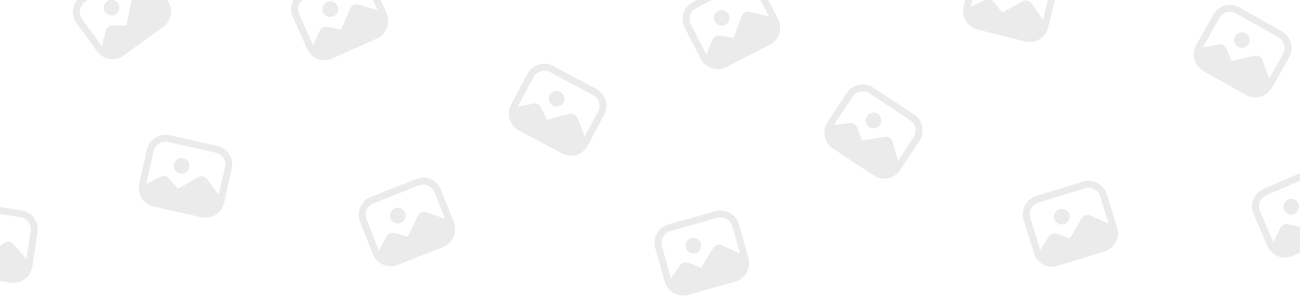
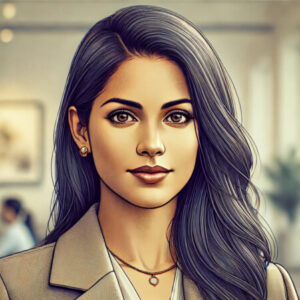
@JerilynShankar
Jerilyn Shankar
I’m deeply passionate about discovering and appreciating urban art.
Please confirm you want to block this member.
You will no longer be able to:
Please note: This action will also remove this member from your connections and send a report to the site admin. Please allow a few minutes for this process to complete.
Notifications