Web Scraping Using API in Python
Whether it’s gathering competitive pricing data, monitoring market trends, or collecting content for research, web scraping helps businesses and individuals automate the data collection process at scale. However, traditional web scraping methods can be complex and time-consuming, especially when dealing with challenges like CAPTCHAs, IP blocking, or dynamic content. This is where a web scraping API in Python comes in.
APIs simplify and streamline the scraping process, enabling developers to access and collect data efficiently without worrying about the underlying technical hurdles. Rayobyte’s Web Scraper API, for example, provides a reliable, scalable, and easy-to-integrate solution that handles proxy rotation, dynamic content, and CAPTCHA bypassing automatically. With its user-friendly interface and robust features, Rayobyte empowers users to focus on data collection, not the logistics of web scraping.
As a leader in web scraping solutions, Rayobyte offers a powerful API designed to meet the needs of both small-scale scrapers and large enterprise projects. In this post, we’ll explore the ins and outs of web scraping using API in Python, breaking down how Rayobyte’s Web Scraper API can help with efficient, reliable web scraping.
Fast, Effective, Efficient
Get the API that can meet your web scraping needs.

What is Web Scraping?
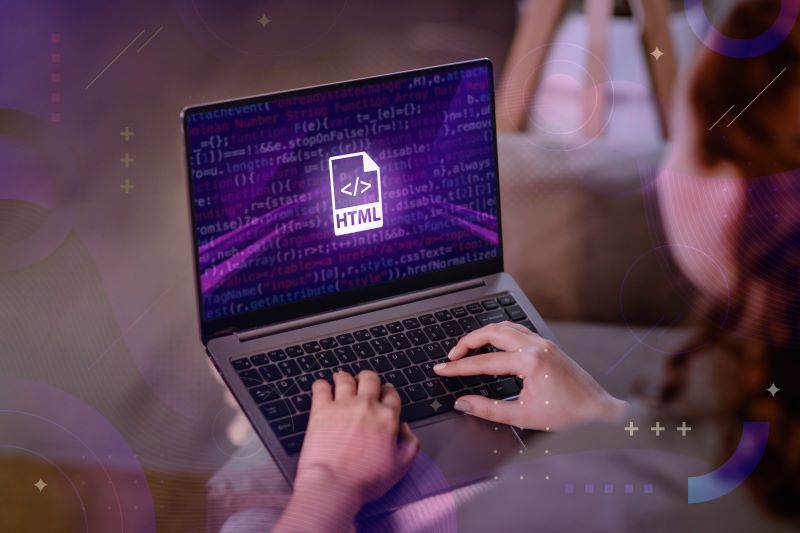
Web scraping is the automated process of extracting data from websites. It involves retrieving content from web pages, parsing it, and structuring it for analysis, storage, or use in other applications.
Despite its many benefits, web scraping can be challenging. Websites are designed to prevent unauthorized scraping in various ways, which can make data extraction more difficult. Common obstacles include:
- CAPTCHAs, which are used to verify that a human is accessing the site
- IP blocks, which prevent scrapers from making repeated requests
- Dynamic content that requires rendering JavaScript before extracting data
To overcome these challenges, APIs like Rayobyte’s Web Scraper API offer an efficient and reliable solution. Rather than handling complex scraping tasks manually, users can leverage the API to automate the process, avoiding many of the hurdles that traditional scraping methods face.
Introduction to Rayobyte Web Scraper API
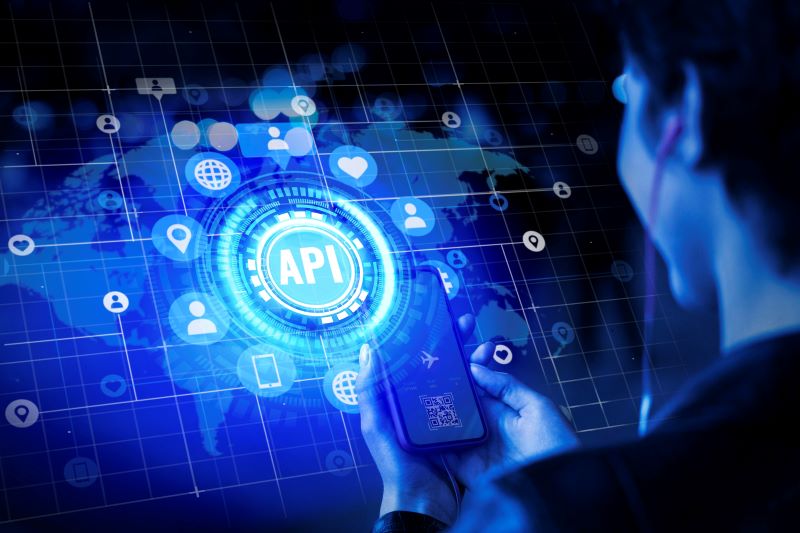
Rayobyte’s web scraping Python API is a powerful, automated solution designed to simplify the web scraping process. It allows users to extract data from websites at scale without having to manage the complexities of proxy rotation, CAPTCHAs, or dynamic content.
