How to Use Python to Scrape Remote Google Jobs Listings
Table of Contents
Google Jobs is a powerful aggregator that gathers job postings from a wide range of sources and offers a seemingly endless list of possibilities. What if you could efficiently navigate this vast landscape and uncover the most relevant opportunities faster and easier?
That’s where web scraping Google Jobs listings comes in.
Learn how to scrape in-person and remote Google job listings using Python below. You’ll also discover the benefits of using residential proxies for web scraping and how you can choose the best proxy provider.
Remote Google Job Listings: The Basics
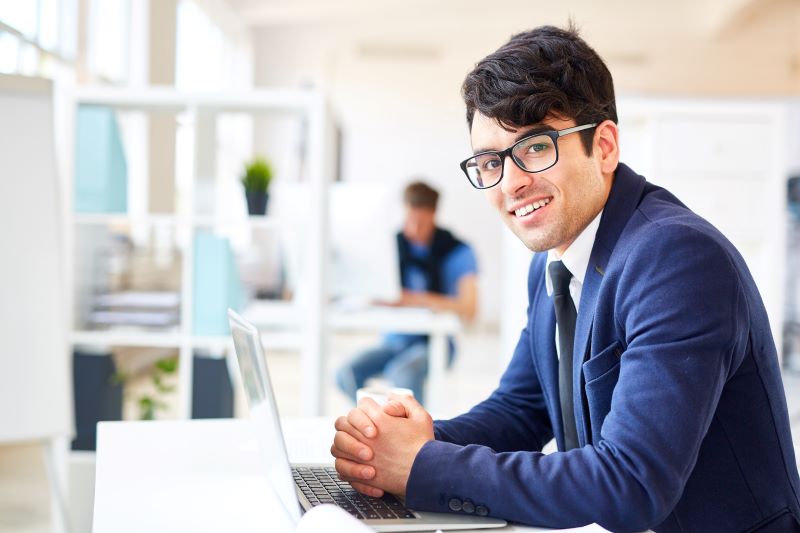
When you conduct a “jobs near me” Google search or look for remote jobs with Google, you’ll be met with an index of job listings. These listings typically feature the following components:
- Job Title: This part clearly states the specific role you’d be applying for.
- Location: This section may specify the physical office location or mention if it’s a remote position.
- Team/Department: This tells you which specific team or department you’d be working under.
- Job Summary: This portion provides a brief overview of the role, highlighting its key responsibilities and requirements.
- Qualifications: This section details the education, experience, and skills needed for the position.
- Responsibilities: This list outlines the specific duties and tasks you’d be expected to perform.
- Impact: This part describes the broader impact and significance of the role within Google.
- Benefits: This piece highlights the perks and benefits offered to Google employees, such as health insurance, paid time off, and professional development opportunities.
If you look at the HTML structure of these pages, you’ll also see that each listing is enclosed in the <li> or “list item” tag and wrapped within the <ul> or “unordered list” tag. This information will come in handy when you begin the actual scraping process.
Benefits of Scraping Job Listings for Google
Why would you want to scrape Google job listings search results? Lots of reasons!
Here are some of the greatest benefits scraping job listings offers to job seekers and others who frequently find themselves scouring Google job ads:
- Gain valuable insights: Analyze trends in skills, job offerings, and hiring practices by employers in your field.
- Gain a competitive edge: Identify skills in high demand or specific employers actively hiring to tailor your application strategy.
- Find hidden opportunities: Discover listings not indexed on popular job boards to potentially expand your job search reach.
- Automate search: Build scraping tools to automatically collect and filter job listings based on your criteria to save time and effort.
- Improve job boards: Data scraped from Google listings can be used to populate job boards and potentially provide broader job search options.
Why Use Python to Scrape Remote Jobs for Google?
