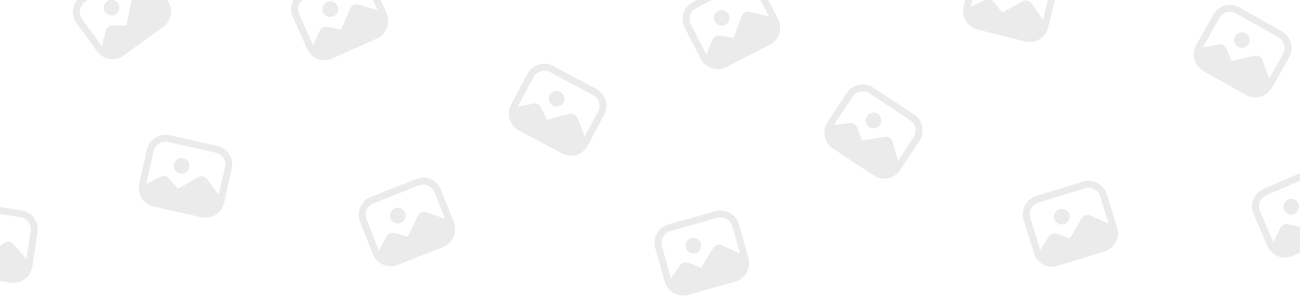
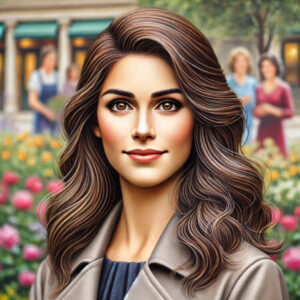
@ShyamalaLaura
Shyamala Laura
I find peace and joy in botanical art and sketching.
Please confirm you want to block this member.
You will no longer be able to:
Please note: This action will also remove this member from your connections and send a report to the site admin. Please allow a few minutes for this process to complete.
Notifications