The API works by enabling users to send requests to a server, which then performs the scraping on their behalf and returns structured data in a format of their choice — whether that’s JSON, CSV, or another format. This enables businesses and developers to automate large-scale data extraction projects without the need for manual intervention, saving both time and resources.
One of the primary advantages of Rayobyte’s Web Scraper API is its ability to avoid many of the common roadblocks encountered in web scraping.
Traditional scraping methods often run into issues like IP blocking, CAPTCHAs, and dynamic content that requires JavaScript rendering. Rayobyte’s API handles all of these challenges automatically, ensuring a smooth and uninterrupted scraping process.
By leveraging proxy rotation, the Python API for web scraping makes it appear as though requests are coming from different IP addresses, preventing websites from blocking or throttling traffic. Additionally, it can bypass CAPTCHAs and other anti-bot measures, allowing you to access even the most secure and protected websites.
The API is designed to handle large-scale scraping tasks with ease. Whether you need to scrape a few pages or millions of them, Rayobyte can scale to meet your needs. It offers a RESTful interface that is easy to integrate with Python or other programming languages, making it simple for developers to incorporate into their existing workflows.
Key features include:
- Custom User Agents: Modify the user agent to mimic different browsers or devices.
- Proxy Rotation: Automatically rotate proxies to avoid IP blocking and maintain anonymity.
- Real-Time Data Extraction: Extract data instantly, without the need for delays.
- Scheduling and Task Management: Set up recurring scraping tasks to run at specific intervals.
- Data Output in Structured Formats: Receive data in well-organized formats like JSON, CSV, or XML.
Why Use Rayobyte’s API?
Rayobyte’s API is the perfect solution for mastering web scraping and API fundamentals in Python. Here are some specific advantages it offers:
- High Reliability and Uptime: Rayobyte’s infrastructure ensures minimal downtime, providing users with reliable data extraction.
- Scalable for Large Projects: Whether you’re scraping thousands or millions of pages, Rayobyte’s Web Scraper API can scale with your project’s demands.
- Excellent Customer Support: Rayobyte’s dedicated support team is available to assist with any issues or questions, ensuring a smooth experience.
- Easy-to-Use: The API is simple to integrate into Python or other languages, making it accessible even for developers with minimal web scraping experience.
Rayobyte’s Web Scraper API is designed to handle the complexities of web scraping while offering flexibility and scalability, making it an ideal solution for developers and businesses seeking efficient data extraction tools.
Getting Started with Python for Web Scraping
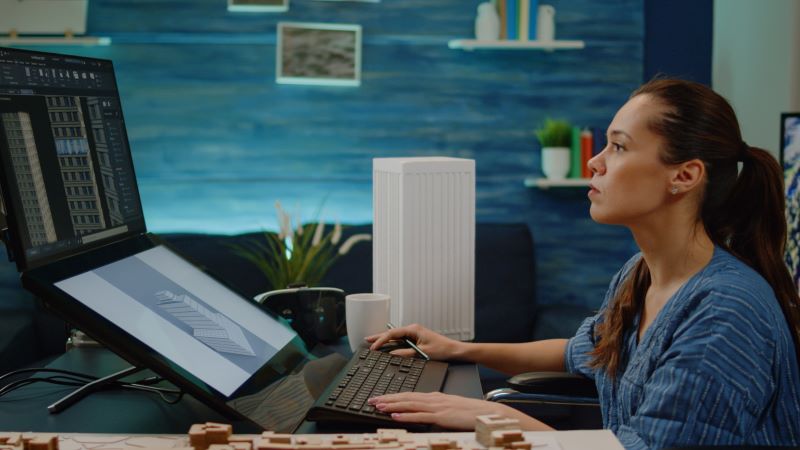
Python’s simplicity, readability, and powerful libraries make it an ideal choice for developers looking to automate data extraction tasks.