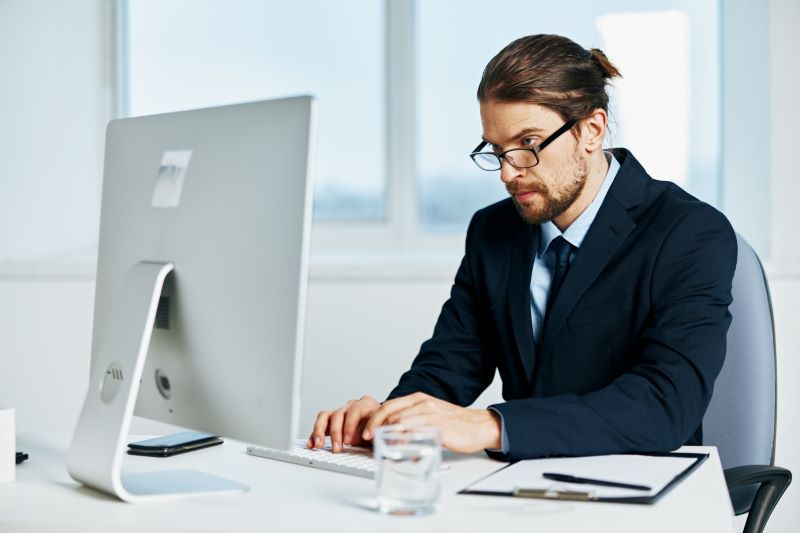
Python is one of the most popular programming languages for scraping Google Jobs listings (and for web scraping in general). The following are some of the top advantages of using Python to scrape remote Google job listings:
- Ease of use: Python has a clear and concise syntax, which makes it relatively easy to learn and write code, even for beginners.
- Large libraries and frameworks: Python offers a rich ecosystem of libraries like Beautiful Soup, Scrapy, and Selenium, which are specifically designed for web scraping and can simplify complex tasks.
- Handles various data formats: Python can efficiently work with diverse data formats like HTML, JSON, and XML, all of which are often encountered in web scraping.
- Integration with other tools: Python seamlessly integrates with data analysis and visualization tools like Pandas and Matplotlib, enabling further insights from scraped data.
- Handles large datasets: Python can handle and manipulate large datasets effectively, which is crucial for scraping significant amounts of data.
- Parallel processing capabilities: Libraries like Scrapy support parallel processing, which allows you to distribute scraping tasks across multiple machines for faster results.
- Active community: Python has a vast and active online community that offers abundant resources, tutorials, and assistance when needed.
- Open-source libraries: Most web scraping libraries for Python are open-source and provide free access and customization options.
- Cross-platform: Python runs on various operating systems like Windows, Mac, and Linux, which makes it adaptable to different environments.
- Automation capabilities: Python has impressive automation capabilities, which allow you to build tools that regularly scrape websites and collect fresh data.
How to Scrape Google Search Results with Python
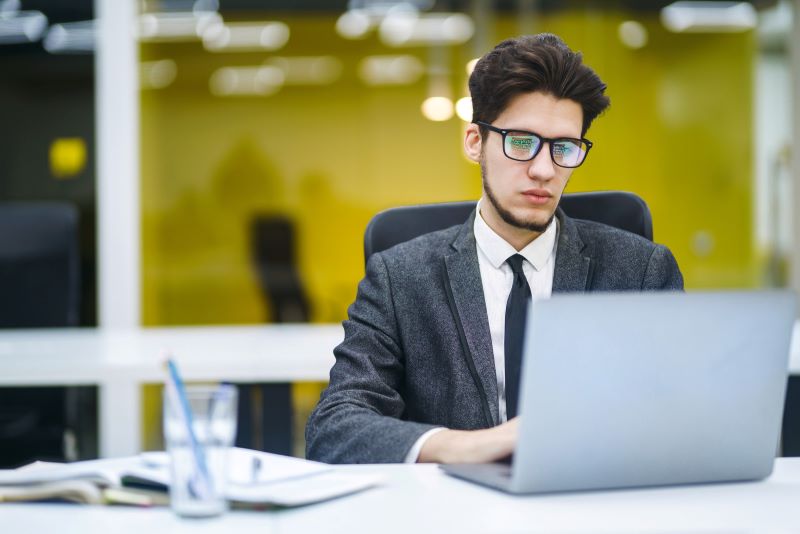
In this section, we’ll provide step-by-step instructions on scraping Google Jobs using Python and the browser automation framework called Playwright.
