How to Web Scrape in Python
Once you learn how to scrape a website with Python, you will find that this process is one of the best routes to gathering data, analyzing that data, and turning it into something valuable. Web scraping is the process of capturing data on other websites to use for your own needs – scraping that website’s information so that you can analyze the details. It is done with a web scraping tool or web scraper, which can be built using Python, one of the most prolific and effective types of computer languages used in design today.
Python offers numerous benefits over other languages. It is robust, highly effective in terms of what it can do, and it is easier to learn than most others. Python is an object-oriented language, which helps to make the process easier to learn than others. Even better, though, you can learn how to scrape a website with Python with all of the libraries that simplify the process even further.
Try Our Web Scraping API
Proxy rotations, CAPTCHA bypass and more.

To help you learn how to scrape a website with Python, we have put together a comprehensive guide. The following tutorial is meant to provide you with the insights you need to learn how to scrape a website in Python as a basic starting point. You’ll find many other resources on our website to help you fully complete this process. Our Advanced Web Scraping in Python is another tutorial that can take your experience to the next level.
If you have not done so yet, read our Introduction to Web Scraping. It will help you understand web scraping and how it works, and it also breaks down what you can use it for!
Get the Tools You Need to Learn How to Webscrape in Python
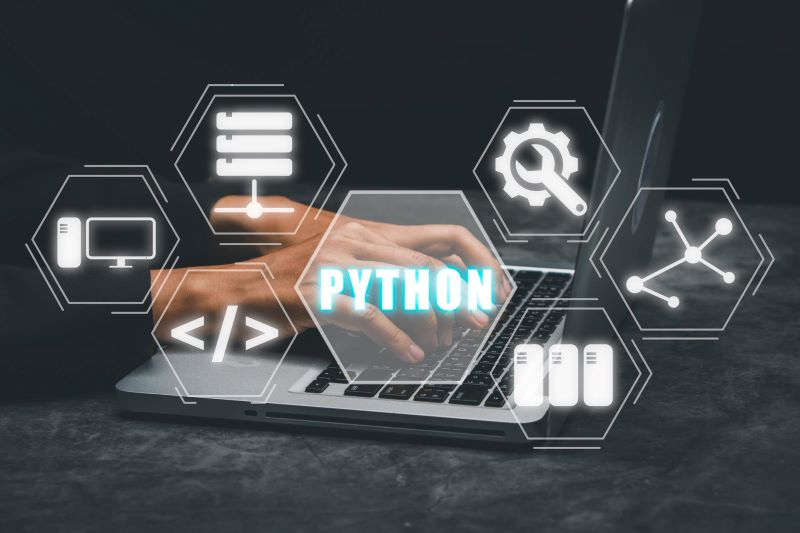
In order to use Python, you need to have the right tools and libraries available to you. Here are some of the specific elements you will need to do this.
You will need to use Python 3.4+, and if you are using a Windows device, you need to check “PATH installation.” This ensures you will have an executable tool to rely on.
Next, you need to have the necessary libraries to help you with the entire process. This is one of the best reasons to use Python for web scraping. You will find most of the details you need on each one of these libraries on our blog. If you are not sure how to scrape a website using Python because you have not learned about these libraries yet, head over to these tutorials to learn more.
Requests
Requests will provide you with the ability to send a request for content to the website you are targeting. Sending HTTP requests, including POST and GET, to a website’s server tells that server what you want from it. It will ultimately return the information you are requesting – that’s the data you will use later.
The Requests library is one of the best ways to use Python for this process because it is easier to use. In fact, it reduces the number of lines of code you need to write, and that reduces the risk of errors of all types. You can get the Requests library by inputting this into the pip command:
python -m pip install requests
This makes it easy for you to send HTTP GET and POST requests. Here is an example of a get() request.
import requests response = requests.get('https://Rayobyte.com/') print(response.text)
In addition to this, you can use proxies as a part of this process (something we recommend doing as you learn how to web scrape in Python). However, proxies often need to be authenticated. You can use the Requests commands to help you move past that as well. Here is a sample to help you with this process. Just input the information as it applies to your need:
proxies={ 'http': 'http://USERNAME:[email protected]:7777', 'https': 'http://USERNAME:[email protected]:7777', } response = requests.get('https://ip.rayobyte.com/location', proxies=proxies) print(response.text)
Beautiful Soup
The next library we need to access is Beautiful Soup, an important parsing tool that can extract data from HTML. Another nice feature is that it will turn invalid markup data into a parse tree, which could be helpful as you learn how to scrape a website with Python.
In most situations, you will need to use Beautiful Soup along with the Requests library. During this process, you will need to have access to a parser. You could use the HTML parser module, another component of the Python library.
