JavaScript Guide: Load JSON Files from Your Computer
It is quite common to need to retrieve and store data when working with servers. That includes data from both external sources and from those sources. It must be stored in a usable, standard format that allows the data to be easily accessed when needed. It is necessary to have a standard format to retrieve data that is both accessible and readable. There are various options available, but JSON, or JavaScript Object Notation, is one of the most effective and useful solutions available. You can learn how JavaScript can load JSON file from desktop in a matter of just a few minutes.
JSON is very useful for several reasons. It is a simple structure. It is lightweight, which makes writing and reading data easier to do. Also, note that JSON can be parsed without a lot of difficulty and can be generated by automated systems, which drastically reduces the amount of time necessary for these tasks. The question is how to make this happen. When it comes to getting JavaScript to read JSON file, there are some important steps you need to consider.
How JSON Works

JSON is an automated system capable of parsing and generating JSON quickly and easily. It is used in various web applications to aid in transmitting data to servers and web applications. In order to do this, you may need a bit of help learning how to load a JSON file in JavaScript. This tutorial aims to help you with that process.
JSON is a way of organizing data in a script file using text. That is what makes it easy to store and share. Web applications must be able to read JSON files, whether they are on a server or stored locally. There are several methods to allow JSON files to be read in JavaScript. Here, we will discuss several of the most important options.
Before moving forward, it is helpful to understand data representation in JSON files. When you are using JSON, the data is represented in (key, value) pairs. This means that a unique key identifies each piece of information or data. Its value could be of various types. This may include arrays, objects, strings, numbers, Booleans, and null.
To help you see how data representation is created, consider this simple JSON file:
{
“products”: [
{
“id”: 1,
“name”: “Tablet”,
“price”: 400,
“description”: “A tablet computer with webcam”,
“stock”: 10
},
{
“id”: 2,
“name”: “Camera”,
“price”: 70,
“description”: “WiFi adapted camera”,
“stock”: 10
}
]
}
We will call this the product’s JSON file or a list of products on hand.
#1: Using Fetch API to Read JSON Files
To provide you with insight into how to do this, we will use the sample.json file as the following. You will need to change this to match the specific task you are performing.
{
“users”:[
{
“site”:”Rayobyte”,
“user”: “Reed”
}
]
}
The first method you can use to read a JSON file is the fetch method, also represented as (). This method is used to read local or uploaded JSON files. You will use the same syntax for both types of files. That syntax is:
fetch(‘JSONFilePath’).then().then().catch();
In order to use fetch () API to read JSON files, you will need to follow these steps.
- Create a JSON file.
- Add the data to that file.
- Open the JavaScript File.
- Use the fetch method described here to pass the path of the JSON file.
- Then, use the .son() method to parse the data within the file using a JSON format.
- Finally, you need to display the content.
So, how do you do this? To create a visual example, input the following code to use the fetch () method to read your JSON files:
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport”
content=”width=device-width, initial-scale=1.0″>
<title>Read JSON File</title>
</head>
<body>
<h1>Rayobyte</h1>
<h3>
Go to the console to see the
fetched data!!
</h3>
<script>
function fetchJSONData() {
fetch(“./sample.json”)
.then((res) => {
if (!res.ok) {
throw new Error
(`HTTP error! Status: ${res.status}`);
}
return res.json();
})
.then((data) =>
console.log(data))
.catch((error) =>
console.error(“Unable to fetch data:”, error));
}
fetchJSONData();
</script>
</body>
</html>
Here is another explanation of the same process using the fetch API to read JSON files. Fetch API is provided by modern browsers as a way to fetch resources from within the network. It can be used to fetch JSON files. When doing so, it will return a Promise. This can be resolved to a response object and used as required.
Promises are objects. They reflect the completion of an asynchronous operation and its result (and it can be a failure as well). When we use the fetch API to read JSON files, the response to the request will be resolved with a promise.
Let’s consider you fetch a products.json file. You will create a new JavaScript file. To do that, follow this code:
fetch(‘https://scraping-demo-api-json-server.vercel.app/products’)
.then(response => {
if (!response.ok) {
throw new Error(‘Network response was not ok ‘ + response.statusText);
}
return response.json();
})
.then(data => {
displayProducts(data);
})
.catch(error => {
console.error(‘There has been a problem with your fetch operation:’, error);
});
function displayProducts(products) {
products.forEach(product => {
console.log(`\x1b[32mProduct:\x1b[0m ${product.game_name}, \x1b[32mIn Stock:\x1b[0m ${product.inStock}`);
});
}
When you do this, the fetch API will go to work. The JavaScript function retrieves the JSON file from the URL you provided to it. In situations where this is successful, the tool will then parse the data into a JSON string and display it. In this example, it is displayed at displayProducts().
