How To Set Up Proxies In PHP
Table of Contents
Don’t waste time typing “how to set up proxies” and “PHP proxy check working” into your favorite search engine. You can learn how to set up proxies in PHP and run a PHP proxy test (and much more) here.
This guide outlines the essential details everyone should know about setting up proxies in PHP and making sure they function properly. You’ll also learn about different types of proxies and the different troubleshooting steps you can take while using them. Let’s dive in!
What Is PHP?
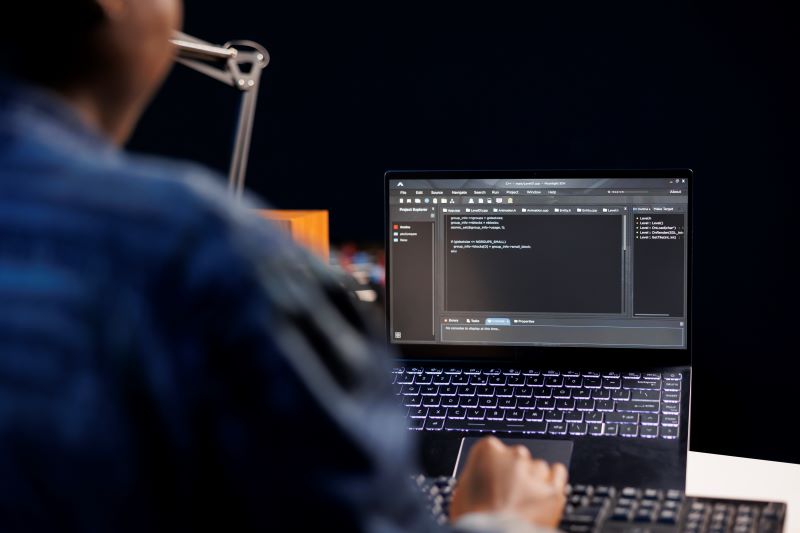
PHP stands for “PHP: Hypertext Preprocessor” in its most recent iteration, though it was originally created as a Personal Home Page tool. It’s a widely used open-source scripting language specifically designed for web development.
Here’s a breakdown of what PHP is and what it does:
- Server-side scripting: Unlike JavaScript, which runs on the user’s web browser, PHP is a server-side scripting language. This means PHP code executes on the web server before the resulting HTML content is sent to the user’s browser.
- Web development focused: PHP excels in creating dynamic web content. Imagine a website where content changes based on user input or information from a database. PHP can handle that by generating HTML based on specific conditions.
- Easy to learn: Compared to some other programming languages, PHP has a reputation for being relatively easy to learn, especially for those with some web development experience. This makes it accessible to a broad range of developers.
- Large community and resources: Due to its popularity, PHP has a vast community of developers and a wealth of online resources, tutorials, and libraries available.
PHP can be used for a variety of tasks. The following are some of the most common ones:
- Creating dynamic web pages: As mentioned earlier, PHP can generate content based on user input or data from databases. This allows for interactive features and personalized experiences on websites.
- Connecting to databases: PHP can connect to various database management systems to retrieve, store, and manipulate data. This is essential for building features like user accounts, shopping carts, or content management systems.
- Developing server-side applications: While primarily used for web development, PHP can also be used to create server-side applications that run on the web server and handle tasks behind the scenes.
- Form processing: When you submit a form on a website, PHP can be used to process the form data, validate user input, and potentially store the information in a database.
What Is a PHP Proxy Server?
