Python Tutorial For Parsing HTML With PyQuery
Web scraping is a powerful tool that allows businesses to quickly and easily extract data from websites. This data can be used for a variety of purposes, including market research, competitor analysis, price comparison, and lead generation. Web scraping enables businesses to gain valuable insights into customer behavior and preferences which can help improve products or services. It also helps companies stay up-to-date with the latest trends in their industry by allowing them to monitor changes on other sites quickly and accurately. It’s an invaluable asset for any business looking to stay ahead of the competition in today’s digital world.
Supporting web scraping are several pillars you’ll need to understand to use the process effectively, such as the programming language you’ll use. Python is a popular programming language for web scraping due to its ease of use and powerful libraries. It can be used to parse HTML, extract data from websites, and automate tasks such as filling out forms or clicking buttons.
Understanding how to parse HTML is essential when using Python for web scraping because it allows developers to identify the elements on a page that contain the desired information. Once these elements are identified, they can be extracted with Python code.
In this PyQuery tutorial, you’ll learn the basics, including how to parse HTML in Python and the fundamentals of Python’s two most popular HTML parsing libraries: BeautifulSoup and PyQuery.
What Is an HTML Parser in Python?
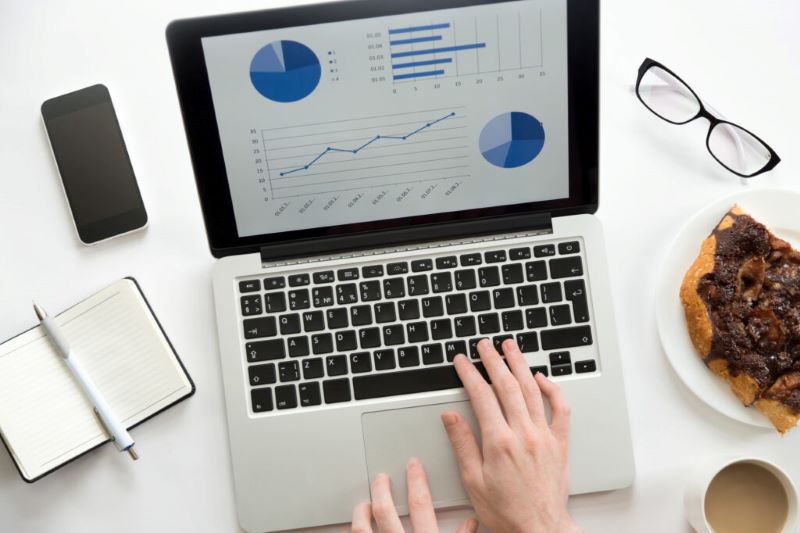
HTML parsers are computer programs that process HTML documents and make it easier for developers to work with them. Parsing HTML using regular expressions in Python is one way, but that’s ideal for well-defined, one-off tasks. If a developer wants to extract some data in a repeatable manner from a web page or site, they are better off using an HTML parser in Python that will quickly break the website into smaller components that can be manipulated separately. This makes it much easier for the developer to get the specific information they need from the page without wasting time sifting through its code manually.
Essentially, HTML parsers allow developers to parse and manipulate HTML documents and can be used for various tasks beyond extracting specific pieces of data from web pages. For example, they can extract links or other data from an HTML document’s Document Object Model (DOM) tree. They can also transform the whole page into structured data.
Say you needed to get the titles of all blog posts on your website. An HTML parser makes it possible to quickly isolate and parse the relevant data or tags within the page containing those titles so that you can loop through them programmatically and add each title to a list for later use. Using an HTML parser makes extracting data much more efficient than manually going through the code line by line and far less error-prone.
As you can imagine, HTML parsing is essential to any web scraping pipeline. This type of data extraction can be used to more efficiently retrieve specific pieces of information from a website, such as product prices for comparison-shopping sites or content for aggregation and dissemination in other formats like JSON or RSS. HTML parsers make it much easier to quickly and accurately isolate the relevant tags within a page, so they are invaluable for efficient data retrieval processes.