Step 1: Install Playwright
Start by installing Playwright. Here is the code you’ll use to install it in Python:
pip install playwright
# to download the necessary browsers
playwright install
Step 2: Write the code
Next, you’ll write your code using the Playwright API. Here’s an example of the code you might use to scrape job listings for web developer positions in Denver:
import asyncio
import json
from playwright.async_api import Playwright, async_playwright
search_keyword = “Web Developer”
search_location = “Denver”
pagination_limit = 23
data = []
def save_data():
“””
Saving the globally stored data as JSON
“””
with open(“google_career_data.json”, “w”) as outfile:
json.dump(data, outfile, indent=4)
def clean_data(data: str or list) -> str:
“””
This function will do basic string cleaning. If the input is a string
It will clean the data and return the cleaned data. If it is listed,
It will iterate through each element to clean and join them with a pipe.
Args:
data (str or list): The input can be string or list
Returns:
str: cleaned string
“””
if isinstance(data, str):
data = ” “.join(data.split()).strip()
return data
data = [” “.join(i.split()).strip() for i in data]
data = ” | “.join(data)
return data
async def extract_data(page, job_element) -> None:
“””This function is to extract data from the job listings page
Args:
page (playwright page object)
job_element (Playwright locator object)
“””
# Initializing necessary xpaths
xpath_title = “//h2[@class=’p1N2lc’]”
xpath_min_qualification = “//h3[text()=’Minimum qualifications:’]/following-sibling::ul[1]/li”
xpath_prefered_qualification = “//h3[text()=’Preferred qualifications:’]/following-sibling::ul[1]/li”
xpath_about_this_job = “//div[@class=’aG5W3′]/p”
xpath_responsibilities = ‘//div[@class=”BDNOWe”]/ul/li’
xpath_job_url = “../../a”
# Extracting necessary data
title = await page.locator(xpath_title).inner_text()
min_qualification = await page.locator(xpath_min_qualification).all_inner_texts()
preferred_qualifications = await page.locator(xpath_prefered_qualification).all_inner_texts()
about_this_job = await page.locator(xpath_about_this_job).all_inner_texts()
responsibilities = await page.locator(xpath_responsibilities).all_inner_texts()
job_url = await job_element.locator(xpath_job_url).get_attribute(“href”)
# Cleaning
title = clean_data(title)
min_qualification = clean_data(min_qualification)
preferred_qualifications = clean_data(preferred_qualifications)
about_this_job = clean_data(about_this_job)
responsibilities = clean_data(responsibilities)
job_url = clean_data(job_url)
job_url = f”https://www.google.com/about/careers/applications{job_url}”
data_to_save = {
“title”: title,
“min_qualification”: min_qualification,
“preferred_qualifications”: preferred_qualifications,
“about_this_job”: about_this_job,
“responsibilities”: responsibilities,
“job_url”: job_url,
}
# Appending to a list to save
data.append(data_to_save)
async def parse_listing_page(page, current_page: int) -> None:
“””This function will go through each jobs listed and click it
and pass the page object to extract_data function to extract the data.
This function also handles pagination
Args:
page (playwright page object)
current_page (int): current page number
“””
xpath_learn_more = “//span[text()=’Learn more’]/following-sibling::a”
xpath_jobs = “//li[@class=’zE6MFb’]//h3”
xpath_title = “//h2[@class=’p1N2lc’]”
xpath_next_page = “//div[@class=’bsEDOd’]//i[text()=’chevron_right’]”
if current_page == 1:
# Clicking Learn more button (For the first page only)
learn_more_buttons = page.locator(xpath_learn_more)
first_learn_more_buttons = learn_more_buttons.nth(0)
await first_learn_more_buttons.click()
# Locating all listed jobs
await page.wait_for_selector(xpath_jobs)
jobs = page.locator(xpath_jobs)
jobs_count = await jobs.count()
# Iterating through each jobs
for i in range(jobs_count):
# Clicking each job
job_element = jobs.nth(i)
await job_element.click()
await extract_data(page, job_element)
await page.wait_for_selector(xpath_title)
# Pagination
next_page = page.locator(xpath_next_page)
if await next_page.count() > 0 and current_page < pagination_limit:
await next_page.click()
await page.wait_for_selector(‘//h3[@class=”Ki3IFe”]’)
await page.wait_for_timeout(2000)
current_page += 1
await parse_listing_page(page, current_page=current_page)
async def run(playwright: Playwright) -> None:
“””This is the main function to initialize the playwright browser
and create a page. Then do the initial navigations.
