How to Set Up A Proxy in C#
Did you know that websites may readily link your IP address to requests you make when you browse the internet directly? This exposure may damage your digital identity and result in online tracking and targeted advertising.
This is where the use of proxies can come in handy. They help you safeguard your digital identity by serving as a go-between for your computer and the internet. A proxy server uses its own IP address to send requests to websites on your behalf when you utilize it.
Proxies can help you circumvent IP bans, avoid geoblocking, and safeguard your identity when web scraping. In this post, you will discover how to use proxies in C# for all of your web scraping endeavors.
What Is C#?
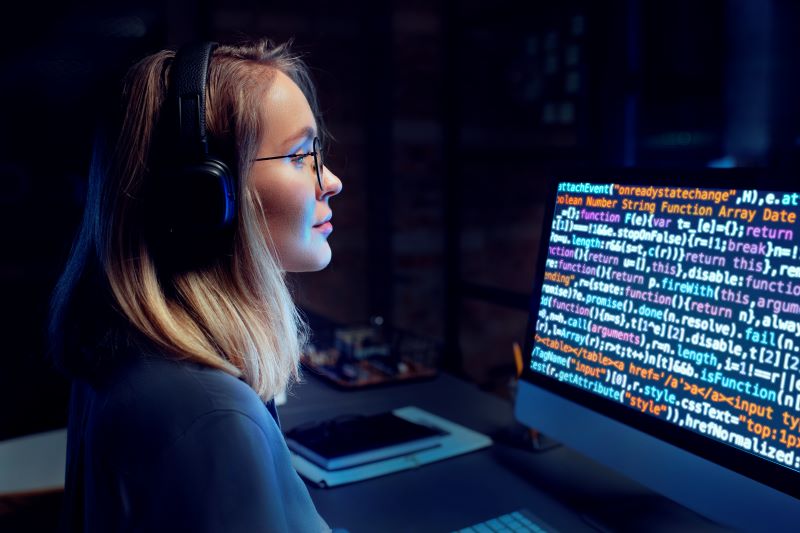
C# (pronounced “C-Sharp”) is a general-purpose, high-level programming language. It can be used to develop a wide variety of applications, from web and mobile apps to games and even enterprise software.
C# takes away the complexities of machine code, making it easier to read and write compared to lower-level languages. C# is also known for the following characteristics:
- Object-oriented: C# allows you to organize code using objects, which makes it more modular, reusable, and easier to maintain.
- Statically typed: In C#, you need to define the data type of variables beforehand, which helps catch errors early in the development process.
- Modern and evolving: C# is constantly being updated with new features and improvements.
Developers like using C# for many reasons, including the fact that it has a clean syntax and borrows concepts from other popular languages like C++ and Java, which makes it easier for beginners to pick up.
There’s also a vast community of C# developers and a wealth of learning materials available online and offline. This language can be used for various development projects, including setting up proxies, making it a valuable skill for programmers to learn.
Benefits of Setting Up a Proxy in C#
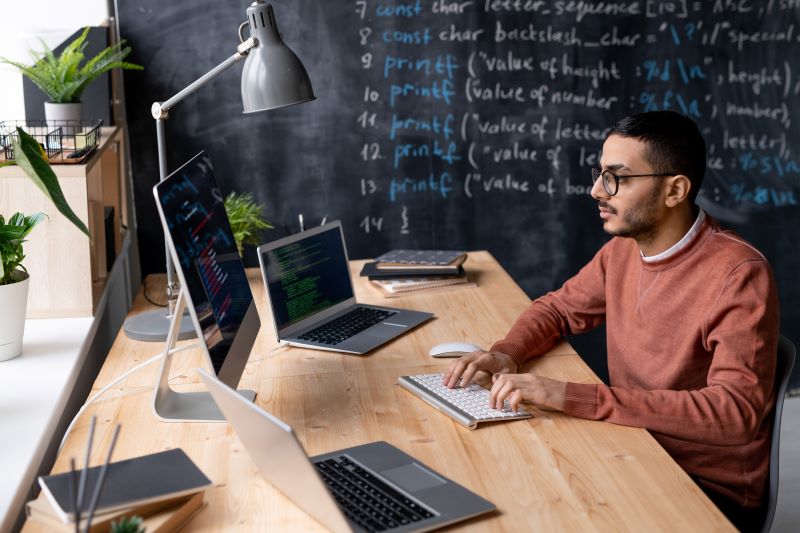
There are several benefits to setting up a proxy in C# applications:
- Security and anonymity: Proxies can hide your application’s IP address from the target server. This can be useful for the following:
- Privacy: By masking your IP, you can potentially avoid being tracked by websites or online services.