How To Parse HTML in Python With Libraries
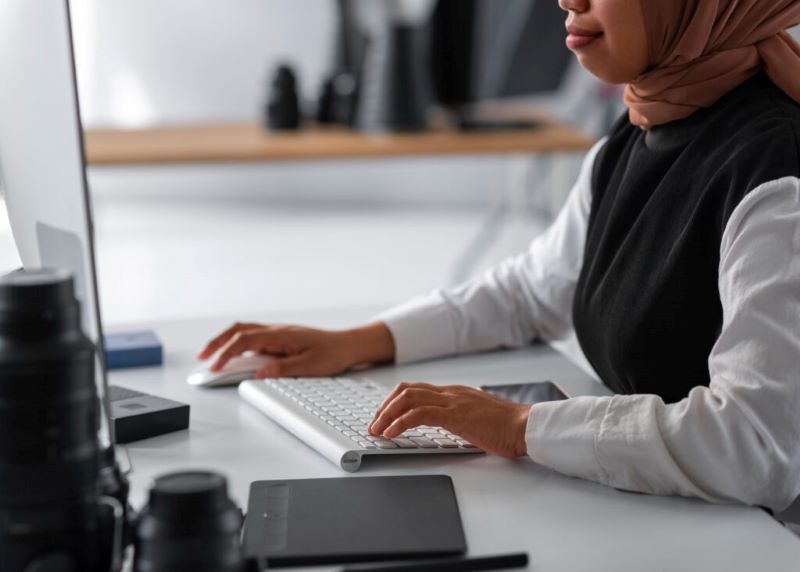
Parsing HTML in Python can be done using one of the many available libraries. Generally speaking, libraries are third-party packages that provide a variety of useful features for dealing with web scraping and parsing tasks. The most popular library for parsing HTML with python is BeautifulSoup, although other popular alternatives include LXML and html5lib. Our particular focus is PyQuery, of course.
To use any of these libraries successfully, you must ensure they are correctly installed on your OS environment. Once installed successfully, you can import the library into your script via an import statement. You then need to connect to your target page by providing its URL or loading it from a file if previously downloaded.
Once connected, you use the appropriate function depending on which parser implementation you selected to parse through all elements in a specified page. You’ll look for desired element matches while returning populated result objects that contain what you need. Upon finding what you want, you may want to manipulate your collected information further, or you can terminate the script after saving your work in an output file, for example.
Below, we’ll use PyQuery and BeautifulSoup — arguably the two top contenders that offer powerful features for parsing HTML documents quickly and efficiently in Python code. We’ll first go through a PyQuery tutorial and briefly see how BeautifulSoup compares.
What Is PyQuery?
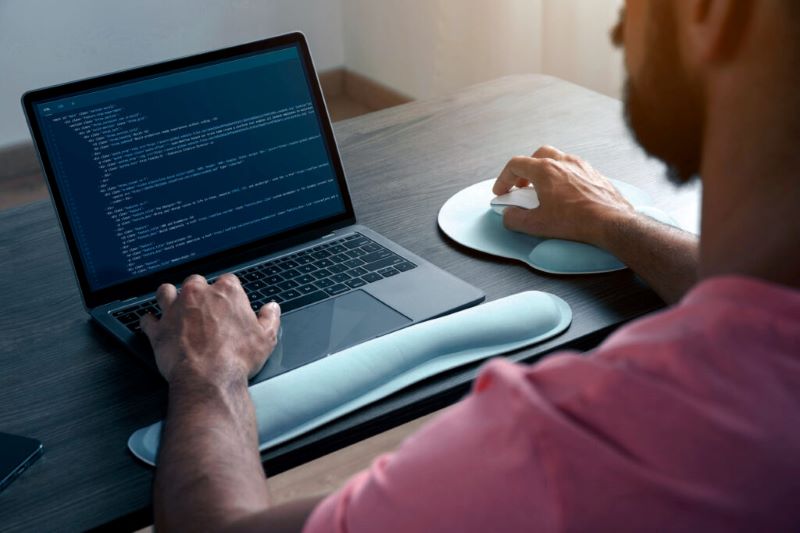
PyQuery is a Python library designed to simplify parsing HTML and XML files. It allows you to navigate DOM hierarchies and provides ways of manipulating the data in those documents. PyQuery offers an intuitive jQuery-like syntax for selecting elements from a document and making changes to them.
The jQuery-like syntax offered by PyQuery makes writing web scraping projects and other automated tasks that require processing large amounts of structured data much easier. By using this jQuery-like syntax, developers can quickly identify document elements without knowing a lot of HTML or CSS. This also reduces the amount of code needed to accomplish tasks, making development more efficient and less prone to error. Ultimately, it saves time and money.
To further contextualize the advantage that jQuery-like syntax offers, you can see how jQuery itself makes it easy for developers to manipulate data in DOM hierarchies with a few lines of code. It uses CSS selectors to find elements within the page and provides methods to make changes directly from within your script. For example, if you want to make sure every link on a webpage has its target attribute set correctly in HTML, you would need to manually add the “target” attribute to each anchor tag in the page’s source code. For instance:
<a href=”www.example.com” target=”_blank”>Link</a>
But you would need to do that for every instance of an anchor tag. If you had a dozen different links on your web page, you’d need to do this a dozen times.