In this example, the function will log the name and the product availability for each of the items within the console, such as an inventory list.
The tool detects situations where there is an error for any component of the fetch process. It is then sent to the console.
The end result is a list of products, such as their name, whether they are in inventory, and how many are in inventory. You can learn more about reading JSON file nodes.
#2: Use the Required Module to Read JSON Files
The second option is a bit different in that it uses the required module to read JSON files. It is used to include modules within your application. You can also use it to include a file in a web application.
To do this, we use the following syntax:
require(JSONFilePath);
Knowing that, you will need to follow the specific steps here to use the required module method to read JSON files in JavaScript:
- Create a JSON file.
- Add the data to that file.
- Open the JavaScript File.
- Create a script.js next.
- Use the required method of the node to import the JSON file.
- Print the data on the console.
In order for this particular method to work properly, we will be using Node instead of running the program on the browser. This means you will need to have the latest version of the node updated and ready to use.
If you have the node installed and updated, you can then use the following code to execute and fetch the JSON data:
const sample = require(‘./sample.json’);
console.log(sample);
When you do that, you get the following output:
{ users: [ { site: ‘Rayobyte’, user: ‘Reed’ } ] }
Import the Module to Read JSON File
The third method to use is called the important statement. This method can be used to import and store JSON file elements in a variable in JavaScript.
To do that, you need to use the following syntax to achieve your goal:
import nameOfVariable from “JSONFilePath” assert {type: ‘json’};
Follow these steps:
- Create a JSON file.
- Add the data to that file.
- Open the JavaScript File.
- Create a script.js file.
- Import the JSON file.
Here is an example of reading a JSON file in JavaScript by importing it using the import statement:
In HTML:
<!– index.html –>
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=
“width=device-width, initial-scale=1.0”>
<title>Read JSON File</title>
</head>
<body>
<h1>Rayobyte</h1>
<h3>
Go to the console to see the
fetched data!!
</h3>
<script type=”module” src=”./script.js”></script>
</body>
</html>
In JavaScript:
// script.js
import user from “./sample.json” assert { type: ‘json’ };
console.log(user)
The required and import modules are a common method for reading JavaScript read JSON file functionality to import data. When you use the node.js, the require () function helps by providing functionalities to read and parse JSON files.
Keep in mind that in modern ES6 standards JavaScript, it is possible to import the JSON file directly. Here is another example to consider. Let’s say you have a JSON file that is a list of products. You name the file products.json. There are two syntaxes that are available to load this JSON file:
Using node.js:
// Node.js
const data = require(‘./products.json’);
console.log(data);
Alternatively, you can choose to use the require() function for the same process. It will look for your file in the same directory as the script and then reads the JSON file in JavaScript object format.
If you are using ES6: The content of your code would look like this:
// Browser with a module bundler (e.g., Webpack) or modern Node.js
import data from ‘./products.json’ with {type: “json”};
console.log(data);
This process has helped you to learn how to read JSON files in JavaScript. This is a common and necessary task for most web developers who are using JSON files. It is commonly used to store user data, pick up static data, and configure data.
An alternative option is to use the file reader API. A FileReader API is a tool to read local JSON files on your desktop. You can use this to learn how to use JavaScript to load JSON files from your desktop. The application asynchronously reads the file’s contents and then extracts the data from it.
Here is an example of the code that will show how a FireReader API works to read the local file on your desktop when you upload it.
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″ />
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″ />
<title>Read JSON File</title>
</head>
<body>
<input type=”file” id=”fileInput” accept=”.json” />
<div id=”productList”></div>
<script>
document
.getElementById(“fileInput”).addEventListener(“change”, function (event) {
const file = event.target.files[0];
if (file) {
const reader = new FileReader();
reader.onload = function (e) {
try {
const data = JSON.parse(e.target.result);
displayProducts(data.products);
} catch (error) {
console.error(“Error parsing JSON:”, error);
}
};
reader.readAsText(file);
}
});
function displayProducts(products) {
const productList = document.getElementById(“productList”);
productList.innerHTML = “”;
products.forEach((product) => {
const productDiv = document.createElement(“div”);
productDiv.innerHTML = `Product: ${product.name}, Price: $${product.price}`;
productList.appendChild(productDiv);
});
}
</script>
</body>
</html>
This code sample generates an HTML file input element. It then adds an event listener to it.