- Bypassing geo-restrictions: Some websites may restrict access based on your geographical location. A proxy server located in a different region can help you access such content.
- Scraping and data collection: Web scraping sometimes involves sending a high volume of requests to a website. Using a proxy can help distribute these requests and avoid overloading the server, potentially preventing you from getting blocked.
- Content filtering: Organizations can leverage proxies to control internet access for their employees. By filtering requests through a proxy, they can restrict access to specific websites or types of content.
- Load balancing and performance: In some cases, proxies can improve performance by caching frequently accessed content. This can reduce the load on the target server and speed up response times for your application.
- Testing and debugging: Proxies can be helpful for simulating different network conditions during development and testing. This can be useful for testing how your application behaves behind firewalls or with limited bandwidth.
Types of Proxies You Can Set Up in C#
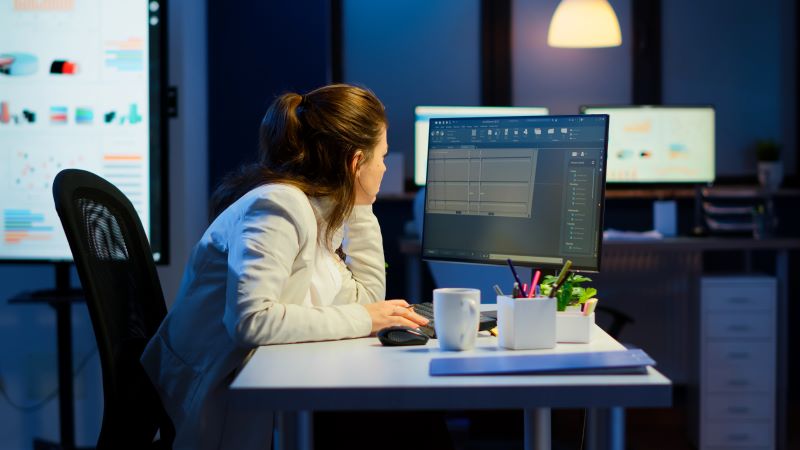
You can choose from several different types of proxies to experience the benefits shared above. The following are some of the most well-known options:
Residential proxies
Residential Proxies use IP addresses assigned to real devices like home computers or smartphones. They essentially borrow the IP address of a physical location from an Internet Service Provider (ISP).
Residential proxies work like this:
- You connect to a residential proxy service provider.
- Some providers allow you to choose a specific location (country, state, city, or ASN) for the residential IP address.
- Your internet traffic is routed through the chosen residential IP address.
- Websites perceive your connection as originating from a regular home or mobile device in the selected location.
Residential proxies offer several unique benefits.
For example, since the IP belongs to a real device, it appears more legitimate and blends in with regular internet traffic, making it harder to track your activity. You can also access websites or services restricted in your region by choosing a residential IP from the allowed location.
Businesses can use residential proxies to collect regional data or compare prices across different locations without being blocked. They can also manage multiple social media accounts from different locations to avoid detection of automation.
Data center proxies
Data center proxies are another type of proxy that routes your internet traffic through servers located in data centers.
These proxies are unique because they reside in data centers, facilities specifically designed to house computer systems and networking equipment. IP addresses are also assigned to these servers, not individual devices like home computers (unlike residential proxies).
Data center proxies are known for their fast performance. They are generally less expensive than residential proxies, too, and are better equipped to handle high-volume traffic.
ISP proxies
ISP proxies combine some aspects of both residential proxies and data center proxies, offering a unique blend of features.
