Comparing Fetch() Vs. Axios In Next.Js For HTTP Requests
When it comes to choosing an NPM (node package manager) software package, how do you determine which of the top software is best suited for your organization’s needs? You may be wondering whether to use Fetch() vs. Axios in Next.Js, so let’s get into the comparison.
While professionals around the world rely on Axios and prefer it over built-in APIs for its ease of use, it isn’t always the ideal solution. There may be better options for executing HTTP requests. Oftentimes, developers using Axios have overestimated their organization’s need for the Axios library. When comparing Axios vs. Fetch() API, the ideal solution for your needs could be Fetch(), as this API can reproduce key features of Axios, and it’s accessible on all updated web browsers.
If you’re trying to determine whether to use Fetch() vs. Axios in Next.Js—particularly if you’re using legacy systems—Axios’ capability to run on older browsers, like Internet Explorer 11, is a significant selling point. Axios does this by running XMLHttpRequest. If you need browser support for anything other than Chrome 42+, Safari 10.3+, FIrefox 39+, or Microsoft Edge 14+, you won’t be able to adequately run Fetch().
That said, if your only reason for using Axios is the need for backward support compatibility, you likely don’t need an HTTP library. Instead, you can access Fetch() by using a polyfill that approximates functionalities for web browsers unable to support Fetch(). Learn more about the key differences between Fetch() vs. Axios in Next.Js below.
Axios Js vs. Fetch API
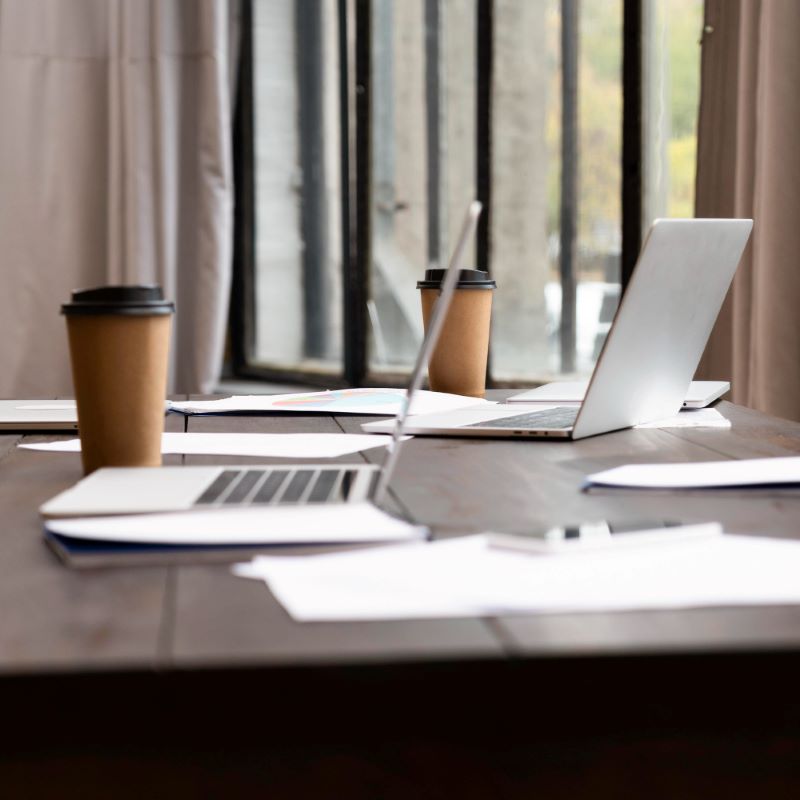
One of the core fundamental tasks of any web application is reliable communication with computer servers by using the HTTP protocol. The only two ways to achieve this with recent NPM software is by using the Fetch API or Axios. If you need to use Next.Js, Axios vs. Fetch() is the decision to consider.
For the sake of clarity regarding Fetch() vs. Axios, React is accessed via Next.Js, allowing developers to create full-stack web applications by extending the latest React features with integrated Rust-based JavaScript tooling. This enables the fastest builds.
Axios
When deciding whether to employ Fetch() vs. Axios in Next.Js, many developers prefer using Axios for its ease of use. Axios is a JavaScript library that you can use to make HTTP requests and XMLHttpRequests from the web browser or node.js. It supports the Promise API that is included as a native API with JS ES6. Users running Axios can also intercept HTTP requests and responses, or cancel requests, along with enabling client-side protection against XSRF (Cross-Site Request Forgery).