Args:
playwright (Playwright)
“””
# Initializing browser and opening a new page
browser = await playwright.chromium.launch(headless=False)
context = await browser.new_context()
page = await context.new_page()
# Navigating to homepage and clicking the “jobs” icon
await page.goto(“https://careers.google.com/”, wait_until=”domcontentloaded”)
await page.get_by_role(“link”, name=”Jobs results page”).click()
# Typing the job name and clicking enter
job_search_box = page.locator(“//input[@id=’c3′]”)
await job_search_box.click()
await job_search_box.type(search_keyword)
await job_search_box.press(“Enter”)
# Clicking the location searchbox icon
await page.locator(“//h3[text()=’Locations’]”).click()
location_filter_box = page.locator(‘//input[@aria-label=”Which location(s) do you prefer working out of?”]’)
await location_filter_box.click()
await location_filter_box.type(search_location, delay=200)
await location_filter_box.press(“Enter”)
await page.wait_for_load_state()
await page.wait_for_timeout(2000)
await parse_listing_page(page, current_page=1)
save_data()
await context.close()
await browser.close()
async def main() -> None:
async with async_playwright() as playwright:
await run(playwright)
asyncio.run(main())
Step 3: Run the code
From here, you can run the code and collect scraped data from Google Jobs.
How to Scrape Remote Jobs on Open Source
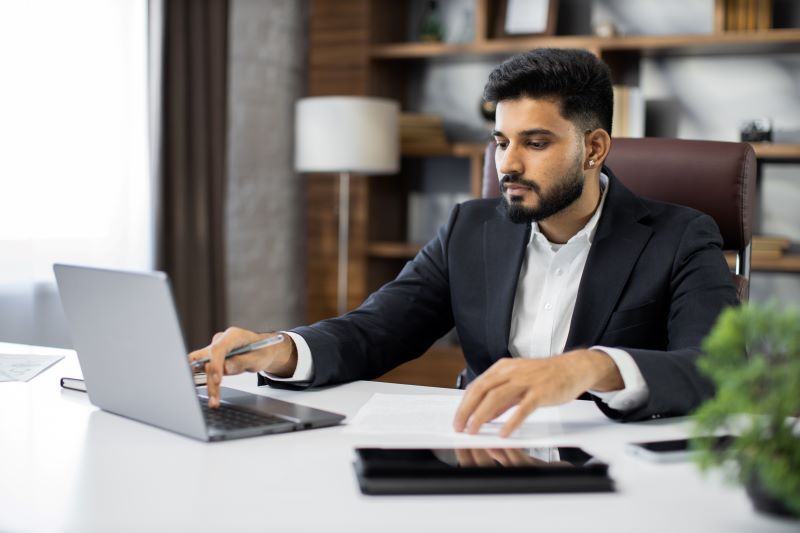
The process of scraping in-person and remote jobs on Open Source is similar to the process of scraping Google Jobs listings. Here’s an example of the code you might use to scrape Open Source jobs using Python and the BeautifulSoup library:
import requests
from bs4 import BeautifulSoup
def scrape_opensource_remote_jobs():
# URL of the opensource remote jobs page
url = “opensource url”
# Send a GET request to the URL
response = requests.get(url)
# Check if the request was successful (status code 200)
if response.status_code == 200:
# Parse the HTML content of the page using BeautifulSoup
soup = BeautifulSoup(response.content, ‘html.parser’)
# Extract relevant information from the HTML
job_listings = soup.find_all(‘div’, class_=’job_content’)
# Process and print the job listings
for job_listing in job_listings:
job_title = job_listing.find(‘h2′, class_=’job_title’).text.strip()
company_name = job_listing.find(‘span’, class_=’job_company_name’).text.strip()
location = job_listing.find(‘span’, class_=’job_city’).text.strip()
print(f”Job Title: {job_title}”)
print(f”Company: {company_name}”)
print(f”Location: {location}”)
print(“=” * 50)
else:
print(f”Failed to retrieve page. Status code: {response.status_code}”)
if __name__ == “__main__”:
scrape_opensource_remote_jobs()
Why Use a Proxy to Scrape Google Jobs Listings?