ISP proxies obtain IP addresses from Internet Service Providers (ISPs), similar to residential proxies. However, unlike residential proxies that use real user devices, ISP proxies route traffic through servers located in ISP data centers.
These proxies combine the strengths of both residential and data center proxies.
For instance, they benefit from the optimized infrastructure of data centers and offer faster connections compared to residential proxies. However, they also have ISP-assigned AIPs that enhance anonymity and appear more legitimate than standard data center IPs.
How to Set Up a Proxy in C#
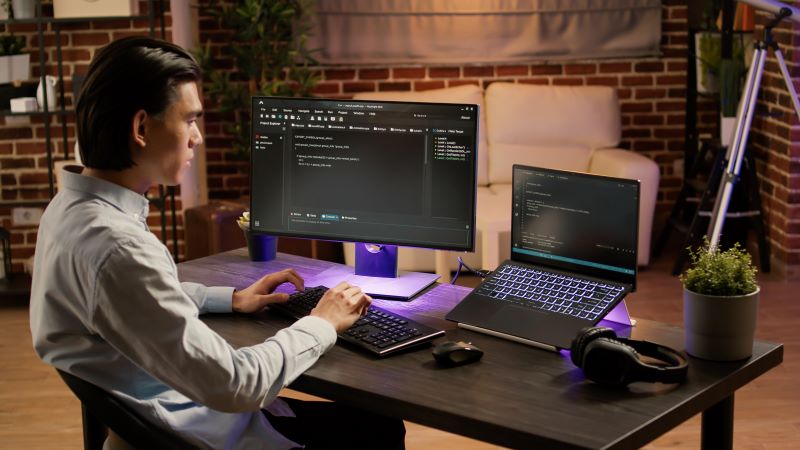
Now that you understand the benefits, let’s address the actual process of setting up a proxy in C#.
The primary approach to setting up a proxy in C# involves using HttpClient with proxy configuration and using system configuration.
This method leverages the HttpClient class, which is a popular choice for making HTTP requests in C#.
If you take this approach, you’ll start by defining the proxy. To do this, you’ll need the proxy address (URL) and port number. You may also need a username and password if the proxy requires authentication.
Next, you’ll create and configure an HttpClientHandler object.
To create an HttpClientHandler object, set the Proxy property of HttpClientHandler to a new WebProxy instance. Then, provide the proxy address and port to the WebProxy constructor.
If authentication is needed, set the Credentials property of WebProxy with your username and password.
Now, you can create an HttpClient with Handler. Here’s an example of the code you might use to accomplish this:
C#
string proxyAddress = “http://your_proxy_address”;
int proxyPort = 8080;
string username = “your_username”; // Optional for authentication
string password = “your_password”; // Optional for authentication
var handler = new HttpClientHandler();
handler.Proxy = new WebProxy(proxyAddress, proxyPort);
if (!string.IsNullOrEmpty(username) && !string.IsNullOrEmpty(password))
{
handler.Proxy.Credentials = new NetworkCredential(username, password);
}
var httpClient = new HttpClient(handler);
What About C# Console Log?
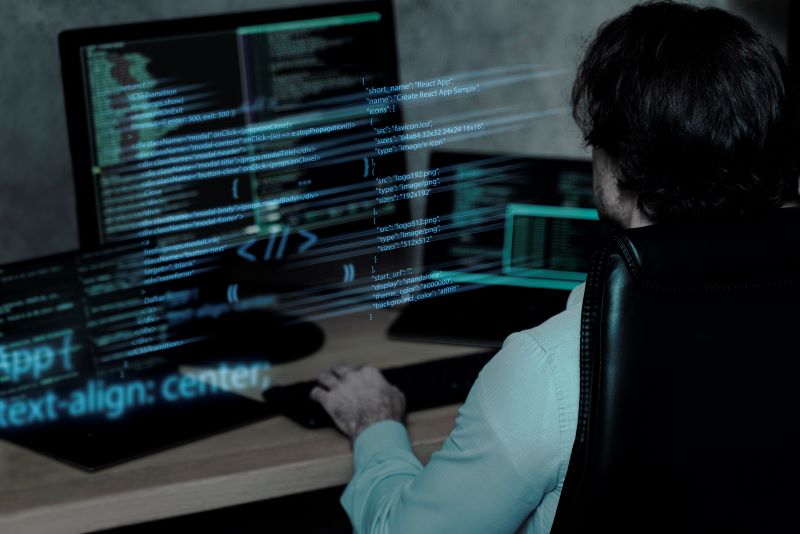
As you learn about the C# programming language and seting up a proxy in C#, you will also likely hear about C# console log. What is this, and what does it have to do with proxies?