What does Axios mean?
Fun fact: Axios is an ancient Greek word meaning “worthy.” This translation aligns with the company’s objective to provide valuable content that is worthy of readers’ time.
Fetch API
On a basic level, Fetch is an API that’s built-in to most modern web browsers, so users don’t need to find and install the software package to use the API. Like Axios, Fetch is a promise-based HTTP client. When comparing Axios vs. Fetch in 2023, it’s important to note that the Fetch API can replicate all of Axios’ core features. Though harder to use in some regards, as a native interface, using Fetch() vs. Axios in Next.Js offers more possibilities.
Fetch() is a method used for beginning the process of fetching a resource from a server. Then, the Fetch() returns a Promise that resolves to a Response object. The Fetch API works by providing a fetch() method defined on the window object. The API also includes a JavaScript interface that’s useful for accessing and manipulating individual parts—requests and responses—of the HTTP pipeline.
To use the fetch() method, there is one mandatory argument: the URL of the resource the user is attempting to fetch. Using this method returns a Promise that you can use to retrieve the response to the user request.
Additional differences between Fetch() vs. Axios in Next.Js
There are quite a few other differences between Fetch() vs. Axios in Next.Js, though both are fully capable of communicating between the web application and web servers.
Axios Js | Fetch() |
URL is in the request object | URL not in the request object |
Easily installed third-party software package | Most modern web browsers have Fetch built-in |
Built-in XSRF (Cross-Site Request Forgery) protection | No XSRF protection |
Functions by using the data property | Functions by using the body property |
Data contains the object | Request body needs to be stringified |
JSON data is automatically transformed | JSON data is handled in two steps: first, making the request; second, call the .json() method on the response |
Can easily cancel requests and request timeout | Cannot easily cancel request and request timeout |
Can readily intercept HTTP requests with default functionality | Doesn’t contain a default method for intercepting requests |
Download progress can be monitored | Does not contain download progress, though a workaround can be implemented |
Wide browser support, even on older browsers | Only supported by Chrome 42+, Firefox 39+, Edge 14+, Safari 10.1+ |
Data content ignored by “GET” call | Can contain body content in “GET” call |
Benefits of Axios vs. Fetch API
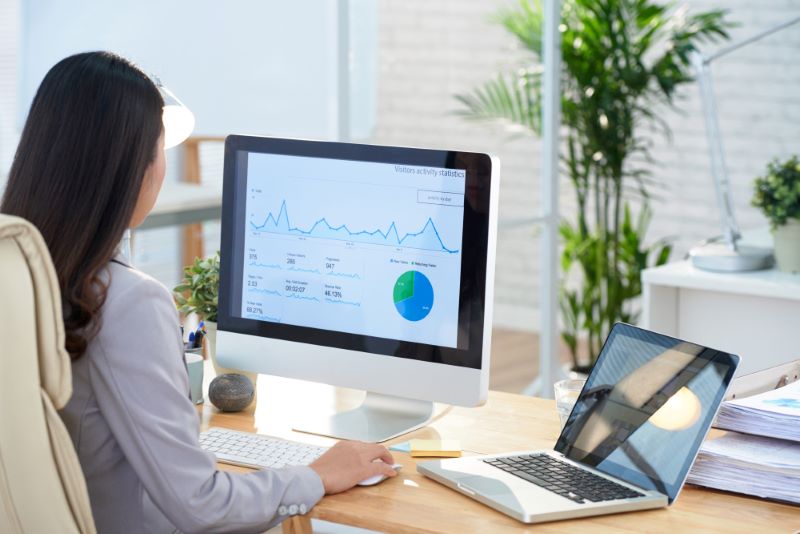
As you decide whether to use Fetch() vs. Axios in Next.Js, there are a few benefits of each to consider.
Setting the response timeout property
One of the biggest advantages of using Axios is how simple it is to set a response timeout property in the config object to the number of milliseconds Axios will run the request before it is aborted. Conversely, Fetch() vs. Axios in Next.Js provides users with a similar response timeout functionality by using the AbortController interface within the API, though it’s not as simple of a method as setting the Axios response timeout property.
Automatic transformation of data
Both Fetch() and Axios allow the automatic transformation of JSON data, but with Axios, the data is automatically stringified when sending requests. With Fetch(), users must implement automatic JSON data transformation by manually inputting the correct code. Overall, for the automatic transformation of JSON data, there isn’t a big difference between Fetch() vs. Axios in Next.Js.
