How to Run Perl Scripts: A Simple Guide
Perl, a high-level programming language created by Larry Wall in 1987, has long been a favorite among developers for its versatility and power. Over the years, it has evolved significantly, with a rich ecosystem of modules and libraries that enhance its functionality, making it a valuable tool in the programmer’s toolkit. It’s no wonder so many people want to know how to run Perl scripts.
In this guide, we’ll walk you through the essential steps to run a Perl script effectively. Whether you’re looking to automate a mundane task, analyze data, or dive deeper into programming, understanding how to execute Perl scripts will be a valuable skill in your repertoire.
Setting Up Your Environment
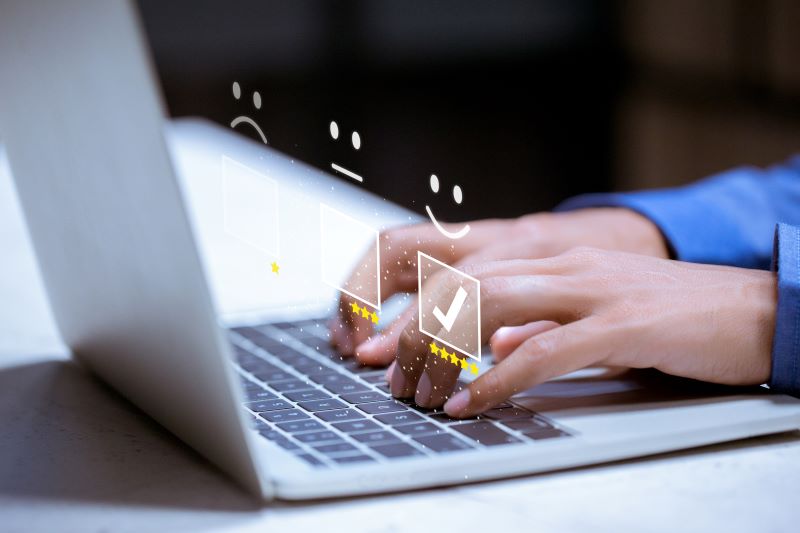
One of the first questions you might be asking is, “How do I run a Perl program?” Before diving into the answer, it’s essential to set up your environment correctly.
The first step is installing Perl on your machine, which varies slightly depending on your operating system.
Installing Perl
The process you use for installing Perl depends on your device:
- Windows: The easiest way to install Perl on Windows is through the Strawberry Perl distribution, which includes everything you need. Simply download it from Strawberry Perl’s website and follow the installation prompts. For a more robust installation, consider using ActivePerl from ActiveState.
- macOS: Perl comes pre-installed on macOS. To check your version, open Terminal, and type perl -v. If you need the latest version, consider using a package manager like Homebrew. Install Homebrew and then run Brew install Perl to get the latest version.
- Linux: Most Linux distributions come with Perl pre-installed. You can check by typing perl -v in your terminal. If you need to install or upgrade, use your package manager (e.g., sudo apt-get install perl for Ubuntu).
For detailed instructions and troubleshooting, refer to the official Perl documentation.
Choosing a Text Editor
Once Perl is installed, the next step is to select a text editor for writing your scripts. Popular choices include:
- Visual Studio Code: A versatile editor with extensive extensions for Perl.
- Sublime Text: Known for its speed and simplicity, with great syntax highlighting for Perl.
- Atom: A hackable text editor that allows for significant customization.
For a more integrated experience, consider using an IDE like Padre, a Perl IDE explicitly designed for Perl development, or Komodo IDE, which supports multiple languages, including Perl.
With your environment set up, you’re ready to write and run Perl script!