The same process would be much more involved with JavaScript. You would need to first select all of the anchor tags in the page with document.querySelectorAll(‘a’) and then use a loop to go through each one and add the attribute target:
for (let element of elements) {
element.setAttribute(‘target’, ‘_blank’);
}
Using jQuery allows you to do all this in just one line, without the need for looping structures, saving significant time:
$( “a” ).attr( ‘target’, ‘_blank’ );
This saves time by allowing web developers to quickly identify and update elements without writing extensive HTML or JavaScript code. Further below, you will see how PyQuery’s jQuery-like syntax confers these same advantages when parsing HTML.
How to use PyQuery to parse HTML in Python
PyQuery offers a more natural and concise way to query HTML documents in Python. To quickly illustrate its use:
- Install PyQuery on your system
- Create an object for the document you wish to parse
- Use CSS selectors such as $(‘div’) or $(‘.class_name’) for locating elements within the source code tree
- Once located, these elements can then be further filtered, iterated over, and manipulated as needed
Installing PyQuery is easy. You can use the pip package manager or download the source code from GitHub. Then, use either pip via the command pip install pyquery for Python 3 or pip3 install pyquery for Python 2. Alternatively, you can download the repository from GitHub to get started.
Next, use the PyQuery constructor to create an object for a parsed page. Here is an example:
from pyquery import PyQuery as pq
my_url = ‘https://example.com’
my_page = open(my_url).read()
my_parsedPage = pq(my_page)
The first line imports the PyQuery library with the alias “pq” for ease of reference later. The second line specifies the URL of our desired web page we want to parse and stores it as a variable called my-url — again, we’ll use example.com for now. We then open and read this URL’s contents using Python’s built-in open() function, storing it within another variable named my_page. Finally, we create an object from this HTML source code by passing my_page into PYQUERY’s constructor that saves that parsed object under our variable name “my_parsedPage.” This automatically parses your desired page’s HTML tree structure so you can easily access its content.
Once we have the parsed page object, you can use CSS selectors such as $(‘div’) or $(‘.class_name’) to retrieve specific elements within your HTML document. Here is an example:
my_tags = my_parsedPage.find(“div”)
print(my_tags)
This code sample calls on PyQuery’s selector method — which allows us to pass in a desired tag type or data attribute we want from our page’s HTML text as arguments (in this case, <div>). It parses through your desired page’s HTML tree structure when called and generates its content into a simple data structure you can access using PyQuery’s functions like find() or select(). The last step simply prints out the elements list, but in reality, the output could be saved in a file or passed to other processes downstream.
This structure is similar to BeautifulSoup, but we can see how PyQuery’s jQuery-style syntax makes it much easier to use when manipulating HTML elements. Here is an example:
my_parsedPage(‘div’).filter(‘.class_name’)
my_parsedPage(‘div’).each(lambda i, e: print(e))
The first line uses PyQuery’s filter() method, which allows you to pass in a CSS selector as an argument. In this case, we filter our parsed page object so that only <div> elements with the class name ‘class-name’ remain. The second line then utilizes PyQuery’s each() method that allows us to loop through all of these remaining <div> elements and access their individual content. Here we use the Python lambda function (an anonymous function) so that each element can be printed out one by one.
If you were to perform the same thing in BeautifulSoup, for example, you would need some formal loop structure (for loop or while loop) to achieve the same result. Some library-specific methods make the work easier (e.g., it would be something like the .find_all() method in BeautifulSoup), but you would still need to iterate the printing of the output within a loop — which takes more lines of code.
Ultimately, in PyQuery, you can do away with approaches like that and simplify a lot of work into a single line of code.
How to use BeautifulSoup to parse HTML in Python
To better illustrate the key differences, let’s dive into BeautifulSoup, which accesses web page content by navigating and searching data in the parsed tree structure. The high-level process is identical to PyQuery, but with steps specific to BeautifulSoup.
To install BeautifulSoup, you can navigate to a terminal or command line and use the command: pip install bs4. After running this line, you should have installed the latest version of BeautifulSoup on your machine.
To create an object for a parsed page, you can use the open() method to get a handle on your web page’s content. Here is an example:
from bs4 import BeautifulSoup as soup
my_url = ‘https://example.com’
my_page = open(my_url).read()
my_parsedPage = soup(my_page,”html.parser”)
It’s essentially the same with PyQuery except for the import at the start and the alias — from “pq” to “soup.”