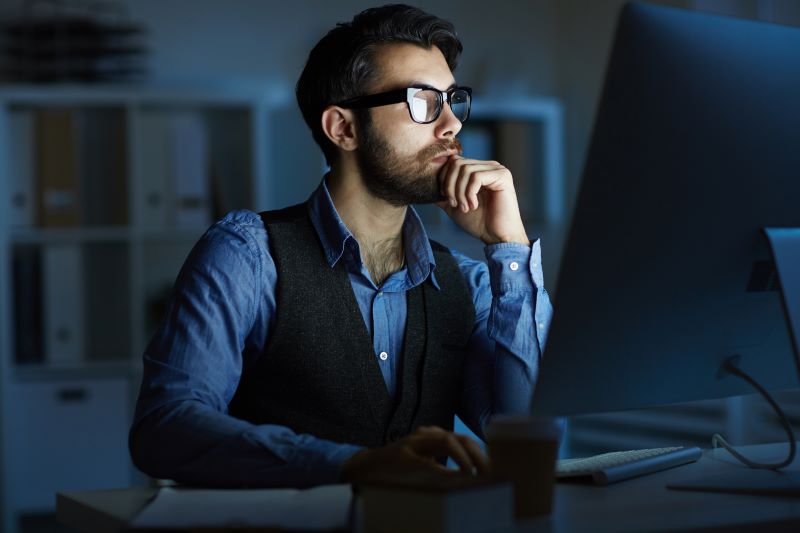
If you want to scrape Google search results using Python, it helps to use a web scraping proxy.
A proxy sits between your computer and the target website you’re scraping (in this case, Google Jobs) and acts as an intermediary. Here’s a breakdown of what proxies can do:
- Hide your IP: By routing your requests through the proxy server, the website only sees the proxy’s IP address, not yours. This helps avoid getting your own IP blocked if you make too many scraping requests, which can trigger anti-bot measures.
- Rotate IP addresses: Many scraping proxies offer IP rotation, meaning they switch the IP address used for each request. This further minimizes the risk of detection and blocking, as the website sees different users accessing their content.
- Access geo-restricted content: Some proxies offer IPs from specific geographic locations. This allows you to scrape content that might be restricted to users in certain regions, like localized product offerings or news articles.
- Increase speed and reliability: Some scraping proxies offer faster connections and higher success rates compared to standard scraping directly with your IP. This can be helpful for large-scale scraping projects.
Benefits of using proxies for web scraping
Now that you know what proxies do, let’s talk about why they can be valuable additions to your tool belt. Here are some specific reasons why using a proxy can be beneficial, especially when it comes to web scraping:
- IP address rotation: Proxies allow you to rotate your IP addresses, helping to prevent IP bans or restrictions imposed by the target website. When scraping a website intensively, using the same IP address repeatedly might trigger automated security measures.
- Avoid rate limiting: Websites often have rate limits in place to prevent automated scraping and to ensure fair usage for all users. By using proxies and distributing your requests across different IP addresses, you can avoid hitting these rate limits.
- Anonymity: Proxies provide a level of anonymity. When scraping a website, it’s a good practice to avoid revealing your actual IP address. Using proxies can help protect your identity and prevent potential consequences.
- Geographical flexibility: Proxies can be located in different geographical locations. If the website you’re scraping has regional restrictions or different content based on location, using proxies with various IP locations allows you to access and scrape content from different regions.
- Scalability: Proxies enable you to scale your scraping efforts. By distributing requests across multiple IP addresses, you can increase the number of simultaneous connections without overloading a single IP address.
Types of proxies for web scraping
It’s important to note that there are several different types of proxies you can choose from for web scraping tasks. The following are some of the most well-known options:
- Residential proxies: Residential proxies use IP addresses assigned by Internet Service Providers (ISPs) to residential users. They appear as legitimate residential IP addresses, making it harder for websites to detect and block them. Residential proxies are ideal for scraping websites with sophisticated anti-scraping measures.
- Data center proxies: Data center proxies are IP addresses provided by data centers. They are faster than residential proxies but might be more easily detected by websites. However, they are cost-effective and suitable for less restrictive scraping tasks.