Writing Your First Perl Script
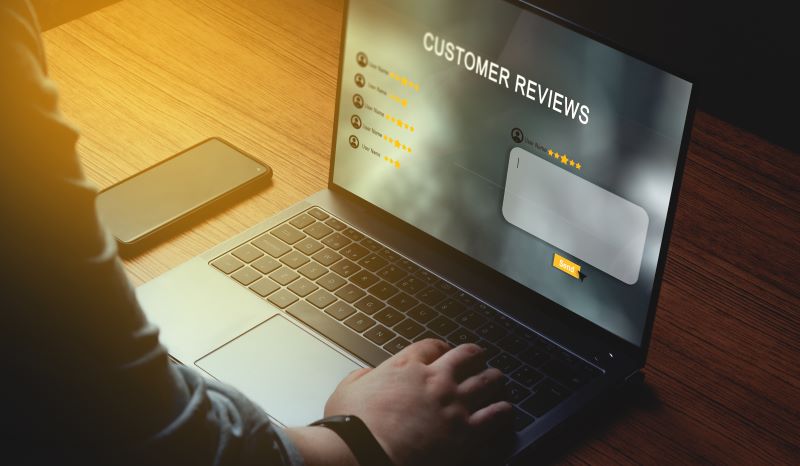
Now that your environment is set up, it’s time to learn how to execute a Perl program! Perl’s syntax is designed to be straightforward, making it accessible for newcomers while still powerful enough for seasoned developers. Let’s explore the basic structure of a Perl script and walk through an example.
Basic Structure of a Perl Script
A typical Perl script consists of the following components:
- Shebang Line: This is the first line of the script, which tells the system where to find the Perl interpreter. It usually looks like this: #!/usr/bin/perl. This line is essential for running the script directly from the command line.
- Comments: Comments in Perl start with the # symbol and continue to the end of the line. They are used to annotate your code and improve readability without affecting the script’s execution.
- Code Blocks: Perl scripts consist of statements that perform various tasks. These can include variable declarations, control structures (like loops and conditionals), and function definitions.
Example Script: “Hello, World!”
Below, you’ll learn how to execute a Perl script that prints “Hello, World!” to the screen. Here’s the complete code:
#!/usr/bin/perl # This is a simple Perl script to print "Hello, World!" use strict; # Enables strict mode use warnings; # Enables warnings print "Hello, World!\n"; # Print statement with newline
Explanation of Each Line of Code
Here’s a quick breakdown of each line:
Shebang Line
#!/usr/bin/perl
This line specifies the path to the Perl interpreter. When you run the script from the command line, the operating system uses this line to determine which interpreter to execute. If you have installed Perl in a different location, you may need to adjust this path accordingly.
Comment
# This is a simple Perl script to print “Hello, World!”
This comment explains the purpose of the script. Comments are invaluable for others (and your future self) to understand your code’s intent.
Use Statements
use strict;
use warnings;
These statements enable strict mode and warnings in your script. Strict mode enforces good programming practices by requiring you to declare variables before using them, helping prevent common mistakes. Warnings provide helpful alerts about potential issues in your code, making debugging easier.
Print Statement
print “Hello, World!\n”;
This line is where the action happens! The print function outputs text to the screen. The string “Hello, World!\n” is the message we want to display. The \n at the end is a special character representing a new line, ensuring that the cursor moves to the next line after printing.
Running the Script
To run this script, follow these steps:
- Open your preferred text editor and copy the code above into a new file. Save the file with a .pl extension, for example, hello_world.pl.
- Open your terminal or command prompt.
- Navigate to the directory where you saved the script.
Make the script executable (on Unix-based systems) by running:
chmod +x hello_world.pl
Finally, execute the script by typing:
./hello_world.pl
You should see the output: Hello, World! Congratulations! You’ve just written and executed your first Perl script. This simple exercise sets the foundation for more complex scripting and programming tasks. As you continue to explore Perl, you’ll discover its rich features and powerful capabilities.