BeautifulSoup creates an object out of HTML source code. It takes two arguments: the HTML-page content (we stored as my_page) and a parser we wish to use (in this case, html.parser). When called, it parses through your desired page’s HTML tree structure and generates an easy-to-navigate data structure you can access using BeautifulSoup’s functions like find() or select().
Once we have the parsed-page object, you can use functions such as find_all() and select() to retrieve the specific elements within your HTML document. Here is an example:
my_tags = my_parsedPage.find_all([‘p’, ‘title’])
This code calls on BeautifulSoup’s .findAll method, allowing us to pass a desired tag type or data attribute from our page’s HTML text as arguments. Here, we specify both <p> tags (representing paragraph blocks) and self-explanatory <title> tags.
The first line uses the .findAll method on the previously created my_parsedPage variable to take all the <p> and <title> tags and include them in my_tags. Once done, you can use the same for loop structure used in the PyQuery example to achieve the same result.
BeautifulSoup vs. PyQuery
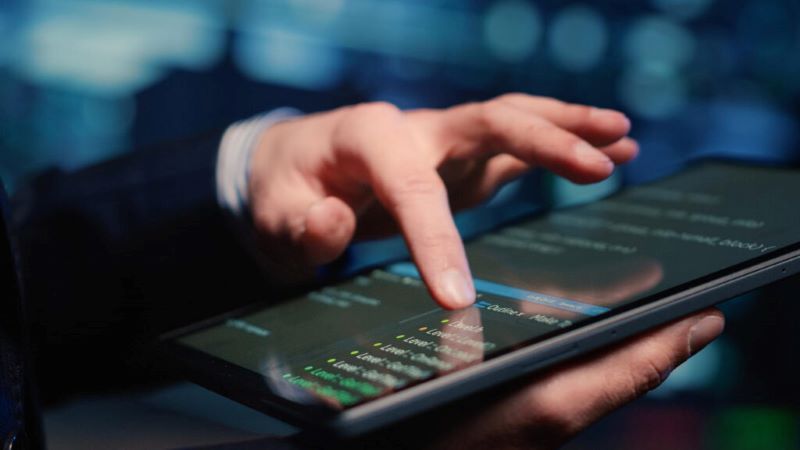
PyQeury is similar to BeautifulSoup in that both make it easier to parse HTML/XML documents into structured data. The main difference is that PyQuery supports a jQuery-like syntax for selecting elements from a document and making changes to them, while BeautifulSoup does not support these features natively.
Of course, BeautifulSoup is quite popular and widely used. So that’s a leg up over PyQuery. It offers a wide range of support for using different types of parsers in conjunction, such as LXML or HTML5lib. It also supports third-party parser libraries for even more flexibility in dealing with tricky HTML documents.
On the other hand, PyQuery is also a powerful library that provides great features and an intuitive API over BeautifulSoup — including CSS selectors and jQuery methods like .find(), .children(), and others, which make it easier to query the DOM elements from HTML pages. Because it operates according to CSS rules similar to those used by most modern browsers and websites, results are more accurate than they would be if trying more complicated matching processes that can be done using libraries or tools like BeautifulSoup. Furthermore, it has support for XPath expressions, making element filtering possible in a much more expressive way than vanilla BeautifulSoup.
You would also find that PyQuery generally offers better performance. PyQuery definitely outperforms traditional RegEx searching methods because there’s inherently less work involved in walking down a specific path already specified with CSS selectors. It also outperforms BeautifulSoup again because of more intuitive and accurate jQuery selectors, which require less backtracking, and queries return results faster. If it matters to your project, PyQuery usually offers easier debugging and logging capabilities. Results from query functions return descriptive objects, so you know exactly what properties information was pulled from certain strings.
In the end, if you need more advanced capabilities (such as manipulating the DOM structure or adding additional selectors), PyQuery would be your best choice. Note, however, that many developers use both in combination, depending on their needs. You may rely on both libraries for various web scraping projects, scopes, and scales.