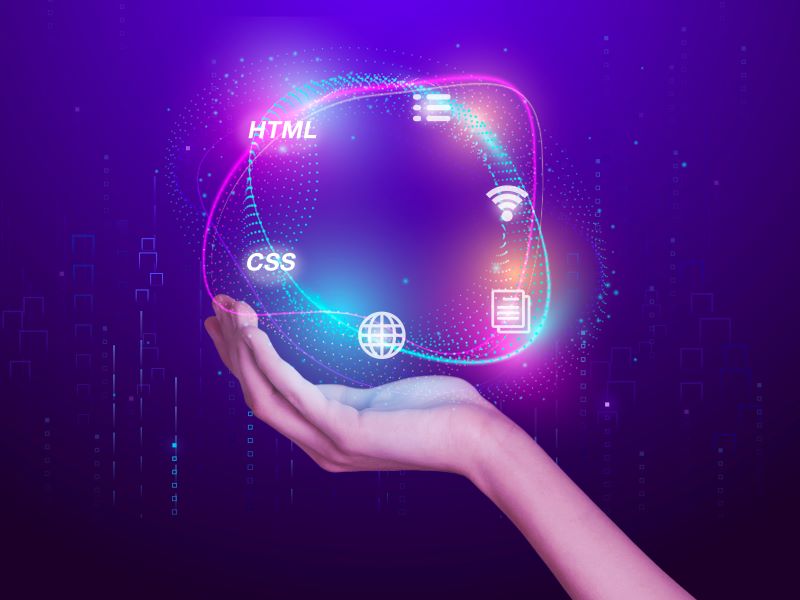
A PHP proxy server is essentially a program written in the PHP scripting language that acts as an intermediary between a client (your device) and the internet.
The server sits in the middle, fielding your requests to access websites and then forwards those requests on your behalf. The target website sees the proxy server’s IP address instead of your own, potentially offering some anonymity.
Here’s a breakdown of how it works:
- Client Makes Request: You, the client, try to access a website through your browser.
- Request to Proxy: Instead of going directly to the website, your request is routed to the PHP proxy server.
- Proxy Forwards Request: The PHP proxy server then takes your request and forwards it to the actual website you’re trying to reach.
- Website Responds: The website processes the request and sends a response back to the PHP proxy server.
- Proxy Delivers Response: Finally, the PHP proxy server receives the response from the website and delivers it back to you, your device.
There are various reasons why someone might use a PHP proxy server. For example, by hiding your IP address from the website, you can achieve a certain level of anonymity.
In some cases, a proxy server can also add an extra layer of security by filtering incoming and outgoing traffic.
PHP proxy server benefits
Using PHP to set up a proxy server offers several benefits, particularly for web developers and those who are already familiar with PHP. The following are some of the most noteworthy advantages:
- Rapid Development: PHP is known for its rapid development capabilities. Setting up a basic proxy server in PHP can be achieved quickly, allowing developers to prototype and deploy solutions rapidly.
- Platform Independence: PHP is a platform-independent language, meaning that PHP proxy servers can be deployed on various operating systems such as Linux, Windows, macOS, etc. This flexibility makes it suitable for a wide range of environments.
- Integration with Existing PHP Applications: PHP proxy servers can be seamlessly integrated with existing PHP applications. Developers can easily incorporate proxy functionality into their web applications, APIs, or services without major architectural changes.
- Customization and Extensibility: PHP’s flexibility allows developers to customize and extend proxy server functionality as needed. They can implement additional features, such as request/response manipulation, caching, logging, authentication, etc., to suit specific requirements.
- Community Support and Resources: PHP has a vast and active community of developers, along with abundant online resources, tutorials, and libraries. Developers can leverage these resources to troubleshoot issues, seek advice, and find solutions when setting up and managing PHP proxy servers.
- Scalability and Performance: While PHP may not be as performant as some other languages for certain tasks, PHP proxy servers can still be scaled and optimized for performance. Techniques such as caching, load balancing, and asynchronous processing can help improve scalability and responsiveness.
- Cost-Effective Solution: Since PHP is open-source and freely available, setting up a PHP proxy server typically incurs minimal costs. There’s no need to invest in proprietary software or licenses, making it a cost-effective solution for many use cases.
- HTTP(S) Proxy Support: PHP has robust support for handling HTTP and HTTPS requests, making it suitable for implementing HTTP(S) proxy servers. Developers can easily handle HTTP methods, headers, cookies, redirects, and other HTTP-related features in their proxy implementations.