Python’s vast ecosystem of libraries for web scraping, combined with strong community support, means that developers can quickly find tools and resources to solve almost any scraping challenge. Whether you’re extracting data from static web pages or interacting with dynamic content, Python provides flexible solutions for both.
There are several key Python libraries commonly used for web scraping, each serving a specific purpose.
Requests
The Requests library is essential for making HTTP requests to fetch web data. It allows you to send GET and POST requests to retrieve HTML content from web pages, making it the foundation of most web scraping scripts. Requests simplifies the process of sending requests and handling responses, making it user-friendly and easy to integrate.
Here’s an example:
import requests response = requests.get("https://example.com") html = response.text
BeautifulSoup
Once you have retrieved the HTML content of a page, BeautifulSoup comes in handy for parsing and extracting data. It provides an intuitive way to navigate and search the HTML structure, enabling you to extract specific elements like text, links, images, or tables.
Here’s an example:
from bs4 import BeautifulSoup soup = BeautifulSoup(html, 'html.parser') title = soup.find('title').text
Selenium
For websites that rely heavily on JavaScript to load content dynamically, Selenium is a valuable tool. Unlike Requests and BeautifulSoup, which only work with static HTML, Selenium automates browser interactions and can render JavaScript content. It simulates user behavior, making it ideal for scraping sites with complex, interactive elements.
Here’s an example:
from selenium import webdriver driver = webdriver.Chrome() driver.get("https://example.com") html = driver.page_source
Installing Required Libraries
To get started, you’ll need to install the necessary libraries. You can easily install Requests, BeautifulSoup, and Selenium using pip:
pip install requests beautifulsoup4 selenium
These libraries are often used together to handle different aspects of web scraping. For instance, you might use Requests to fetch the HTML content of a page, then parse it with BeautifulSoup to extract the data you need. If the website is JavaScript-heavy, you can use Selenium to load the page and execute any necessary scripts before scraping the content.
How to Use Rayobyte’s Web Scraper API with Python
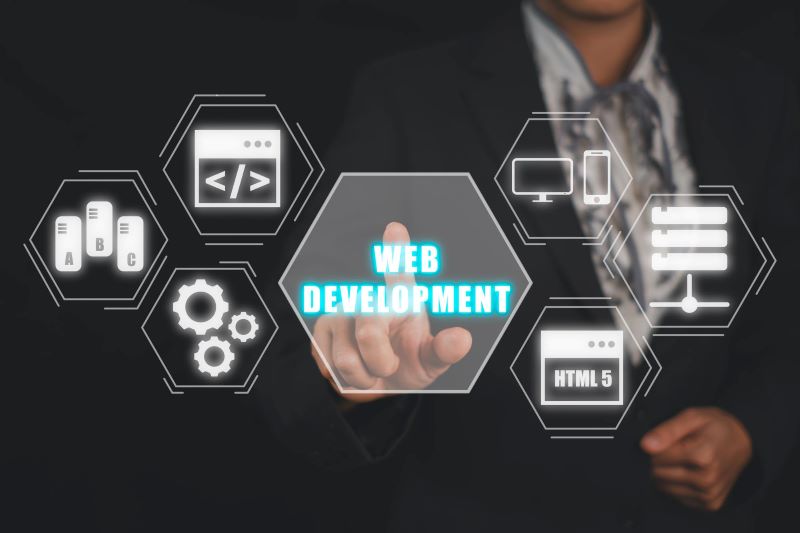
Getting started with Rayobyte’s Web Scraper API is straightforward and can significantly streamline your web scraping projects. Below, we walk through the process of setting up and using the Python API for web scraping, from creating an account to scraping data from a website.
Setting Up the API
Here are the steps you’ll take to set up the API:
- Create an Account at Rayobyte: First, you’ll need to create an account on Rayobyte’s platform. Sign up for an account here.