Automating Web Scraping Pipelines
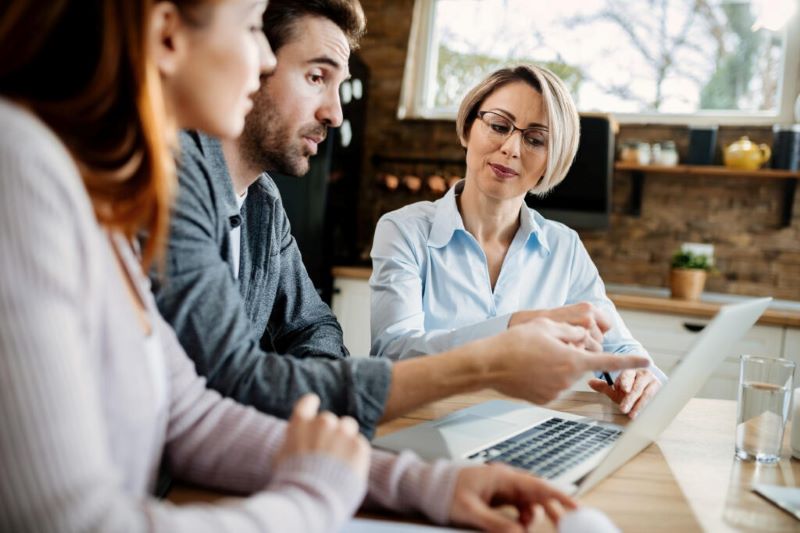
The libraries that enable the HTML parsing aspect of your project are important, but it’s also critical that you support your web scraping efforts with the right automation infrastructure. To successfully perform web scraping at scale, you need a dependable proxy server provider. You need a solid infrastructure built on reliable proxies.
As you know, web scraping employs automated bots to extract desired data from websites that a regular search engine may be unable to access. By setting parameters, this technique expedites the process of gathering vast numbers of information. However, these programs can activate security systems installed by search engines and web pages to protect against malicious bots.
Proxies change your IP address, making it difficult for websites to track and identify you. They also help you complete automated tasks more easily without limitations or restrictions. Using a proxy provides an extra layer of protection that can greatly increase the effectiveness and dependability of form automation tools.
Finding reliable proxies
Rayobyte can cater to your needs with residential, data center, or ISP proxies. Security is key, and that’s why Rayobyte takes an ethical yet professional approach when handling client data.
Residential proxies are often optimal for web scraping. These IPs stem from the ISPs of real people, so they are authenticated and continually refreshed. This helps your scrapers execute their duties without being noticed.
Proxies located in data centers can be advantageous when needing higher speed. These proxies send traffic through a server center, which increases connection rates. Unfortunately, you’re less likely to be able to access nonbusiness and rare IP addresses with this solution. However, it may still prove useful if you need an inexpensive option for obtaining a lot of information from the internet.
Using a proxy provided by an Internet Service Provider is a great option if you are seeking the perks of speed as well as data security. Rather than utilizing just a data center, these proxies are also associated with an ISP, allowing customers to benefit from fast speeds and the dependability of using said ISP.
Final Thoughts
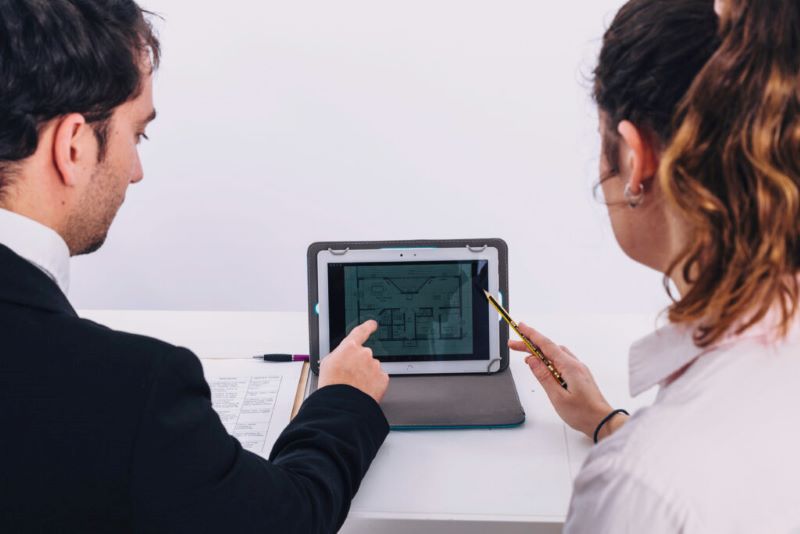
Web scraping offers businesses a wealth of data they can use to gain valuable insights into customer behavior and preferences, analyze their competitors and stay up-to-date with the latest market trends. The process is typically automated through coding and is made much easier through widely used programming languages such as Python.
Python is a popular language for web scraping due to its powerful libraries, providing developers with tools such as BeautifulSoup or PyQuery to help them quickly parse HTML documents. Using PyQuery, in particular, makes it much easier for developers by leveraging jQuery-like syntax, significantly cutting down on code needed to navigate tree hierarchies and manipulate elements within parsed pages.
For successful web scraping, a reliable proxy provider is essential. Rayobyte has many features and products, such as Scraping Robot, to strengthen and automate your data collection efforts. To take advantage of these tools and more, check out our services today!
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.