HTTP interceptors
Another benefit of Axios in the Fetch() vs. Axios in Next.Js debate is its key feature that allows users to intercept HTTP requests. Intercepting HTTP requests is useful when you must change or examine HTTP requests between your application and the server, or the server to your application. Example situations include logging, authenticating, and retrying an HTTP request.
When using interceptors, it’s not necessary to write separate code for each individual HTTP request. HTTP interceptors are also helpful when organizations need to set a global strategy for handling requests and responses.
When using Fetch() vs. Axios in Next.Js, users have to deal with the lack of a default method for intercepting requests, though a workaround isn’t overly complicated or difficult to initiate. Users can overwrite the global Fetch() method and define their own interceptor with a few lines of code.
fetch = (originalFetch => {
return (…arguments) => {
const result = originalFetch.apply(this, arguments);
return result.then(console.log(‘Request was sent’));
};
})(fetch);
fetch(‘https://api.github.com/orgs/axios’)
.then(response => response.json())
.then(data => {
console.log(data)
});
Implementing a progress indicator
In the past, JavaScript programmers implemented a progress indicator for large assets with the XMLHttpRequest.onprogress callback handler. Fetch() doesn’t include an onprogress handler. However, users can enable an instance of ReadableStream via the response object’s body property.
Fortunately for Axios users, implementing a progress indicator is simpler and can be enabled via the Axios Progress Bar module. The code uses the FileReader API to read the downloaded image and then returns the image’s data as a Base64-encoded string. Next, users insert that string into the src attribution under the img tag, displaying the image. This simplicity can be a dealbreaker when deciding between Fetch() vs. Axios in Next.Js
Simultaneous requests
When it comes to Fetch() vs. Axios in Next.Js, both allow users to make simultaneous requests with relative ease. For Axios, users can pass an array of requests to the axios.all() method, then use axios.spread() to separate variables by assigning the properties of the response array.
For those using Fetch, users can achieve the same results of simultaneous requests. Simply pass all fetch requests as an array to the built-in Promise.all() method. Then, handle the fetch responses with an async function.
NPM (Node Package Manager) Comparisons Beyond Axios Js vs. Fetch
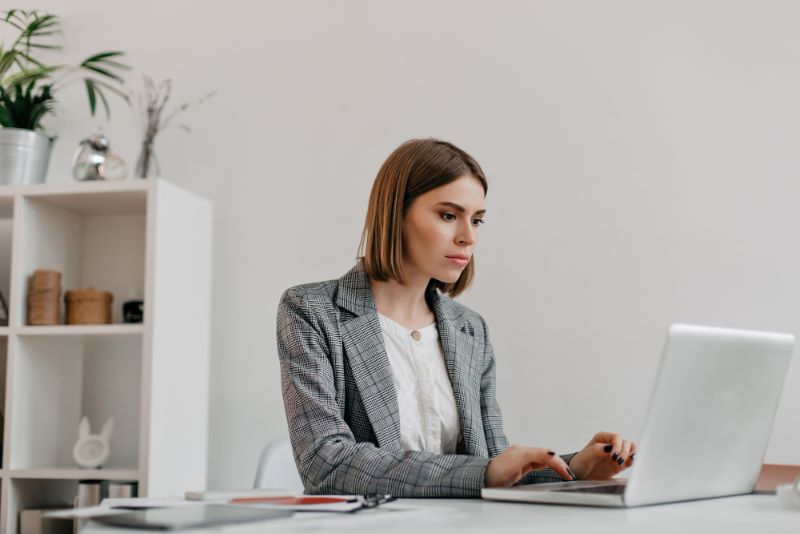
For the sake of providing a full picture of comparable software packages, examining Axios vs. Fetch in 2023 isn’t the only comparison we should cover, as plenty of users use other NPM software.
Node-fetch
Node-fetch enables users to access Fetch API directly using minimal code for a window.fetch compatible API on Node.Js. How does node-fetch vs. Axios’ performance compare? Compared to Axios, node-fetch has more updates and more weekly downloads.
Request
Request is a simplified HTTP request client authored by Mikeal Rogers in January 2011. How does node-fetch vs. Axios vs. request compare? For one, request has been out a lot longer than any of the other NPM software packages and has more versions than the others. However, it was deprecated in 2020.