Running Perl Scripts
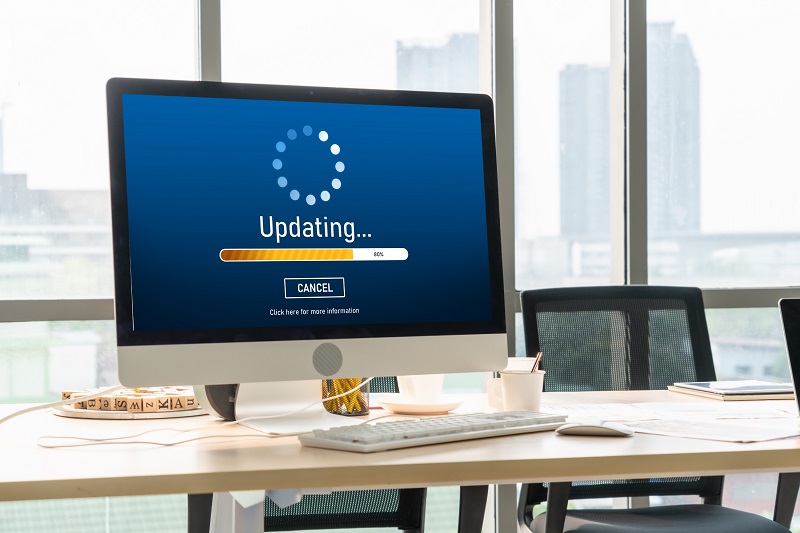
Once you’ve written your Perl script, the next step is executing it. There are various methods to run Perl scripts, with the command line and Integrated Development Environments (IDEs) being the most common. In this section, we’ll explore both methods, along with troubleshooting tips for common issues.
Using the Command Line
Executing a Perl script from the command line is straightforward. Here’s a step-by-step guide:
Open the Terminal or Command Prompt
- On Windows, search for “Command Prompt” or “PowerShell.”
- On macOS, use “Terminal,” found in Applications > Utilities.
- On Linux, open your preferred terminal emulator.
Navigate to Your Script’s Directory
Use the cd command (change directory) to navigate to the folder containing your Perl script. For example:
cd path/to/your/script
Run the Perl Script
To execute your script, type the following command:
perl your_script.pl
Replace your_script.pl with the name of your actual script. If you’ve included the shebang line (#!/usr/bin/perl), you can also make the script executable and run it directly:
chmod +x your_script.pl
./your_script.pl
Common Command-Line Flags and Options:
- -w: Enables warnings for potential issues in your code.
- -c: Checks the syntax of the script without executing it.
- -d: Runs the script in debugging mode, allowing you to step through the code.
Using an IDE
Many developers prefer using an IDE for writing and running Perl scripts due to its integrated features. Here’s how to run Perl scripts in some popular IDEs:
Visual Studio Code (VS Code)
- Install the Perl extension from the marketplace.
- Open your Perl script and run it by right-clicking in the editor and selecting “Run Perl Script” or by using the integrated terminal.
Padre
- Open your script within Padre.
- Click on the “Run” button or use the shortcut (usually F5) to execute the script. The output will appear in the output pane.
Komodo IDE
- Open your script and click on the “Run” button on the toolbar.
- You can configure run configurations for different Perl environments if needed.
Troubleshooting Common Errors
Errors are a natural part of programming, and understanding common Perl error messages can significantly improve your debugging skills.
Common Error Messages
- “Can’t locate module…”: This means Perl cannot find the specified module. Ensure the module is installed and the @INC paths are correctly set.
- “Syntax error at line X”: Indicates an issue with the syntax at the specified line. Check for missing semicolons, mismatched parentheses, or unclosed quotes.
- “Use of uninitialized value…”: This warning suggests you’re trying to use a variable that hasn’t been defined yet. Ensure all variables are initialized before use.
Tips for Debugging Perl Scripts
- Use Warnings and Strict Mode: Always include use warnings; and use strict; at the top of your scripts. These help catch common mistakes and enforce good coding practices.
- Print Debugging: Insert print statements throughout your code to display variable values and program flow, helping you identify where things go wrong.
- Perl Debugger: Use the built-in Perl debugger by running your script with the -d flag. This allows you to step through your code interactively.
By mastering the execution of Perl scripts, whether through the command line or an IDE, and understanding how to troubleshoot common issues, you’ll be well-equipped to tackle more complex scripting tasks. Happy coding!