First, let’s define C# console log.
C# console logging refers to displaying messages or information in a text-based window called the console. This window is separate from the graphical user interface (GUI) of your application — if it has one.
Console logging involves the following components:
- Console class: The primary tool for console logging in C# is the Console class from the System namespace.
- Logging methods: This class provides methods to write data to the console:
- WriteLine: This is the most common method. It writes the provided string to the console, followed by a newline character, which moves the cursor to the next line.
- Write: This method writes the string to the console without adding a new line. You can use this to gain more control over formatting the output.
Here’s a basic example of console logging in action:
Console.WriteLine(“Hello, world!”); // Prints “Hello, world!” followed by a newline
Console.Write(“This is part 1″);
Console.Write(” This is part 2.”); // Prints “This is part 1 This is part 2.”
There’s no direct relationship between console logging and proxies in their core functionalities. However, there can be some indirect connections.
For example, if you’re setting up a proxy in your C# code using the HttpClient class, you might use console logging to print details about the proxy configuration (address, port) for verification purposes.
If you encounter issues with your proxy connection, console logging might also be helpful for debugging. You could log error messages returned by the HttpClient or related libraries to understand the problem.
How to Do Web Scraping in C# with a Proxy
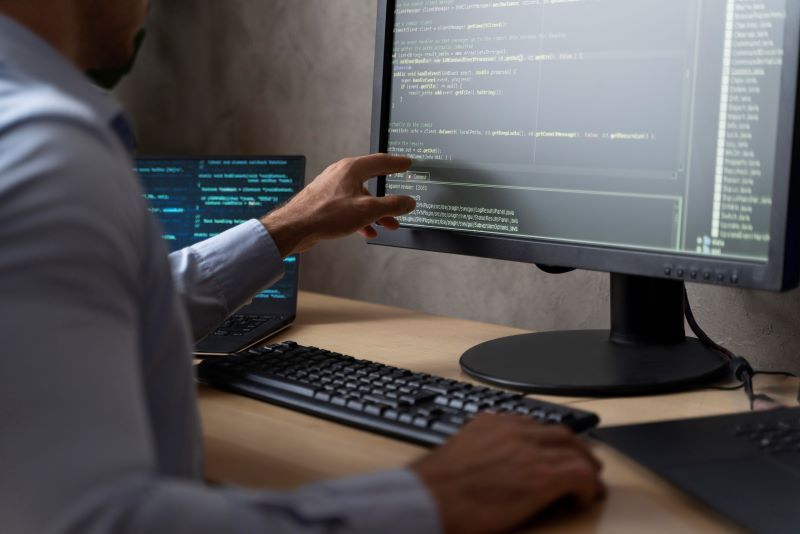
Many people use proxies for web scraping, a process of collecting data automatically from various websites across the internet. Web scraping involves fetching the HTML code of a web page, parsing it, and then extracting the desired information from it.
Now that you’ve learned how to set default proxy in C#, you might be wondering how to use that proxy for web scraping tasks.
Web scraping with a proxy in C# involves two main steps: Making HTTP requests with a proxy and parsing and extracting data.
When making HTTP requests, you will leverage the HttpClient class and configure it to use a specific proxy server — as we described above. Once you have collected webpage content using HttpClient, your next job is to parse it to extract the specific desired data.
A popular library for this task is HtmlAgilityPack. This library can help you navigate and manipulate HTML content.
Step by step
The process of conducting a web scraping task with a proxy in C# starts with defining proxy details. To do this, you’ll need to specify the proxy address, port, and relevant authentication credentials (i.e., your username and password).