How to Set Up Proxies in PHP
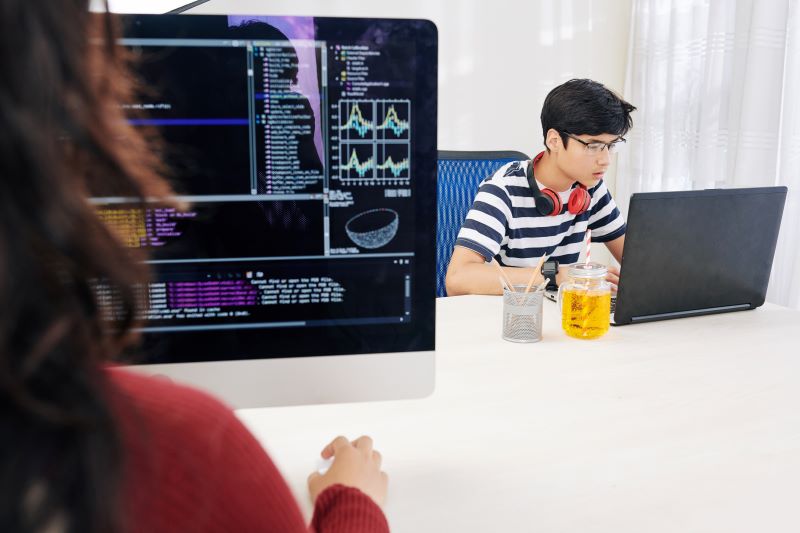
Are you intrigued by the idea of using a powered-by-PHP proxy? It’s easier than you might think to set one up.
There are two main ways to set up proxies in PHP: using cURL (a command-line tool that enables data exchange between a device and a server via a terminal) and using stream contexts (a way to set parameters or options for streams when working with file operations, network connections, or other stream-related operations).
Below is a breakdown of each option:
Using cURL
cURL is a popular library in PHP for making HTTP requests. You can configure cURL to use a proxy server by setting specific options using the curl_setopt function.
Here’s a general outline of what you’ll do if you take this approach:
- Define the proxy server address and port (e.g., “127.0.0.1:8080”).
- Initialize a cURL session using curl_init().
- Set the target URL you want to access.
- Use curl_setopt to set the following options:
- CURLOPT_PROXY to the proxy server address.
- CURLOPT_PROXYPORT to the proxy server port.
- (Optional) Authentication: If the proxy requires a username and password, use CURLOPT_PROXYUSERPWD or CURLOPT_USERPWD to provide credentials.
- Execute the cURL session with curl_exec().
Here’s an example of how the code might look when setting up a PHP cURL proxy:
<?php
// Proxy settings
$proxyHost = ‘proxy.example.com’;
$proxyPort = 8080;
$proxyUsername = ‘username’; // If your proxy requires authentication
$proxyPassword = ‘password’; // If your proxy requires authentication
// Target URL
$url = ‘https://www.example.com’;
// Initialize cURL session
$ch = curl_init();
// Set the target URL
curl_setopt($ch, CURLOPT_URL, $url);
// Set cURL options for proxy
curl_setopt($ch, CURLOPT_PROXY, $proxyHost);
curl_setopt($ch, CURLOPT_PROXYPORT, $proxyPort);
// If proxy requires authentication
curl_setopt($ch, CURLOPT_PROXYUSERPWD, “$proxyUsername:$proxyPassword”);
// Set other options if needed
// For example:
// curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Return the transfer as a string
// Execute the request
$response = curl_exec($ch);
// Check for errors
if ($response === false) {
echo ‘cURL error: ‘ . curl_error($ch);
}
// Close cURL session
curl_close($ch);
// Output the response
echo $response;
?>
Using stream contexts
Another option is to use stream contexts.
You can create a stream context with proxy settings and then use it with functions like file_get_contents to fetch content through the proxy.
The following is a simplified explanation:
- Define an array with proxy details like the server address and protocol (e.g., “tcp://127.0.0.1:8080”).