Understanding Perl Modules and Libraries
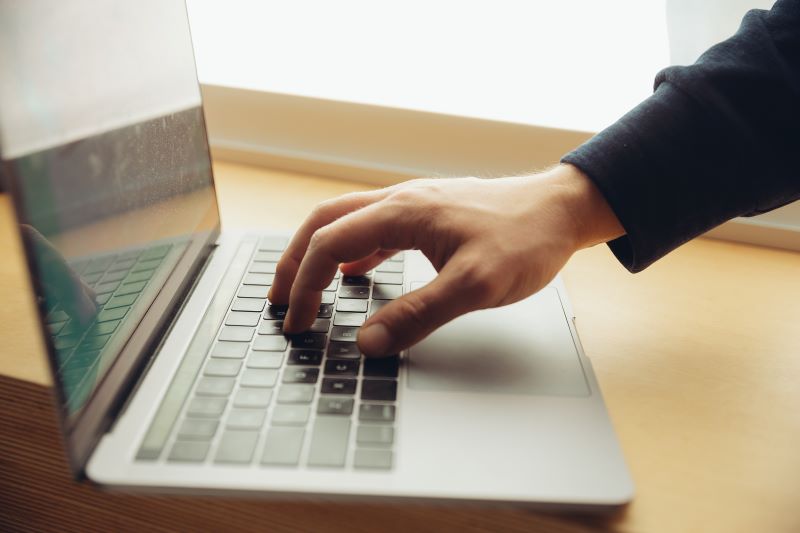
Perl is renowned for its rich ecosystem of modules and libraries that enhance its capabilities and simplify complex tasks. Understanding how to leverage these modules can significantly boost your productivity as a Perl developer and help you learn to launch Perl script effectively.
What are Modules and Why They’re Useful?
In Perl, a module is a reusable package of code that contains functions, variables, and other resources. Modules allow developers to organize code into manageable components, promoting reusability and modular design. Instead of writing everything from scratch, you can utilize existing modules to save time and effort.
Modules are beneficial for several reasons:
- Code Reusability: By using modules, you can avoid duplicating code across different scripts. If a particular functionality has been implemented in a module, you can simply include that module in your script, which helps in maintaining consistency and reducing errors.
- Community Contributions: Perl has a vibrant community that actively contributes to its ecosystem. Many developers have shared their modules through the Comprehensive Perl Archive Network (CPAN), which hosts thousands of modules covering a wide array of functionalities—from database interaction to web development.
- Simplified Development: Modules can simplify complex tasks. For instance, instead of writing intricate code for handling regular expressions or web requests, you can utilize specialized modules that encapsulate this functionality.
Installing and Using CPAN
The Comprehensive Perl Archive Network (CPAN) is a central repository where you can find, install, and manage Perl modules. Here’s how to get started with CPAN:
Installing CPAN
Most modern Perl installations come with CPAN pre-installed. To check if CPAN is available, open your terminal and type:
cpan
If it’s not installed, you can set it up by following the instructions in the Perl documentation or using your package manager (e.g., sudo apt-get install cpanminus on Debian-based systems).
Using CPAN
Once you have access to CPAN, you can install modules easily. Start by launching the CPAN shell:
cpan
To install a module, use the command:
install Module::Name
For example, to install the popular LWP::UserAgent module, you would type:
install LWP::UserAgent
To exit the CPAN shell, simply type:
exit
Using CPAN Minus
Alternatively, you can use cpanm, a simpler and faster interface for installing modules:
cpanm Module::Name
Example of Using a Popular Perl Module
One of the most commonly used Perl modules is LWP::UserAgent, which allows you to send HTTP requests and interact with web services. Here’s a simple example demonstrating how to use it to fetch the content of a webpage.
Install the Module (if you haven’t already)
cpanm LWP::UserAgent
Create a Script
Here’s a sample script that uses LWP::UserAgent to fetch and print the content of a webpage:
#!/usr/bin/perl
use strict;
use warnings;
use LWP::UserAgent;
my $url = ‘http://www.example.com’; # URL to fetch
my $ua = LWP::UserAgent->new; # Create a new UserAgent object
my $response = $ua->get($url); # Send a GET request
if ($response->is_success) {
print $response->decoded_content; # Print the content of the response
} else {
die “Error fetching $url: ” . $response->status_line;
}
Run the Script
Save the script to a file, for example, fetch_example.pl, and execute it from the command line:
perl fetch_example.pl
If the script runs successfully, it will output the content of the specified webpage.
By understanding how to utilize Perl modules and libraries, along with tools like CPAN, you can greatly enhance your scripting capabilities and streamline your development process. This modular approach not only saves time but also allows you to build robust applications efficiently.