Next, you’ll configure HttpClient by creating an HttpClientHandler and setting the proxy property to a WebProxy instance that has been configured with your unique proxy details.
From here, you can instantiate an HttpClient object using the configured HttpClientHandler.
Now, you’re ready to make a C# webrequest.
Use the HttpClient to send a GET request to the target URL. Then, store the response content in a string variable.
Your next job is to parse the HTML Content (using HtmlAgilityPack).
To do this, you’ll create an HtmlDocument object from the retrieved HTML content. Then, use HtmlAgilityPack methods to navigate the HTML structure and locate the elements containing your desired data.
Finally, the data will be extracted using popular methods like InnerText or Attributes[“attribute_name”].
Here’s an example of how the code might look when carrying out this process:
using System.Net.Http;
using HtmlAgilityPack;
// Define proxy details (replace with your actual values)
string proxyAddress = “http://your_proxy_address”;
int proxyPort = 8080;
// Configure HttpClient with proxy
var handler = new HttpClientHandler();
handler.Proxy = new WebProxy(proxyAddress, proxyPort);
var httpClient = new HttpClient(handler);
// Make request to target URL
string url = “https://www.example.com”;
var response = await httpClient.GetStringAsync(url);
// Parse HTML content
var htmlDoc = new HtmlDocument();
htmlDoc.LoadHtml(response);
// Extract data (replace selectors with your target elements)
var titles = htmlDoc.DocumentNode.SelectNodes(“//h1 | //h2”); // Get all h1 or h2 elements
var data = new List<string>();
foreach (var title in titles)
{
data.Add(title.InnerText); // Extract text content of each element
}
// Use the extracted data (titles in this example)
Console.WriteLine(“Extracted Titles:”);
foreach (var title in data)
{
Console.WriteLine(title);
}
How to Create a C# New List
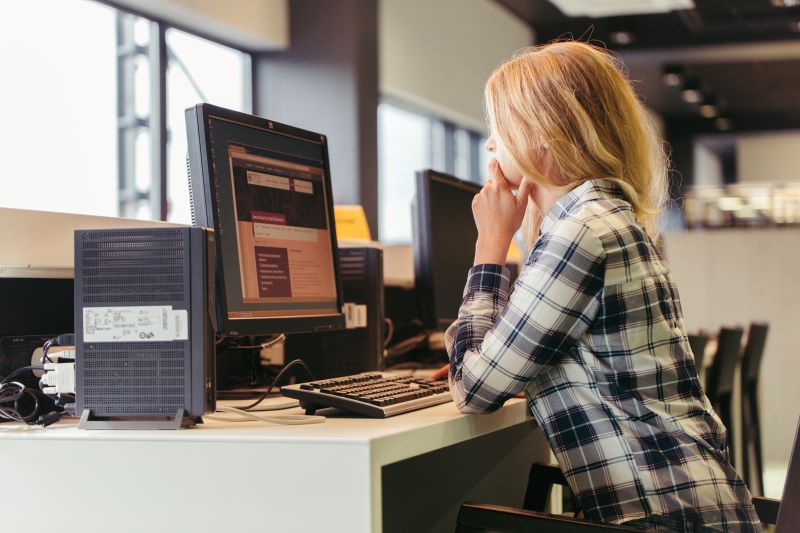
Once you’ve collected a bunch of data through web scraping, you’ll need to sort and organize it so you can use it to make more informed decisions. The good news is that it’s relatively easy to create a new list in C#.
There are two main ways to create a new list in C#: Using the List<T> constructor and using a collection initializer.
Using the List<T> constructor
This is the most common and flexible approach. Here’s a breakdown of the code you would use:
List<T> listName = new List<T>();
List<T> specifies that you’re creating a list collection.
T is a placeholder for the data type that the list will hold. It can be any data type, like int, string, a custom class, etc.
listName is the name you choose for your list.
Using a collection initializer
A collection initializer is a shorthand way to create a list with initial elements. Here’s a breakdown of the code involved in this process:
List<T> listName = new List<T>() { item1, item2, …, itemN };
Same as before, T represents the data type and listName is your chosen name.
The curly braces {} enclose a comma-separated list of initial elements you want to add to the list.
