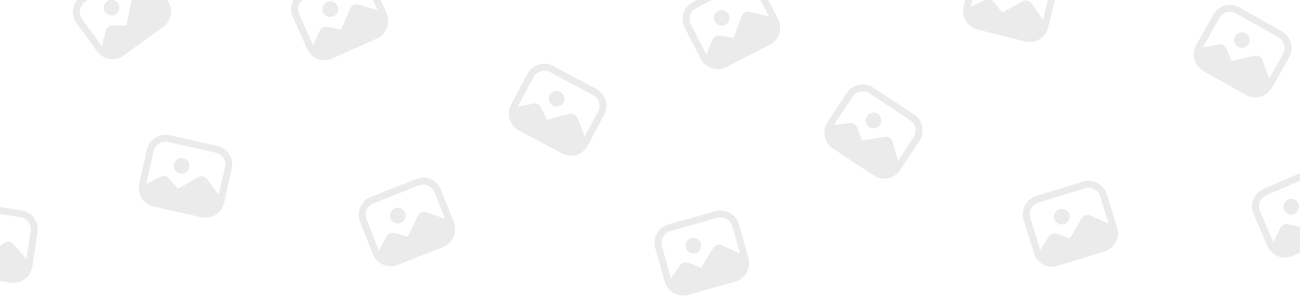
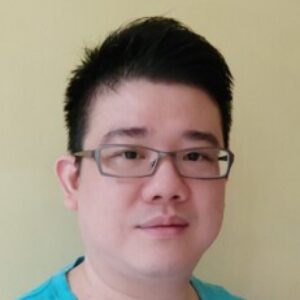
Michael Woo
Forum Replies Created
-
Michael Woo
Administrator01/01/2025 at 12:32 pm in reply to: How to scrape restaurant data from DoorDash.com using Python?Adding pagination handling is crucial for collecting data from all restaurant listings on DoorDash. Restaurants are often distributed across multiple pages, and automating navigation ensures a complete dataset. Random delays between requests mimic human browsing behavior and reduce detection risks. Pagination functionality enhances the scraper’s ability to gather comprehensive data for analysis. This makes the scraper more effective in collecting insights from a large number of listings.
-
Michael Woo
Administrator01/01/2025 at 12:31 pm in reply to: Scraping flight details using Go for performance efficiencyI use Go’s goroutines to scrape multiple endpoints simultaneously, ensuring high performance even for large datasets. Adding proper error handling ensures smooth operation.
-
Michael Woo
Administrator01/01/2025 at 12:29 pm in reply to: Use Go to scrape product categories from Media Markt PolandSaving the scraped categories to a database or file, such as JSON or CSV, would make the data easier to analyze and integrate with other systems. This would be particularly useful for building a product classification system.
-
Michael Woo
Administrator01/01/2025 at 12:27 pm in reply to: How to scrape electronics prices from Euronics.de using JavaScript?Adding advanced error logging to the scraper enhances its functionality. Detailed logs provide insights into issues encountered during scraping, such as failed requests or missing elements. This information helps in refining the script and ensuring reliable operation. Combining logs with automated retries for failed requests improves the scraper’s overall success rate. These features make the scraper more dependable and efficient.
-
Michael Woo
Administrator12/04/2024 at 2:20 pm in reply to: How can I scrape travel deals from JTB Japan using Java?To scrape travel deals from JTB Japan using Java, you can use the popular Jsoup library, which simplifies HTML parsing and web scraping in Java. Below is a basic example of how to scrape the data from a webpage (in this case, JTB Japan), keeping in mind that you need to comply with their robots.txt and any legal guidelines when scraping.
Prerequisites:- Add Jsoup dependency to your project. If you’re using Maven, add the following to your `pom.xml`
<dependency> <groupId>org.jsoup</groupId> <artifactId>jsoup</artifactId> <version>1.15.3</version> <!-- Make sure to check for the latest version --> </dependency>
2. You will need to adjust the URL and HTML parsing logic according to the actual structure of the JTB Japan webpage and the specific travel deals you want to scrape.
import org.jsoup.Jsoup; import org.jsoup.nodes.Document; import org.jsoup.nodes.Element; import org.jsoup.select.Elements; import java.io.IOException; public class JTBTravelDealsScraper { public static void main(String[] args) { // URL of the JTB Japan travel deals page String url = "https://www.jtb.co.jp/travel-deals"; // Replace with actual URL of deals try { // Connect to the URL and fetch the HTML document Document doc = Jsoup.connect(url).get(); // Example: Scraping travel deal titles, assuming they are in <h2> tags with class "deal-title" Elements dealTitles = doc.select("h2.deal-title"); // Update the selector based on actual HTML System.out.println("Travel Deals from JTB Japan:"); // Loop through and print the travel deal titles for (Element title : dealTitles) { System.out.println("Deal: " + title.text()); } // Optionally, you could scrape more info like prices or URLs: Elements dealLinks = doc.select("a.deal-link"); // Update this selector accordingly for (Element link : dealLinks) { String dealUrl = link.attr("href"); System.out.println("More Info: " + dealUrl); } } catch (IOException e) { System.out.println("Error connecting to the page: " + e.getMessage()); } } }
- Jsoup: A Java library used to parse HTML and extract data from web pages.
- URL: Replace the `url` variable with the actual URL of the JTB Japan travel deals page.
- Selectors: You’ll need to inspect the page source (using browser developer tools) to find the correct CSS selectors for the elements you want to scrape, such as titles, prices, and URLs.
- Error Handling: The `IOException` is caught to handle issues like network errors or invalid URLs.
-
Michael Woo
Administrator10/31/2024 at 1:16 pm in reply to: What’s the best tool for scraping JavaScript-heavy websites?Selenium works too, but it’s slower and can be more cumbersome for complex JavaScript interactions.
-
Michael Woo
Administrator02/21/2025 at 3:12 pm in reply to: How to scrape news headlines from a news aggregator website?Normally, mobile proxies or residential proxies would work best, as these are purely actual mobile or residential IPs – how is your scraping project going? 🙂