- Get Your API Credentials: After creating your account, log in to your Rayobyte dashboard to obtain your API key. This key is essential for authenticating your requests and allows you to interact with the Web Scraper API securely. Once you have your API key, keep it safe as you’ll need it in your code.
- Install Necessary Libraries:
To interact with the Rayobyte API in Python, you’ll need to install the requests library, which simplifies making HTTP requests. Run the following command in your terminal to install it:- pip install requests
The requests library will allow you to send GET and POST requests to the Rayobyte API, enabling you to perform web scraping tasks.
Example Python Code to Authenticate and Make a Request
Once the setup is complete, you can use the following Python code to authenticate and make a simple request to Rayobyte’s API.
import requests # API URL for scraping url = "https://api.rayobyte.com/v1/scrape" # Set up headers with your API key for authentication headers = { "Authorization": "Bearer <YOUR_API_KEY>" } # Send a GET request to the Rayobyte Web Scraper API response = requests.get(url, headers=headers) # Check if the request was successful if response.status_code == 200: data = response.json() # Parse JSON response print(data) # Output the response data else: print(f"Error: {response.status_code}") # Output error message
This code sends an authenticated request to the API and prints the response. If the request is successful (status code 200), it will print the scraped data in JSON format. If there’s an issue, it will print the error code.
Making a Web Scraping Request
Now that you have a basic understanding of how to interact with the API, let’s move on to actually scraping data from a specific website using the Rayobyte Web Scraper API. In this example, we’ll scrape product data from an e-commerce site.
Here’s how to set up your scraping request:
- Set the Target URL: The target_url parameter specifies the website you want to scrape. In this case, we’ll scrape a hypothetical e-commerce site’s product page.
- Customize the Request: You can also pass custom headers (like the User-Agent), and specify the desired output format (such as JSON).
scrape_url = "https://example.com/products" api_url = "https://api.rayobyte.com/v1/scrape" # Define payload with target URL and headers payload = { "target_url": scrape_url, "headers": {"User-Agent": "Mozilla/5.0"}, "format": "json", # Data format (JSON, CSV, etc.) } # Send the request to Rayobyte's API response = requests.post(api_url, json=payload, headers=headers)
In this example, the payload includes the following parameters:
- target_url: The URL of the website you want to scrape (in this case, product data).
- headers: Custom headers to avoid detection, such as a common User-Agent string.
- format: Specifies the output format (JSON is used in this case).
Handling API Responses
Once you’ve sent the scraping request, you’ll need to handle the API’s response. The response will usually contain the scraped data in the format you requested (e.g., JSON). Here’s how you can process and output the data:
if response.status_code == 200: result = response.json() # Parse JSON response # Assuming the response contains product data in a 'data' field for item in result['data']: print(item['product_name'], item['price']) # Print product name and price else: print("Error: Could not fetch data") # Handle errors if request fails
If the request is successful (status code 200), the response.json() method parses the JSON response into a Python dictionary. You can then loop through the data to extract specific details, such as the product name and price.
If the request fails, the script will print an error message indicating the problem.
Advanced Features of Rayobyte’s Web Scraper API
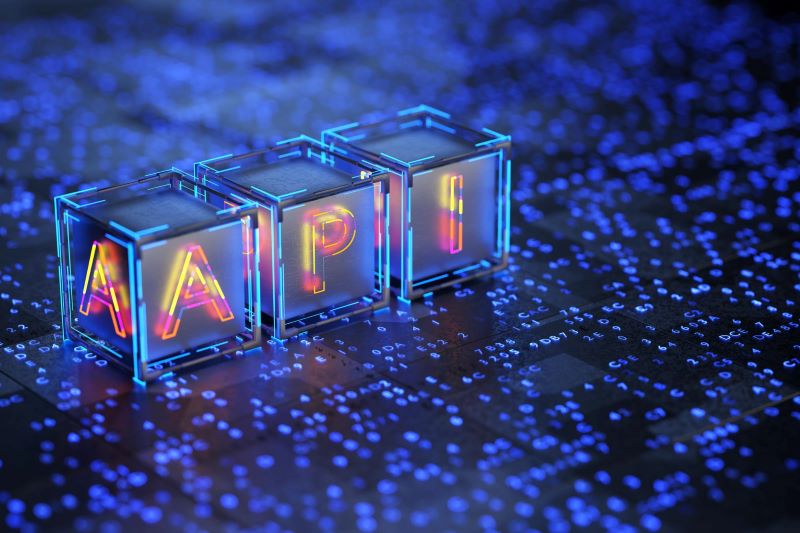
Python web scraping using this API provides a range of advanced features that help users overcome common web scraping challenges.