How to Create a Set in C#
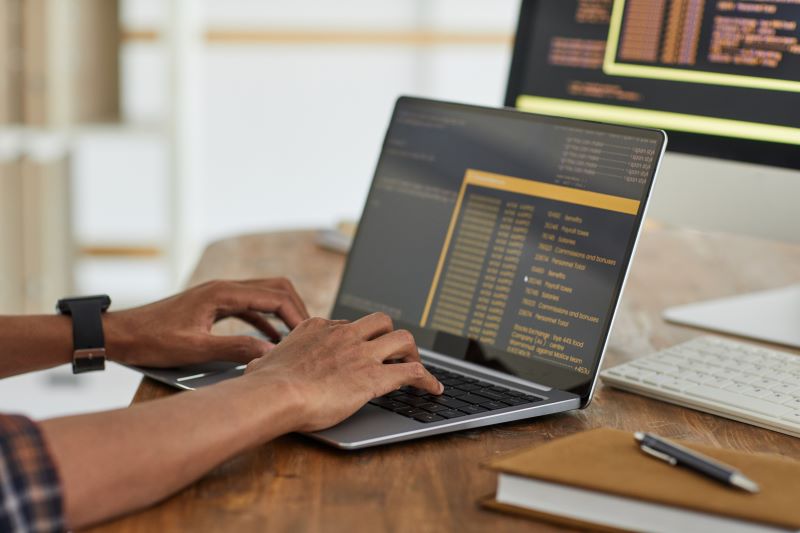
You can also use C# to organize your data by creating sets. A set is similar to a list, but the main difference is that lists can store duplicates, and sets cannot.
There are two main ways to create sets in C#: using HashSet<T> and SortedSet<T>.
HashSet<T>
This option is the most common way to create a set. It stores elements in a hash table for efficient lookups. Elements are not ordered, and duplicates are not allowed.
Here’s an example of the code you would use to create a HashSet:
HashSet<string> names = new HashSet<string>();
This code creates an empty set of strings. You can also initialize it with a collection of elements:
string[] colors = { “red”, “green”, “blue”, “red” };
HashSet<string> uniqueColors = new HashSet<string>(colors);
This creates a HashSet named uniqueColors containing only unique elements from the colors array.
SortedSet<T>
If you need the elements in a set to be ordered, you can use this option. It features lower lookups than the previous approach, but duplicates are still not allowed.
Use this code to create a SortedSet
SortedSet<int> numbers = new SortedSet<int>();
This code would create an empty set of integers that will be sorted in ascending order.
Need a Working Proxy ASAP?
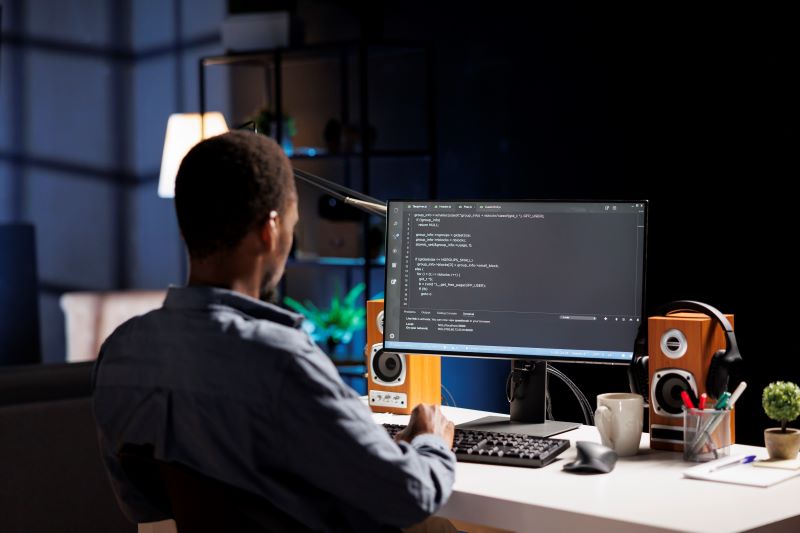
Now that you’re better versed in the ins and outs of C# and understand how it can be used to set up proxies (and organize the data you’ve collected using proxies during web scraping), are you feeling more confident in your abilities?