Whenever you select a file, the FileReader API will read the content within that file as text. Once it reads it, it will then parse that data as JSON. In this example, the displayProducts() method is used to parse the desired data in this JS reading JSON file method. In this case, the name and the price of the products are logged to the site, and the function is iterated through the product array.
Why You Would Use JavaScript Loading JSON File Methods
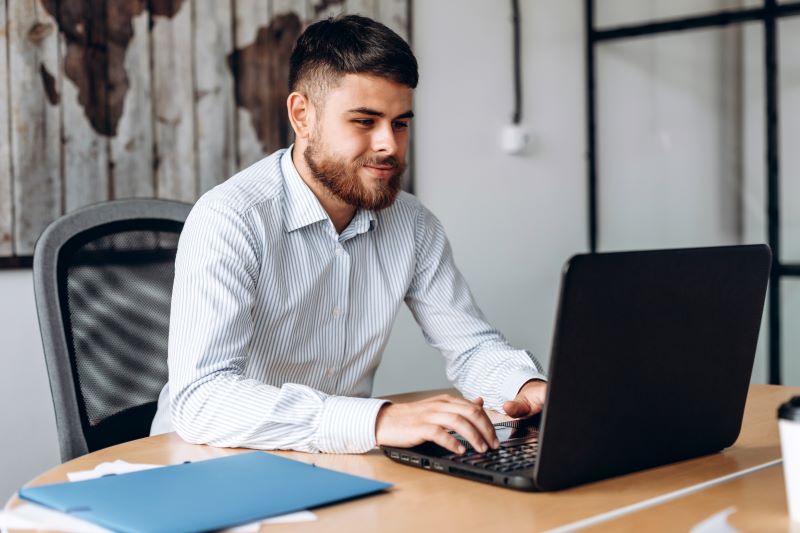
As described, there are various ways of loading JSON files in JavaScript, and for the most part, you can select what is right for you. Why would you use NodeJS to read JSON files or other methods? There are some excellent reasons why you may wish to do this. Here are some examples.
Web scraping: JS reading JSON files is a common task when it comes to web scraping. Web scraping is a process in which you want to pull data from a website to use for various reasons, such as monitoring inventory or pricing. JSON is a very common method for extracting structured data from web pages. The work is done by scrapers, and then it is parsed into JSON format. It can then be used for dozens of tasks, including monitoring changes on websites, data analysis for decision-making, or even the creation of a database.
There are various reasons why you may load JSON files in JavaScript for web scraping. First, it is lightweight, and that makes it easier to use and navigate. Also note that it is a very organized method, which is a great resource when you want an efficient way to manage scraped data. This is one of the most important resources and reasons to use this type of tool.
Configuring files: Another reason why you may wish to learn how to read JSON files from a desktop is for configuration files. It is quite common to use JSON for configuration files. This is done within both applications and servers and works very well for various reasons.
Configuration files typically have specific settings and parameters. Those will play a role in the way the software behaves and operates. With the lightweight structure and organization of JSON, it tends to be an excellent choice for this type of application, and it is often used. The readability of the JSON file makes it a good choice overall for handling any of these settings and configuration needs.
APIs and Data Exchanges: Another way that you may use Read JSON file Node methods like these is for data exchange and APIs. It is possible for web servers and clients to exchange data in standard JSON. That is not as common as some of the other tasks listed here, but they can be done easily enough.
APIs often use JSON to transmit and receive data. This is because of its organization and overall functionality. Using JSON helps to make the process easier overall and makes web integration of various systems and services more streamlined and efficient.
Data Storage and Retrieval: Another reason why you may be using JSON is for data storage and retrieval needs. This can be done in file systems and databases. JSON is often used to both store and retrieve data in these databases.
You will also be able to use JSON-like formats, such as BSON. These are often used by NoSQL databases as an effective way to store data, including documents. It provides a hierarchical data representation that is easy to use and provides good data representation overall.