Proxy Rotation and Avoiding Blocks
One of the most significant challenges in web scraping is dealing with IP blocking.
Websites often block IPs that make too many requests in a short time or that appear to be scraping data. Rayobyte’s Web Scraper API addresses this issue by implementing proxy rotation.
Proxy rotation allows the API to automatically switch between different IP addresses, making it appear as though requests are coming from different users or devices. This prevents the scraper from getting flagged or blocked by the target website.
This feature is crucial for scraping large-scale websites or those that are frequently targeted by scrapers, such as e-commerce sites or real-time data providers. By rotating proxies at regular intervals, Rayobyte ensures that the scraper can continue working without interruption.
Scheduling and Automation
Rayobyte’s Web Scraper API also supports scheduling and automation. With these features, users can set up recurring scraping tasks to run at specific intervals, such as hourly, daily, or weekly. This is especially useful for tasks like price monitoring, market analysis, or gathering updated product information from e-commerce sites.
For instance, if you want to scrape data every hour from a target website, you can set up an automated task that triggers the scraping process at the desired frequency, ensuring that you always have the latest data without manual intervention.
Rayobyte’s scheduling features can be set up via the API dashboard, where users can specify the frequency and time of scraping tasks, streamlining data collection and minimizing the need for constant oversight.
Error Handling and Retries
Web scraping is rarely a flawless process, and there are always potential errors like network issues, timeouts, or server-side restrictions that may cause scraping tasks to fail.
Rayobyte’s API includes automatic error handling and retry logic to minimize downtime. If a request fails due to a temporary issue (e.g., a timeout or rate-limiting), Rayobyte’s API can automatically retry the request based on predefined retry settings.
Fast, Effective, Efficient
Get the API that can meet your web scraping needs.

Try Rayobyte’s Web Scraper API Today
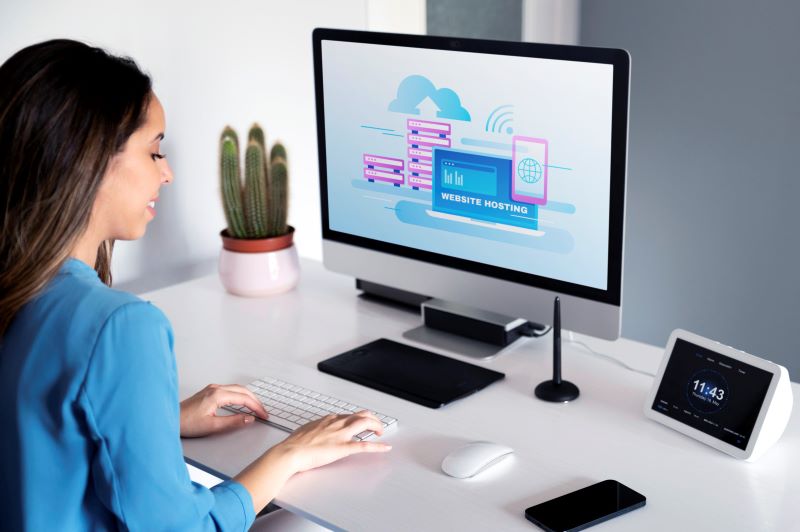
From e-commerce and finance to SEO and market research, web scraping helps businesses and developers collect data at scale efficiently. However, as beneficial as web scraping is, it often comes with challenges such as IP blocking, CAPTCHA bypassing, and handling dynamic content.
That’s where APIs like Rayobyte’s Web Scraper API shine. By offering an easy-to-use, scalable solution, Rayobyte eliminates many of the complexities of traditional web scraping, such as proxy rotation, handling CAPTCHAs, and automating scraping tasks.
If you’re ready to take your web scraping projects to the next level, try out Rayobyte’s Web Scraper API today. Sign up and start your free trial here!