To get these details, follow this process:
pip install beautifulsoup4
Now that you have Beautiful Soup, you can then start the process. Create the same get request as listed above. Then, find the element you desire using the following code:
import requests from bs4 import BeautifulSoup url = 'https://rayobyte.com/blog' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') print(soup.title)
When you take those steps, it should provide you with the following:
<title>Rayobyte Blog | Rayobyte</title>
As you dive deeper into learning how to scrape data from a website in Python, you will be able to go further with this by tapping into the various developer tools available.
LXML
Are you ready for the next part, which is to find out how to scrape a website using Python? We need LXML. It is a very fast and powerful library purposefully built for parsing. You can use it with both HTML and XML files. This is important if you are doing any type of web scraping with large amounts of data.
You can download this library at the following:
pip install lxml
Here is an important note. This library has the HTML module in it. That means it will work with HTML. But, the LXML library still needs the HTML string first. You can obtain that using the requests library, as noted above. Once you have the HTML, you can then build a tree using the following fromString code:
import requests from lxml import html url = 'https://rayobyte.com/blog' response = requests.get(url) tree = html.fromstring(response.text)
With this, we can now use XPath to find the information you desire. You can dive into more details on how to use LXML as well, as it can do much more for you over time.
Selenium
The next tool we need to explore in this web scraping Python tutorial is Selenium. Most of the websites you visit each day are built with JavaScript. This specific language allows the developer to create dynamic content. That means that they can create components of the website that require input and data. Think about those sign-in forms you encounter every time you visit a site. Python gets hung up on these areas, and that is why, to learn how to web scrape in Python, you need a tool that can help you overcome the limitations associated with dynamic content.
Selenium is an important tool for web scraping overall. Selenium helps you to get around the wide range of limitations on the web – including CAPTCHAs and forms. Many websites have these interactive tools and features, called dynamic content, which can make it difficult for the web scraper to capture information. Selenium provides an opportunity to get around this.
Websites that use JavaScript are common. If you plan to scrape these sites, you need to use Selenium web scraping to help you. Selenium has three components:
- You will need to use a web browser such as Chrome, Firefox, Safari, or Edge.
- You will need a driver for that browser, which you will already have if you have Selenium 4.6 or higher.
- The Selenium package itself.
You can get at:
pip install selenium
You will then need to obtain the driver for the specific browser you plan to use. Most commonly, web scraping is done in Chrome. To help you install the necessary Chrome driver, you will need to use this code:
from selenium import webdriver from selenium.webdriver.common.by import By driver = webdriver.Chrome() Now you have the ability to complete the next step of the Python tutorial for web scraping using the following using the get() method: driver.get('https://rayobytel.com/blog')
Scrapy
Now that you have done all of this work to learn web scraping with Python, there are just a few more steps to consider in the process. The next step is to install Scrapy. The Scrapy framework has a Python scraping and crawling package. This package includes a lot of the tools you need, including request handling, parsing responses, and data pipelines, and it allows you to do all of this without having to learn a lot of code to do so.
Scrapy also works very well with other Python libraries, including those that we have included here as a starting point. When you incorporate Scrapy, you get more data processing pipelines and automation workflows.
There are several reasons why you will want to incorporate Scrapy, including the fact that it is faster and capable of handling numerous HTTP requests at the same time. When it comes to learning how to scrape data with Python, if you have a significant amount of data to scrape, this can become a very powerful tool for you.
Scrapy offers a lot of key benefits, including the use of proxies, which can help you minimize data risks, protect your privacy, and provide better access to sensitive information. With Scrapy, you are able to export your data, too, including through XML, CSV, or JSON formats.
We encourage you to check out our Web Scraping with Scrapy tutorial to learn how to use Scrapy completely for web scraping.
Comprehensive Guide to Web Scraping in Python
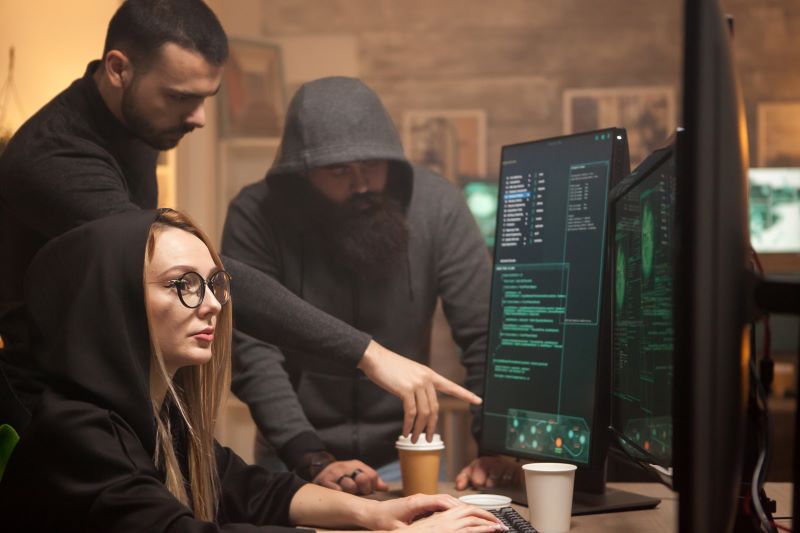
Now that we have put all of the individual tools together, it is time to consider the next steps in the process – bringing it all together to complete your project.