Request-promise and request-promise-native
As extensions of request, request-promise and request-promise-native are simplified HTTP request clients. Because they depends on request, request-promise and request-promise-native are also deprecated, and there will likely be no new changes.
Summing up NPMs
According to data provided by GitHub, Axios is currently the most popular NPM software package with 102,000 Stars, more followers than the others, and more forks. That said, node-fetch has more frequent updates than Axios, along with more downloads.
As users can reproduce the key features of Axios while using Fetch, ultimately, the superior NPM software package primarily depends on whether you are comfortable working with built-in APIs or prefer the ease of use provided by Axios.
If you’re considering Fetch() vs. Axios in Next.Js or other NPM software packages, you could likely benefit from professionally hosted proxy providers and web scraping APIs. Rayobyte offers the world’s most reliable proxies. You can avoid blocks and bans with access to hundreds of thousands of IPs, 20+ petabytes per month of bandwidth, and over 25 proxy locations. Rayobyte offers residential proxies, ISP proxies, data center proxies, and mobile proxies.
Rayobyte’s residential proxies give you the highest authority and an unmatched commitment to ethics for larger projects—regardless of whether you choose Fetch() vs. Axios in Next.Js. ISP proxies have the authority of residential proxies and the speed of data center proxies when applied to non-scraping use cases. Rayobyte’s data center proxies provide 99% uptime and swift operating speed for countless use cases. If you need mobile proxies, Rayobyte provides continuous internet connectivity with seamless browsing experiences for web scraping projects.
Deciding Between Fetch() vs. Axios in Next.Js
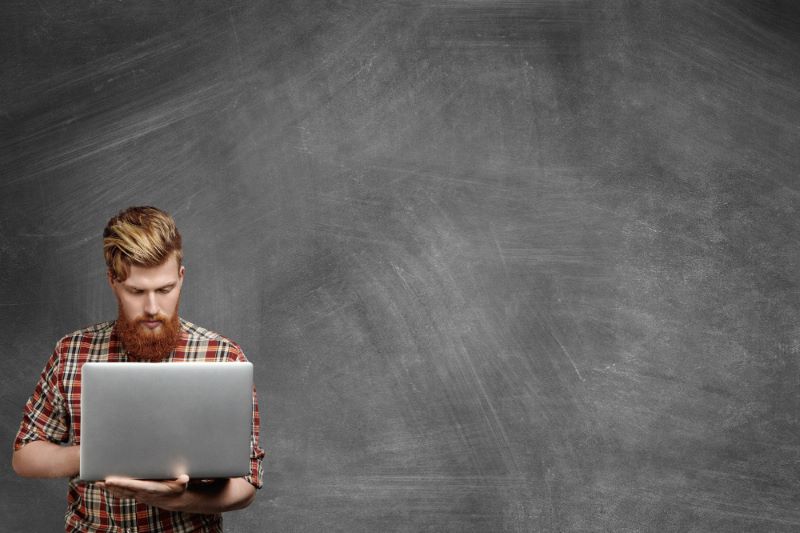
At the end of the day, Fetch() vs. Axios in Next.Js are nearly identical in functionality. The main differences come down to your personal preferences regarding each software package’s features, plus how comfortable you are working with built-in APIs. Both Fetch and Axios return a promise that is either resolved or rejected. Users that prefer Axios tend toward it because of its ease of use. Fetch can be more challenging for inexperienced users.
No matter if you’re using Fetch() vs. Axios in Next.Js, Scraping Robot enables users of both methods to easily scrape websites into JSON. Scraping Robot’s system was built by developers for developers and operates with a plug-and-play functionality. With Scraping Robot, you’ll be up and running in minutes, and all APIs provide structured JSON output of metadata from a parsed website. With Scraping Robot, you’ll no longer need to worry about the difficulties of web scraping, such as proxy management and rotation, browser scalability, server management, CAPTCHA solving, and avoiding anti-scraping measures running on target websites.
By partnering with Scraping Robot and Rayobyte, your organization gets secure proxies that are ideal no matter which NPM you choose, plus advanced data analytics. Check out our products to learn more.
The information contained within this article, including information posted by official staff, guest-submitted material, message board postings, or other third-party material is presented solely for the purposes of education and furtherance of the knowledge of the reader. All trademarks used in this publication are hereby acknowledged as the property of their respective owners.