as search results, cURL can automate the process. For example, a researcher might need to extract information from a database that uses form-based search queries. They could write a cURL command to submit the form with various search terms and process the results.
- Automated login: For applications that require interaction with websites demanding authentication through a form, cURL can be utilized. The command-line tool can send a cURL POST request to the login form with the necessary credentials and maintain the session for subsequent requests.
Handling File Uploads
Handling file uploads is a common task in various applications, and cURL offers a powerful way to automate this process. Here are some real-world examples:
- Automated backups: Many services offer APIs that allow users to upload files. Cloud storage providers, for example, allow file uploads to their systems. cURL can be used in a backup script to automatically upload new or modified files to the cloud for safekeeping. The script could be scheduled to run regularly, providing automated, off-site backups.
- File transfers between systems: In an organization where files need to be shared across different systems, cURL can be an efficient tool. If a report is generated daily on a local server — and it needs to be uploaded to an internal web application via a REST API — a cURL POST request could automate this task, making it seamless and error-free.
- Testing file upload features: If you’re developing or testing a web application that includes file upload functionality, you can use cURL to simulate this action. This helps to ensure that the application can handle the upload as expected and be used for load testing.
- Data upload to machine learning platforms: Many machine learning platforms provide APIs to upload datasets. If you have a large local dataset that you want to upload for analysis, you can use a cURL POST request to automate the upload process. This can save considerable time and help streamline the data analysis workflow.
- Bulk media uploads to CMS: Content management systems often expose an API for uploading files. If you’re migrating a site or setting up a new one and have a large number of media files to upload, a script using cURL POST requests to automate the process can be a lifesaver.
Using Proxies with cURL post request
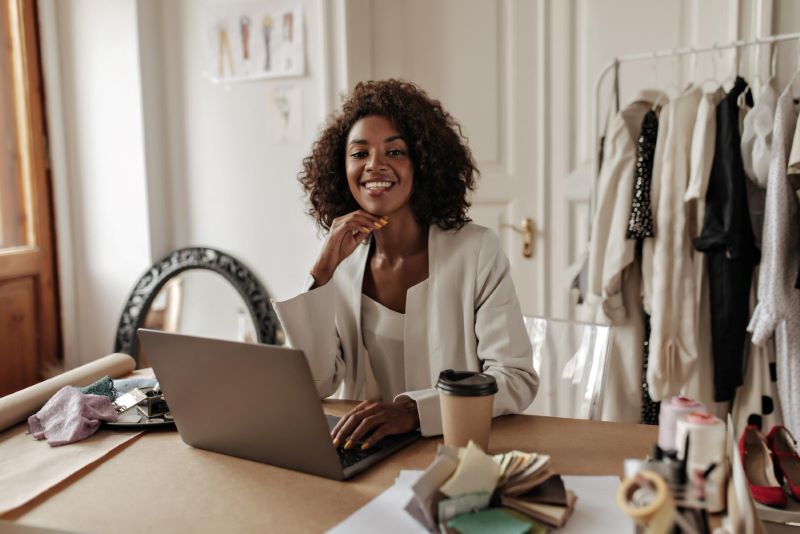
In some scenarios, you may need to use a cURL POST request with a proxy. This can be necessary if you’re behind a corporate firewall, need to obfuscate your location, or want to manage rate limits when interacting with certain APIs. To use a cURL POST request with a proxy, you can use the -x or –proxy option followed by the proxy address:
curl -x http://proxy-server:port https://example.com
If your proxy requires authentication, you can use the -U or –proxy-user option followed by the username and password:
curl -U user:password -x http://proxy-server:port https://example.com
All information sent over a proxy can potentially be viewed or logged by the proxy owner. So always ensure that you’re using a trusted proxy, such as Rayobyte, and secure connections. As always, respect privacy rules and terms of service. cURL’s versatility and robustness, combined with the user’s awareness of security and proper usage, ensure the most effective and safe application of this powerful command-line tool.
At Rayobyte, we want to be more than just your proxy provider. We want to be your data partner. Whether you need proxies to make your POST requests more efficient or a web scraping partner to collect metadata for you, we’re here for you.
Reach out today to find out how we can help with all your data needs.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.