- ISP proxies: ISP proxies combine some characteristics of both data center proxies and residential proxies. They use real IP addresses to enhance security but are typically hosted on data center infrastructure, which increases speed and reduces latency.
Why Scrape Google Jobs Listings Using Python with a Residential Proxy?
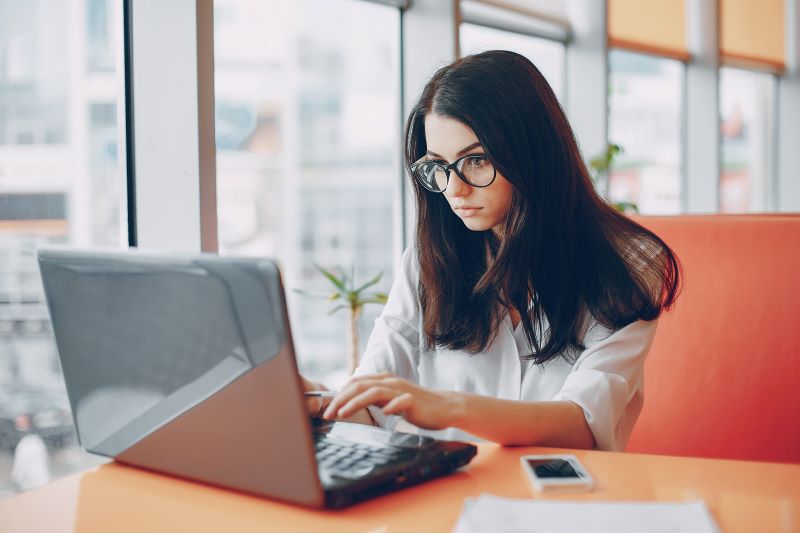
At this point, you know the advantages of using Python for scraping Google Jobs listings (as well as other types of web data. You also know the benefits of using proxies for your web scraping activities.
Now, you need to choose the type of proxy you’ll use to carry out these tasks.
If you’re ready to start using proxies to scrape results for jobs near me on Google, residential proxies are some of the best options to utilize. Here are some of the top reasons for relying on residential proxies:
- Anonymity and legitimacy: Residential proxies use IP addresses assigned by ISPs to residential users. As a result, they closely mimic real user behavior, making it more challenging for websites to identify and block scraping activities. This level of legitimacy and anonymity can help you avoid IP bans.
- Low detection risk: Websites are often more tolerant of residential IP addresses because they represent real users. This lowers the risk of being detected as a scraper, resulting in a decreased likelihood of IP bans or other anti-scraping measures.
- Geographical diversity: Residential proxies provide IP addresses from different geographic locations. If a website serves content based on users’ locations, residential proxies allow you to access and scrape data as if you were a user from various regions.
- Bypass IP blocks and rate limiting: Websites may impose IP blocks or rate limits to prevent automated access. Residential proxies help you overcome these restrictions by rotating through a pool of IP addresses, preventing your scraping activity from being easily identified and restricted.
- Reliability and stability: Residential proxies generally offer better stability and reliability compared to other types of proxies. Since they use real residential IP addresses, they are less likely to be flagged as suspicious or blocked.
- User-agent spoofing: Residential proxies can be configured to rotate user agents, further emulating real user behavior. This helps avoid detection based on consistent user-agent patterns and adds an additional layer of stealth to your scraping activities.
- Scalability: Residential proxies are scalable, allowing you to distribute your scraping requests across a large pool of IP addresses. This scalability is crucial for handling larger scraping tasks without encountering rate limits or performance issues.