- Create a stream context using stream_context_create with the proxy options array.
- Use the created stream context with functions like file_get_contents to fetch content through the proxy server.
Here’s an example of how the code might look using stream contexts for a proxy.php script:
<?php
// Proxy settings
$proxyHost = ‘proxy.example.com’;
$proxyPort = 8080;
$proxyUsername = ‘username’; // If your proxy requires authentication
$proxyPassword = ‘password’; // If your proxy requires authentication
// Target URL
$url = ‘https://www.example.com’;
// Proxy URL
$proxyUrl = “tcp://$proxyHost:$proxyPort”;
// Proxy authentication string
$auth = base64_encode(“$proxyUsername:$proxyPassword”);
// Stream context options
$contextOptions = array(
‘http’ => array(
‘proxy’ => $proxyUrl,
‘request_fulluri’ => true,
‘header’ => “Proxy-Authorization: Basic $auth”
),
‘ssl’ => array(
‘verify_peer’ => false, // Depending on your needs, you may need to adjust SSL verification settings
‘verify_peer_name’ => false
)
);
// Create stream context
$context = stream_context_create($contextOptions);
// Make request using file_get_contents() with stream context
$response = file_get_contents($url, false, $context);
// Check for errors
if ($response === false) {
echo ‘Failed to retrieve data from URL’;
} else {
// Output the response
echo $response;
}
?>
PHP Proxy Check Working
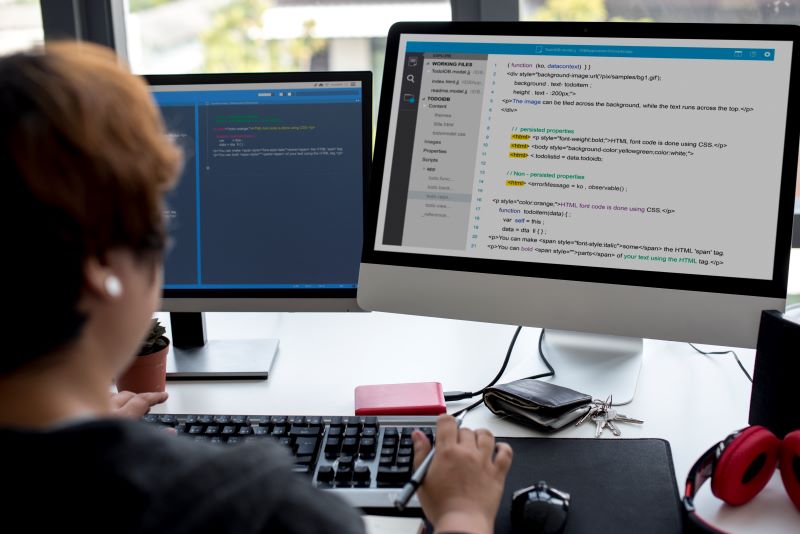
There are a few ways to check if your PHP proxy server is working effectively. The methods listed below are some of the most commonly used options:
Accessing an external resource
Accessing an external resource is a basic test to see if the proxy can route requests and deliver responses. Here is an example of how you might run this test:
- Write a simple PHP script using cURL or file_get_contents
- Set the script to retrieve content from a well-known website like https://www.google.com/ or https://en.wikipedia.org/wiki/Main_Page.
- Configure the script to use your PHP proxy server.
- Execute the script.
If the script retrieves content from the target website and displays it, your proxy server is likely functioning at a basic level.
Verifying IP address
Verifying an IP address allows you to check and see if the proxy is effectively hiding your real IP address. To run this test, follow these steps:
- Visit a website that displays your IP address, like https://www.whatismyip.com/.
- First, access the website without using the proxy. Note down the displayed IP address.
- Then, access the same website using your PHP proxy server. The displayed IP address should be different (the IP of your proxy server).
If the IP addresses differ when using and not using the proxy, your proxy is masking your real IP successfully.