Practical Applications of Perl Scripts
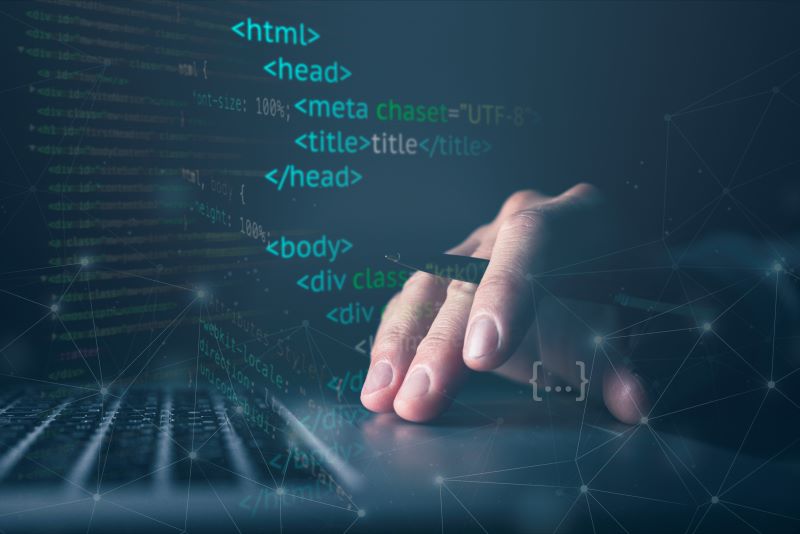
Perl is a versatile scripting language that excels in various domains, making it a powerful tool for developers and system administrators alike. Its strengths in text manipulation, data handling, and system interaction lend themselves to a wide array of practical applications. Here, we explore some of the most common use cases for Perl scripts in web development, system administration, data analysis, and automation.
Web Development
Perl has been a longstanding player in web development, particularly with the Common Gateway Interface (CGI). Although many developers now favor frameworks in other languages, Perl remains valuable for web applications, especially when leveraging frameworks like Dancer or Mojolicious. Perl scripts can handle HTTP requests, manage sessions, and interact with databases, making them suitable for building dynamic websites.
For instance, a Perl script can be used to create a simple web form that collects user input. The script processes the input and stores it in a database or sends an email. This functionality is invaluable for creating interactive websites, such as content management systems (CMS) or customer relationship management (CRM) tools.
System Administration
Perl is a powerful ally for system administrators. Its robust text processing capabilities make it ideal for writing scripts that automate routine tasks, such as log file analysis, user account management, and system monitoring. For example, a Perl script can parse log files to extract meaningful information, such as error messages or usage statistics. By automating this process, administrators can quickly identify issues and take corrective actions.
Additionally, Perl can be used to manage configuration files and automate system updates. With its ability to read and write files easily, system administrators can create scripts that update system settings or install necessary packages, streamlining operations and improving efficiency.
Data Analysis
In the era of big data, Perl’s ability to handle large datasets and perform complex data manipulations makes it a valuable tool for data analysis. Its built-in support for regular expressions allows for sophisticated pattern matching and text parsing, which are essential for cleaning and transforming data.
For example, a data analyst might use Perl to process CSV files, filter out irrelevant information, and aggregate data for reporting purposes. The script could read the file, apply transformations, and output the cleaned data into a new file or directly into a database. Additionally, Perl’s integration with databases through modules like DBI allows for seamless data retrieval and manipulation.
Automation
One of the most compelling use cases for Perl scripts is automation. Whether it’s automating backups, sending notifications, or generating reports, Perl can significantly reduce manual effort and increase productivity. For instance, a Perl script can be scheduled to run at regular intervals (using cron jobs on Unix-like systems) to back up important directories to a remote server.
Another common automation task is monitoring system performance. A Perl script can periodically check system metrics, such as CPU usage or disk space, and send alerts via email if certain thresholds are exceeded. This proactive approach helps system administrators maintain optimal performance and address potential issues before they escalate.