Static Page Script
If you are learning how to webscrape with Python to capture data that is on a static page – meaning it is not dynamic content, you can use the following code to get started.
Try Our Web Scraping API
Proxy rotations, CAPTCHA bypass and more.

Now, for this, we are looking at a jobs page on a website, and we want to capture information about those jobs.
<div class="card"> <div class="card-content"> <div class="media"> <div class="media-left"> <figure class="image is-48x48"> <img src="https://files.rayobyte.com/media/real-python-logo-thumbnail.7f0db70c2ed2.jpg" alt="Real Python Logo" /> </figure> </div> <div class="media-content"> <h2 class="title is-5">Sen Python Developer</h2> <h3 class="subtitle is-6 company">Payne, Roberts and Davis</h3> </div> </div> <div class="content"> <p class="location">Stewartbury, AA</p> <p class="is-small has-text-grey"> <time datetime="2021-04-08">2021-04-08</time> </p> </div> <footer class="card-footer"> <a href="https://www.rayobyte.com" target="_blank" class="card-footer-item" >Learn</a > <a href="https://rayobyte.github.io/job-listings/jobs/senior-python-developer-0.html" target="_blank" class="card-footer-item" >Apply</a > </footer> </div> </div>
You can alter these details to match the website that you are capturing. The key to remember is that this code is meant to provide you with details of how the process works – but you need to personalize it to ensure it operates in the way you desire.
Web Drivers and Browsers
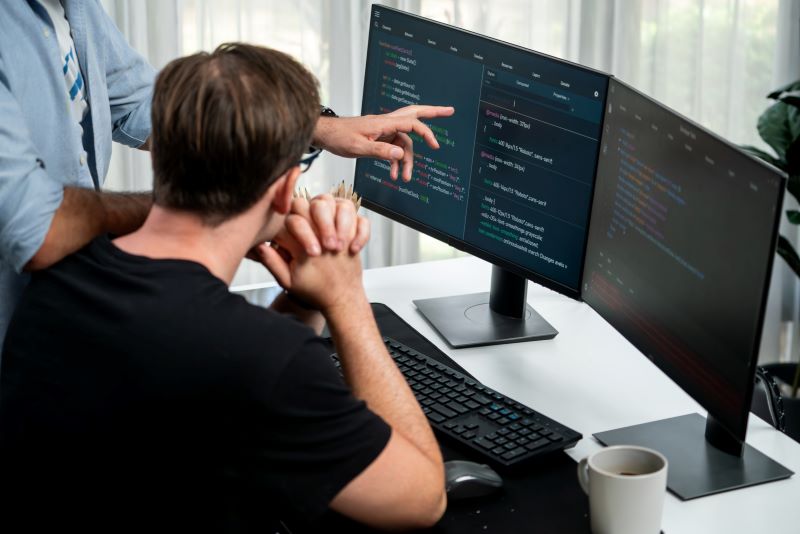
Another component of the process of learning how to do web scraping using Python is understanding web drivers and browsers a bit. Every type of web scraper will use a browser to connect to the URL you desire. Most of the time, you will want to use a traditional browser, as noted previously. This can offer a number of benefits, especially for those who are newer to coding. It also makes it easier for you to debug and troubleshoot your information.
As your skill improves and your challenges with web scraping become more detailed, it becomes necessary to tackle bigger tasks. For that, a headless browser would be helpful. If you are at that point, we encourage you to read our guide on headless browsers. It will provide you with more insight into how you can use it for more complex web scraping solutions. As noted, we will use Chrome for this demonstration.
Coding Environment
The coding environment you use matters. You can use a simple text editor, which is typically the ideal choice for most people. However, there are benefits to creating a .py file that works well. Over time, you will be able to increase your skills and consider the use of an integrated development environment.
Choosing the Right URL
Another step in the process is to choose the URL for your project – the URL is the destination from which you want the scraper to grab information.