Advanced testing
For a more thorough evaluation, you can consider more advanced options. For example, if your proxy supports various protocols (HTTP/HTTPS), try accessing websites using both to ensure proper functionality.
You can also compare the response times for accessing a website directly and through the proxy. This test can help you identify potential performance bottlenecks.
If your proxy offers content filtering functionalities, you can also test if it’s working as intended by trying to access blocked or allowed websites.
Troubleshooting Proxy Setup in PHP
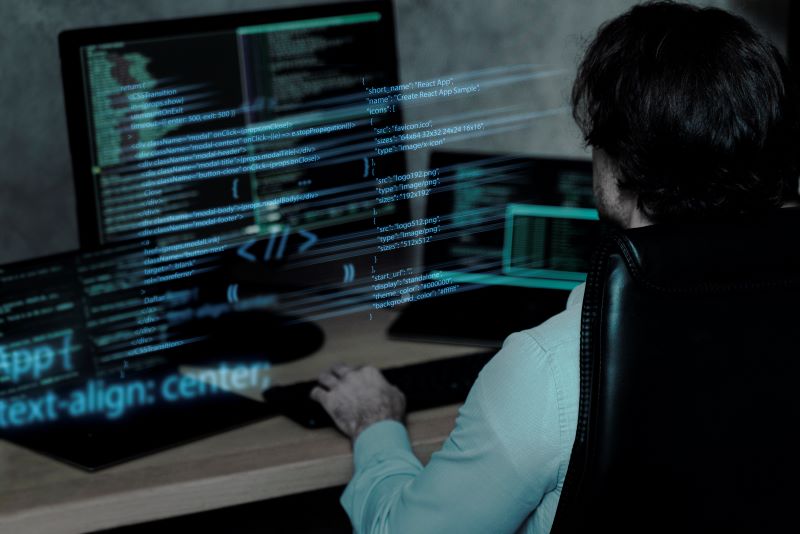
If you run the tests mentioned above and find that your proxy isn’t working as it should, you can take a few different steps to troubleshoot the problem. The following are some of the most effective troubleshooting practices to try:
Verify proxy server details
Double-check the proxy server hostname, port number, and authentication credentials (if required). Ensure they are correctly configured in your PHP script.
Test Proxy Connection:
Verify that the proxy server is accessible from your network. You can use tools like telnet or curl from the command line to test the connection to the proxy server.
Check PHP configuration
Ensure that PHP is properly configured to use proxy settings. Verify that the cURL extension is enabled if you’re using cURL for proxy communication.
Review Stream Context Options:
Check the stream context options in your PHP script. Ensure that the proxy settings are correctly specified and that there are no typos or syntax errors.
Debugging output
Add debugging statements to your PHP script to output relevant information such as error messages, response headers, and response content. This can help you identify where the issue lies.
Enable error reporting
Set error_reporting to E_ALL and display_errors to On in your PHP script or configuration file. This will display any errors or warnings that occur during script execution, including issues related to proxy setup.
Check firewall and network settings
Ensure that there are no firewall rules or network restrictions blocking outgoing connections to the proxy server. Check if the proxy server allows connections from your PHP server’s IP address.
Test direct connection
Temporarily modify your PHP script to bypass the proxy and make a direct connection to the target URL. This can help determine if the issue is with the proxy configuration or something else.
Inspect proxy server logs
Check the logs on the proxy server to see if there are any error messages or indications of failed connections from your PHP script. This can provide insights into potential issues on the proxy server side.
Consult documentation and resources
Refer to the documentation and resources for the proxy server software you’re using. Look for troubleshooting tips and common issues that may affect proxy setup.