Best Practices for Writing Perl Scripts
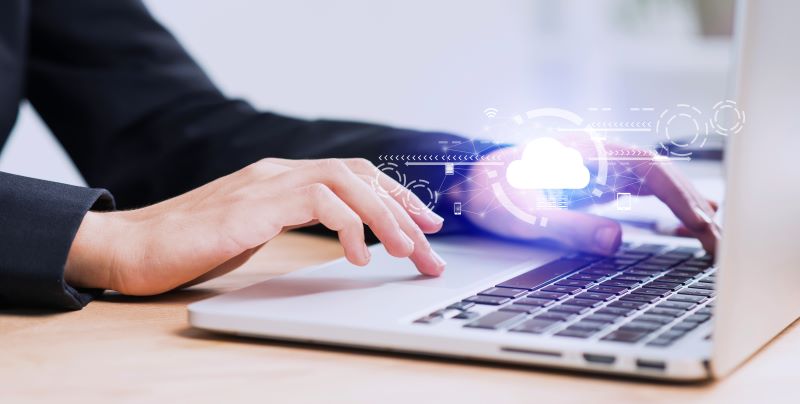
Writing effective Perl scripts requires adherence to certain best practices that enhance code quality, maintainability, and readability. By following these guidelines, you can create scripts that are easier to understand, debug, and share with others.
Coding Standards and Readability
Adopting a consistent coding style is crucial for improving the readability of your Perl scripts. Stick to common conventions, such as using meaningful variable names, following indentation rules, and avoiding overly complex expressions. For instance, use camelCase or snake_case for variable names and maintain consistent formatting throughout your script. This not only makes your code more approachable but also helps others who may work with your code in the future.
Tips for Maintaining and Organizing Perl Code
To keep your Perl code organized, consider the following strategies:
- Modular Design: Break your code into smaller, reusable subroutines or modules. This promotes code reuse and makes it easier to test and maintain individual components.
- File Structure: Organize your scripts and related files in a logical directory structure. Group related scripts together and use descriptive folder names to make navigation easier.
- Version Control: Utilize version control systems like Git to track changes, collaborate with others, and manage different versions of your code. This practice allows you to roll back changes if necessary and maintains a history of your development process.
Importance of Comments and Documentation
Comments play a vital role in enhancing code comprehension. Use comments to explain the purpose of complex code segments, document the expected input and output of functions, and provide context for your logic. Well-placed comments can significantly reduce the time spent deciphering your code later.
In addition to inline comments, consider maintaining external documentation. This can include an overview of your script’s functionality, installation instructions, and usage examples. Tools like Pod (Plain Old Documentation) in Perl allow you to create documentation directly within your scripts, ensuring that it stays up to date with your code changes.
Final Thoughts
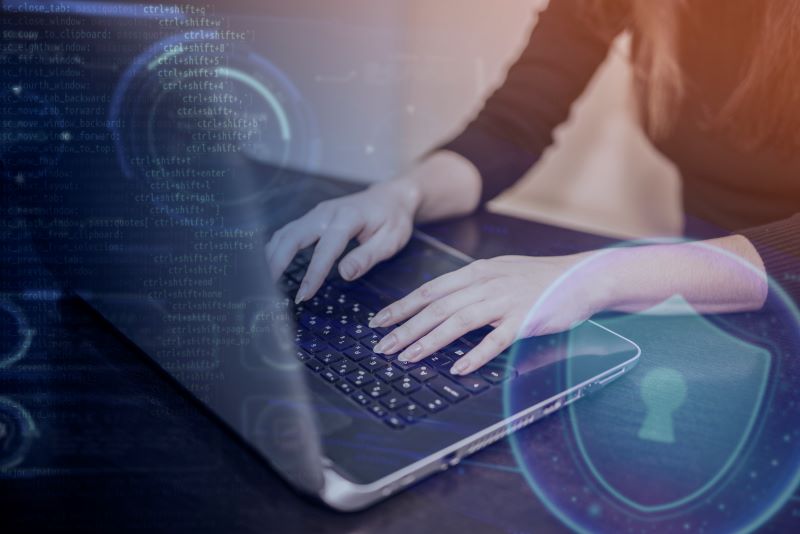
As you continue your journey with Perl, practice regularly and explore more advanced topics such as object-oriented programming, web frameworks, and database interactions. The more you experiment with Perl’s features and modules, the more proficient you will become.
For those looking to expand their tech knowledge beyond learning how to execute Perl script, be sure to check out Rayobyte’s blog. We regularly publish tutorials and insights on a variety of programming languages, tools, and best practices to help you stay ahead in the tech world.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.