In most cases, you need the exact URL, and for that, we recommend the use of one that does not have any components of JavaScript in it (remember, dynamic content can be difficult to navigate at first). Scraping data with JavaScript requires a higher level of support.
Defining Objects within Your Code
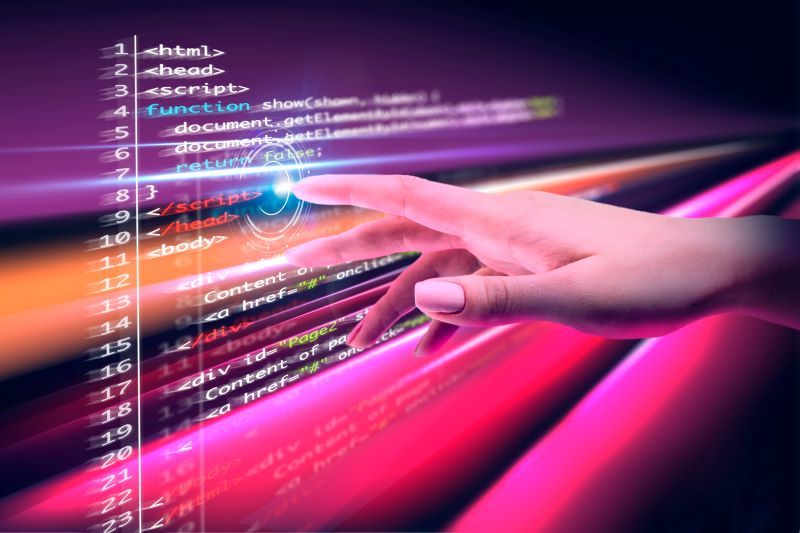
Python will provide you with the ability to design objects without having to assign a specific type. You can actually create an object using Python by just putting in some basic information, such as the title, and then assigning a value to it.
# Object is "results", brackets make the object an empty list. # We will be storing our data here. results = []
Lists are another important component of this process. In Python, the list is ordered and mutable. It is also important to note that they are duplicated members. It is important to know how to use lists since they are such a common component of the process of web scraping.
Here is an example of how to use this:
# Add the page source to the variable `content`. content = driver.page_source # Load the contents of the page, its source, into BeautifulSoup # class, which analyzes the HTML as a nested data structure and allows to select # its elements by using various selectors. soup = BeautifulSoup(content, 'html.parser')
Now that you have completed all of these steps for this web scraping in Python tutorial, you may be ready to get to work. You certainly can do so. The next part of the process is to extract data from the HTML file so that you can use it.
During this process, focus on smaller sections of the big file. Specifically, you can take small sections of different parts of a page with web scraping, and you will need to store that data in a list. To do this, it may be beneficial to process smaller sections and then add them to the list.
Once you complete these steps, you will then be ready to explore the data to a CSV file, and before doing that, be sure you check to ensure there are no errors in the process.
How Rayobyte Can Help You with Your Project
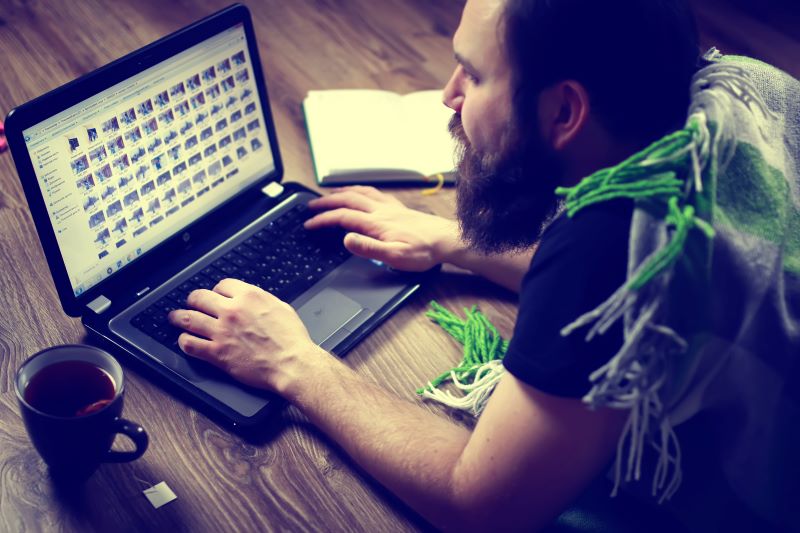
There are many more intricate steps to learning how to web scraping Python, and that means there are many more ways to customize the process and make this result one that fits your needs specifically.
At Rayobyte, you have all of the tools and resources you need to learn how to web scrape in Python with confidence. We also provide you with access to the proxies you need to do so safely. Take the time to check out how Rayobyte is supporting the use of highly effective web scraping tasks.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.