Alternatives to Setting Up Proxies in PHP
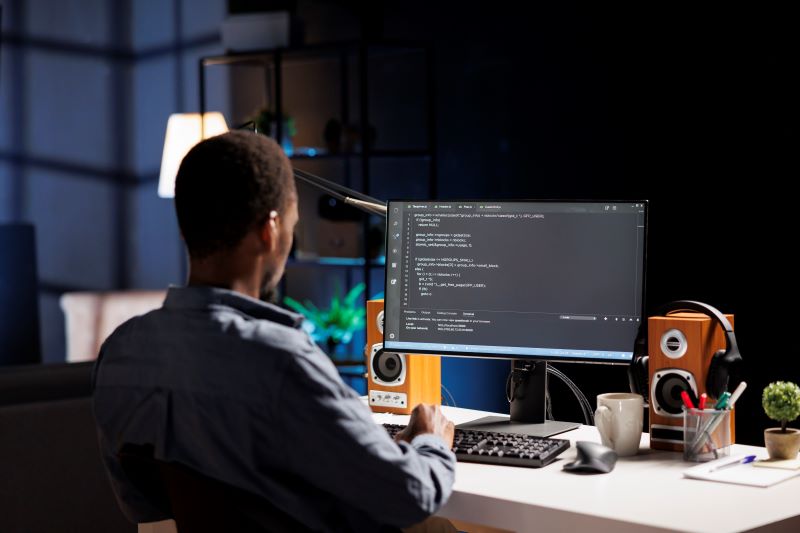
You can certainly use the strategies discussed above to set up proxies in PHP and check that they’re working correctly. If that much coding is a little outside of your comfort zone, though, there is an alternative.
Working with a proxy service allows you to experience all the benefits that proxies provide without having to handle the setup and troubleshooting entirely on your own. Here are some additional advantages that come with using a proxy service:
Easy to use
Proxy services are designed to be user-friendly and accessible to a broad audience, even those without technical expertise.
Most proxy services offer straightforward setup processes. They also offer intuitive interfaces that make it easy to manage your connection.
Round-the-clock customer support
Most proxy services offer some form of customer support, too, such as email ticketing systems, live chat, or knowledge bases. This can be helpful if you encounter any issues during setup or usage.
Multiple proxy options
The most reputable proxy services deliver multiple proxy options, including residential proxies, data center proxies, and ISP proxies. This variety allows you to choose the proxies that work best for your needs and will help you see the best results.
Affordable prices
You don’t have to spend an arm and a leg to get access to reliable proxies. Many proxy services have reasonable prices and even free trials, so you can experiment with their offerings before you make a final decision.
How to Choose a Proxy Service
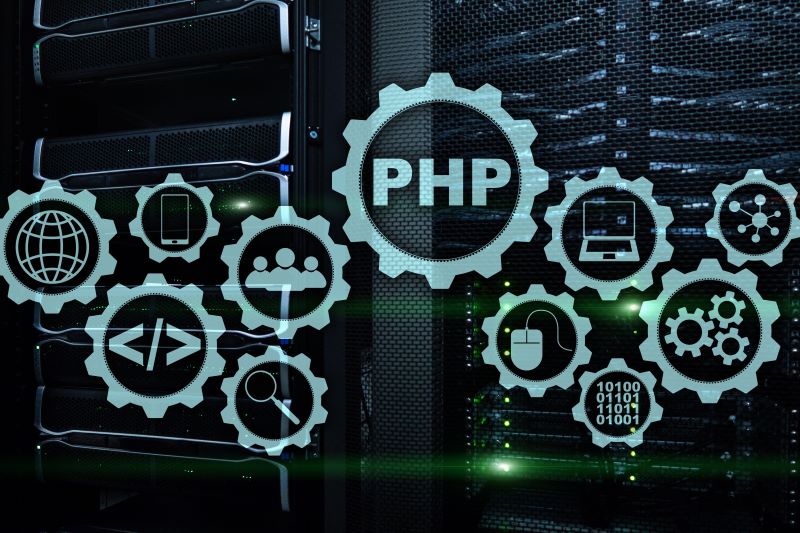
To select the right proxy service, you must consider several different factors to ensure it meets your specific needs. Here’s a breakdown of key points to keep in mind as you make your choice:
Identify your needs
There are several different types of proxies you can choose from, each with their strengths. Do you want the speed of data center proxies? The anonymity of residential proxies? A combination of both (which ISP proxies offer)?