How to Choose a Residential Proxy Provider
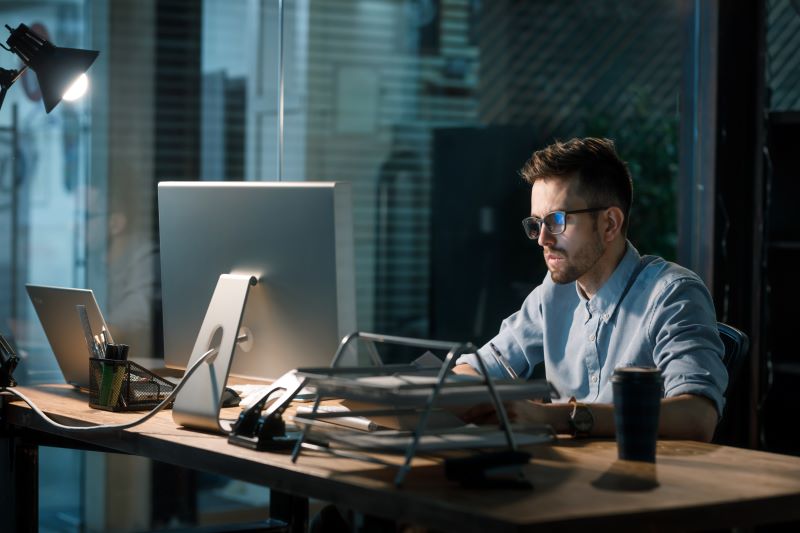
If you want to use residential proxies when using Python to scrape Google Jobs listings, you have plenty of options to choose from — not all of them are created equal, though. Here are some of the most important factors to keep in mind when picking a residential proxy provider:
- Size: A larger pool of IPs means less chance of encountering blocks or slowdowns. Look for providers with a large pool of residential IPs across various countries.
- Location: Consider your specific needs. Do you need global coverage or targeting by city or ISP? Choose a provider offering the locations you require.
- Speed: Ideally, you want low latency and fast connection speeds for smooth browsing and data gathering.
- Uptime: Ensure the provider offers high uptime guarantees to avoid service disruptions.
- Bandwidth: Check the included bandwidth and whether it aligns with your usage expectations.
- Targeting options: Many providers offer features like country, state, and city targeting for location-specific needs.
- Concurrency: Determine how many simultaneous connections you need and choose a provider offering enough slots.
- Pricing: Compare pricing models (monthly pricing, pay-as-you-go, etc.) and features included in different plans. Look for transparency and avoid any providers that are known for sneaking in hidden fees.
- Security: Make sure the provider offers secure connections and adheres to data privacy regulations.
- Customer support: Responsive and helpful customer support is crucial if you encounter any issues.
- Free trial: Many providers offer free trials, allowing you to test their service before committing.
Final Thoughts
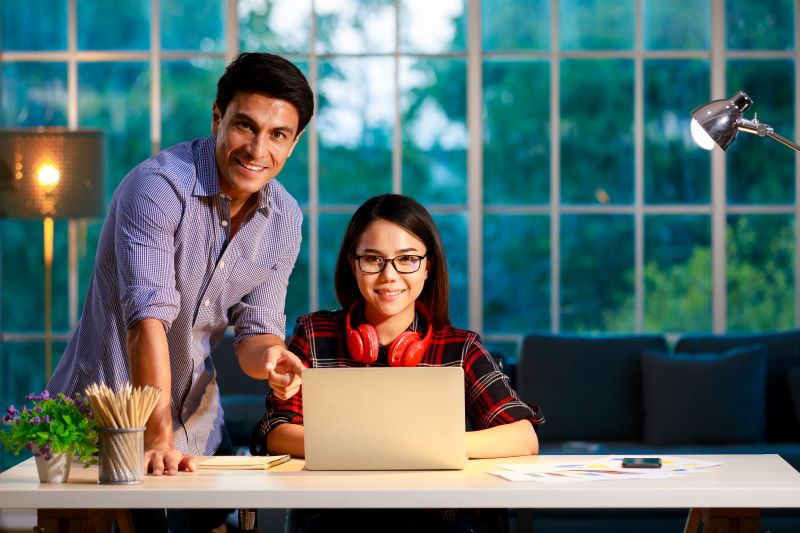
The process of using Python to scrape remote Google Jobs listings might seem daunting at first. If you use the right tools, though, including residential proxies, it’s much easier to collect the job-related data you need without facing some of the biggest web scraping challenges.
Are you looking for a residential proxy provider that meets all the criteria listed in the previous section? If so, Rayobyte has got you covered.
Rayobyte is an award-winning proxy provider with a strong commitment to reliability and ethics. We are the United States’ largest proxy provider and deliver a wide range of services, including residential proxies for scraping job listings.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.
Sign Up for our Mailing List
To get exclusive deals and more information about proxies.
Start a risk-free, money-back guarantee trial today and see the Rayobyte
difference for yourself!