Beware of the Most Common Errors When You Load JSON File in JavaScript
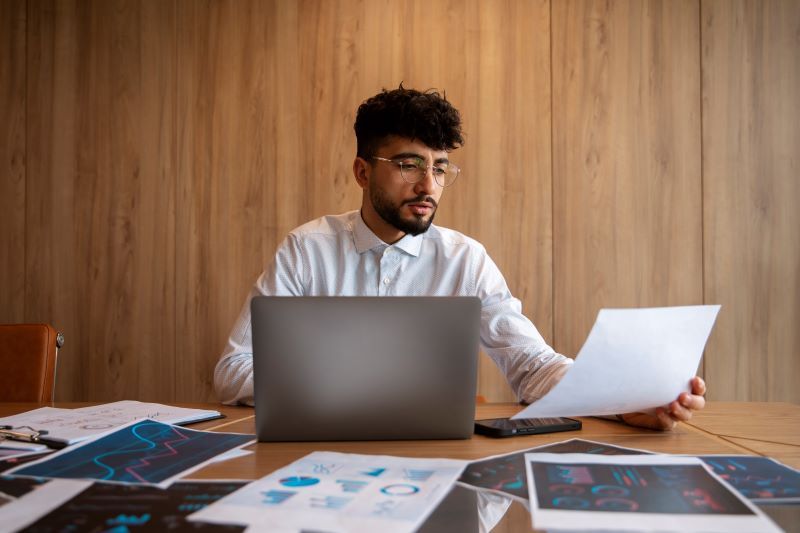
There are plenty of mistakes that can be made in any component of these processes, but there are several specific errors that most commonly are the problem when your code fails to operate in the way you deserve. While numerous errors are possible, the following are some of the most common that we see in this process:
- Parsing mistakes: Parsing is such an incredibly important process, but it is very common for errors to arise in this area. If you have an invalid JSON format, you could end up with a JSON.parse() error. That is the most common reason for invalid formats. JSON data needs to be validated before you use it to make sure this does not happen.
- Security issues: Another component that can take down the process and limit your overall success is security. Unfortunately, this is a growing problem across various areas, and it is something that can be hard to manage. Specifically, look for attacks that use JSON injection. The only way to minimize these risks, then, is to handle data in a safe manner and to make sure you sanitize all inputs. It may take a bit more time, but it is worth doing so.
- CORS errors: Perhaps the most common error is cross-origin resource sharing or CORS error. This can happen when you are retrieving JSON from different origins. The only way to minimize this risk is to ensure the server contains the necessary headers to permit requests from different origins.
There are a few more things you should know and consider regarding JavaScript load for JSON files from desktop applications.
First, when it comes to performance, there are various ways to improve it. If you are working with a very large JSON file, you may want to alter the way you send it. For example, instead of loading the entire file into the memory, which can be resource-heavy and hard to navigate, the better option could be to use streaming parsers. There are various options available, including Node.js JSONStream. This will allow for the data to be managed in portions instead, which can help to improve the overall speed and functionality of the process.
Consider XML as well. Another consideration is why JSON is often used over other solutions, including XML, for data exchanges. JSON is a more streamlined and condensed format and structure. That is why it is often used over XML when it comes to interchanging data. Often, the key-value pairs and the arrays are more concise in JSON than they are in XML. That makes it easier to avoid some of the more challenging aspects of XML.
Utilizing the Resources of Rayobyte to Improve Your Outcome
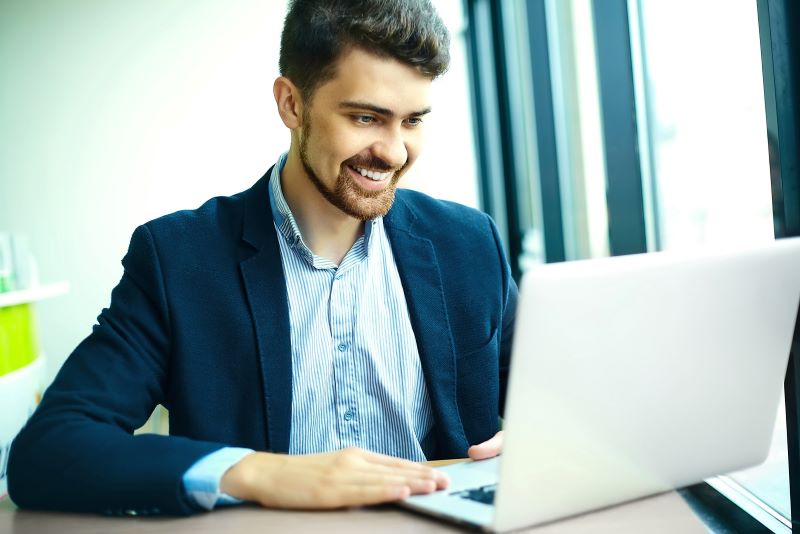
When it comes to using JavaScript to load JSON files from desktop applications, the process can be a bit overwhelming. When it comes to web scraping, it has to go well. For that reason, it is often helpful to develop strategies that can help you get around the blocks and anti-bot tools out there.
One of the most effective ways to do that is through Rayobyte. We encourage you to take a closer look at our proxies and overall tools that can help improve the way you navigate web scraping, saving you time and money and making sure you have more of the information and resources you need. Contact us with any questions you have.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.