Consider what you’ll be using the proxy for and pick the one that best aligns with your goals (e.g., data center proxies for basic web browsing, residential proxies for enhanced anonymity, etc.). Then, make sure you choose a proxy service that offers that particular proxy type.
Consider proxy pool size and location
A bigger pool of proxy IPs generally offers more choices and reduces the likelihood of getting blocked by websites.
If geo-restriction bypass is your primary concern, it’s also a good idea to choose a provider with servers in desired locations. Consider a mix of countries, states, cities, etc., for broader access, too.
Evaluate performance and reliability
Look for a provider known for fast connection speeds to minimize any browsing slowdowns — nobody wants to deal with those while they’re trying to complete a project. Ensure the service offers high uptime, too, to avoid disruptions while using the proxy.
Consider security and privacy
Choose a service that offers secure encryption to protect your data while it travels through the proxy server. Be wary of providers that log your activity extensively, too, especially if you have concerns about privacy (for many people, that’s the reason they’re using proxies in the first place).
Check additional features and pricing
Some services provide features like bandwidth caps, dedicated proxies, or user authentication. Choose based on your specific requirements.
Proxy services also vary in price. Free proxies often have limitations, especially when it comes to speed and bandwidth. Paid services, on the other hand, tend to offer more features and reliability.
Compare plans and find one that fits your budget. Remember, though, that the cheapest option isn’t always the best.
Review testing and customer support options
Look for providers offering trial periods or money-back guarantees to test the service before committing. This gives you a chance to see how the proxies work and if they meet your needs.
Remember, too, that reliable customer support is crucial in case you encounter any issues with the proxy service. Ideally, you’ll choose a proxy service that offers 24-7 customer support so you can reach a professional at any time.
Final Thoughts
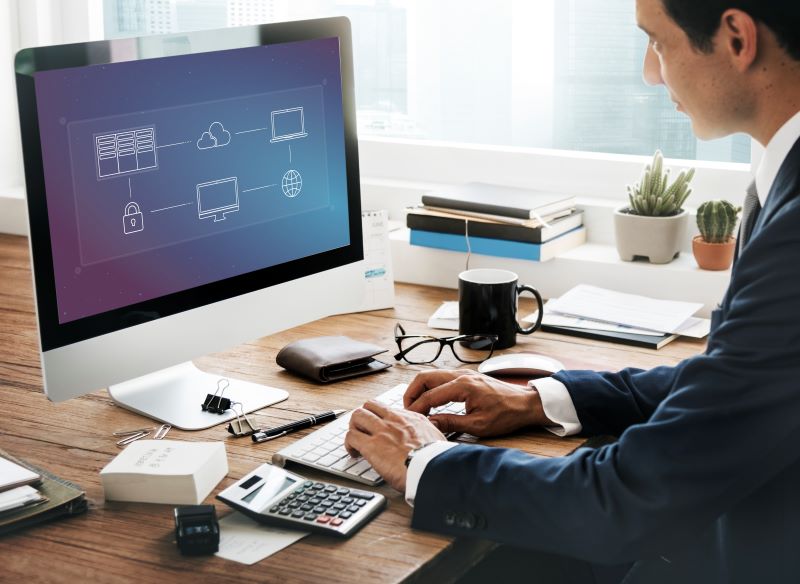
Learning how to set up a powered-by-PHP proxy might seem intimidating initially. If you follow the tips and tricks shared here, though, you’ll have a much easier time navigating this process and figuring out the basics.
If you don’t want to deal with the process of running a PHP proxy check working test, you’re in luck. You can use Rayobyte proxies instead. Rayobyte is an award-winning provider that offers the world’s most reliable proxies for personal and professional use with 24-7 customer support. Sign up today for a free trial.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.
Sign Up for our Mailing List
To get exclusive deals and more information about proxies.
Start a risk-free, money-back guarantee trial today and see the Rayobyte
difference for yourself!