The information shared above can be very useful for those interested in using proxies. What if you’re not very tech-savvy or knowledgeable of C#, though? Is there an option for you?
Short answer — yes. This is where a proxy service can be useful.
A proxy service is a third party that provides access to various types of proxies that will act as a middleman between devices and the internet. Working with a proxy service is an excellent alternative that allows you to experience all the benefits of proxies without having to do a lot of in-depth coding.
What should you look for in a proxy service? Here are some of the most important factors to consider:
Proxy types offered
Start by evaluating the different types of proxies each service offers. Do they specialize in one specific proxy type, such as residential or ISP proxies, or do they have multiple options for you to choose from?
Consider your specific needs and what you want to do with proxies so you can pick the right kind (and then choose a provider that offers them).
Customer service
Look for a proxy service that offers round-the-clock, 24/7 customer support. It should be easy for you to get in touch with a representative if something goes wrong when you’re setting up or using your proxies.
Pricing
Of course, you’ll need to take pricing into account as well.
Be wary of free proxies, as they often have limitations when it comes to speed and overall performance. At the same time, though, that doesn’t mean you have to spend a fortune to experience the advantages proxies offer.
Pay attention to the pricing structure and options available for different proxy types, then weigh your options to determine which proxy service gives you the best deal.
Free trial
Free proxies can be problematic, but there’s nothing wrong with a provider offering a free trial so you can experiment with their proxies before you commit. If you’re on the fence and having a hard time choosing between two prosy services, try both of them out to see which one works better for you.
User experience
Take particular note of the user experience each proxy service provides.
How easy is it to navigate the platform and set up the proxies? How well do they work to help you accomplish your specific objectives? Are you able to figure out issues as they arise, or do you get stuck with nowhere to turn?
Reviews and recognition
Finally, don’t forget to look for positive reviews from past customers. Check out third-party review sites to find objective testimonials and get a better sense of what users like and dislike about a particular proxy service.
Check to see if the proxy service has been recognized by any professional organizations, too. That can be a sign that they are a credible provider and worth working with.
Final Thoughts
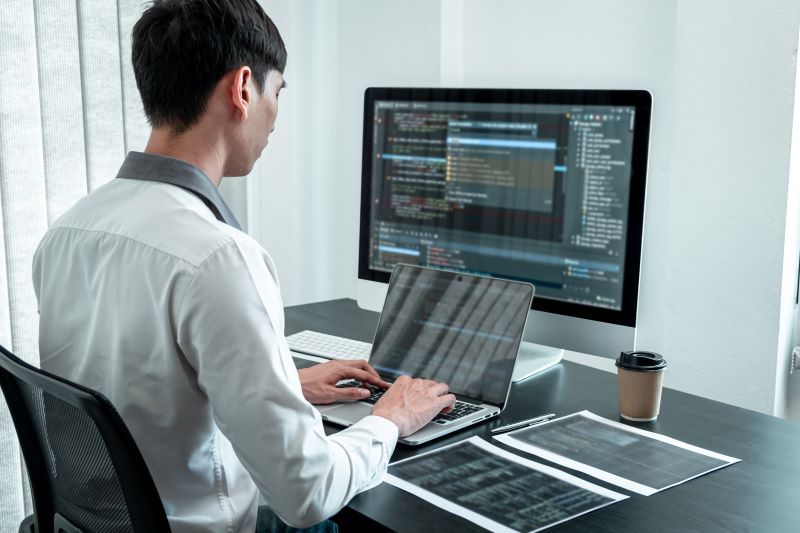
Figuring out how to set up a proxy in C sharp might seem like an impossible task at first. It’s not as complicated as you might think, though, especially if you follow the tips and suggestions shared in this guide.
Are you ready to use your newfound skills — including those related to C# console log — to set up proxies in C#, or are you looking for a more ready-made solution? If you prefer the latter, check out Rayobyte today. Rayobyte is a leading proxy provider focused on sharing reliable, easy-to-use proxies. Sign up today to start your free trial!
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.